Arduino Nano ESP32 - MG996R
In this tutorial, we are going to learn how to use the MG996R high-torque servo motor with Arduino Nano ESP32.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of Servo Motor
The MG996R servo motor is a high-torque servo motor capable of lifting up to 15kg in weight. The motor can rotate its handle from 0° to 180°, providing precise control of angular position. For basic information about servo motors, please refer to the Arduino Nano ESP32 - Servo Motor tutorial.
Pinout
The MG996R servo motor used in this example includes three pins:
- VCC pin: (typically red) needs to be connected to VCC (4.8V – 7.2V)
- GND pin: (typically black or brown) needs to be connected to GND (0V)
- Signal pin: (typically yellow or orange) receives the PWM control signal from an ESP32's pin.
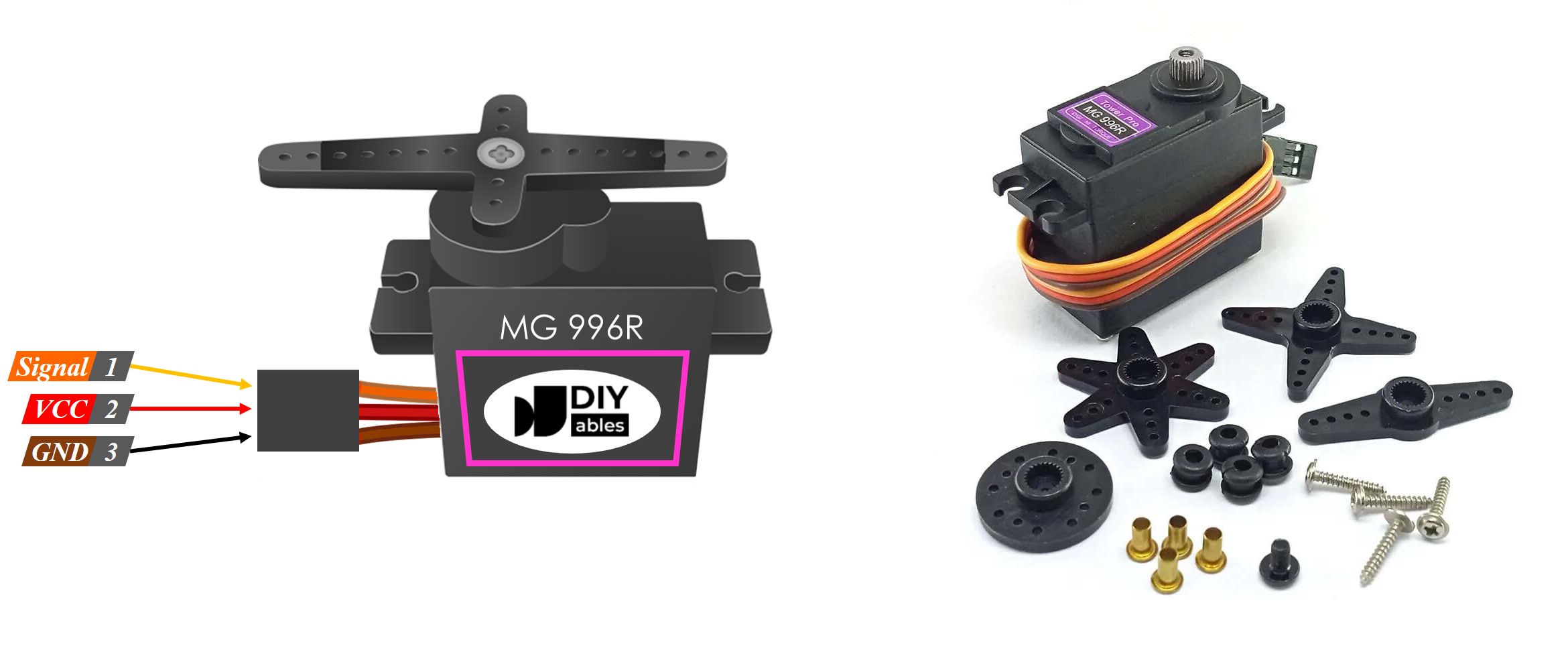
Wiring Diagram
Since the MG996R is a high-torque servo motor, it draws a lot of power. We should not power this motor via the 5v pin of Arduino Nano ESP32. Instead, we need to use the external power supply for the MG996R servo motor.
- When powering the Arduino Nano ESP32 board via USB port.
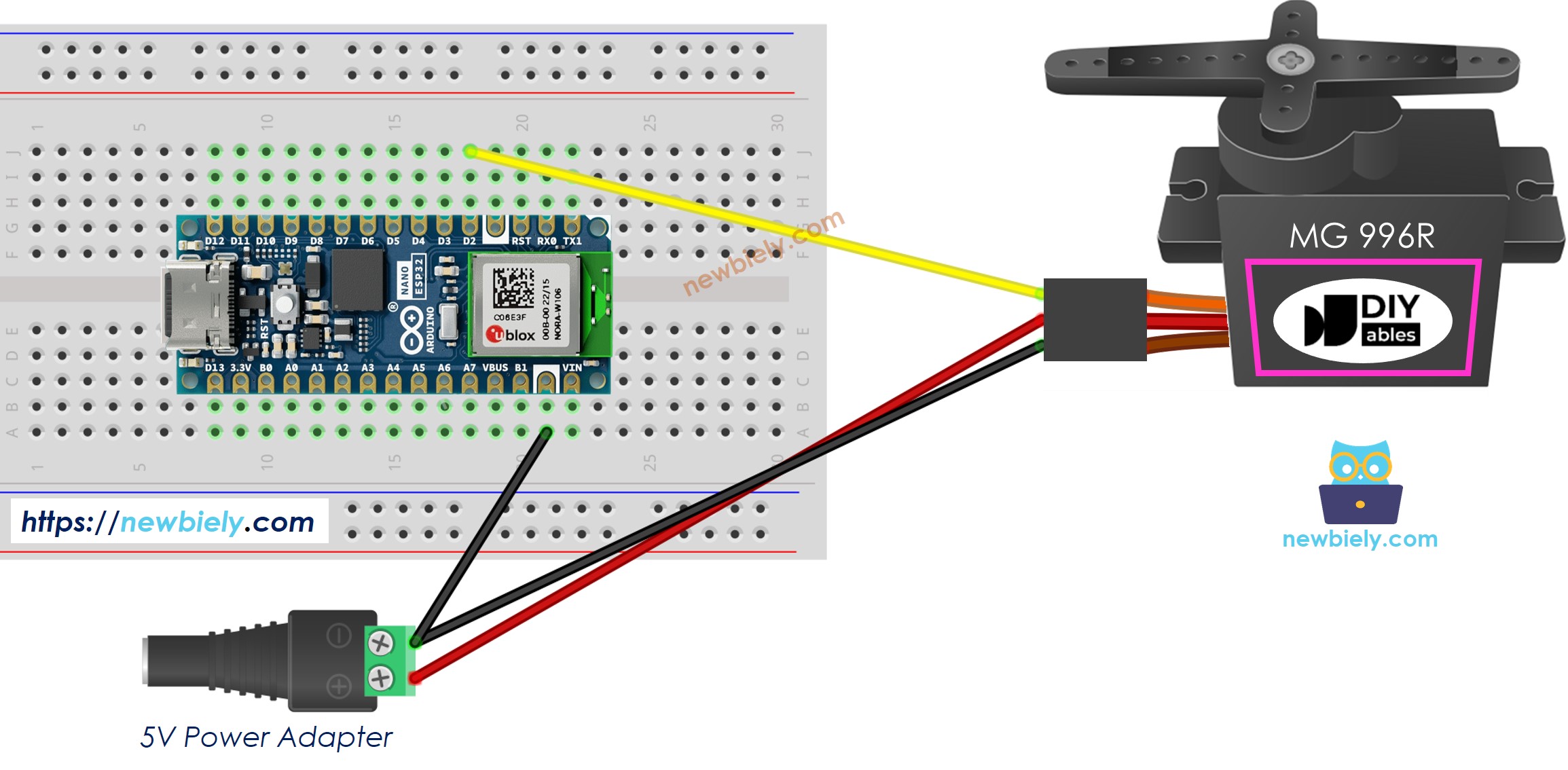
This image is created using Fritzing. Click to enlarge image
- When powering the Arduino Nano ESP32 board via Vin pin.
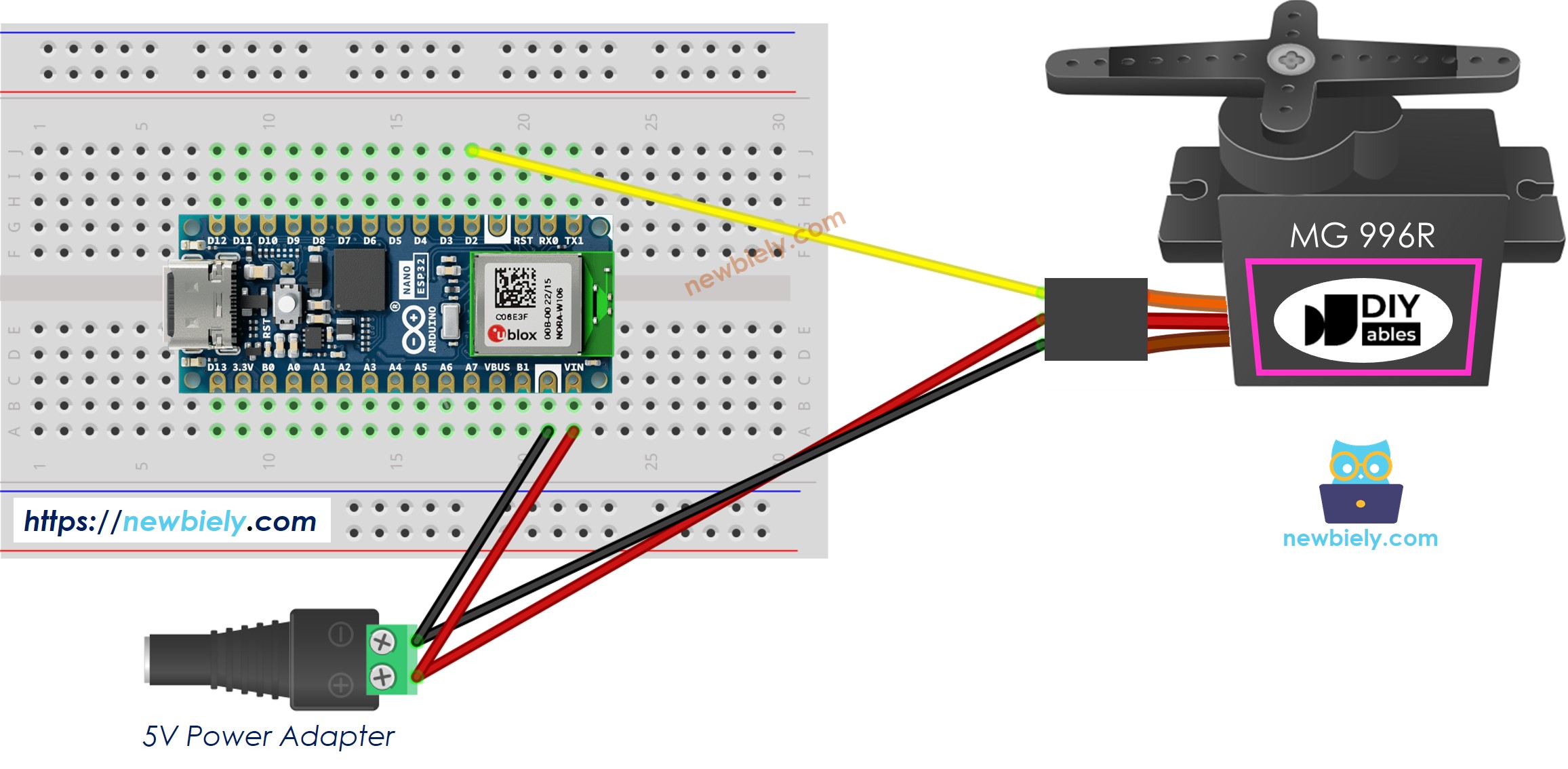
This image is created using Fritzing. Click to enlarge image
Arduino Nano ESP32 Code
Detailed Instructions
To get started with Arduino Nano ESP32, follow these steps:
- If you are new to Arduino Nano ESP32, refer to the tutorial on how to set up the environment for Arduino Nano ESP32 in the Arduino IDE.
- Wire the components according to the provided diagram.
- Connect the Arduino Nano ESP32 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano ESP32 board and its corresponding COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Type ServoESP32 on the search box, then look for the servo library by Jaroslav Paral. Please be aware that both version 1.1.1 and 1.1.0 are affected by bugs. Kindly choose a different version.
- Click Install button to install servo motor library for Arduino Nano ESP32.
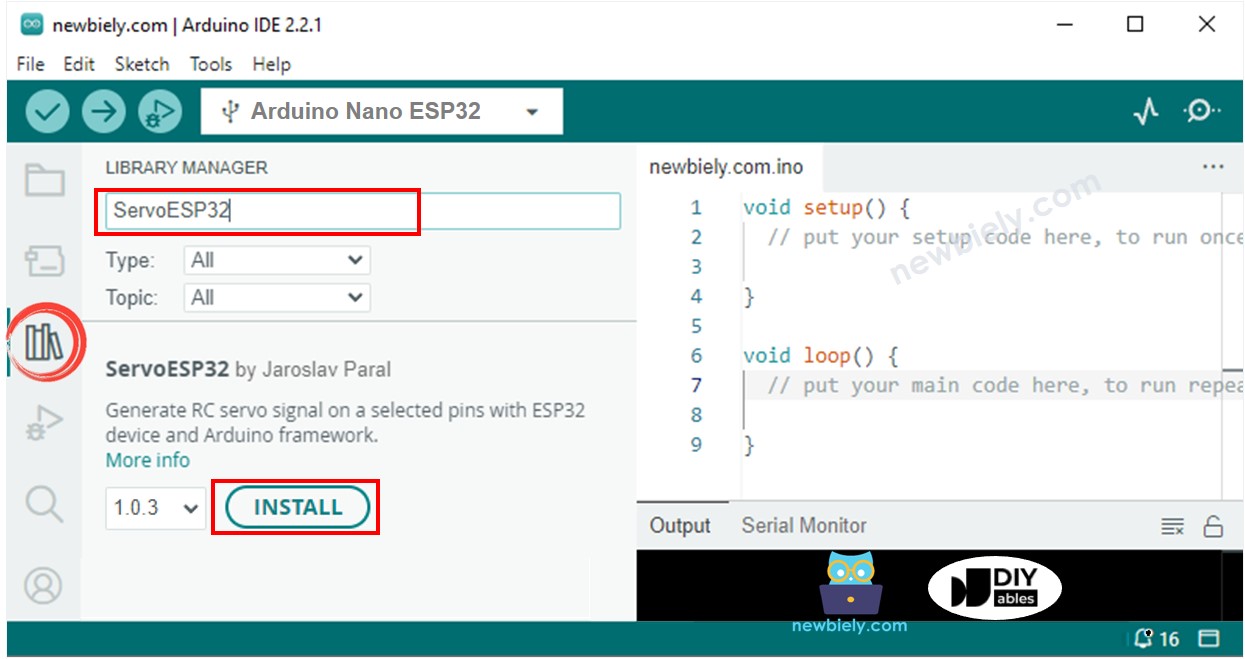
- Connect Arduino Nano ESP32 to PC via USB cable
- Open Arduino IDE, select the right board and port
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino Nano ESP32
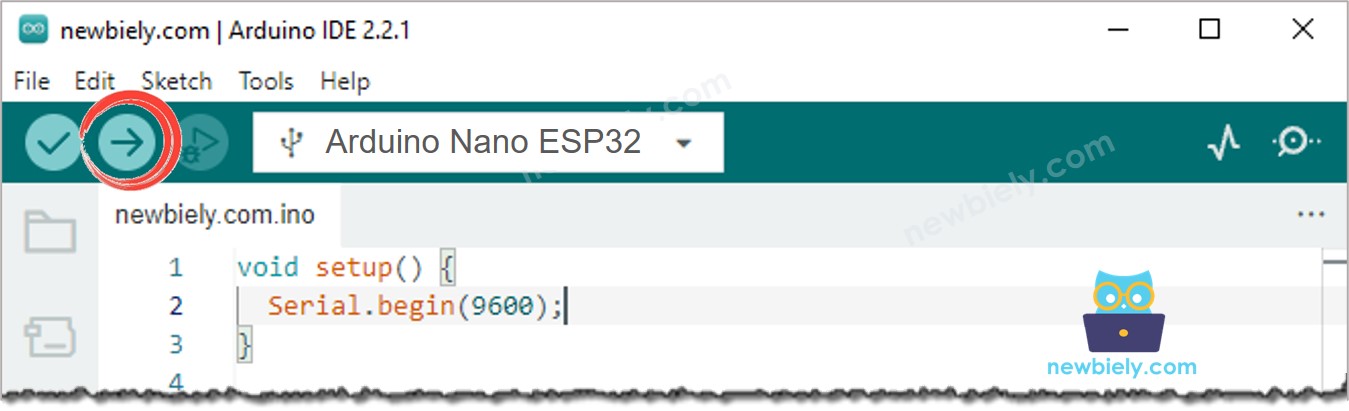
- See the result: Servo motor rotates slowly from 0 to 180° and then back rotates slowly from 180 back to 0°
Code Explanation
Read the line-by-line explanation in comment lines of code!
How to Control Speed of Servo Motor
By using map() and millis() functions, we can control the speed of servo motor smoothly without blocking other code