Arduino Nano ESP32 - Obstacle Sensor
This tutorial instructs you on how to use Arduino Nano ESP32 with the infrared obstacle avoidance sensor to detect the presence of the obstacle.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of IR Obstacle Avoidance Sensor
The IR (infrared) obstacle sensor is used to if any obstacle is present in front of the sensor module by using the IR signal. The detection range is from 2cm to 30cm and is adjustable by a built-in potentiometer.
Pinout
An IR obstacle avoidance sensor has three pins:
- GND pin: connect this pin to GND (0V)
- VCC pin: connect this pin to VCC (5V or 3.3v)
- OUT pin: is an output pin: LOW if an obstacle is present, HIGH if no obstacle is present. This pin needs to be connected to ESP32's input pin.
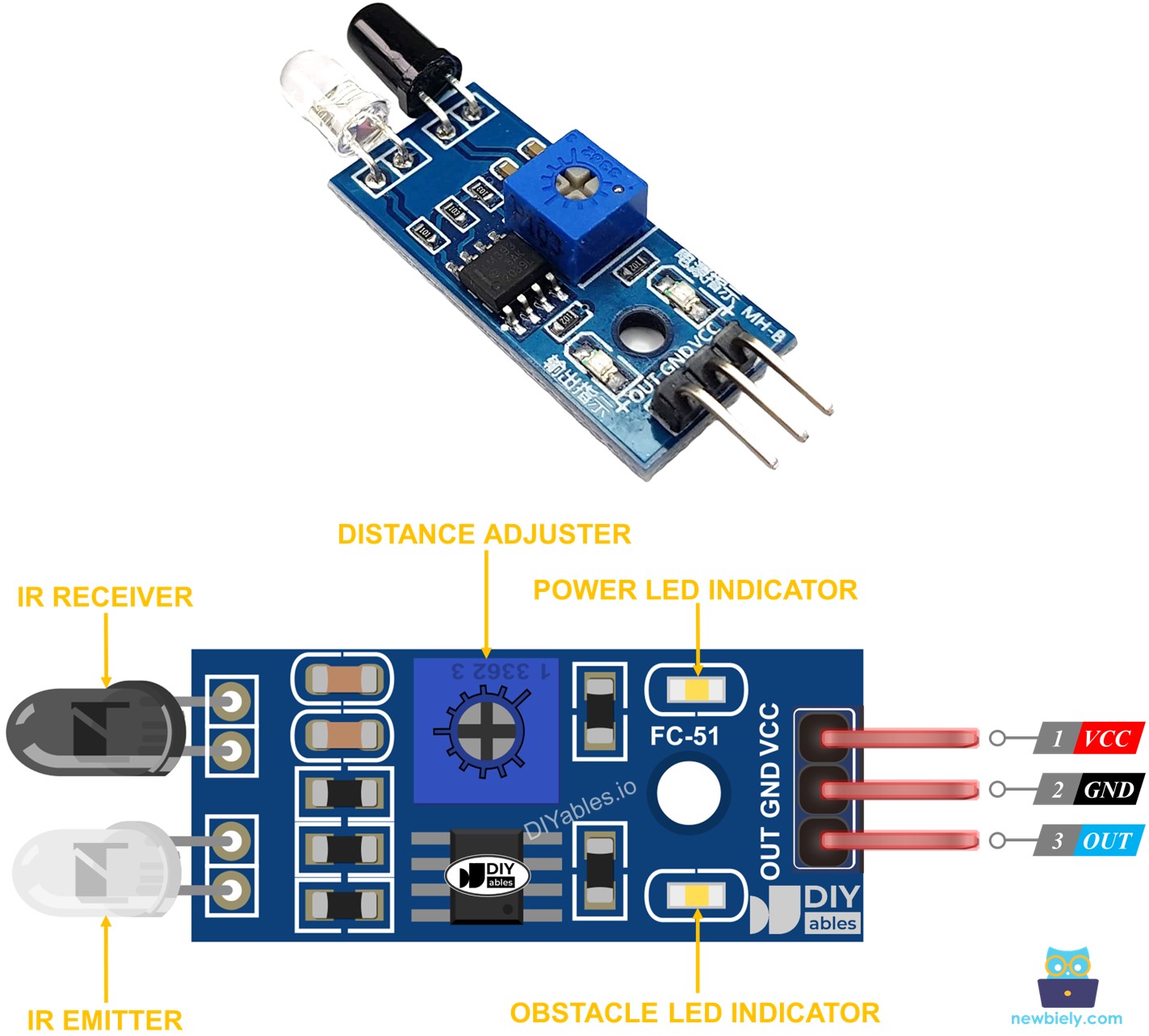
How It Works
An infrared obstacle sensor module consists of an IR transmitter and an IR receiver. The IR transmitter emits the IR signal while the IR receiver searches for the reflected IR signal to determine if the object is present or not. The presence of obstacle is reflected in the OUT pin:
- If the obstacle is present, the sensor's OUT pin is LOW
- If the obstacle is NOT present, the sensor's OUT pin is HIGH
※ NOTE THAT:
During shipment, the sensor may get deformed, which can cause it to malfunction. If the sensor is not working properly, adjust the IR transmitter and receiver to ensure they are parallel.
Wiring Diagram
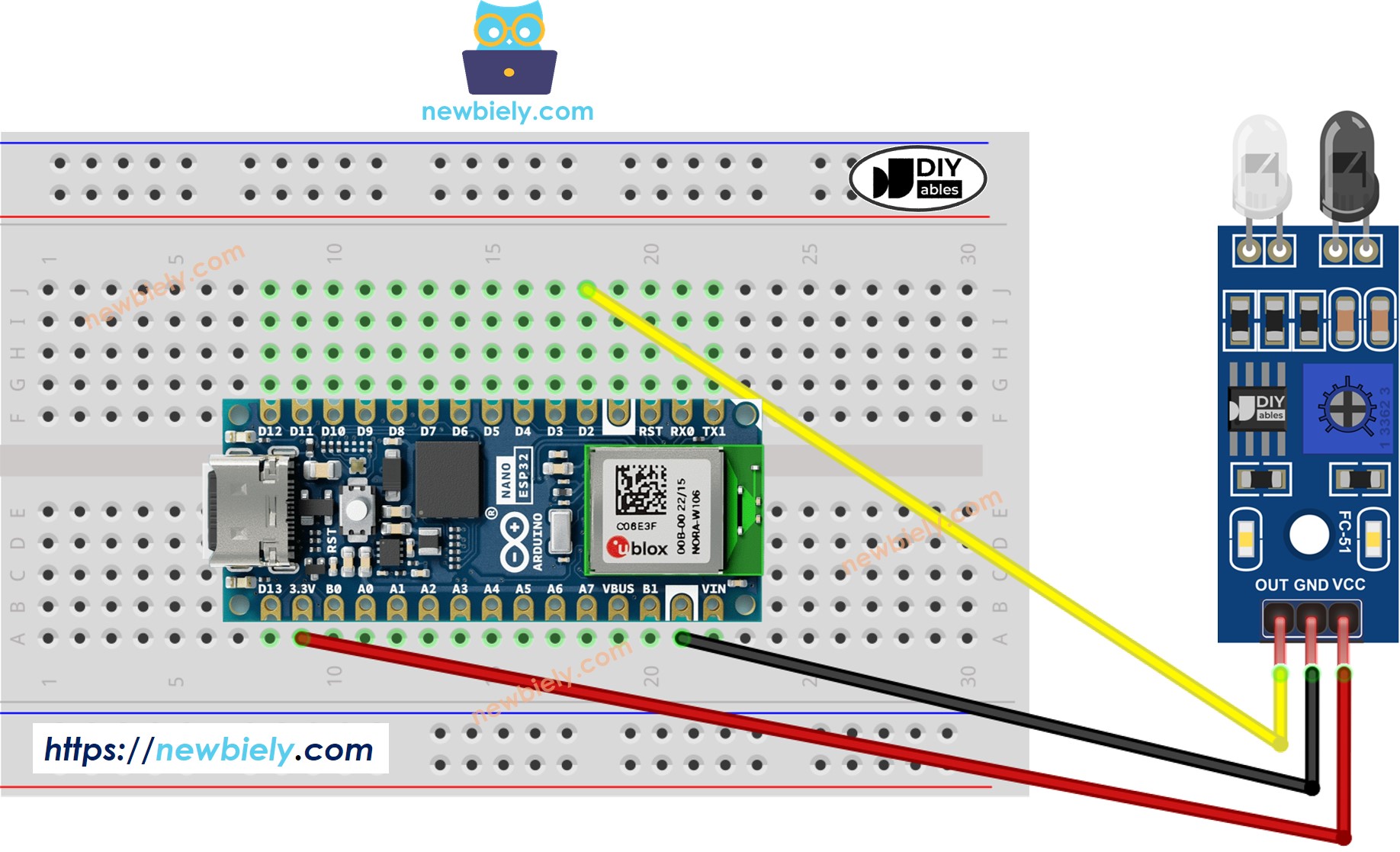
This image is created using Fritzing. Click to enlarge image
How To Program For IR Obstacle Avoidance Sensor
- Initializes the Arduino Nano ESP32 pin to the digital input mode by using pinMode() function. For example, pin D2
- Reads the state of the Arduino Nano ESP32 pin by using digitalRead() function.
Arduino Nano ESP32 Code
There are two use cases to implement an obstacle avoidance application:
- Take actions while the obstacle is present or not present
- Take actions when the obstacle is detected or cleared
Arduino Nano ESP32 code for checking if the obstacle is present
Detailed Instructions
- If this is the first time you use Arduino Nano ESP32, see how to set up environment for Arduino Nano ESP32 on Arduino IDE.
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to Arduino Nano ESP32 board by clicking Upload button on Arduino IDE
- Place an obstacle in front of the sensor for a while, and then withdraw it.
- Check out the result on the Serial Monitor. It looks like the below:
Arduino Nano ESP32 code for detecting obstacle
Detailed Instructions
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to Arduino Nano ESP32 board by clicking Upload button on Arduino IDE
- Place an obstacle in front of the sensor for a while, and then withdraw it.
- Check out the result on the Serial Monitor. It looks like the below: