Arduino Nano ESP32 - RTC
In this guide, we are going to learn how to use Arduino Nano ESP32 with DS3231 Real-Time Clock Module. In detail, we'll cover the following topics:
- How to connect the DS3231 RTC module to Arduino Nano ESP32.
- How to program Arduino Nano ESP32 to read date and time from the DS3231 RTC module (second, minute, hour, day, date, month, and year)
Hardware Preparation
1 | × | Arduino Nano ESP32 | |
1 | × | USB Cable Type-C | |
1 | × | Real-Time Clock DS3231 Module | |
1 | × | CR2032 battery | |
1 | × | Jumper Wires | |
1 | × | Breadboard | |
1 | × | (Optional) DC Power Jack | |
1 | × | (Recommended) Screw Terminal Adapter for Arduino Nano |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of Real-Time Clock DS3231 Module
Arduino Nano ESP32 itself has some time-related functions such as millis(), micros(). However, they can not provide the date and time (seconds, minutes, hours, day, date, month, and year). To get date and time, we needs to use a Real-Time Clock (RTC) module such as DS3231, DS1370. DS3231 Module has higher precision than DS1370. See DS3231 vs DS1307
Pinout
Real-Time Clock DS3231 Module includes 10 pins:
- 32K pin: outputs the stable(temperature compensated) and accurate reference clock.
- SQW pin: outputs a nice square wave at either 1Hz, 4kHz, 8kHz or 32kHz and can be handled programmatically. This can further be used as an interrupt due to alarm condition in many time-based applications.
- SCL pin: is a clock pin for I2C interface.
- SDA pin: is a data pin for I2C interface.
- VCC pin: supplies power for the module. It can be anywhere between 3.3V to 5.5V.
- GND pin: is a ground pin.
For normal use, it needs to use 4 pins: VCC, GND, SDA, SCL
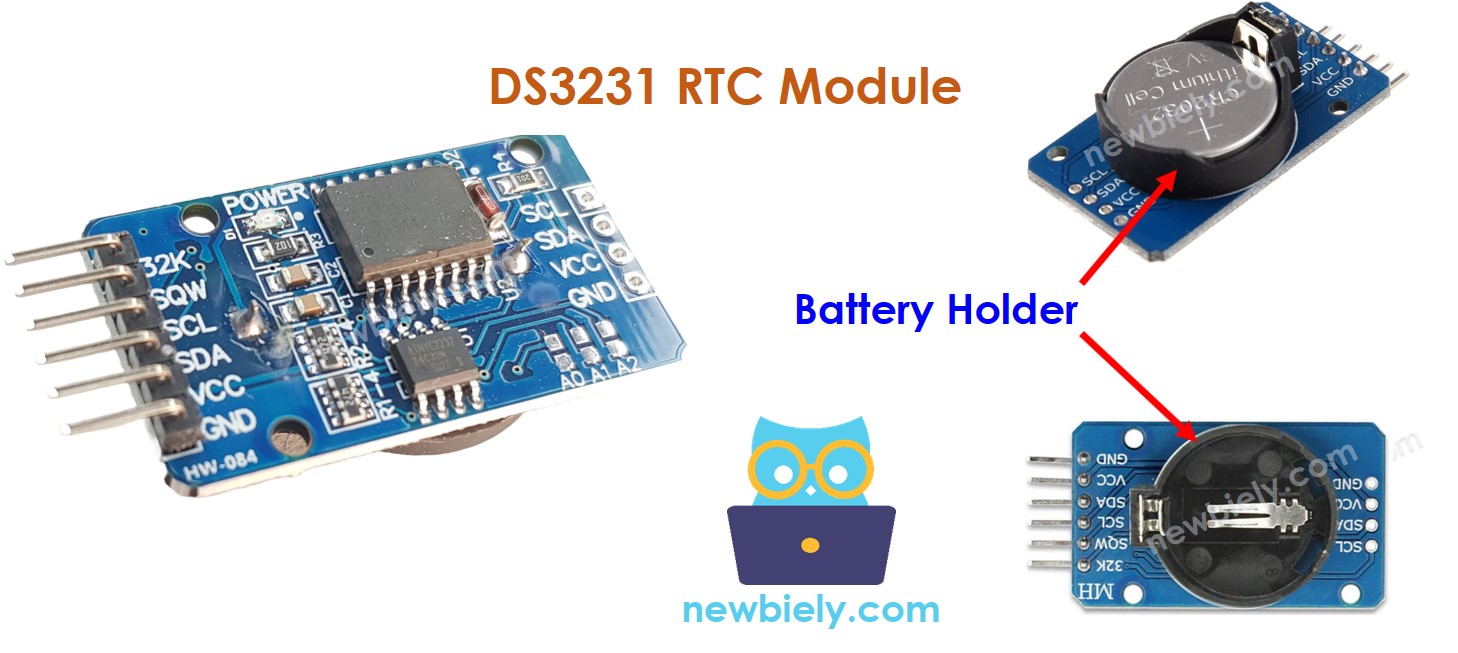
The DS3231 Module also has a battery holder.
- If we insert a CR2032 battery, It keeps the time on module running when the main power is off.
- If we do not insert the battery, the time information is lost if the main power is off and you need to set the time again.
Wiring Diagram
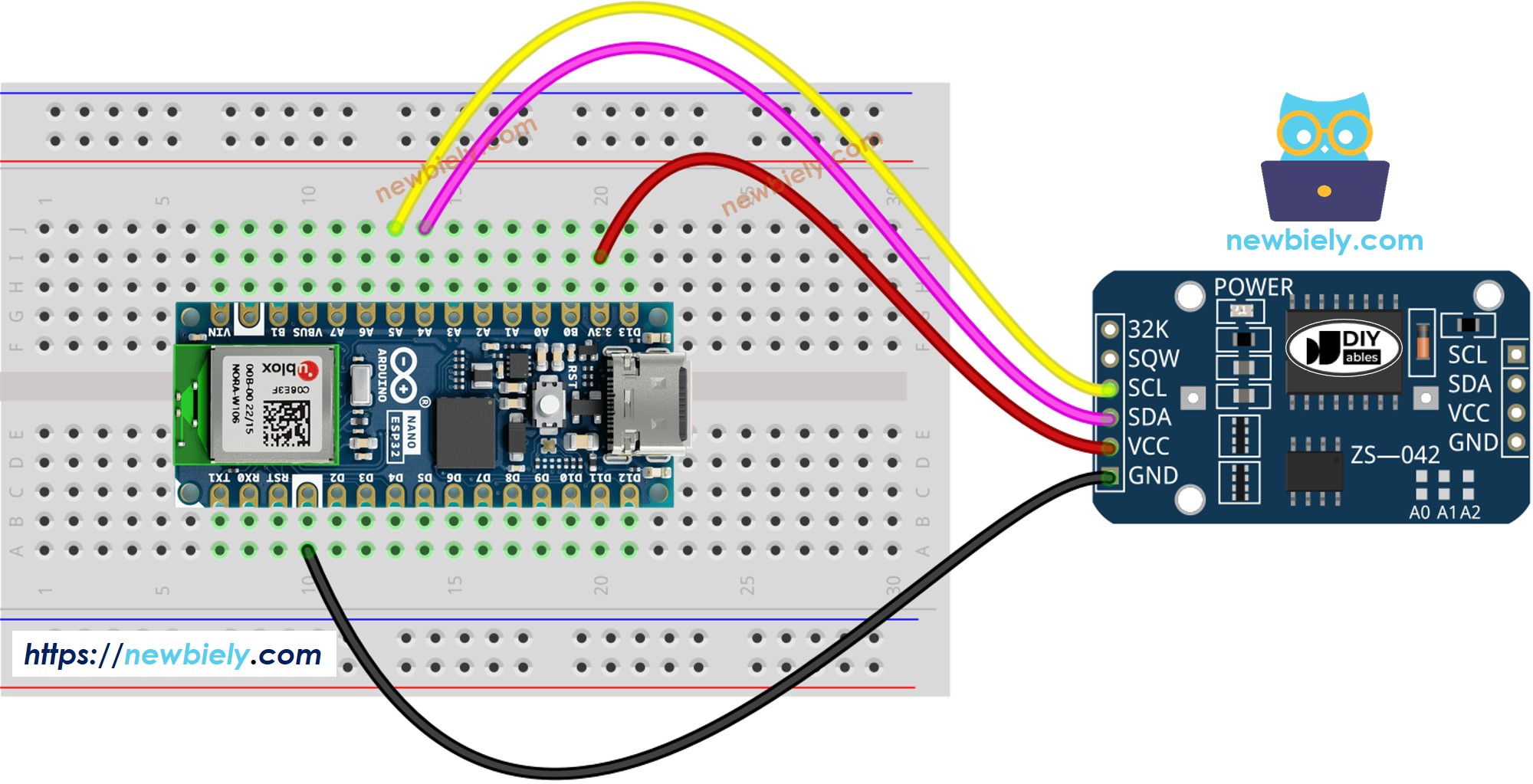
This image is created using Fritzing. Click to enlarge image
Arduino Nano ESP32 - DS3231 RTC Module
DS1307 RTC Module | Arduino Nano ESP32 |
---|---|
Vin | 3.3V |
GND | GND |
SDA | A4 |
SCL | A5 |
How To Program For DS3231 RTC Module
- Include the library:
- Declare a RTC object:
- Initialize RTC:
- For the first time, set the RTC to the date & time on PC the sketch was compiled
- Reads date and time information from RTC module
Arduino Nano ESP32 Code – How to get data and time
Detailed Instructions
To get started with Arduino Nano ESP32, follow these steps:
- If you are new to Arduino Nano ESP32, refer to the tutorial on how to set up the environment for Arduino Nano ESP32 in the Arduino IDE.
- Wire the components according to the provided diagram.
- Connect the Arduino Nano ESP32 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano ESP32 board and its corresponding COM port.
- Open the Library Manager by clicking on the Library Manager icon on the left navigation bar of Arduino IDE.
- Search “RTClib”, then find the RTC library by Adafruit
- Click Install button to install RTC library.
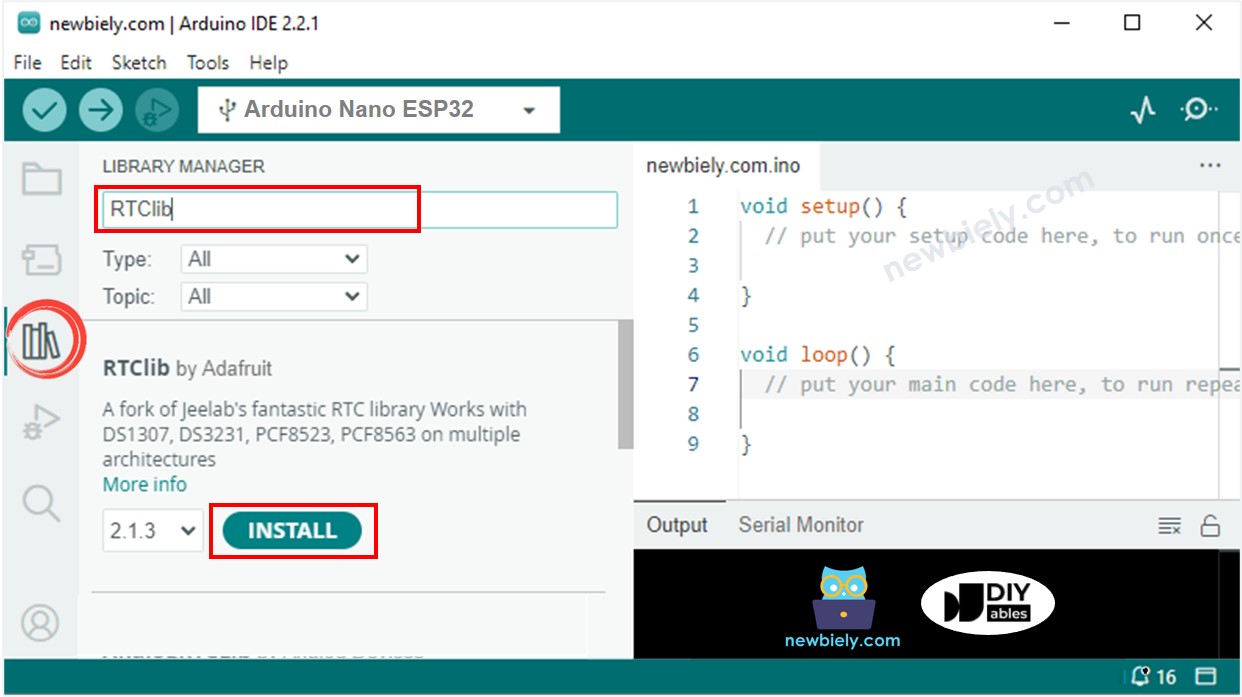
- A windows may appear to ask you to install dependencies for the library
- Install all dependencies for the library by clicking on Install All button.
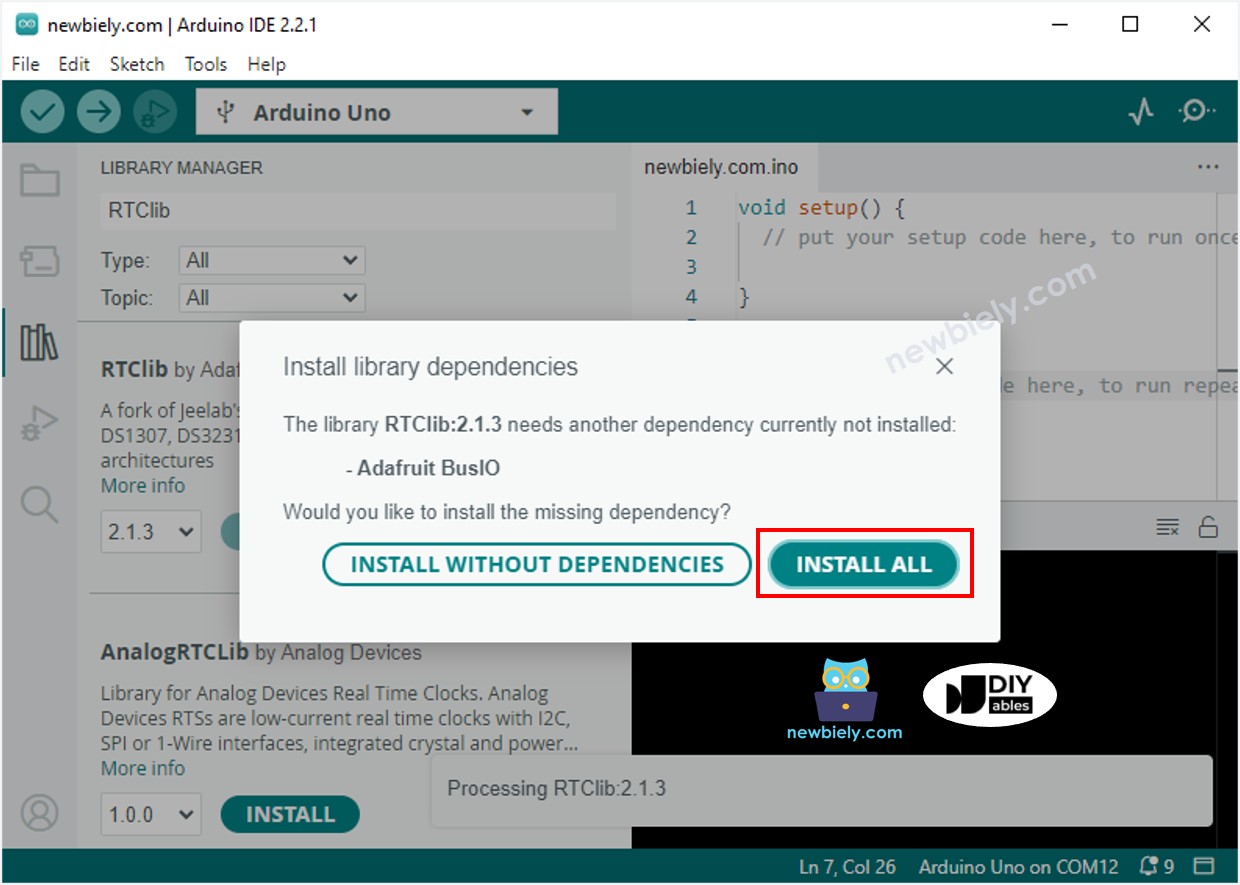
- Copy the above code and paste it to Arduino IDE
- Compile and upload code to Arduino Nano ESP32 board by clicking Upload button on Arduino IDE
- Open Serial Monitor on Arduino IDE
- See the output on Serial Monitor.