Arduino Nano ESP32 - Web Server Password
In this guide, we'll learn how to create an Arduino Nano ESP32 web server with username and password protection for login. Prior to accessing any web pages on the ESP32, users will be prompted to input their username and password.
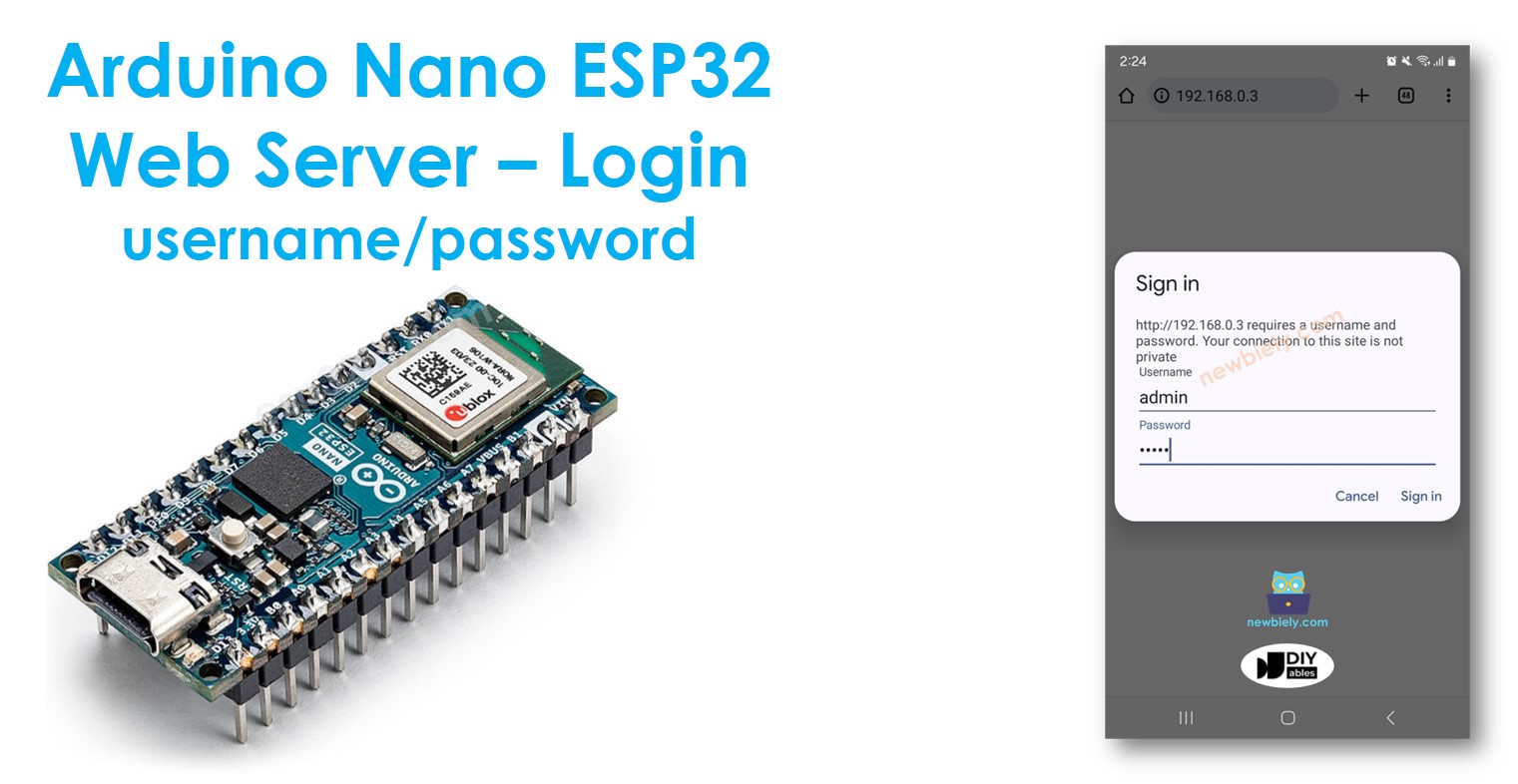
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand, DIYables .
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Arduino Nano ESP32 and Web Server
If you're not familiar with Arduino Nano ESP32 and Web Server (including pinout, how it works, and programming), you can learn about them through the following tutorials:
Arduino Nano ESP32 Code - Web server Username/Password
/*
* This Arduino Nano ESP32 code was developed by newbiely.com
*
* This Arduino Nano ESP32 code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-nano-esp32/arduino-nano-esp32-web-server-password
*/
#include <WiFi.h>
#include <ESPAsyncWebServer.h>
// Replace with your network credentials
const char* ssid = "YOUR_WIFI_SSID"; // CHANGE IT
const char* password = "YOUR_WIFI_PASSWORD"; // CHANGE IT
AsyncWebServer server(80);
const char* www_username = "admin";
const char* www_password = "esp32";
void handleRoot(AsyncWebServerRequest* request) {
if (!request->authenticate(www_username, www_password)) {
return request->requestAuthentication();
}
// send your web content here. The below is simplest form.
request->send(200, "text/html", "<html><body><h1>Login Successful!</h1><p>You are now logged in.</p></body></html>");
}
void handleNotFound(AsyncWebServerRequest* request) {
request->send(404, "text/plain", "Not found");
}
void setup() {
Serial.begin(9600);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
server.on("/", HTTP_GET, handleRoot);
server.onNotFound(handleNotFound);
server.begin();
// Print the ESP32's IP address
Serial.print("ESP32 Web Server's IP address: ");
Serial.println(WiFi.localIP());
}
void loop() {
}
Detailed Instructions
- If this is the first time you use ESP32, see how to setup environment for Arduino Nano ESP32 on Arduino IDE.
- Connect the Arduino Nano ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right Arduino Nano ESP32 board (e.g. Arduino Nano ESP32 Dev Module) and COM port.
- Open the Library Manager by clicking on the Library Manager icon on the left navigation bar of Arduino IDE.
- Search “ESPAsyncWebServer”, then find the ESPAsyncWebServer created by lacamera.
- Click Install button to install ESPAsyncWebServer library.
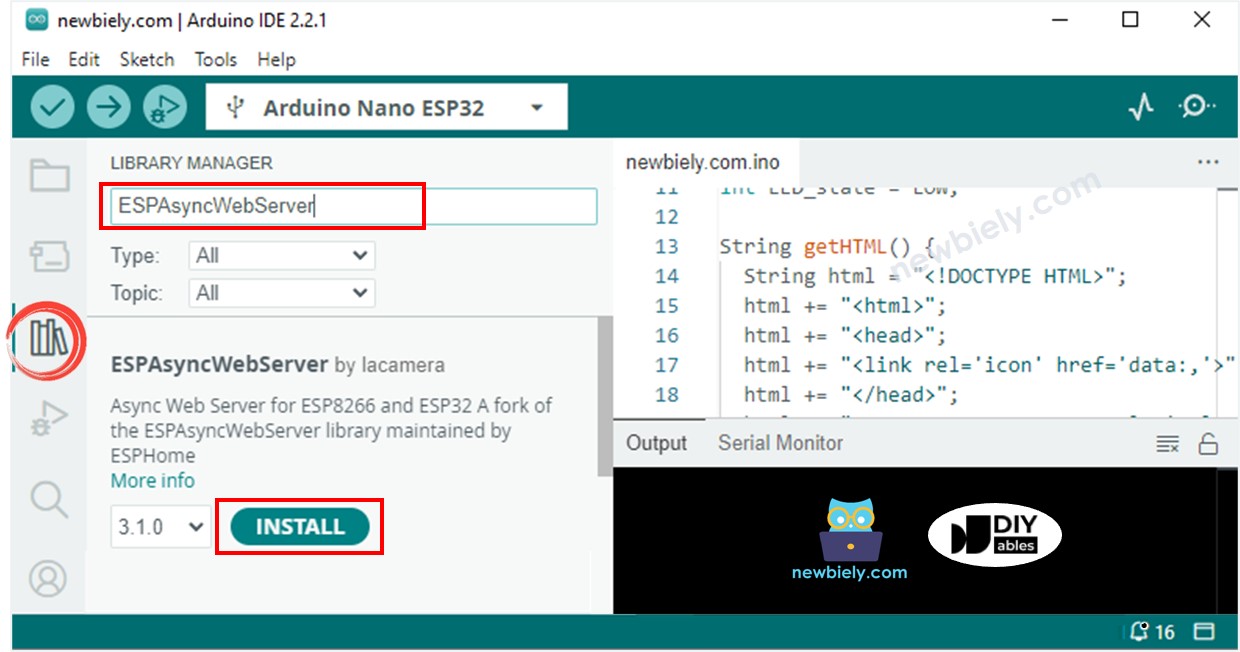
- You will be asked to install the dependency. Click Install All button.
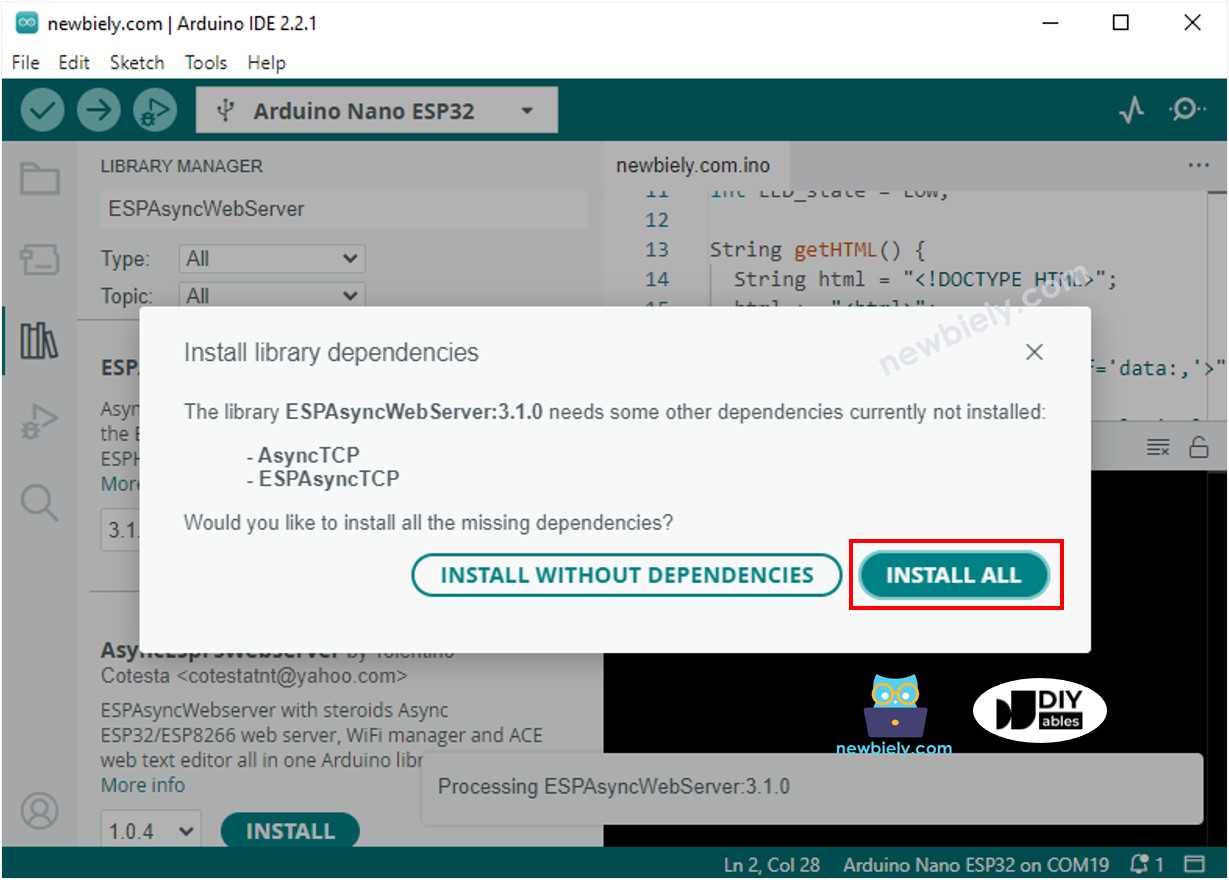
- Copy the above code and open with Arduino IDE
- Change the wifi information (SSID and password) in the code to yours
- Click Upload button on Arduino IDE to upload code to ESP32
- Open the Serial Monitor
- Check out the result on Serial Monitor.
COM6
Connecting to WiFi...
Connected to WiFi
Arduino Nano ESP32 Web Server's IP address: 192.168.0.3
Autoscroll
Clear output
9600 baud
Newline
- You will see an IP address on the Serial Monitor, for example: 192.168.0.3
- Type the IP address on the address bar of a web browser on your smartphone or PC.
- Please note that you need to change the 192.168.0.3 to the IP address you got on Serial Monitor.
- You will see a page that promont you to input username/password.
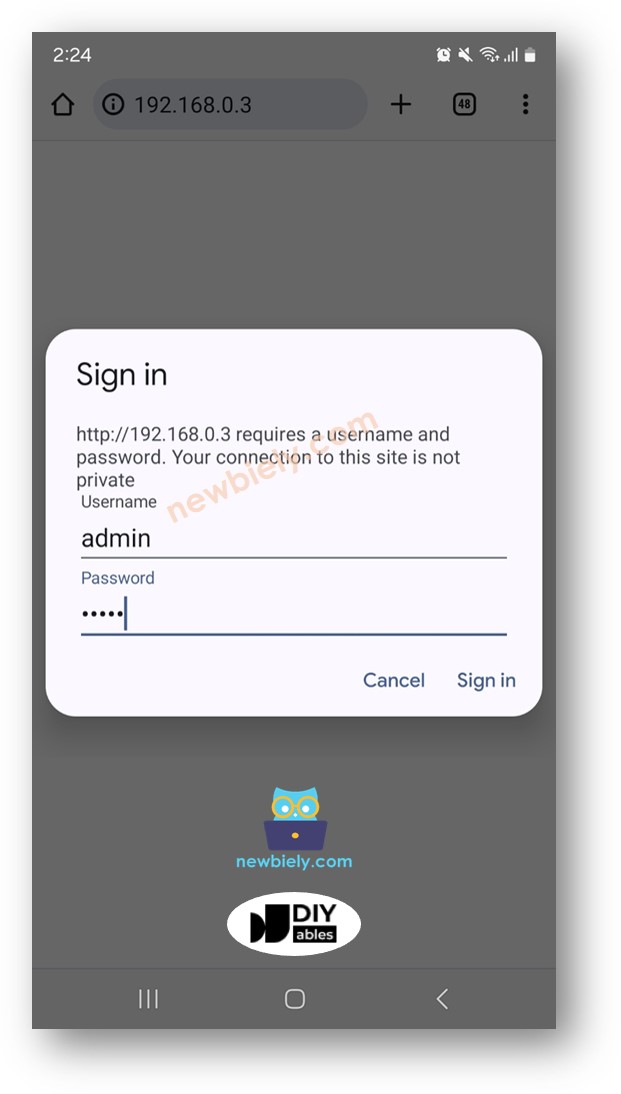
- Type username/password that are in the Arduino Nano ESP32 code, in this case: admin as username, esp32 as password
- If you input the username/password correctly, the web content of Arduino Nano ESP32 will be shown:
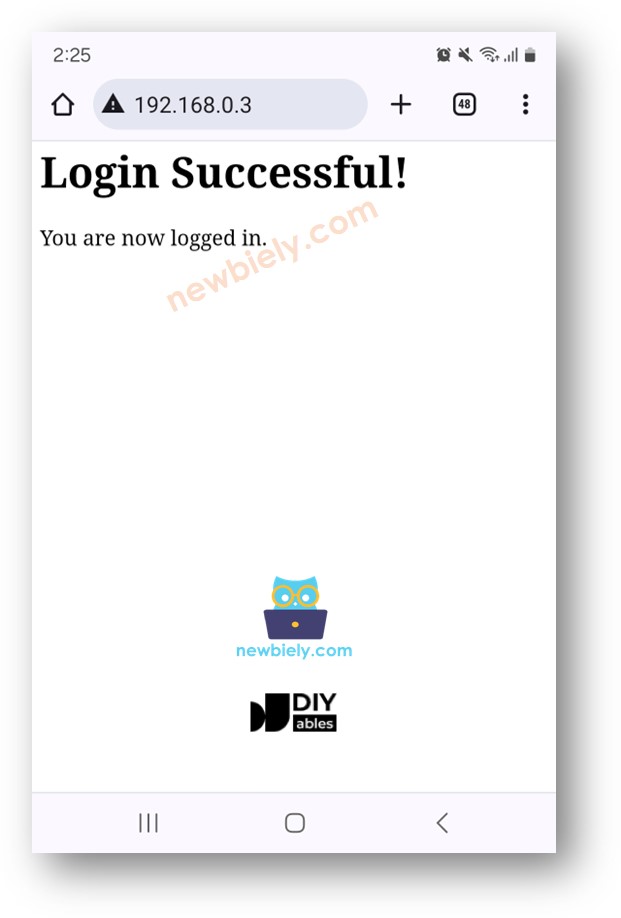
※ NOTE THAT:
- You can adjust the web username and password directly within the code by altering the values assigned to two variables: www_username and www_password.
- You have the option to customize this code by incorporating your own HTML, CSS, and JavaScript code for your webpage.
- It's worth noting that there is no HTML code within the code itself for the login form (username/password). Don't be surprised! Instead, the login form is generated dynamically by the Web Browser.
Advanced Knowledge
This section provides advanced insights into how the username/password system operates without HTML for the login form:
- Initially, when you input the IP address of the Arduino Nano ESP32 into a web browser, the browser sends an HTTP request to the Arduino Nano ESP32 without any username/password credentials.
- Upon receiving this request, the Arduino Nano ESP32 code checks if there are any username/password credentials provided. If not, the Arduino Nano ESP32 does not respond with the content of the requested page. Instead, it responds with an HTTP message containing headers instructing the browser to prompt the user for their username/password. Importantly, this response does not include HTML code for the login form.
- Upon receiving this response, the web browser interprets the HTTP headers, understanding the ESP32's request for username/password. Consequently, the browser dynamically generates a login form, allowing the user to input their credentials.
- The user then inputs their username/password into the form.
- The web browser includes the entered username/password within an HTTP request and sends it to the ESP32.
- The Arduino Nano ESP32 verifies the username/password included in the HTTP request. If correct, it returns the content of the requested page. If incorrect, it repeats the process, prompting the user to input the correct credentials again.
- Once the user inputs the correct username/password for the first time, subsequent requests do not require them to re-enter their credentials. This is because the web browser automatically saves the credentials, including them in subsequent requests.