Arduino Nano ESP32 - Servo Motor
This tutorial provides instructions on how to use Arduino Nano ESP32 to control a servo motor.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Servo Motor
The standard servo motor is a motor that can rotate between 0° and 180°.
Servo Motor Pinout
The servo motor has three pins:
- GND pin: (brown or black) connects this pin to GND (0V)
- VCC pin: (red) connects this pin to VCC (5V)
- Signal pin: (yellow or orange) receives the PWM control signal from an ESP32's pin.
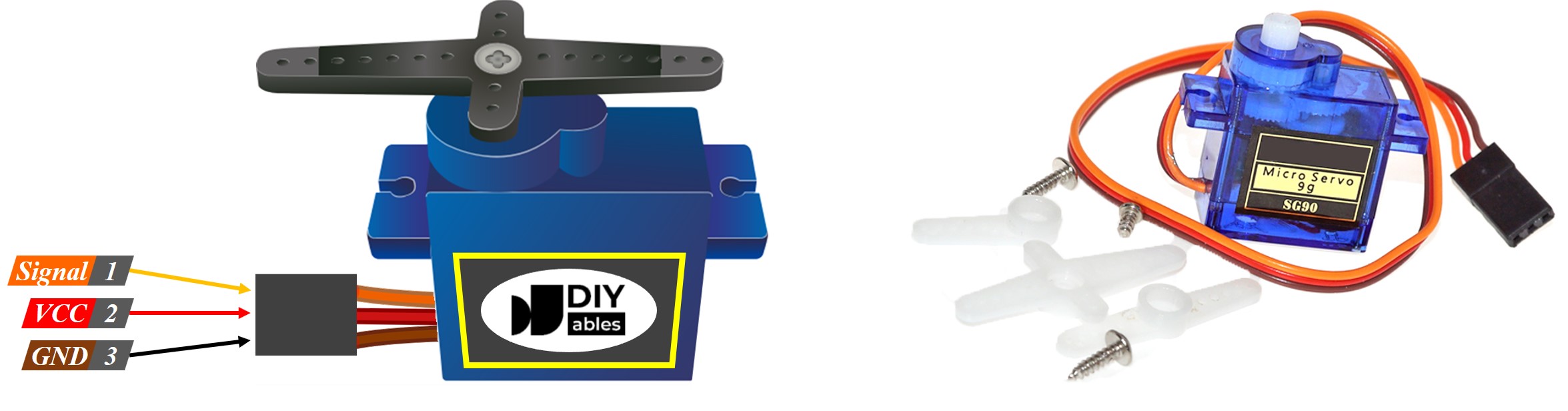
How Servo Motor Works
Wiring Diagram between Servo Motor and Arduino Nano ESP32
- When powering the Arduino Nano ESP32 board via USB port.
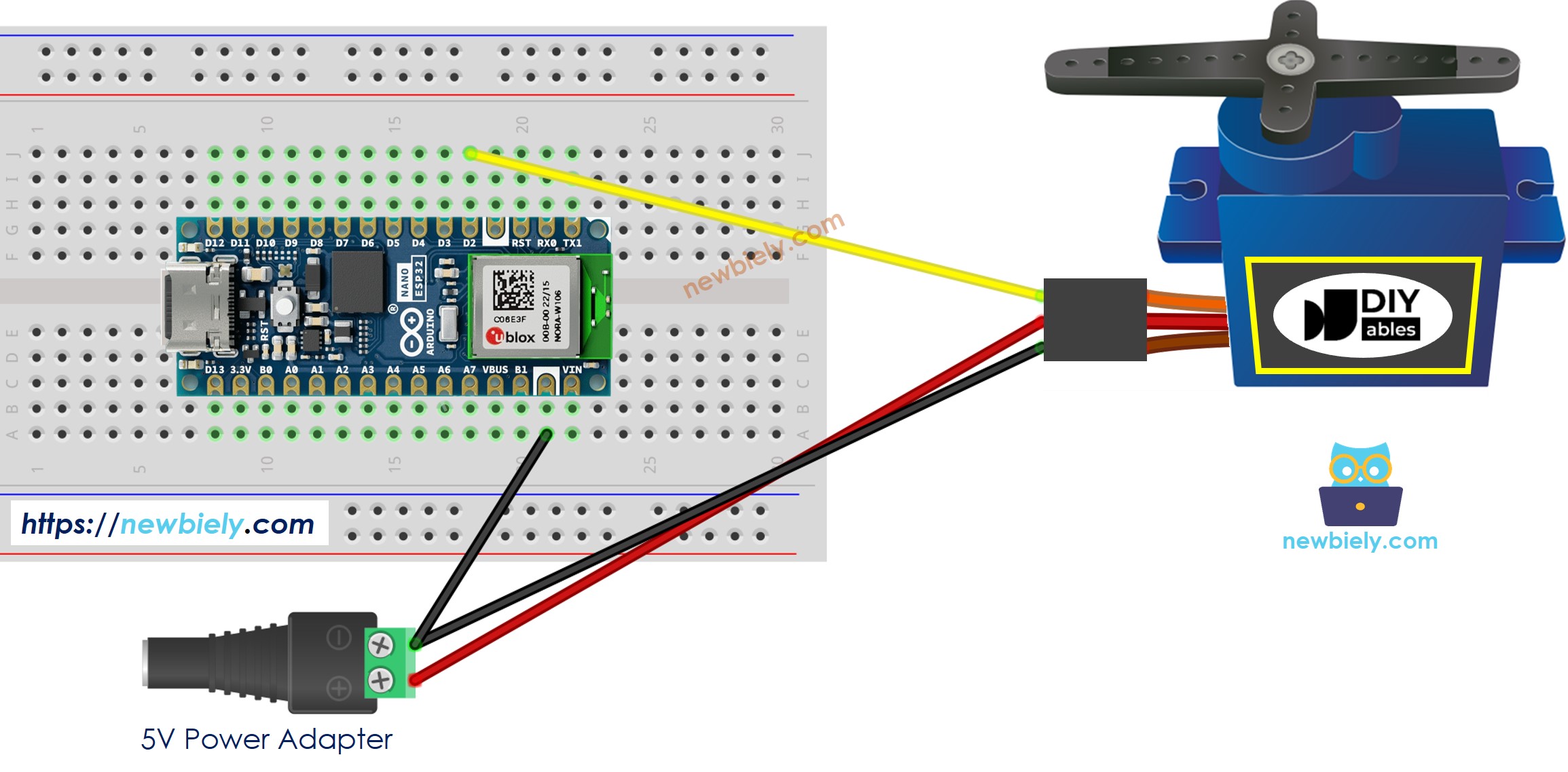
This image is created using Fritzing. Click to enlarge image
- When powering the Arduino Nano ESP32 board via Vin pin.
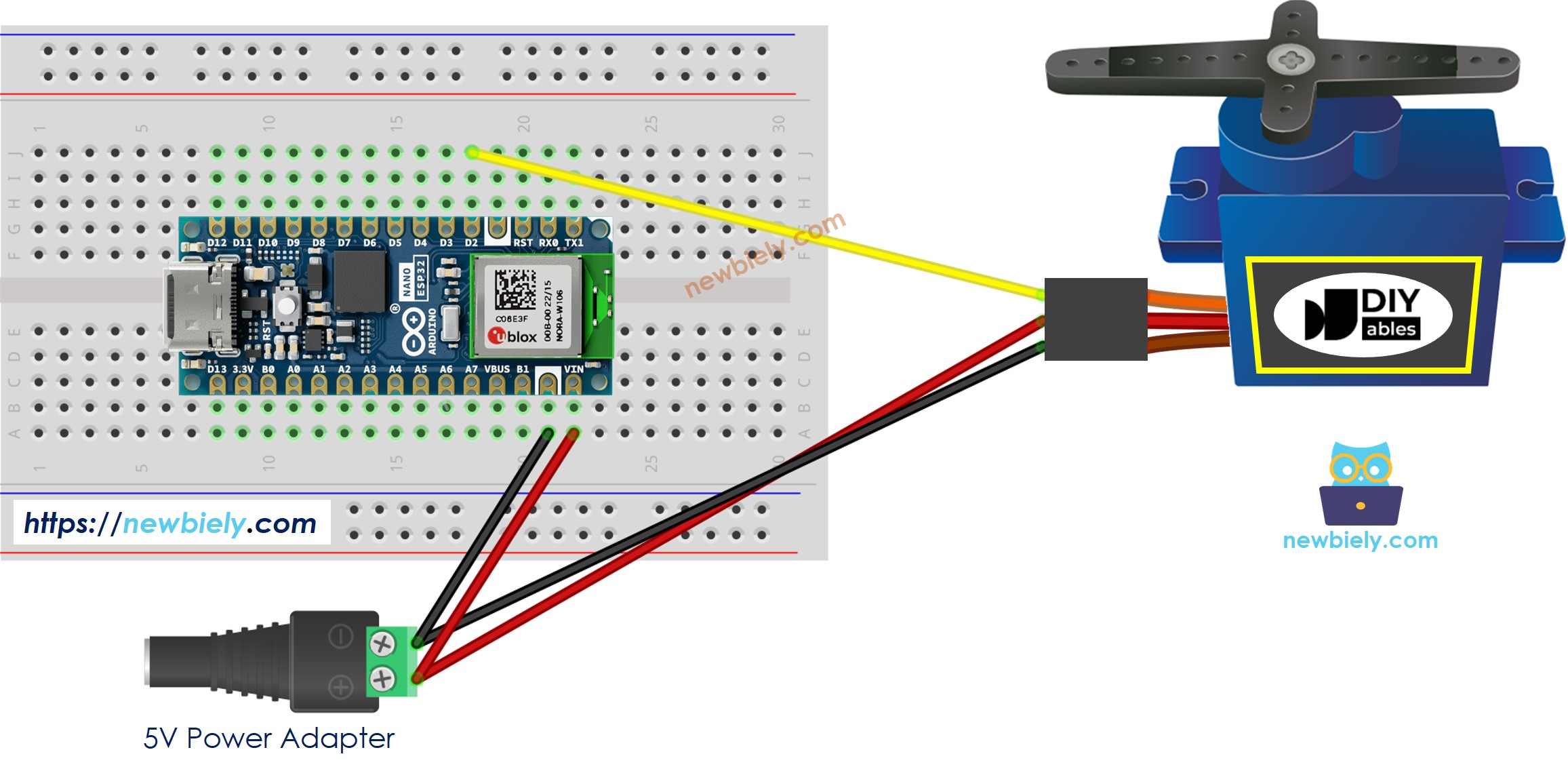
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
When powering the Arduino Nano ESP32 board via USB port, it should NOT power the servo motor via Vin pin VBUS pin. If you power the servo motor via this pin, your board may be get burned.
Arduino Nano ESP32 Code
Detailed Instructions
To get started with Arduino Nano ESP32, follow these steps:
- If you are new to Arduino Nano ESP32, refer to the tutorial on how to set up the environment for Arduino Nano ESP32 in the Arduino IDE.
- Wire the components according to the provided diagram.
- Connect the Arduino Nano ESP32 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano ESP32 board and its corresponding COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Type ServoESP32 on the search box, then look for the servo library by Jaroslav Paral. Please be aware that both version 1.1.1 and 1.1.0 are affected by bugs. Kindly choose a different version.
- Click Install button to install servo motor library for Arduino Nano ESP32.
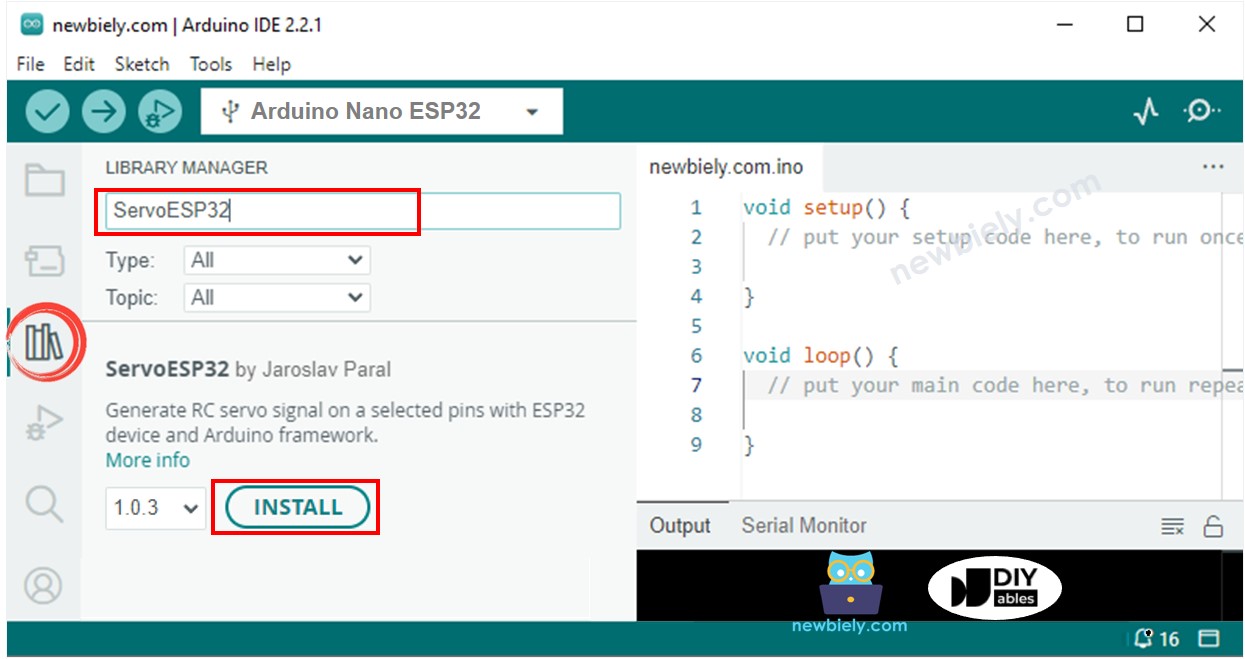
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to Arduino Nano ESP32 board by clicking Upload button on Arduino IDE
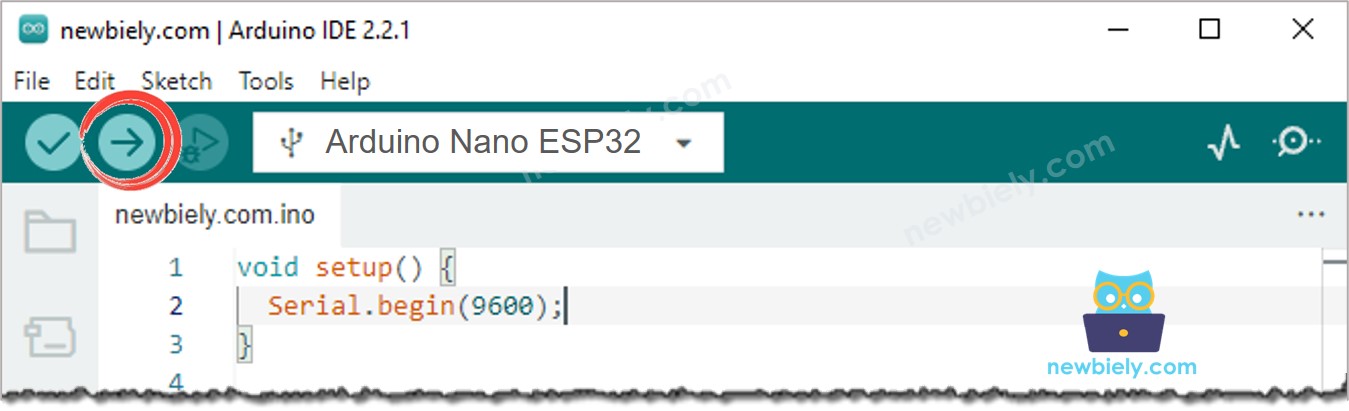
- See the result: Servo motor rotates slowly about 180° in clockwise and counter-clockwise direction
Line-by-line Code Explanation
The above Arduino Nano ESP32 code contains line-by-line explanation. Please read the comments in the code!
How to Control Speed of Servo Motor
Video Tutorial
The instruction and source code for the above video available at how to control servo motor via web tutotiral