Arduino Nano ESP32 - OLED
This tutorial provides instructions on how to use Arduino Nano ESP32 with OLED display. In detail, we will learn:
- How to connect OLED display with Arduino Nano ESP32.
- How to program Arduino Nano ESP32 to display text and number on OLED.
- How to program Arduino Nano ESP32 to center align text and number vertically and horizontally on OLED.
- How to program Arduino Nano ESP32 to draw on OLED.
- How to program Arduino Nano ESP32 to display an image on OLED.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of OLED Display
There are various kinds of OLED displays available. The most common-used OLED with Arduino Nano ESP32 is the SSD1306 I2C OLED 128x64 and 128x32 display.
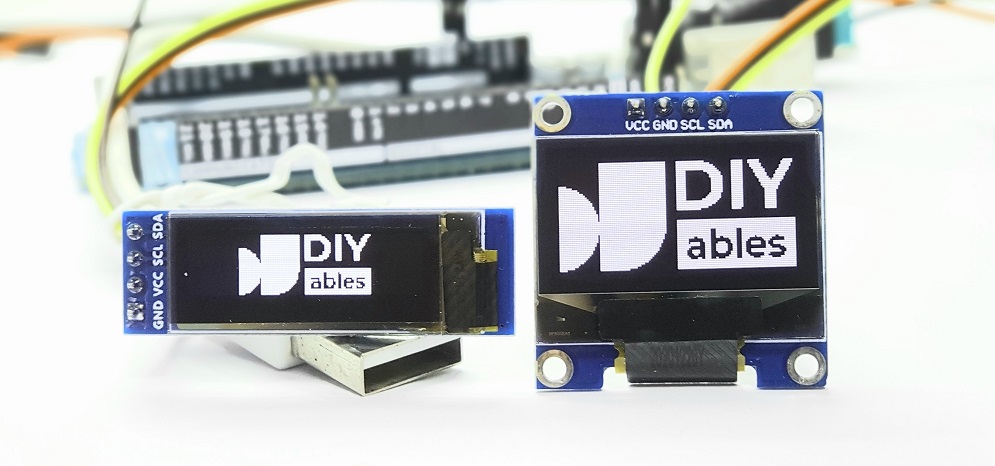
I2C OLED Display Pinout
- GND pin: This should be connected to the ground of the Arduino Nano ESP32.
- VCC pin: This is the power supply for the display which should be connected to the 3.3V or 5V.
- SCL pin: This is a serial clock pin for I2C interface.
- SDA pin: This is a serial data pin for I2C interface.
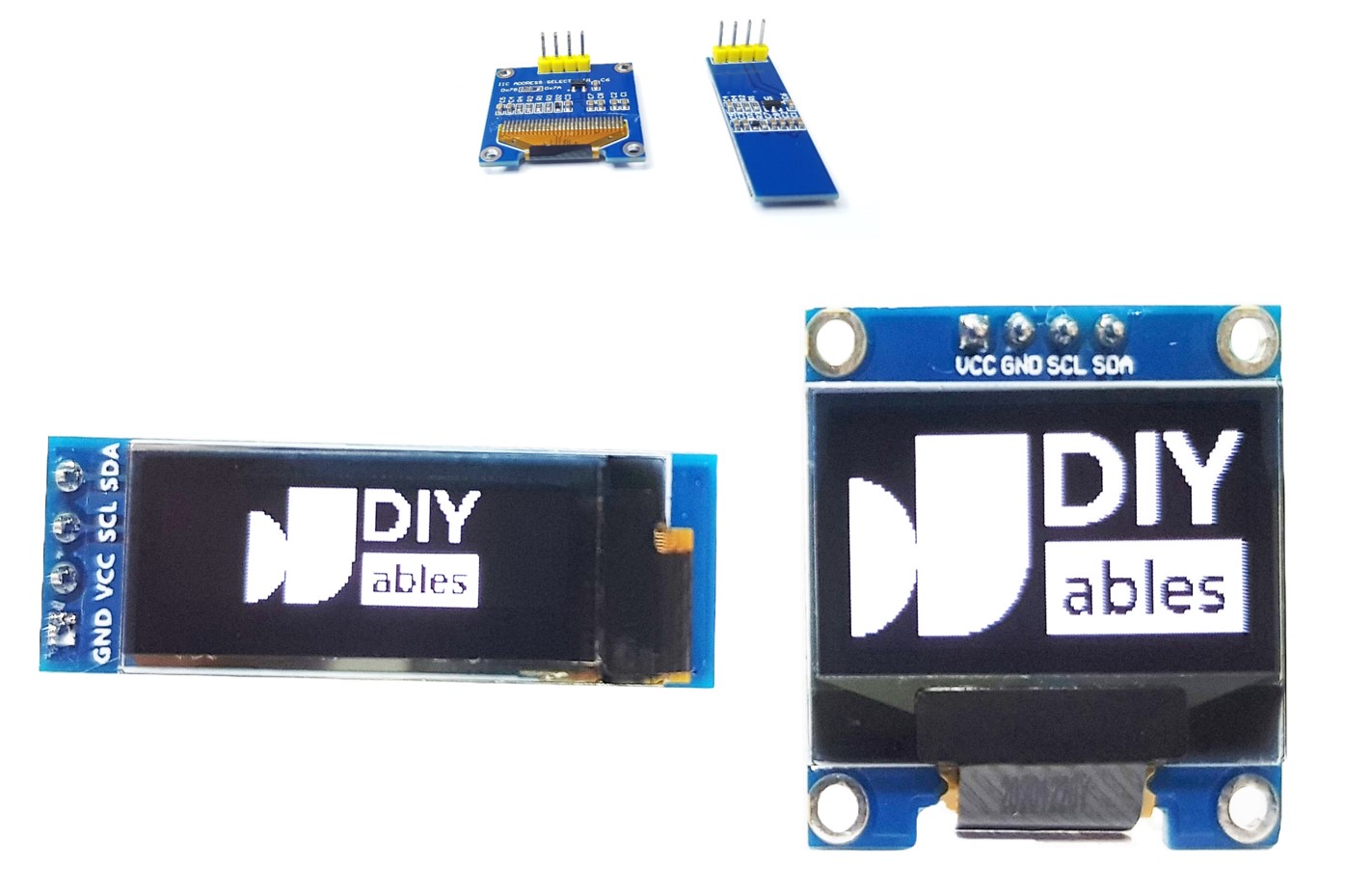
※ NOTE THAT:
- The pins of the OLED module can differ depending on the manufacturer and type. Please use the labels printed on the OLED module. Please take a close look!
- This tutorial uses the OLED display that is driven by the SSD1306 I2C driver. We have tested it with the OLED display from DIYables and it works perfectly.
Wiring Diagram
- Wiring diagram between Arduino Nano ESP32 and OLED 128x64
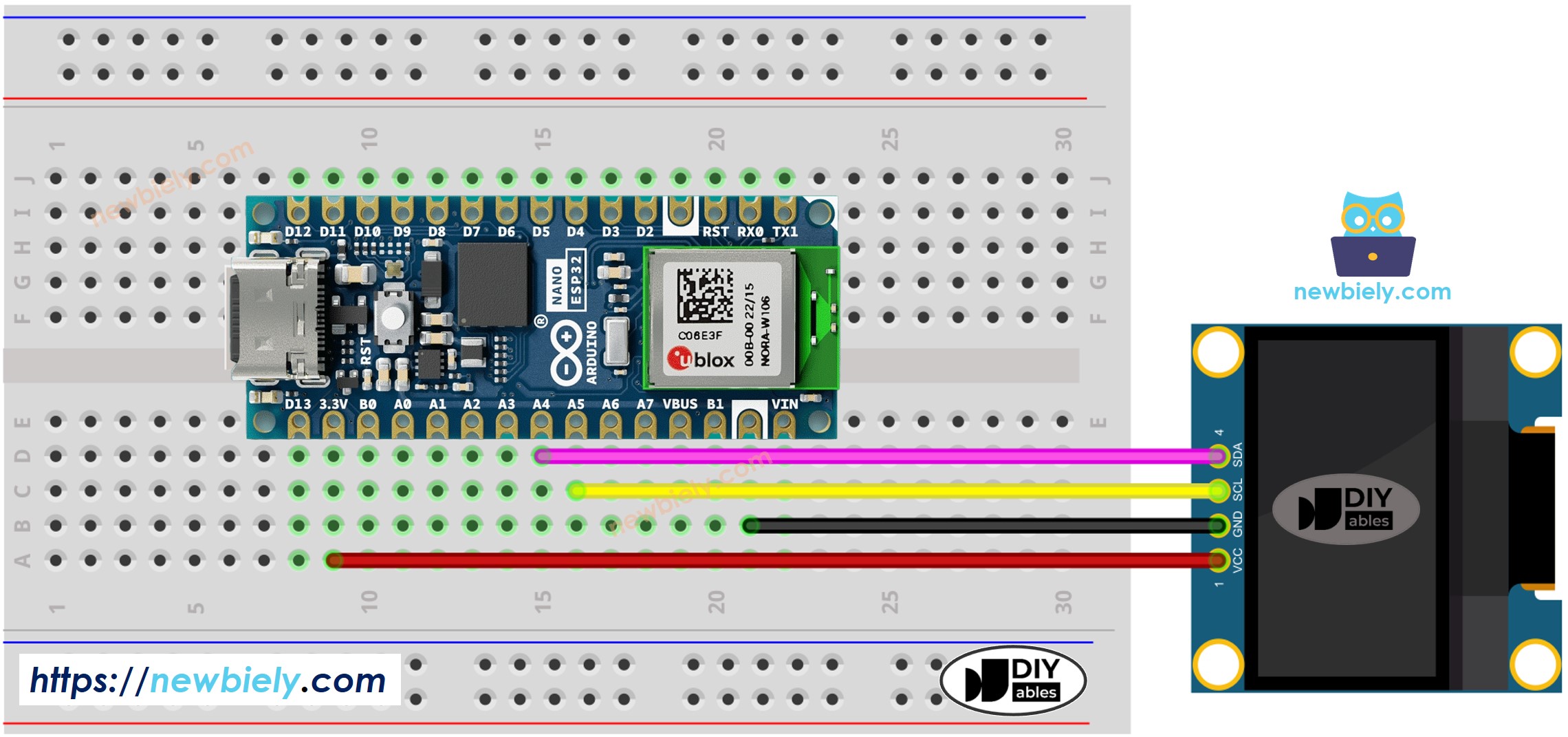
This image is created using Fritzing. Click to enlarge image
- Wiring diagram between Arduino Nano ESP32 and OLED 128x32
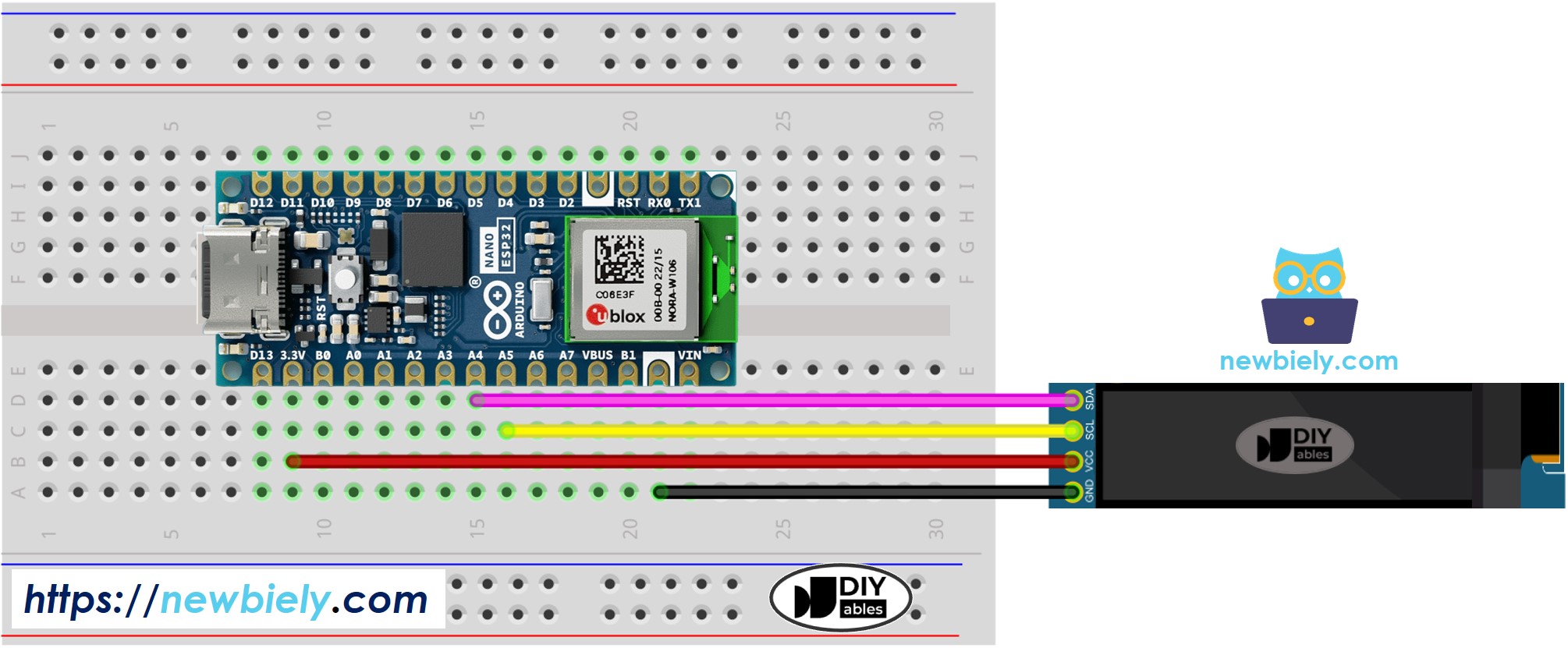
This image is created using Fritzing. Click to enlarge image
The wiring table between Arduino Nano ESP32 and OLED display:
OLED Module | Arduino Nano ESP32 |
---|---|
Vin | 3.3V |
GND | GND |
SDA | A4 |
SCL | A5 |
How To Use OLED with Arduino Nano ESP32
Install SSD1306 OLED library
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “SSD1306” and locate the SSD1306 library from Adafruit.
- Then, press the Install button to complete the installation.
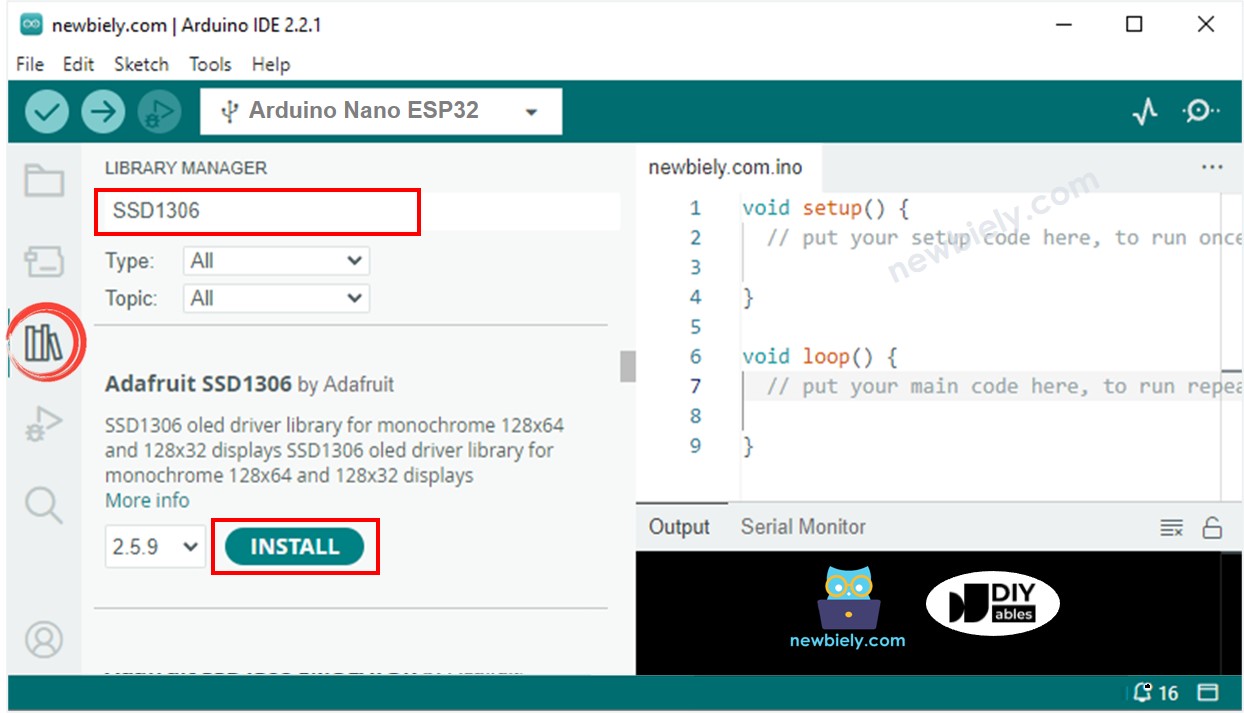
- You will be prompted to install additional library dependencies.
- To install all of them, click the Install All button.
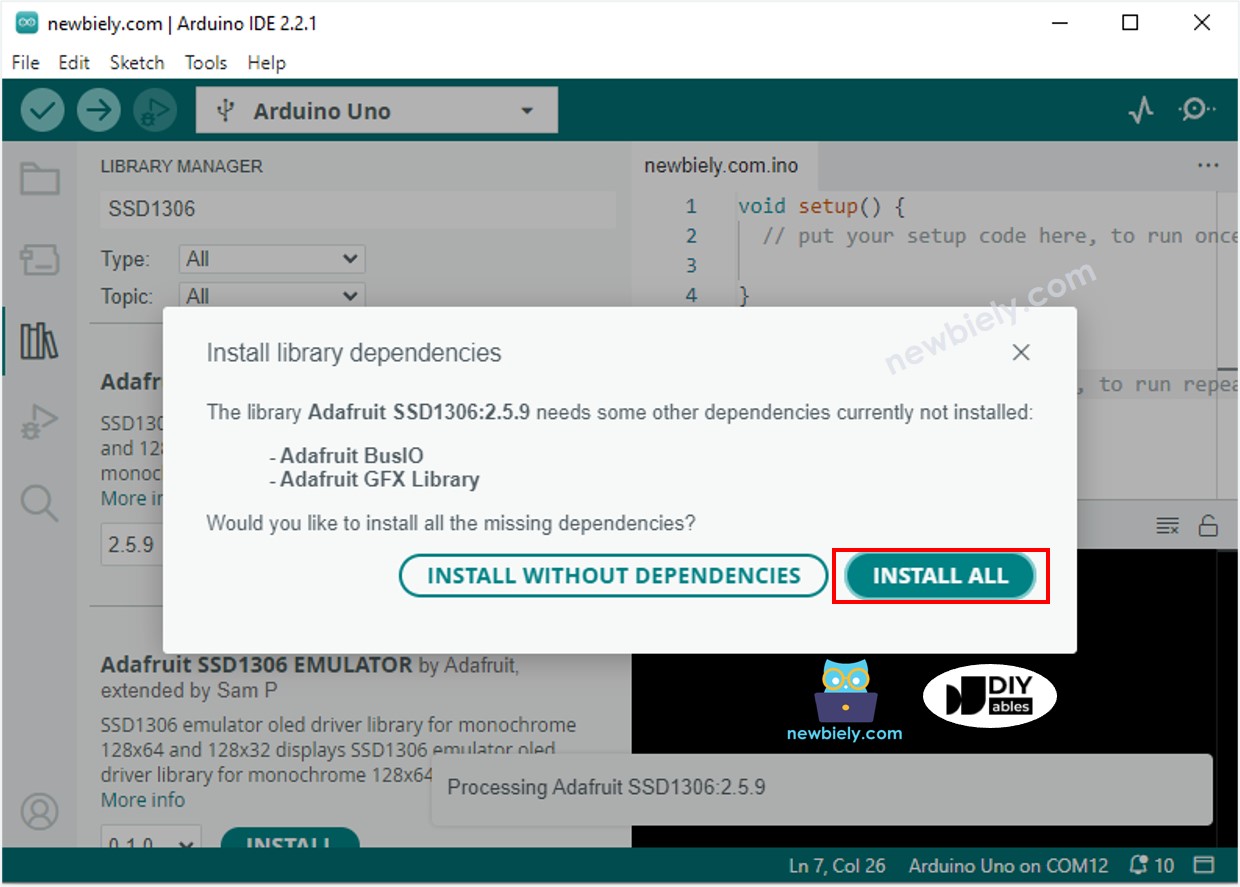
How to program for OLED
- Include the library
- Specify the dimensions of the OLED screen as 128 x 64.
- Or specify the dimensions of an OLED screen that is 128x32.
- Create an object of type SSD1306 OLED.
- In the setup() function, initiate the OLED display.
- Then, you can display text, pictures, and draw a line...
Going forward, all codes will be for OLED 128x64. However, it is easy to modify them for OLED 128x32 by altering the screen size and adjusting the coordinates if necessary.
Arduino Nano ESP32 Code - Display Text on OLED
These are some functions that can be used to display text on the OLED:
- oled.clearDisplay(): all pixels are off.
- oled.drawPixel(x,y, color): plot a pixel in the x,y coordinates.
- oled.setTextSize(n): set the font size, supports sizes from 1 to 8.
- oled.setCursor(x,y): set the coordinates to start writing text.
- oled.setTextColor(WHITE): set the text color.
- oled.setTextColor(BLACK, WHITE): set the text color, background color.
- oled.println(“message”): print the characters.
- oled.println(number): print a number.
- oled.println(number, HEX): print a number IN hex format.
- oled.display(): call this method for the changes to make effect.
- oled.startscrollright(start, stop): scroll text from left to right.
- oled.startscrollleft(start, stop): scroll text from right to left.
- oled.startscrolldiagright(start, stop): scroll text from left bottom corner to right upper corner.
- oled.startscrolldiagleft(start, stop): scroll text from right bottom corner to left upper corner.
- oled.stopscroll(): stop scrolling.
How to vertical and horizontal center align text/number on OLED
For information on how to align text and numbers vertically and horizontally on an OLED display, please refer to How to vertical/horizontal center on OLED.
Arduino Nano ESP32 Code - Drawing on OLED
Arduino Nano ESP32 Code – Display Image
In order to display an image on OLED, we must first convert the image (in any format) to a bitmap array. This can be accomplished by using the online tool. The following image demonstrates how to convert an image to a bitmap array; I have used the Arduino Nano ESP32 icon as an example.
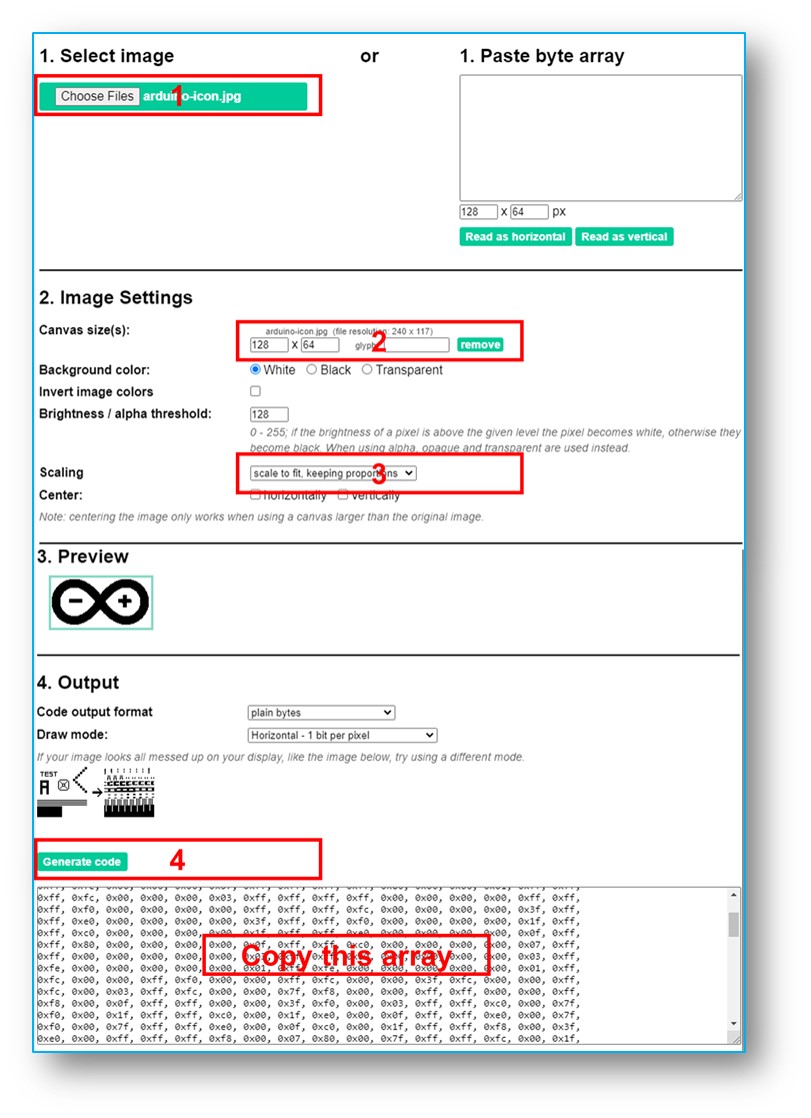
After conversion, take the array code and put it in place of the ArduinoIcon array in the code below.
※ NOTE THAT:
- The size of the image should not be larger than the size of the screen.
- If you wish to use the code for an OLED 128x32, you must resize the image and adjust the width and height parameters in the oled.drawBitmap(); function.
OLED Troubleshooting
Verify that the OLED is functioning properly by doing the following:
- Ensure that your wiring is correct.
- Confirm that your OLED utilizes the SSD1306 driver.
- Utilize the I2C Address Scanner code on Arduino Nano ESP32 to check the I2C address of the OLED.
The output displayed on the Serial Monitor looks like below: