Arduino Nano ESP32 - Door Sensor
The door sensor (also known as entry sensor, contact sensor, or window sensor) is widely used in many kinds of application, especially for security. It is used to detect/monitor entrances (such as door, window ...). This tutorial provides instructions on how to use Arduino Nano ESP32 with the door sensor.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Door Sensor
Door Sensor Pinout
The door sensor has two components:
- One magnet
- One reed switch, which has two wires
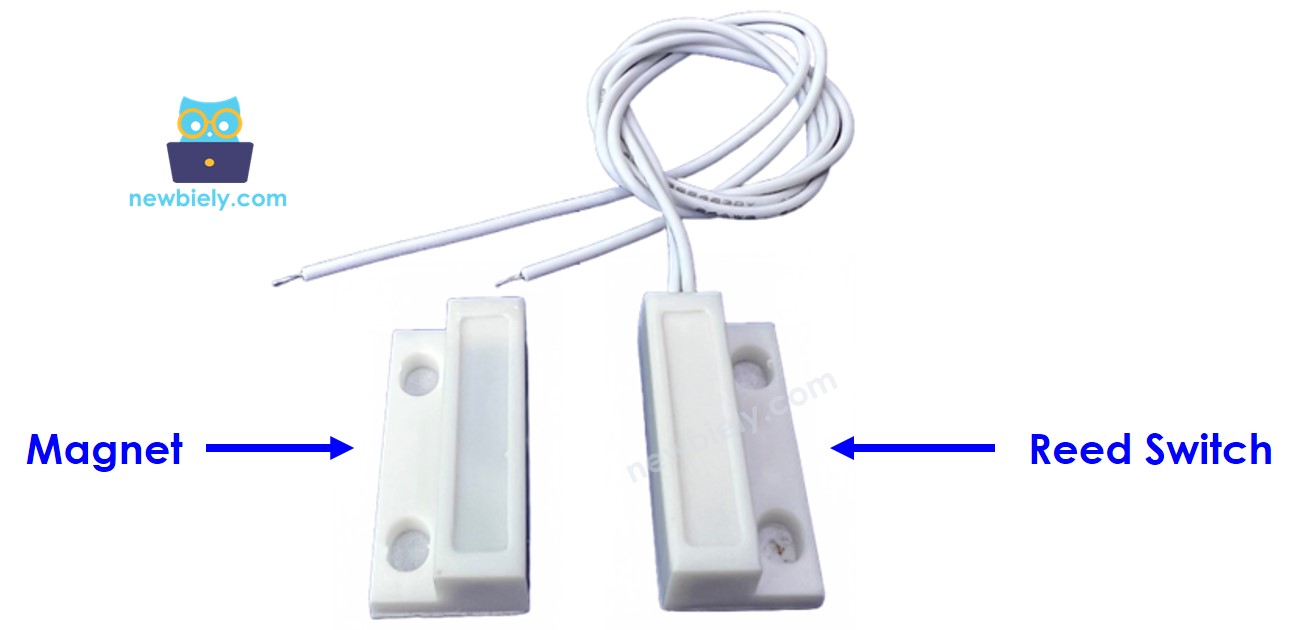
Just like the switch/button, we do NOT need to differentiate the two wires of the reed switch.
How Door Sensor Works
The magnet and the reed switch are installed on the door/windows as follows:
- The magnet is the movable part. It should be attached to the door/window
- The reed switch is the fixed part. It should be attached to the door frame
The two components are in contact when the door is closed.
- The reed switch circuit is closed when it is near to the magnet
- The reed switch circuit is open when it is far from the magnet
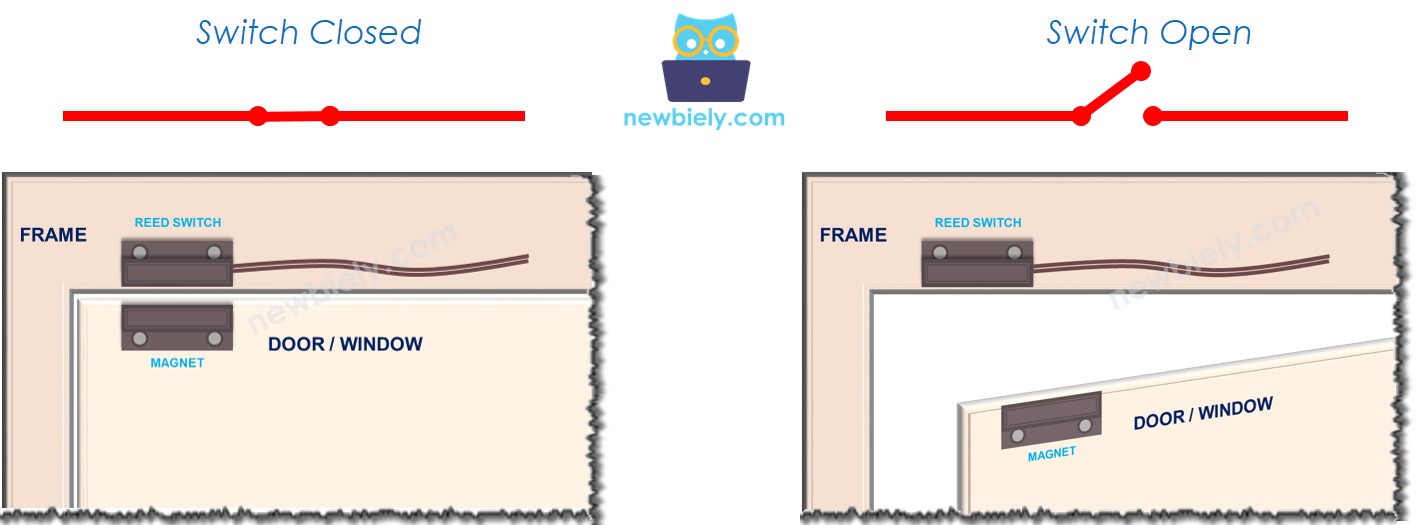
※ NOTE THAT:
Just like a button, we MUST use the pull-up or pull-down resistor on the Arduino Nano ESP32 pin, which connects to the reed switch
If we connect reed switch as follows: one wire to GND, the other to ESP32's input pin with a pull-up resistor:
- The ESP32's input pin is LOW when the magnet is near to the reed switch
- The ESP32's input pin is HIGH when the magnet is far from the reed switch
So:
- If the ESP32's input pin is LOW, the door is closed
- If the ESP32's input pin is HIGH, the door is opened
- If the ESP32's input pin changes from LOW to HIGH, the door is opening
- If the ESP32's input pin changes from HIGH to LOW, the door is closing
Wiring Diagram between Door Sensor and Arduino Nano ESP32
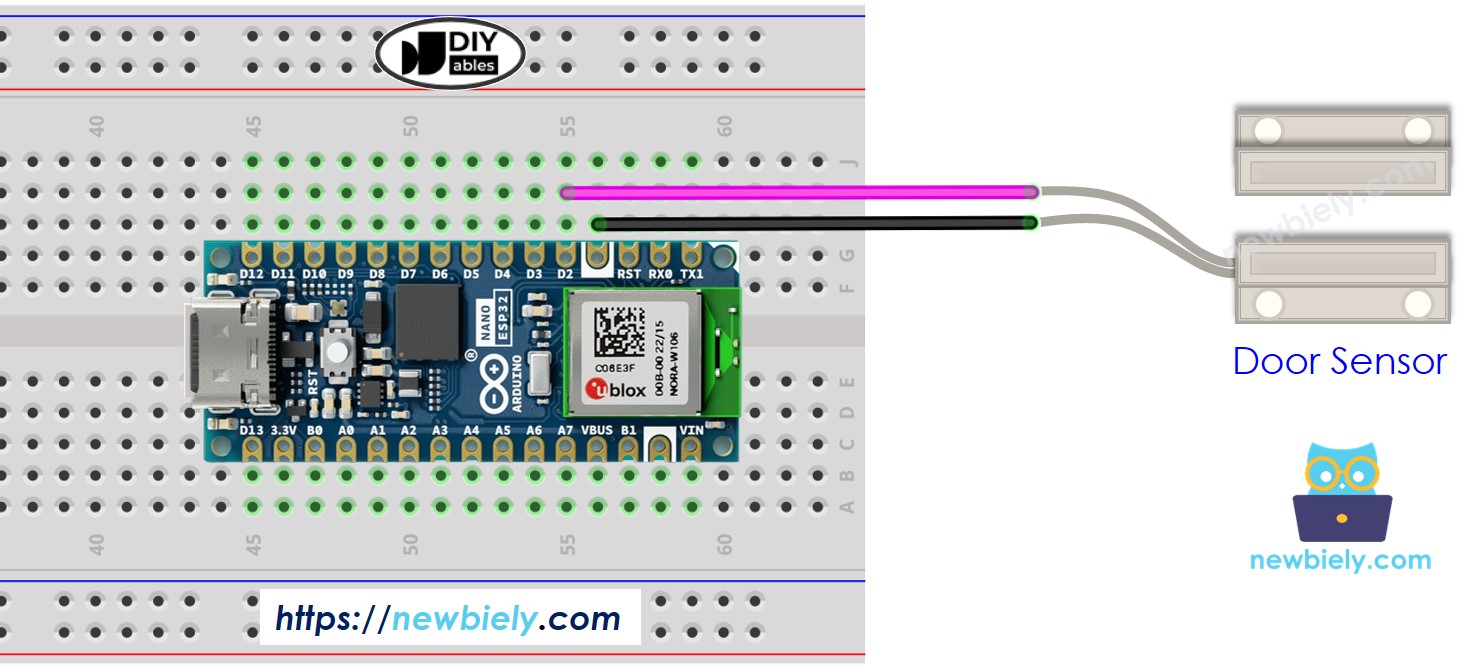
This image is created using Fritzing. Click to enlarge image
How To Program Door Sensor
- Initializes the Arduino Nano ESP32 pin to the digital input mode by using pinMode() function. For example, pin D2
- Reads the state of the Arduino Nano ESP32 pin by using digitalRead() function.
Arduino Nano ESP32 Code - Check Door Open and Close State
Detailed Instructions
- If this is the first time you use Arduino Nano ESP32, see how to setup environment for Arduino Nano ESP32 on Arduino IDE.
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to Arduino Nano ESP32 board by clicking Upload button on Arduino IDE
- Move the magnet close to the reed switch and them move it far from the reed switch.
- Check out the result on the Serial Monitor. It looks like the below:.
Arduino Nano ESP32 Code - Detect Door-opening and Door-closing Events
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to Arduino Nano ESP32 board by clicking Upload button on Arduino IDE
- Move the magnet close to the reed switch and them move it far from the reed switch.
- Check out the result on the Serial Monitor. It looks like the below:.