Arduino Nano ESP32 - Keypad - LCD
This tutorial instructs you how to use Arduino Nano ESP32 with the keypad and LCD display. In detail, We will learn how to program the Arduino Nano ESP32 to display the pressed key on LCD.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Keypad and LCD
We have specific tutorials about keypad and LCD. Each tutorial contains detailed information and step-by-step instructions about hardware pinout, working principle, wiring connection to Arduino Nano ESP32, Arduino Nano ESP32 code... Learn more about them at the following links:
Wiring Diagram
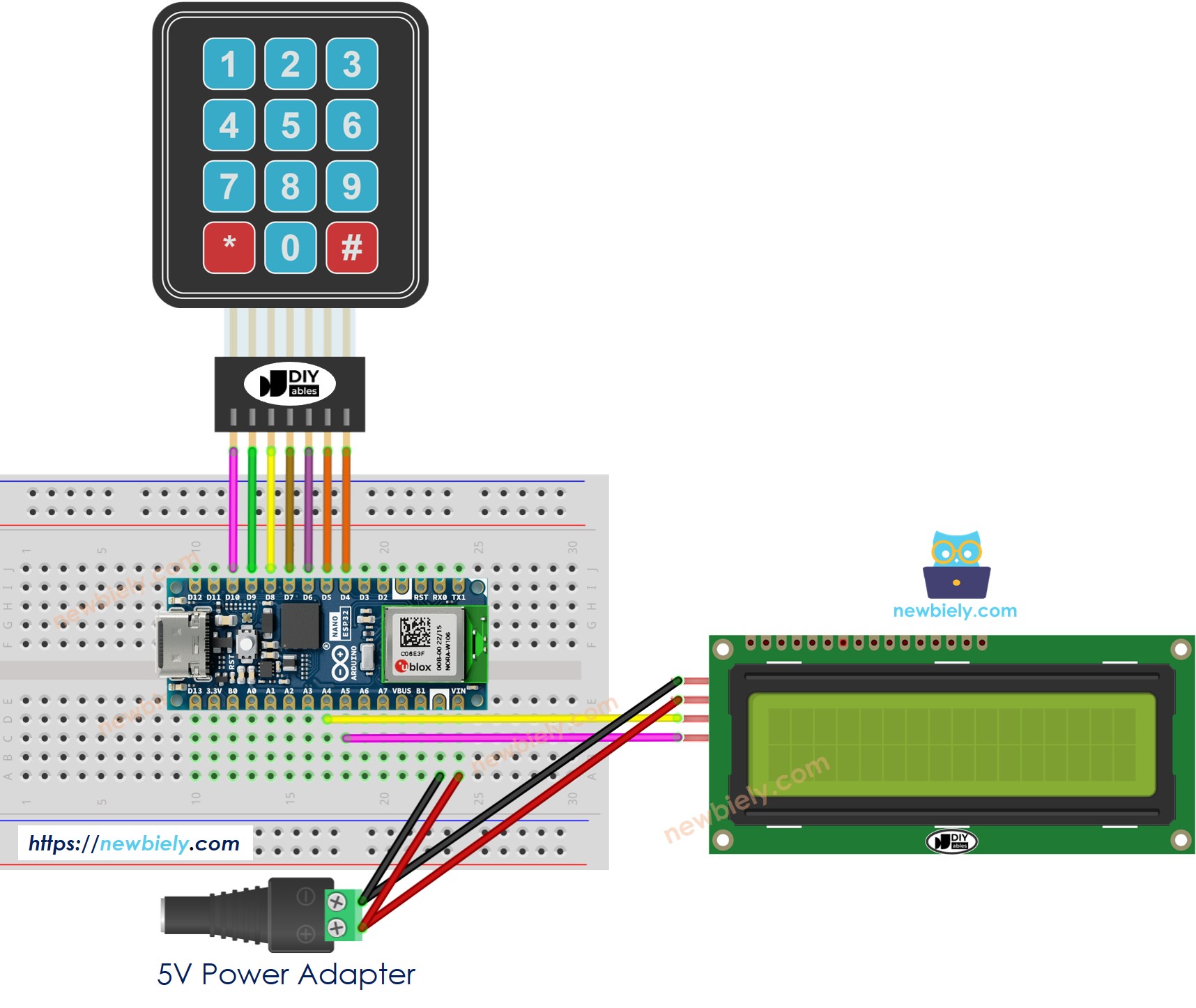
This image is created using Fritzing. Click to enlarge image
Arduino Nano ESP32 Code - Keyoad 3x4 - LCD I2C
※ NOTE THAT:
The LCD I2C address can be different from each manufacturer. In the code, we used address of 0x27 that is specified by DIYables manufacturer
Detailed Instructions
- If this is the first time you use Arduino Nano ESP32, see how to setup environment for Arduino Nano ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the Arduino Nano ESP32 board to your PC via a USB cable
- Open Arduino IDE on your PC.
- Select the right Arduino Nano ESP32 board (e.g. Arduino Nano ESP32 and COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Type “keypad” on the search box, then look for the keypad library by Mark Stanley, Alexander Brevig
- Click Install button to install keypad library.
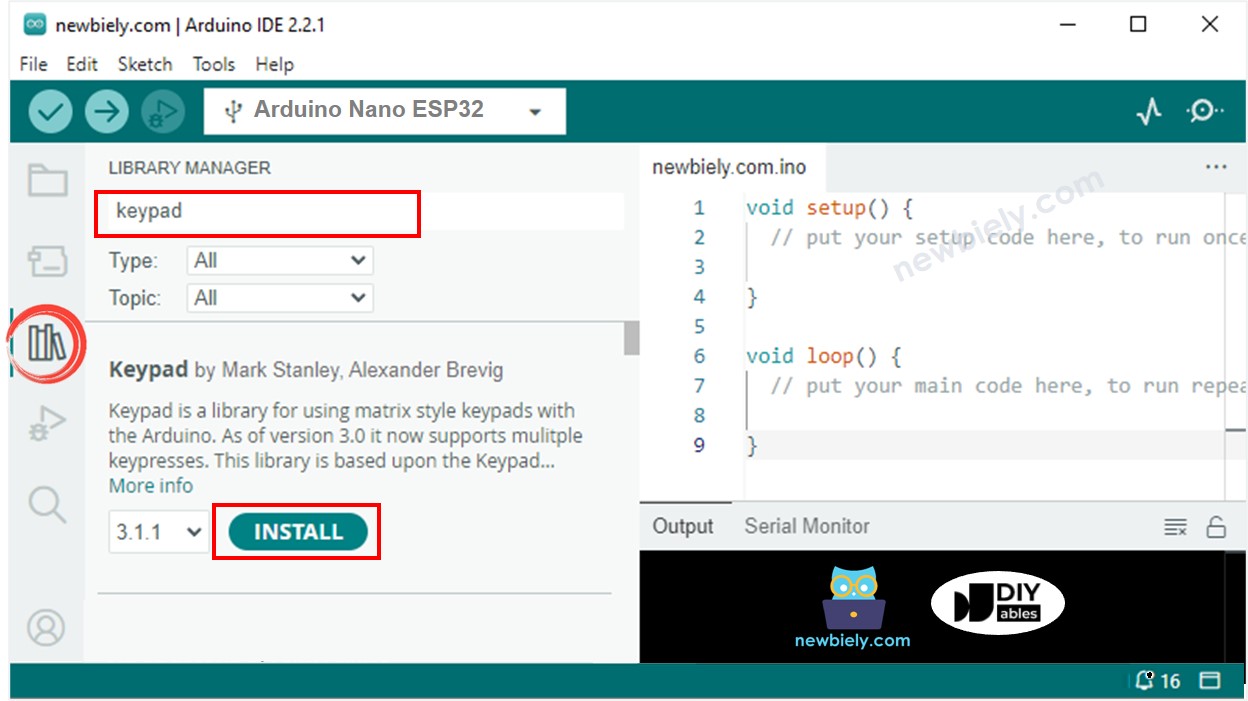
- Type “LiquidCrystal I2C” on the search box, then look for the LiquidCrystal_I2C library by Frank de Brabander
- Click Install button to install LiquidCrystal_I2C library.
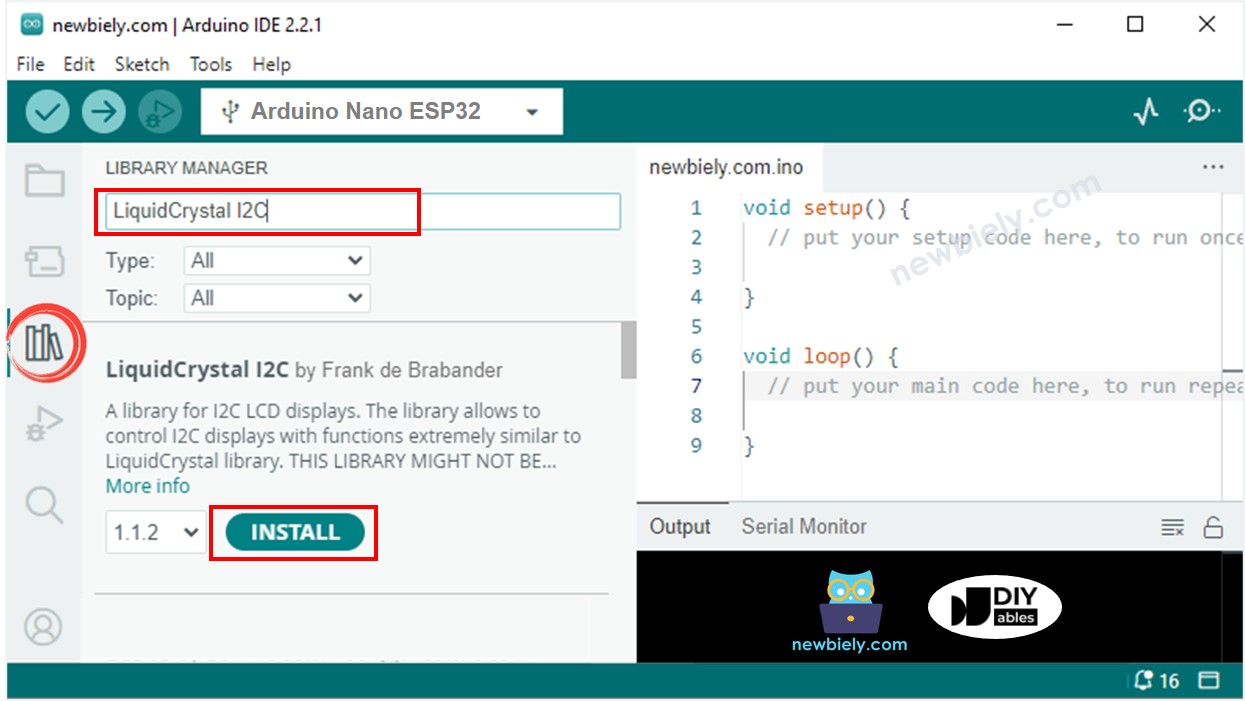
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to Arduino Nano ESP32 board by clicking Upload button on Arduino IDE
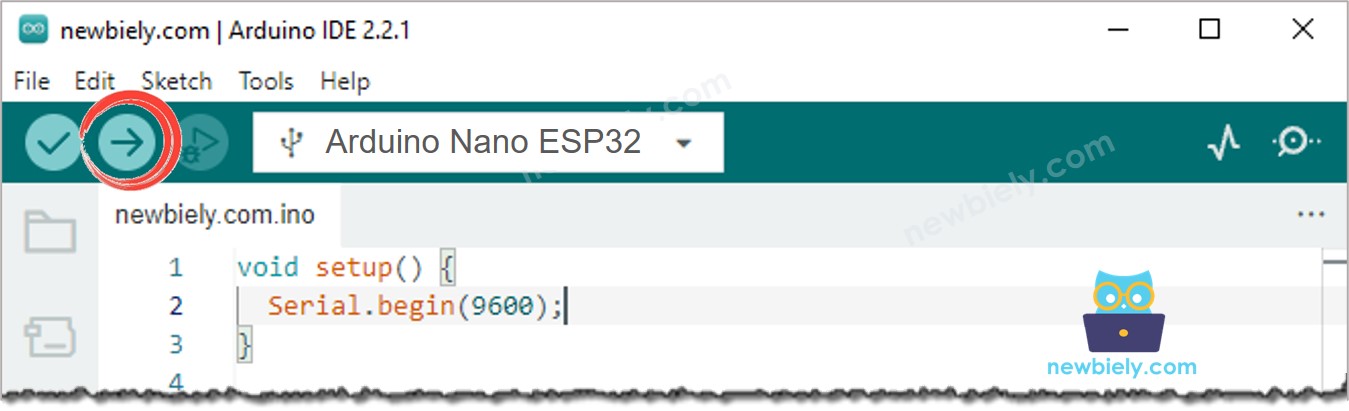
- Press some keys on keypad
- See the result in LCD
If the LCD does not display anything, see Troubleshooting on LCD I2C
Line-by-line Code Explanation
The above Arduino Nano ESP32 code contains line-by-line explanation. Please read the comments in the code!