Communication between two Arduino Nano ESP32 via MQTT
In this tutorial, we'll cover the following:
- Bidirectional communication between two Arduino Nano ESP32s using MQTT.
- Example application: Using a button or switch connected to Arduino Nano ESP32 #1 to control an LED connected to Arduino Nano ESP32 #2 via MQTT.
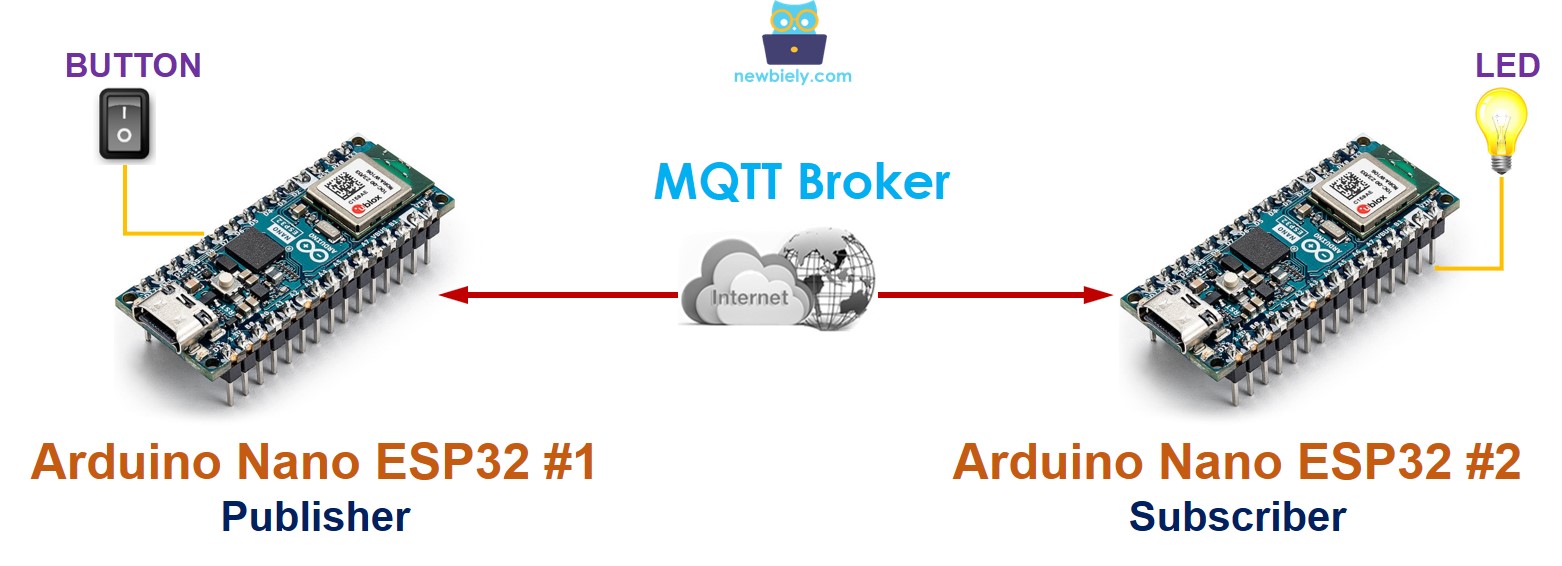
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Arduino Nano ESP32 and MQTT
We have a detailed tutorial on how to use Arduino Nano ESP32 with MQTT here:
Communication between two Arduino Nano ESP32 via MQTT
Two Arduino Nano ESP32 boards can communicate with each other using an MQTT server. If you prefer for them to communicate directly without using an MQTT server, please refer to the tutorial on Arduino Nano ESP32 to Arduino Nano ESP32 TCP Client/Server communication.
When Arduino Nano ESP32 #1 and Arduino Nano ESP32 #2 exchange data via an MQTT broker:
- Both Arduino Nano ESP32s connect to the MQTT broker.
- To have Arduino Nano ESP32 #2 send data to Arduino Nano ESP32 #1:
- Arduino Nano ESP32 #1 subscribes to a topic, for example: arduino-nano-esp32-1/data.
- Arduino Nano ESP32 #2 can send data to Arduino Nano ESP32 #1 by publishing the data to the topic that Arduino Nano ESP32 #1 is subscribed to.
- Similarly, to have Arduino Nano ESP32 #1 send data to Arduino Nano ESP32 #2:
- Arduino Nano ESP32 #2 subscribes to a topic, for example: arduino-nano-esp32-2/data.
- Arduino Nano ESP32 #1 can send data to Arduino Nano ESP32 #2 by publishing the data to the topic that Arduino Nano ESP32 #2 is subscribed to.
Following this method, two Arduino Nano ESP32s can exchange data bidirectionally.
Example Use Case
Let's realize the following application: A button/switch connected to Arduino Nano ESP32 #1 controls an LED connected to Arduino Nano ESP32 #2 via MQTT.
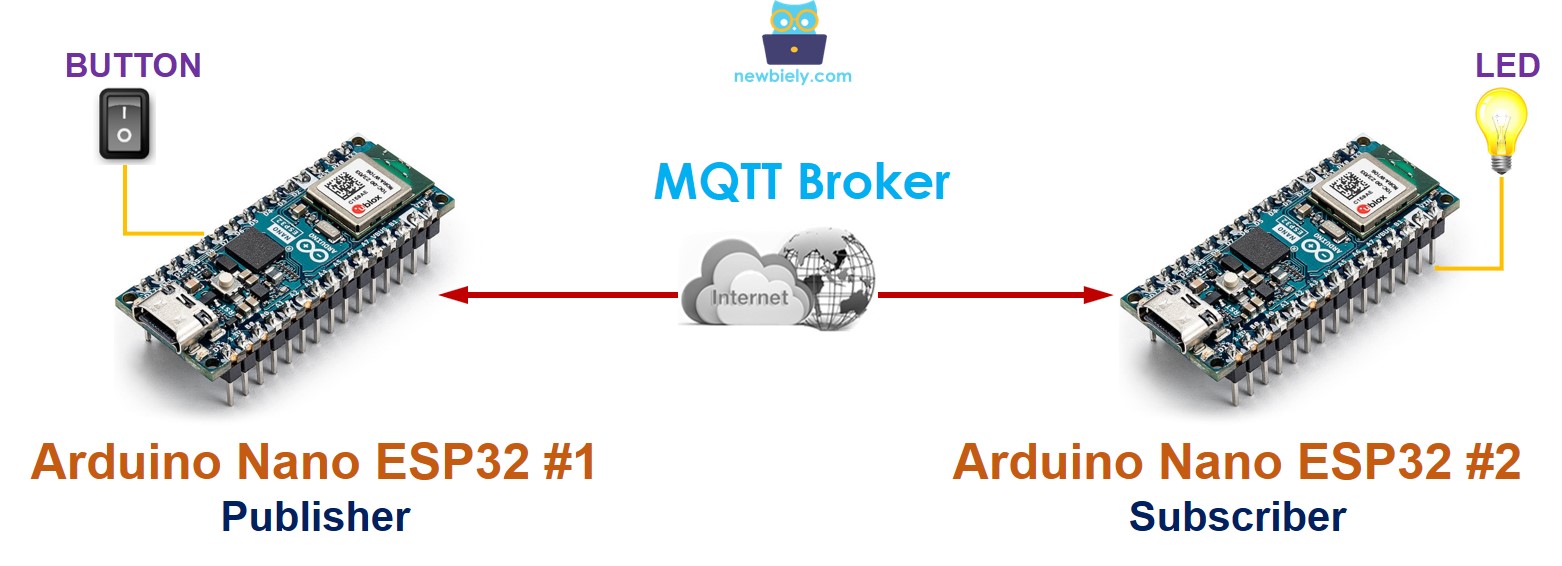
As mentioned above, there are some application protocols we can use. In this example, to make it simple, we will define a protocol by ourself (a self-defined protocol)
How It Works
Let's establish a simple protocol:
- Both Arduino Nano ESP32 #1 and Arduino Nano ESP32 #2 connect to an MQTT Broker (MQTT server).
- For Arduino Nano ESP32 #1:
- Arduino Nano ESP32 #1 sends an MQTT message to a topic whenever the state of a switch changes.
- When the button or switch is turned on, the MQTT message payload is set to 1.
- When the button or switch is turned off, the MQTT message payload is set to 0.
- For Arduino Nano ESP32 #2:
- Arduino Nano ESP32 #2 subscribes to the topic.
- If Arduino Nano ESP32 #2 receives an MQTT message with a payload of 1, it turns on an LED.
- If Arduino Nano ESP32 #2 receives an MQTT message with a payload of 0, it turns off the LED.
- Arduino Nano ESP32 #1 - Wiring diagram between Arduino Nano ESP32 and button
- Arduino Nano ESP32 #2 - Wiring diagram between Arduino Nano ESP32 and LED
Wiring Diagram
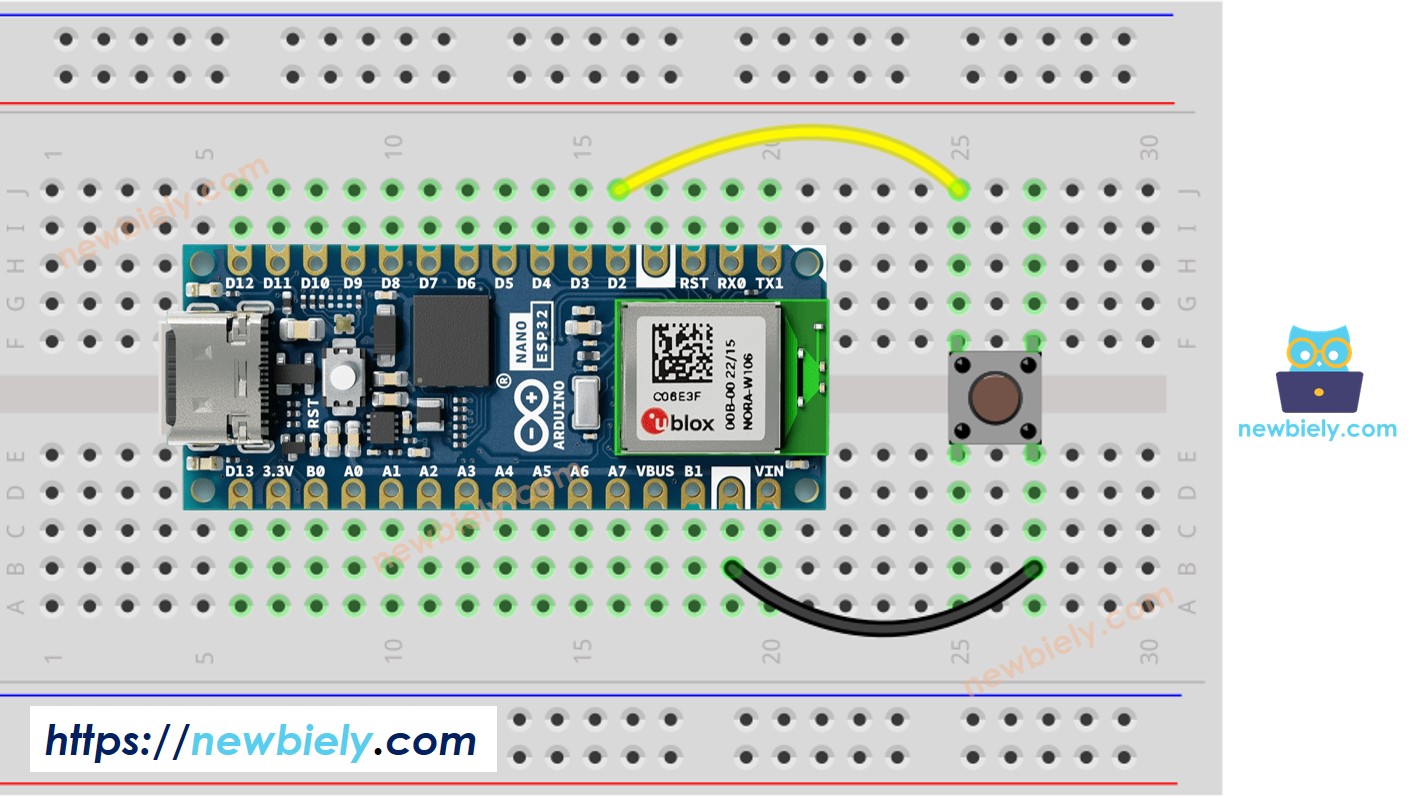
This image is created using Fritzing. Click to enlarge image
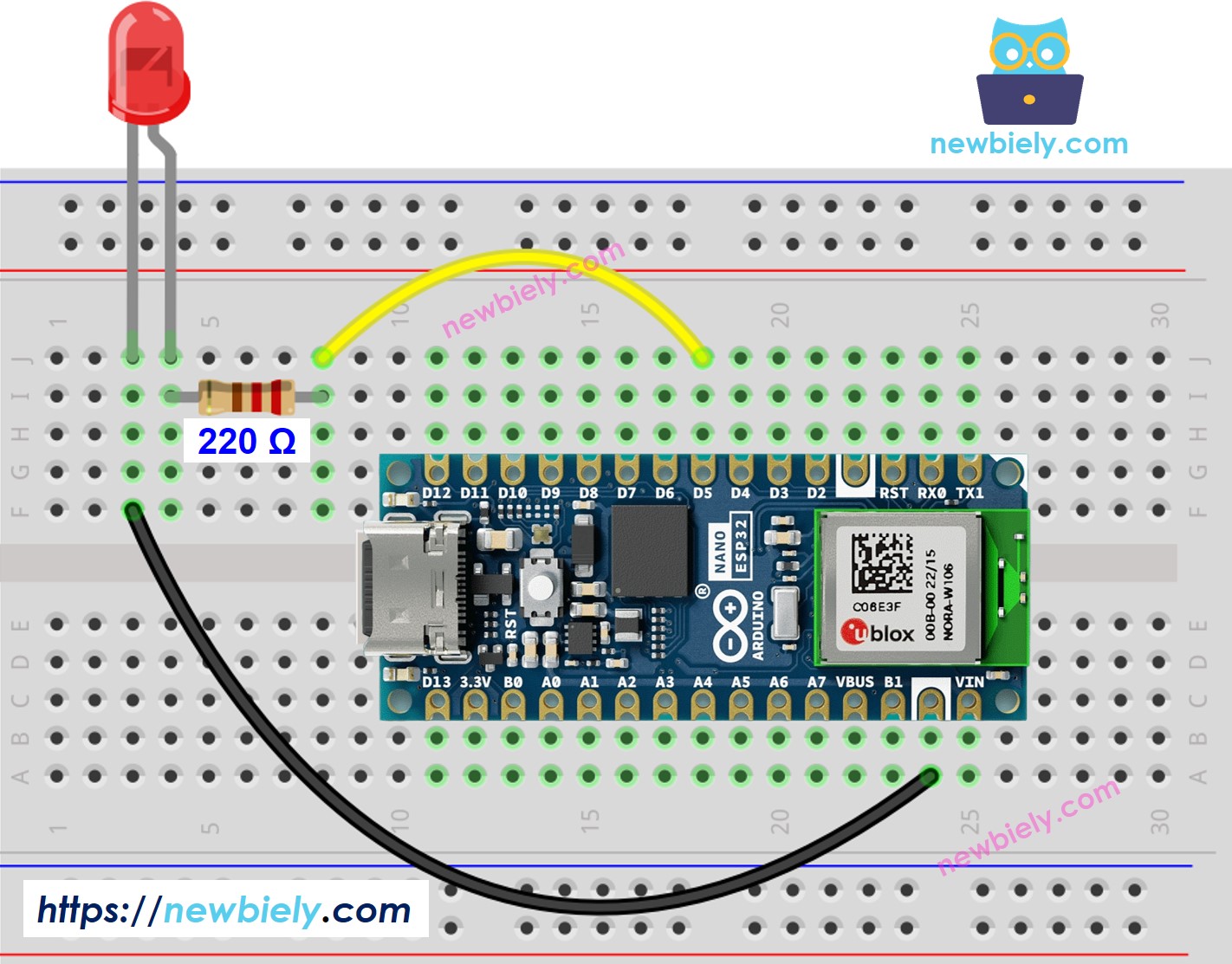
This image is created using Fritzing. Click to enlarge image
Communication between two Arduino Nano ESP32 via MQTT
Arduino Nano ESP32 Code #1
Arduino Nano ESP32 Code #2
Detailed Instructions
To get started with Arduino Nano ESP32, follow these steps:
- If you are new to Arduino Nano ESP32, refer to the tutorial on how to set up the environment for Arduino Nano ESP32 in the Arduino IDE.
- Wire the components according to the provided diagram.
- Connect the Arduino Nano ESP32 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano ESP32 board and its corresponding COM port.
- Wire a button/switch to Arduino Nano ESP32 #1
- Wire an LED to Arduino Nano ESP32 #2
- Open Arduino IDE (called Arduino IDE #1)
- Open another Arduino IDE window (called Arduino IDE #2) by clicking on Arduino IDE icon on your PC (important!())
- Open the Library Manager by clicking on the Library Manager icon on the left navigation bar of Arduino IDE
- Type MQTT on the search box, then look for the MQTT library by Joel Gaehwiler.
- Click Install button to install MQTT library.
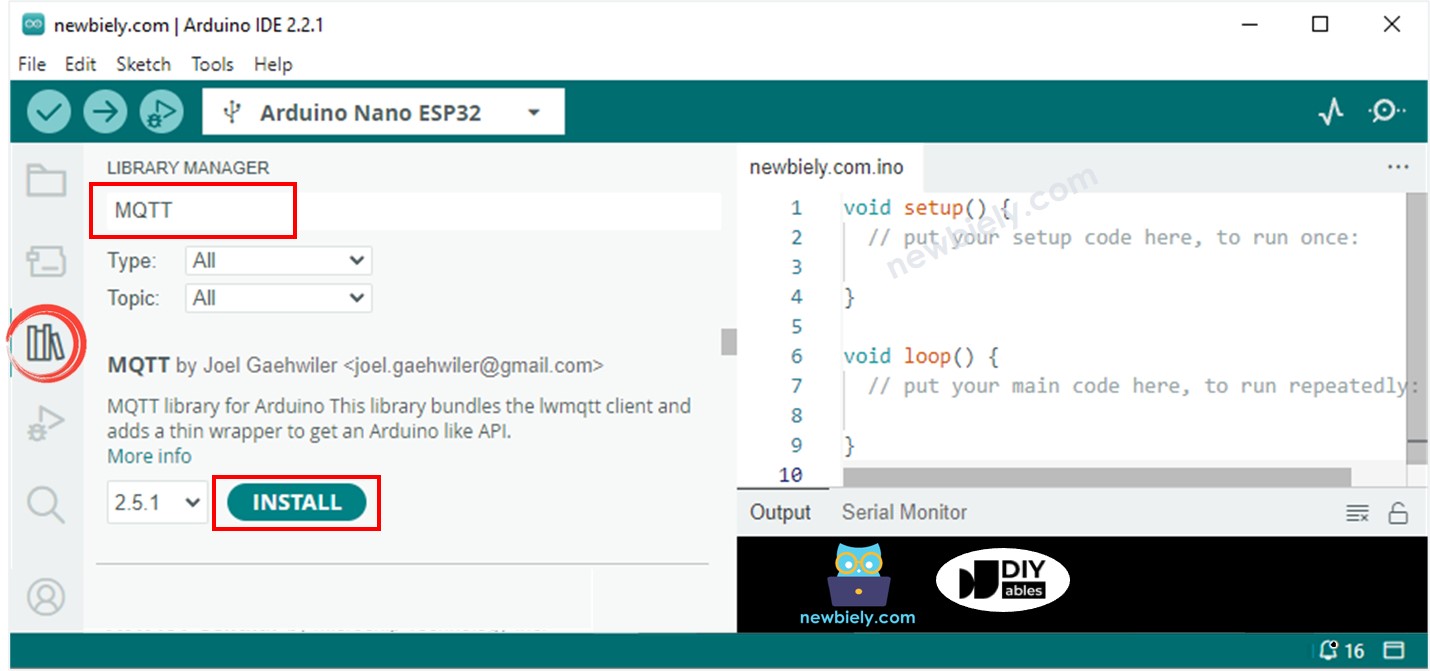
- Type ezButton on the search box, then find the button library by ArduinoGetStarted
- Click Install button to install ezButton library.
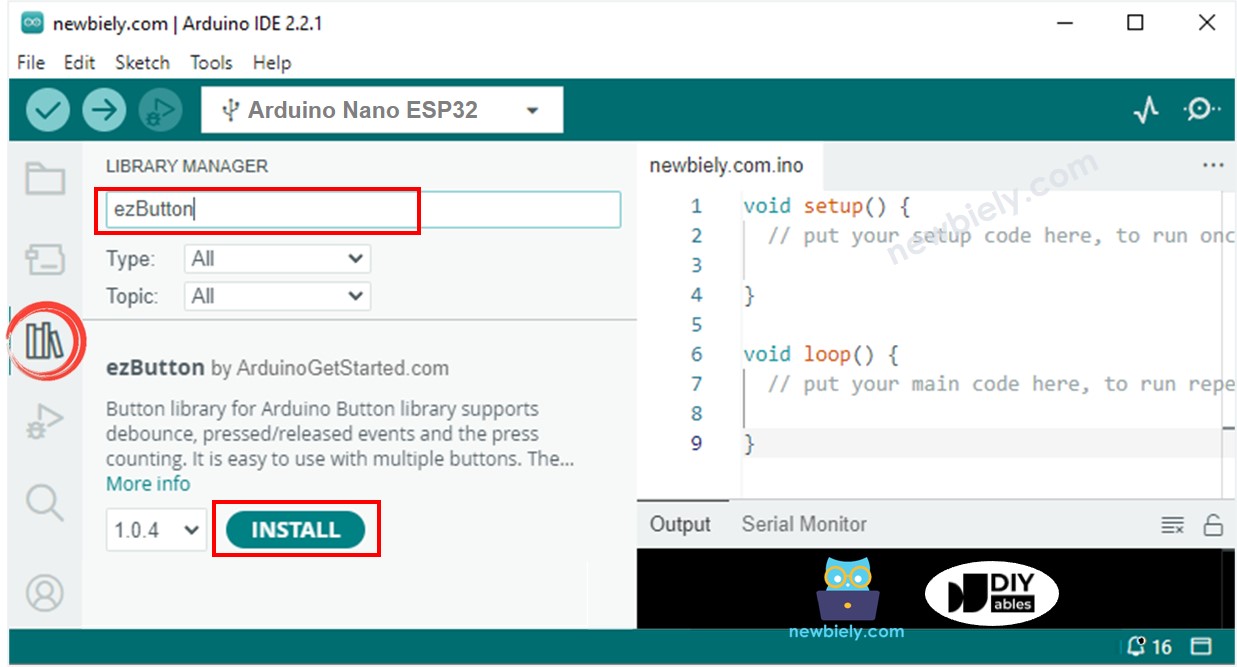
- Connect Arduino Nano ESP32 #1 to PC via USB cable and select COM port of Arduino Nano ESP32 #1 on Arduino IDE #1
- Connect Arduino Nano ESP32 #2 to PC via USB cable and select COM port of Arduino Nano ESP32 #2 on Arduino IDE #2
- Copy Arduino Nano ESP32 #1 code, paste to Arduino IDE #1 and save it (named Arduino Nano ESP32-1)
- Copy Arduino Nano ESP32 #2 code, paste to Arduino IDE #2 and save it (named Arduino Nano ESP32-2)
- Replace the WiFi information (SSID and password) in both codes with your own.
- Replace the MQTT broker address in both codes (domain name or IP address).
- Upload Arduino Nano ESP32 #1 code to Arduino Nano ESP32 #1
- Upload Arduino Nano ESP32 #2 code to Arduino Nano ESP32 #2
- Open Serial Monitor on Arduino IDE #1
- Open Serial Monitor on Arduino IDE #2
- Press and hold the button on Arduino Nano ESP32 #1 → see LED's state on Arduino Nano ESP32 #2 (ON)
- Release button on Arduino Nano ESP32 #1 → see LED's state on Arduino Nano ESP32 #2 (OFF)
- Press, hold, and release the button several times.
- See output on both Serial Monitors
- Serial Monitor of Arduino Nano ESP32 #1
- Serial Monitor of Arduino Nano ESP32 #2