Arduino Nano ESP32 - 74HC595 4-Digit 7-Segment Display
This tutorial guides you on using an Arduino Nano ESP32 to control a 74HC595 4-digit 7-segment display module. It covers the following topics:
- Connecting Arduino Nano ESP32 to the 74HC595 4-digit 7-segment display module
- Programming Arduino Nano ESP32 to display integer numbers on the module
- Programming Arduino Nano ESP32 to display float numbers on the module
- Programming Arduino Nano ESP32 to display numbers and characters on the module.
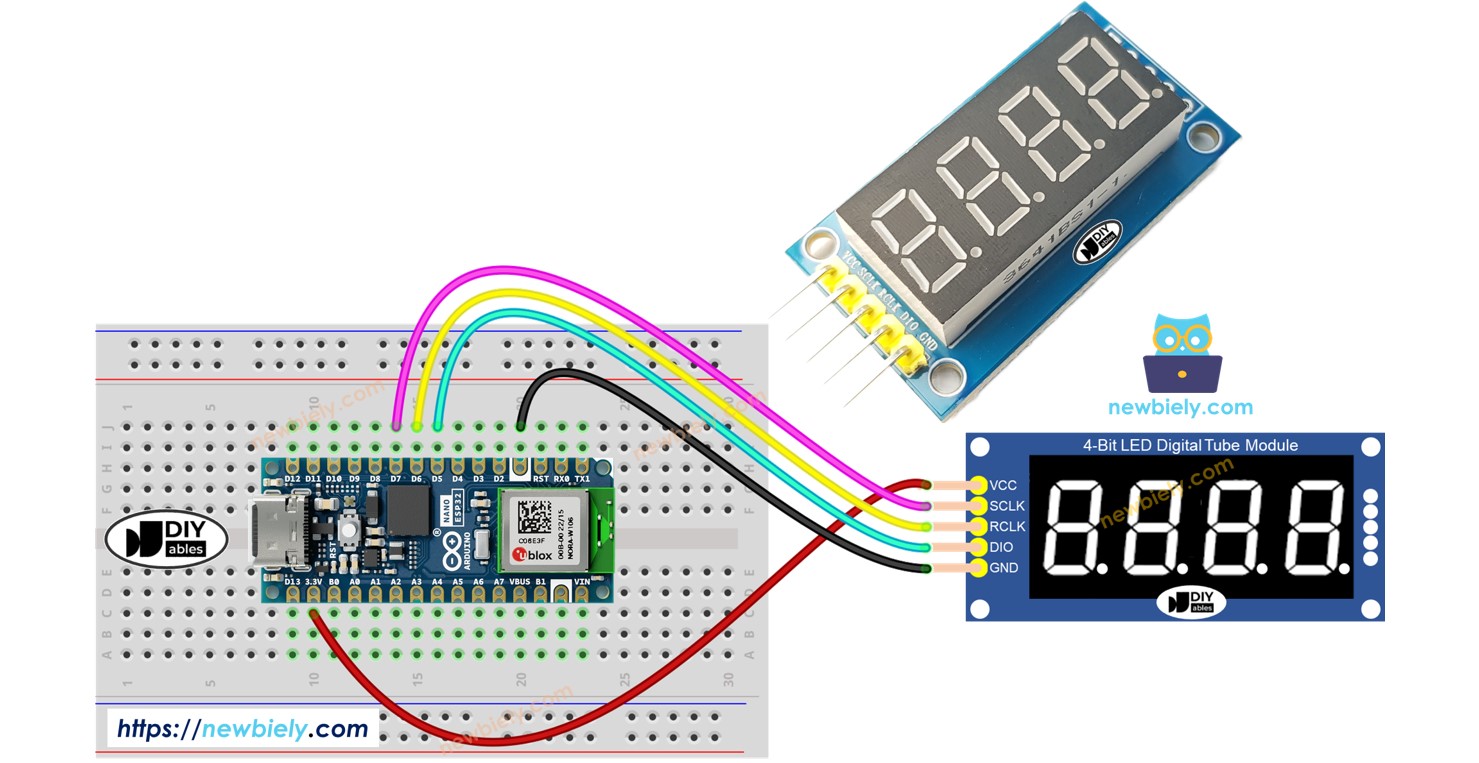
This tutorial will utilize a 4-dot 4-digit 7-segment display module capable of displaying float values. If you need to display a colon separator, please refer to the TM1637 4-digit 7-segment Display Module
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of 74HC595 4-digit 7-segment Display
An ideal module for displaying temperature or any float value is the 74HC595 4-digit 7-segment display. This module typically includes four 7-segment LEDs, four dot-shaped LEDs, and two 74HC595 drivers for each digit.
Pinout
The 74HC595 4-digit 7-segment display module includes 5 pins:
- SCLK pin: is a clock input pin. Connect to any digital pin on Arduino Nano ESP32.
- RCLK pin: is a clock input pin. Connect to any digital pin on Arduino Nano ESP32.
- DIO pin: is a Data I/O pin. Connect to any digital pin on Arduino Nano ESP32.
- VCC pin: supplies power to the module. Connect it to the 3.3V to 5V power supply.
- GND pin: is a ground pin.
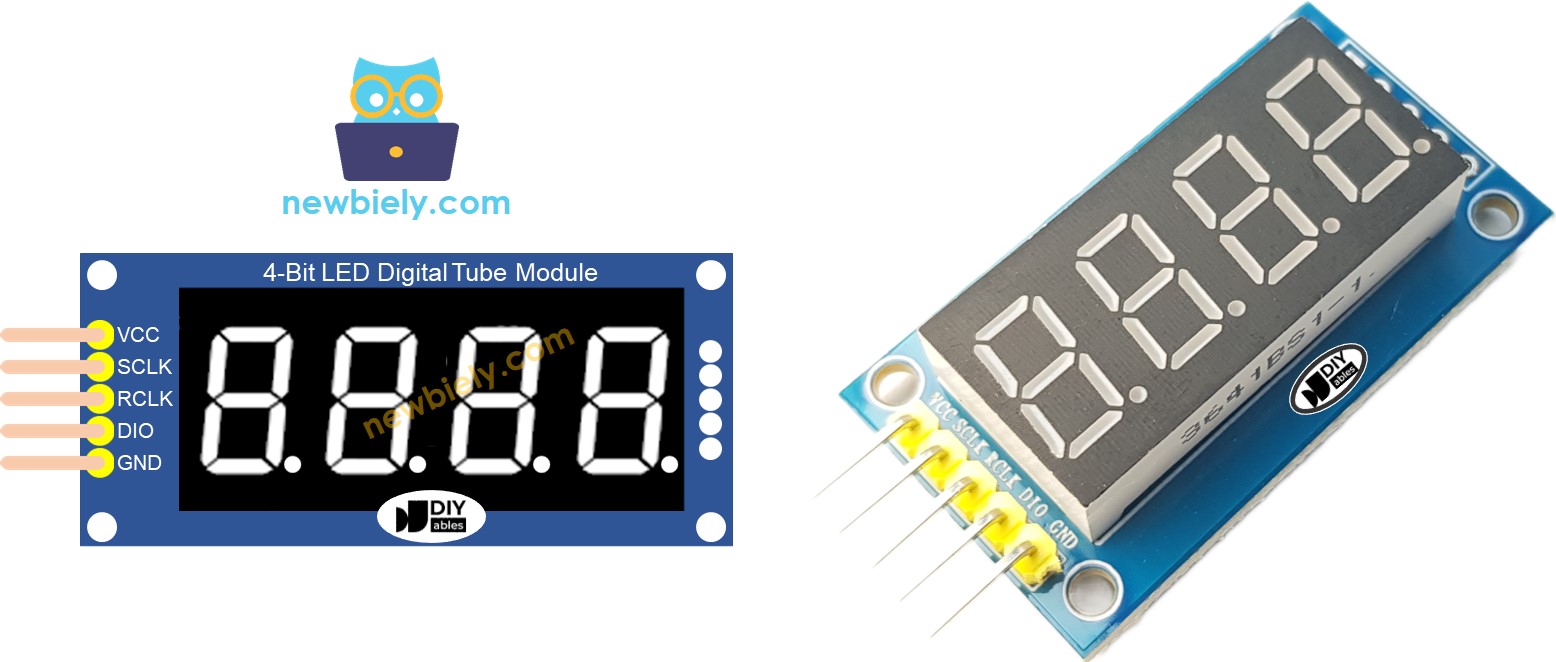
Wiring Diagram
The table below shown the wiring between Arduino Nano ESP32 pins and a 74HC595 4-digit 7-segment display pins:
Arduino Nano ESP32 | 74HC595 7-segment display |
---|---|
3.3V | VCC |
D7 | SCLK |
D6 | RCLK |
D5 | DIO |
If you are using different pins, make sure to modify the pin numbers in the code accordingly.
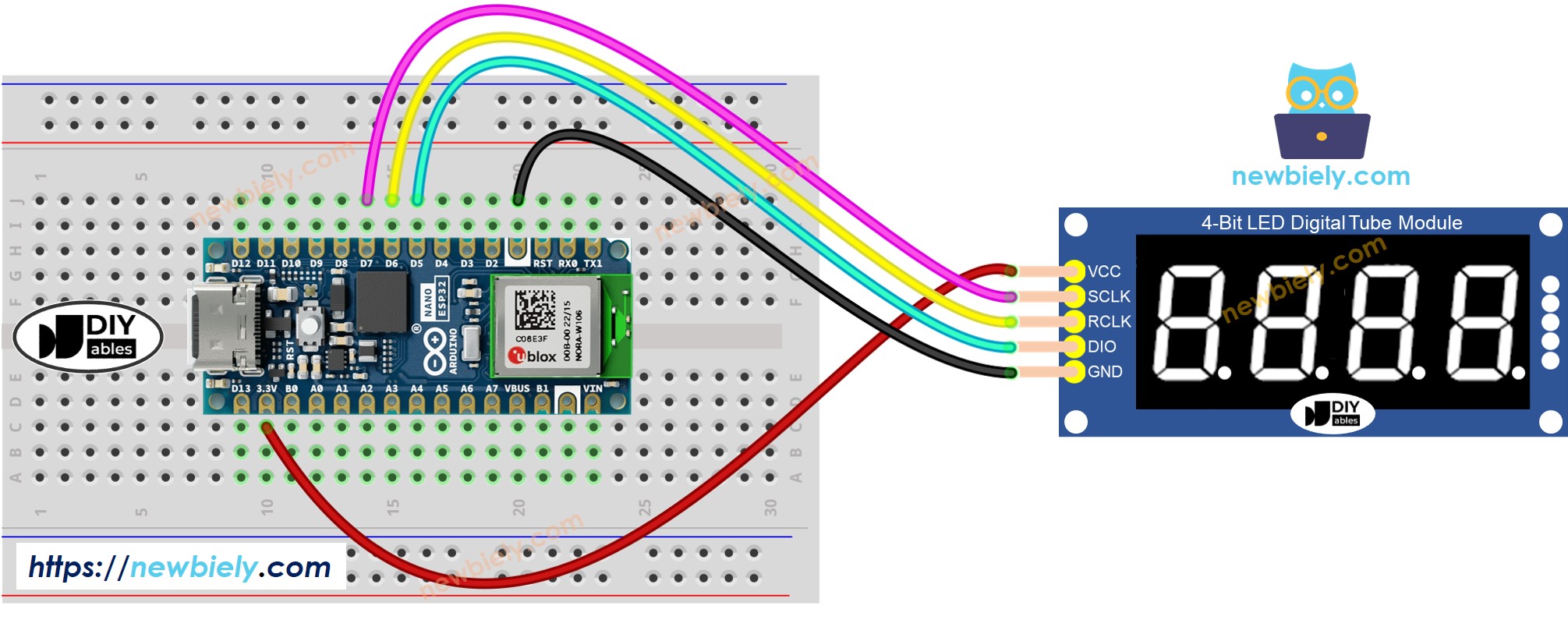
This image is created using Fritzing. Click to enlarge image
Library Installation
To program easily for 74HC595 4-digit 7-segment Display, we need to install DIYables_4Digit7Segment_74HC595 library by DIYables.io. Follow the below steps to install the library:
- Open the Library Manager by clicking on the Library Manager icon on the left navigation bar of Arduino IDE
- Search “DIYables_4Digit7Segment_74HC595”, then find the DIYables_4Digit7Segment_74HC595 library by DIYables.io
- Click Install button.
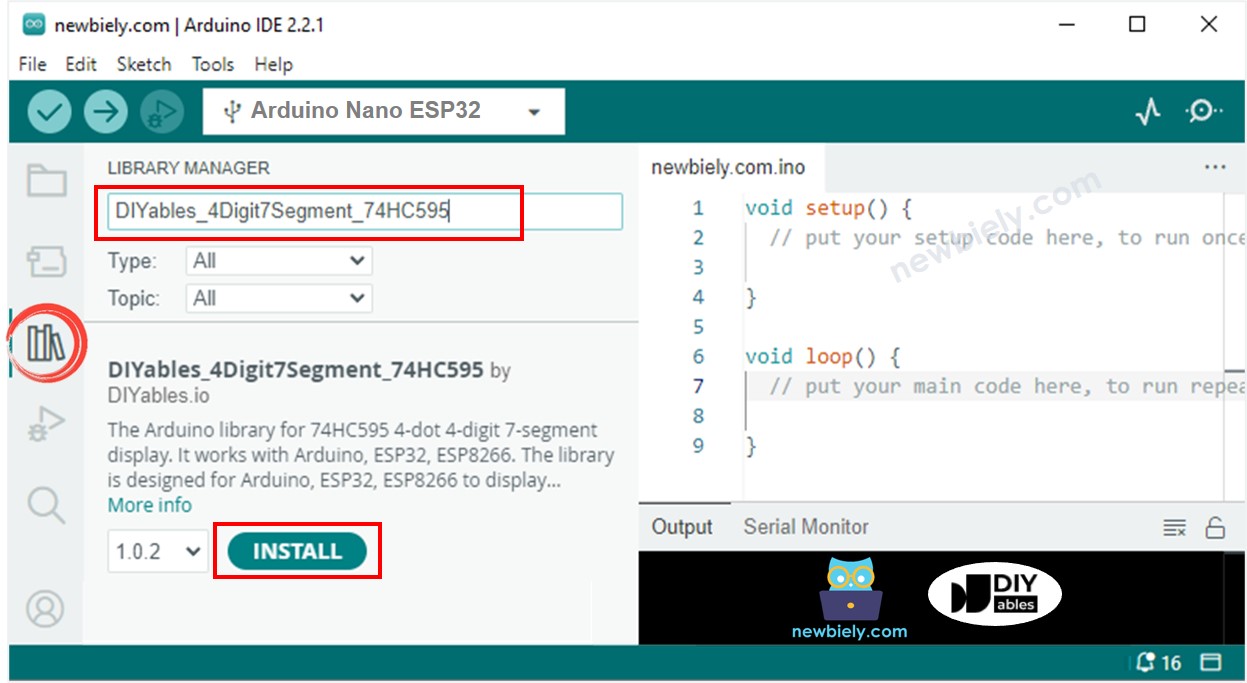
You can also see this library on Github
How To Program For 74HC595 4-digit 7-segment using Arduino Nano ESP32
- Include the library
- Define ESP32's pins that connects to SCLK, RCLK and DIO of the display module. For example, pin D7, D6 and D5
- Create a display object of type DIYables_4Digit7Segment_74HC595
- Then you can display the integer numbers with the zero-padding option, supporting the negative number:
- You can display the float numbers with the decimal place, zero-padding options, supporting the negative number:
- You can also display number, decimal point, character digit-by-digit by using lower-level functions:
- Because the 74HC595 4-digit 7-segment module uses the multiplexing technique to control individual segments and LEDs, Arduino Nano ESP32 code MUST:
- Call display.show() function in the main loop
- Not use delay() function in the main loop
You can see more detail in the the library reference
Arduino Nano ESP32 Code - Display Integer
Detailed Instructions
To get started with Arduino Nano ESP32, follow these steps:
- If you are new to Arduino Nano ESP32, refer to the tutorial on how to set up the environment for Arduino Nano ESP32 in the Arduino IDE.
- Wire the components according to the provided diagram.
- Connect the Arduino Nano ESP32 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano ESP32 board and its corresponding COM port.* Copy the above code and open with Arduino Nano ESP32 IDE
- Click Upload button on Arduino Nano ESP32 IDE to upload code to Arduino Nano ESP32
- See the states of the 7-segment display