Arduino Nano ESP32 - Soil Moisture Sensor
This tutorial provides instructions on how to use Arduino Nano ESP32 to read the soil moisture from sensor. In detail, we will learn:
- Capacitive Moisture Sensor vs Resistive Moisture Sensor
- How to use Arduino Nano ESP32 to read the value from a capacitive moisture sensor
- How to calibrate a capacitive moisture sensor
- How Arduino Nano ESP32 determines if the soil is wet or dry
Hardware Preparation
1 | × | Arduino Nano ESP32 | |
1 | × | USB Cable Type-C | |
1 | × | Capacitive Soil Moisture Sensor | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Optional) DC Power Jack | |
1 | × | (Recommended) Screw Terminal Adapter for Arduino Nano |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Buy Note: Many soil moisture sensors available in the market are unreliable, regardless of their version. We strongly recommend buying the sensor from the DIYables brand using the link provided above. We tested it, and it worked reliably.
Overview of Soil Moisture Sensor Sensor
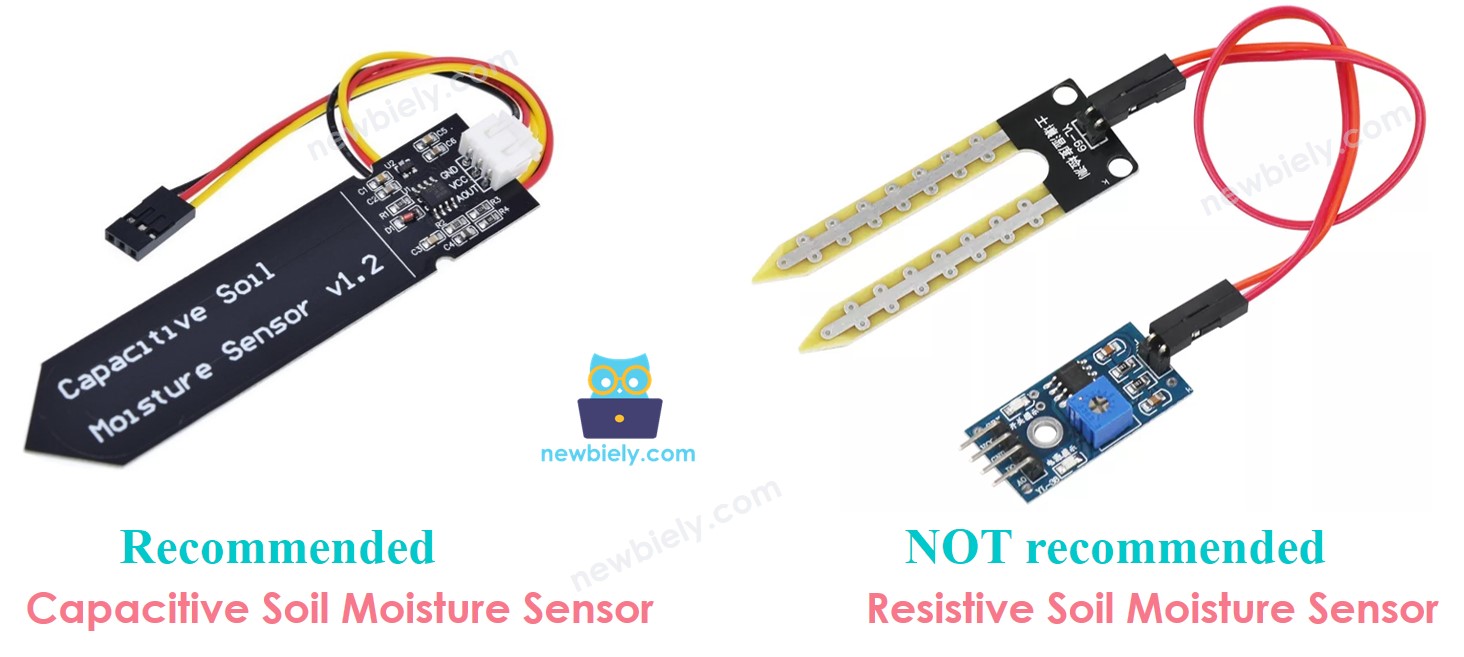
There are two types of moisture sensors:
- Resistive moisture sensor
- Rapacitive moisture sensor.
Both sensors output the soil moisture value. However, their working principles are different. We highly recommend using the capacitive moisture sensor, because of the following reasons:
- The resistive soil moisture sensor corrodes over time. The electrical current that flows between the sensor's probes causes electrochemical corrosion.
- The capacitive soil moisture sensor does NOT corrode over time. The sensor's electrodes are not exposed and no electrical current folows between them.
The below image shows the corrosion on a resistive soil moisture sensor.
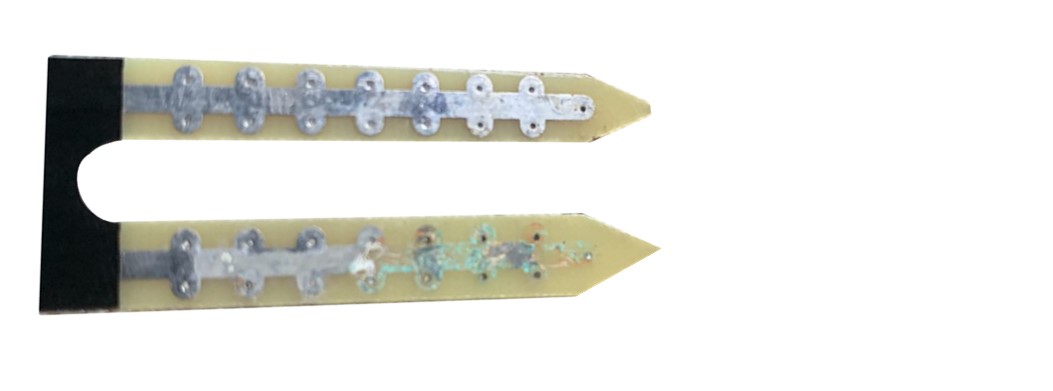
The rest of this tutorial uses the capacitive soil moisture sensor.
Capacitive Soil Moisture Sensor Pinout
A capacitive soil moisture sensor has three pins:
- GND pin: connect this pin to GND (0V)
- VCC pin: connect this pin to VCC (5V or 3.3v)
- AOUT pin: Analog signal output pin outputs the voltage in inverse proportion to the soil moisture level. Connect this pin to an ESP32's analog input pin.
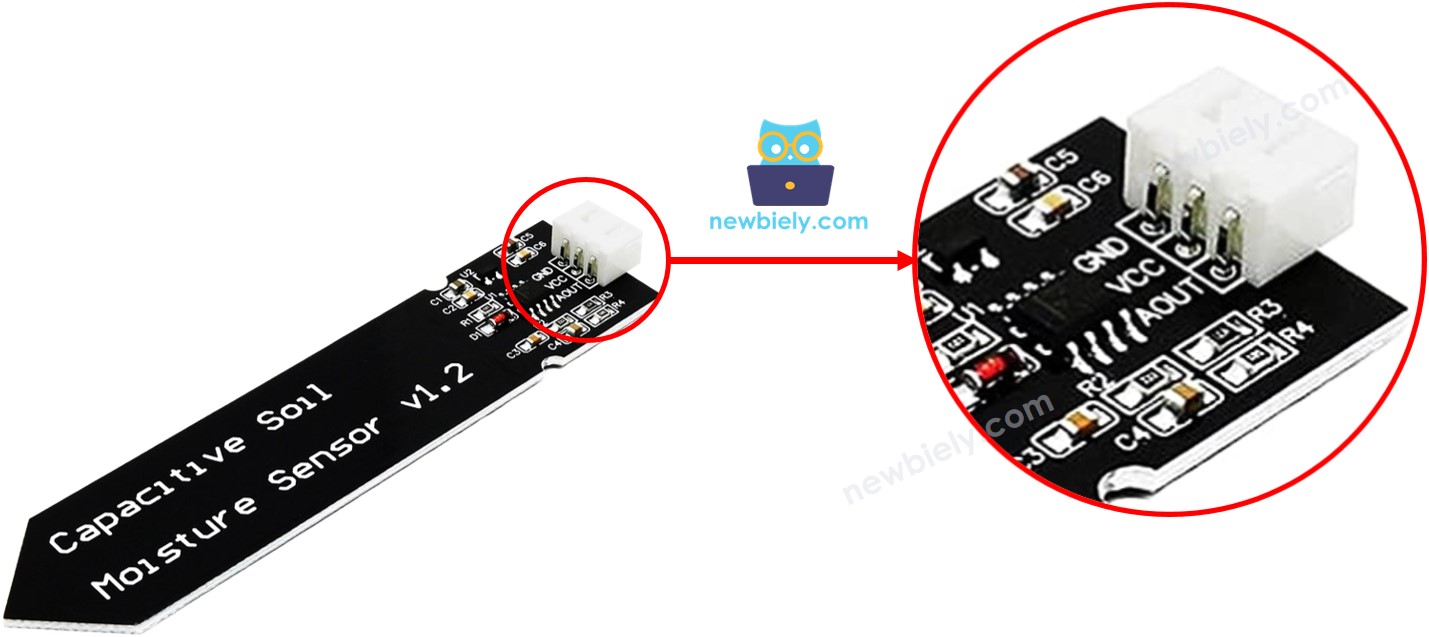
How It Works
The more the water presents in the soil, the lower the voltage in the AOUT pin is
Wiring Diagram
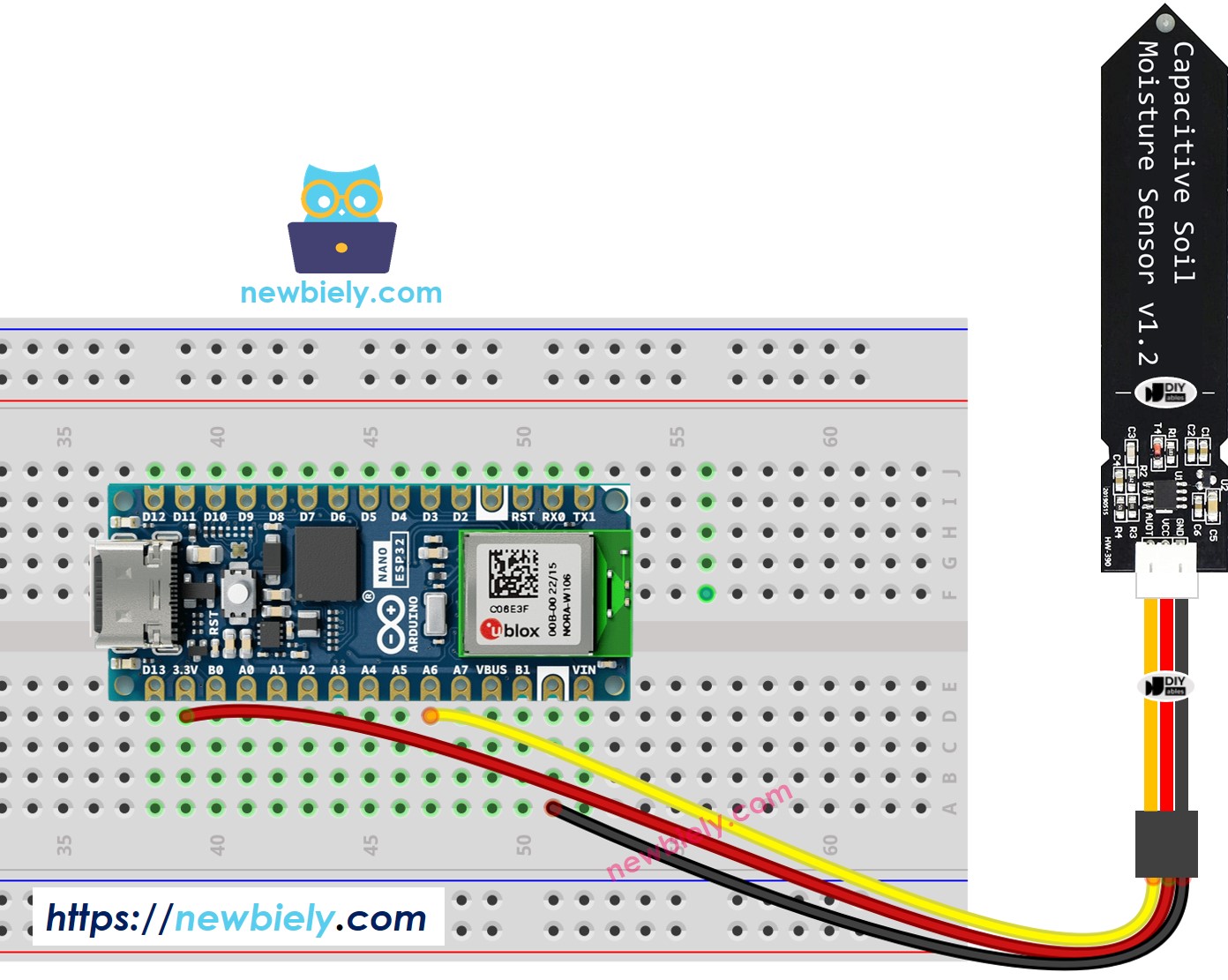
This image is created using Fritzing. Click to enlarge image
Arduino Nano ESP32 Code
Detailed Instructions
- Copy the above code and paste it to Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino Nano ESP32 board
- Bury the sensor in soil, then pour water into the soil. Or slowly submerge it into a cup of salt water.
- Check out the result on Serial Monitor. It looks like the below:
※ NOTE THAT:
- Do not use pure water for testing because it doesn't conduct electricity, so it won't impact sensor readings.
- Sensor readings never drop to zero. It's normal for values to be between 3100 and 2600, although this may change due to factors such as the depth of sensor placement, soil or water composition, and power supply voltage.
- Avoid burying the circuit part (located on top of the sensor) in soil or water, as this could potentially harm the sensor.
Calibration for Capacitive Soil Moisture Sensor
The measured value from the moisture sensor is relative. It depends on the soil's composition and water. In practice, we need to do calibration to determine a threshold that is a border between wet and dry.
How to do calibration:
- Run the above code on Arduino Nano ESP32
- Plant the moisture sensor into the soil
- Plant the moisture sensor into the soil
- Irrigate the soil slowly
- Watch Serial Monitor.
- Write down a value at the time you feel that the soil changes its moisture from dry to wet. This value is called THRESHOLD.
Determine if the soil is wet or dry
After the calibration, update the THRESHOLD value you wrote down to the below code. The below code determines if the soil is wet or dry
The result on Serial Monitor.
※ NOTE THAT:
This tutorial uses the analogRead() function to read values from an ADC (Analog-to-Digital Converter) connected to a sensor or component. The Arduino Nano ESP32's ADC is suitable for projects that do not require high accuracy. However, for projects needing precise measurements, keep the following in mind:
- The Arduino Nano ESP32's ADC is not perfectly accurate and might require calibration for correct results. Each Arduino Nano ESP32 board can vary slightly, so calibration is necessary for each individual board.
- Calibration can be challenging, especially for beginners, and might not always yield the exact results you want.
For projects requiring high precision, consider using an external ADC (e.g ADS1115) with the Arduino Nano ESP32 or using another Arduino, such as the Arduino Uno R4 WiFi, which has a more reliable ADC. If you still want to calibrate the Arduino Nano ESP32's ADC, refer to the ESP32 ADC Calibration Driver.