Arduino Nano ESP32 - LED - Blink
This tutorial provides instructions on how to use Arduino Nano ESP32 to blink an LED. This is one of the first tutorials that beginers learn.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of LED
LED Pinout
LED includes two pins:
- Cathode(-) pin: connect this pin to GND (0V)
- Anode(+) pin: is used to control LED's state
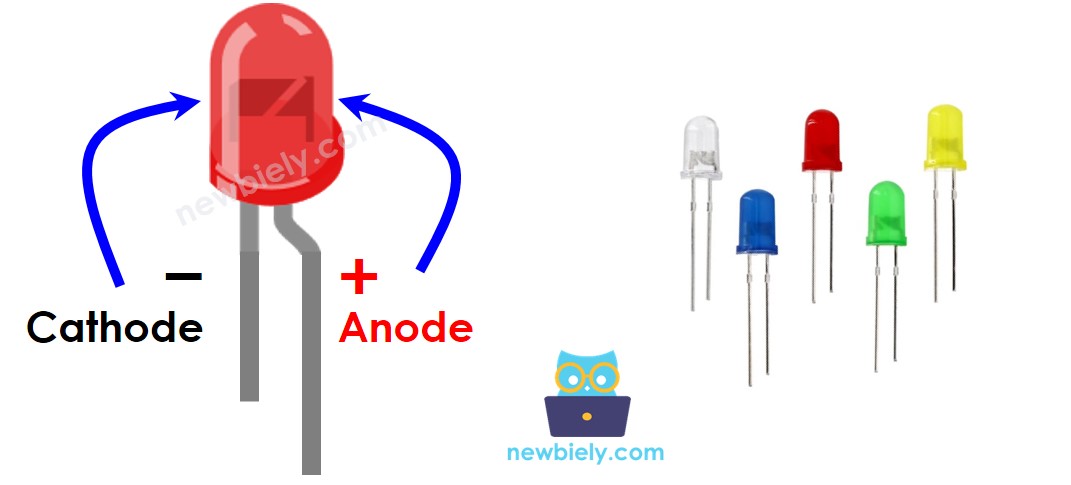
How LED Works
After connecting the cathode(-) to GND:
- If we connect VCC to the anode(+), LED is ON.
- If we connect GND to the anode(+), LED is OFF.
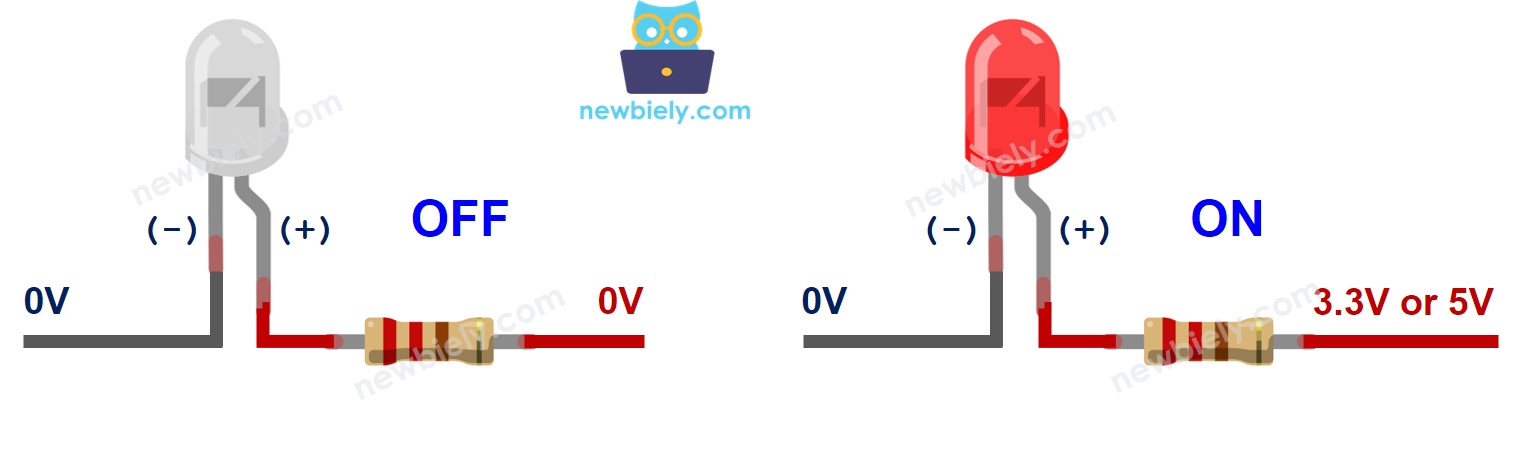
In addition, if a PWM signal is generated to the anode(+), the LED's brightness is changed in proportion to the PWM duty cycle. See more detailed in Arduino Nano ESP32 fade LED tutorial.
※ NOTE THAT:
- Usually, A resistor is required to protect LED from burning. The resistor can be placed between the anode(+) and VCC or between the cathode(-) and GND. The resistance value depends on the LED's specification.
- Some LEDs have a built-in resistor, so there is no need to use a resistor for them.
Arduino Nano ESP32 - LED
The ESP32's digital output pin's voltage can be programmed to VCC or GND. By connecting the digital output pin to LED, we can programmatically control the LED's state.
Wiring Diagram between LED and Arduino Nano ESP32
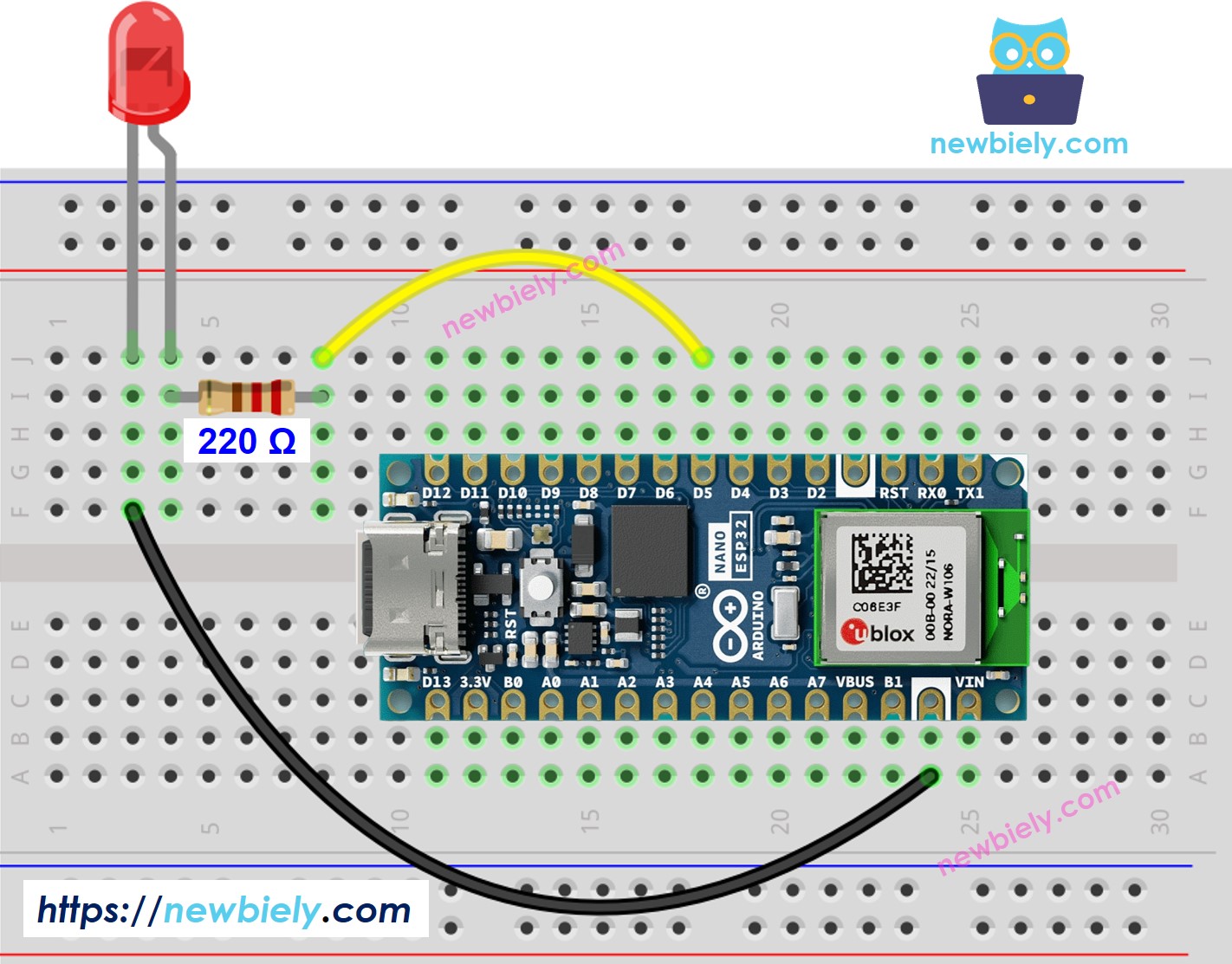
This image is created using Fritzing. Click to enlarge image
How To Program
- Configure an Arduino Nano ESP32's pin to the digital output mode by using pinMode() function. For example, pin D5:
Arduino Nano ESP32 Code
Detailed Instructions
To get started with Arduino Nano ESP32, follow these steps:
- If you are new to Arduino Nano ESP32, refer to the tutorial on how to set up the environment for Arduino Nano ESP32 in the Arduino IDE.
- Wire the components according to the provided diagram.
- Connect the Arduino Nano ESP32 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano ESP32 board and its corresponding COM port.
- Copy the below code and paste it to Arduino IDE.
- Compile and upload code to Arduino Nano ESP32 board by clicking Upload button on Arduino IDE
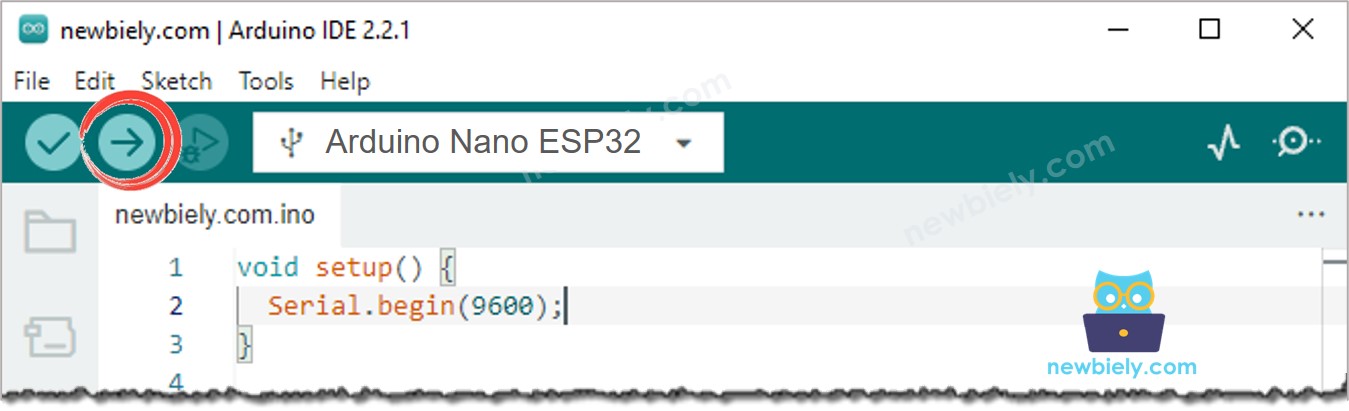
- See the result: The LED blinks one time per second.
Line-by-line Code Explanation
The above Arduino Nano ESP32 code contains line-by-line explanation. Please read the comments in the code!
※ NOTE THAT:
The above code uses the delay(). This function blocks Arduino Nano ESP32 from doing other tasks . To avoid blocking ESP32, see Arduino Nano ESP32 blink without delay