Arduino Nano ESP32 - Touch Sensor
This tutorial provides instructions on how to use Arduino Nano ESP32 with the touch sensor (also known as touch switch, or touch button).
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand, DIYables .
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Touch Sensor
Touch Sensor Pinout
Touch sensor has 3 pins:
- GND pin: connect this pin to GND (0V)
- VCC pin: connect this pin to VCC (5V or 3.3v)
- SIGNAL pin: is an output pin: LOW when NOT touched, HIGH when touched. This pin needs to be connected to ESP32's input pin.
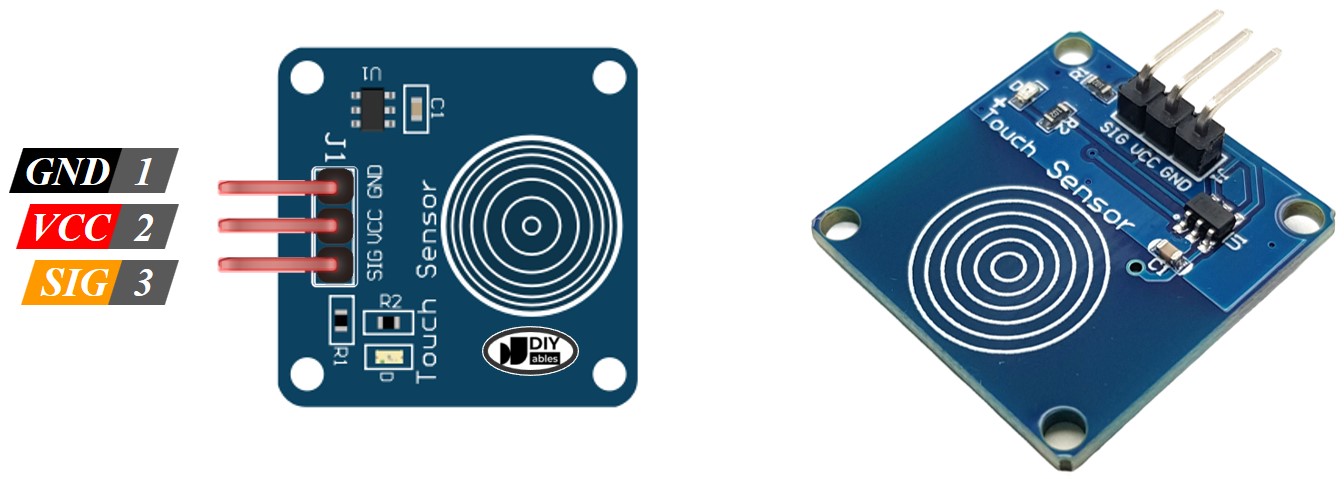
How Touch Sensor Works
- The state of the SIGNAL pin is LOW when the touch sensor is NOT touched
- The state of the SIGNAL pin is HIGH when the touch sensor is touched
Arduino Nano ESP32 - Touch Sensor
We can connect the touch sensor's SIGNAL pin to an ESP32's input pin and use the Arduino Nano ESP32 code to read the state of touch sensor.
Wiring Diagram between Touch Sensor and Arduino Nano ESP32
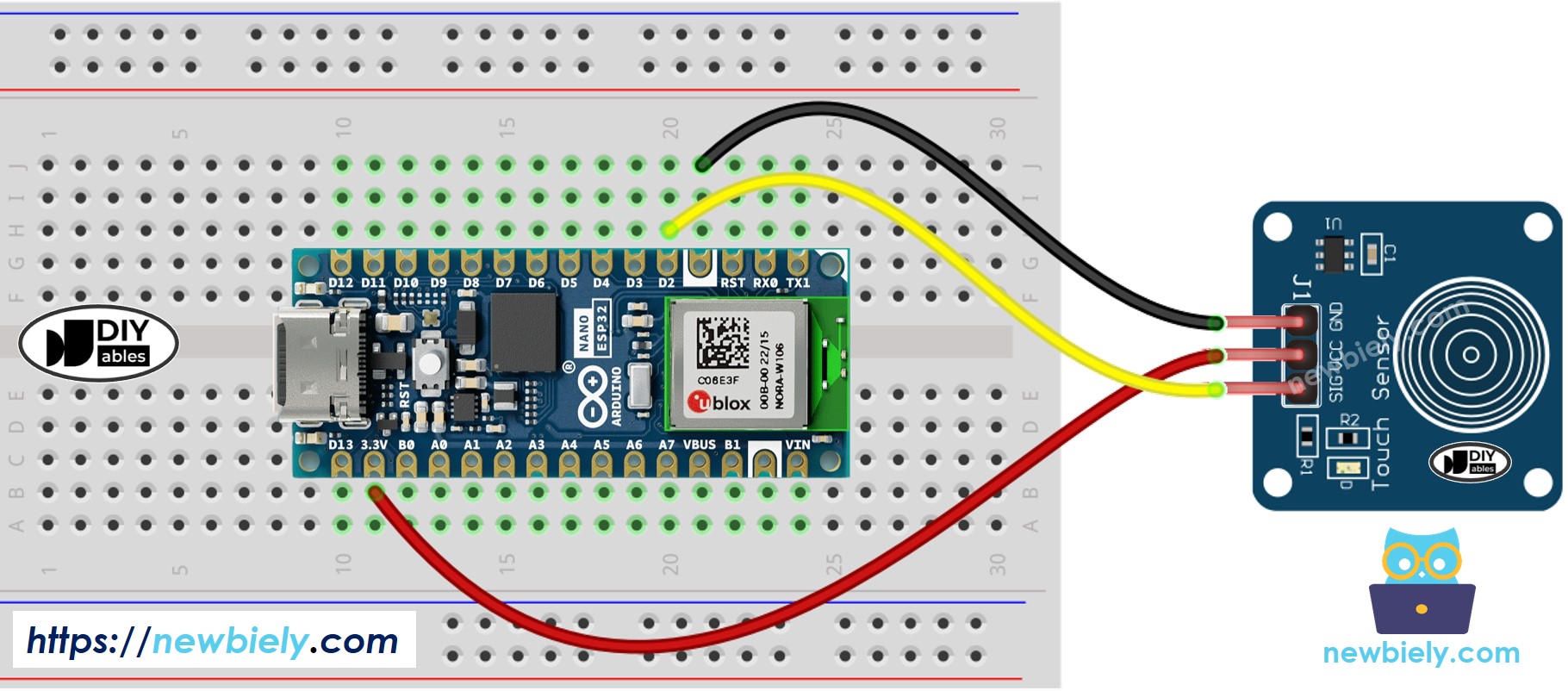
This image is created using Fritzing. Click to enlarge image
How To Program Touch Sensor
- Initializes the Arduino Nano ESP32 pin to the digital input mode by using pinMode() function. For example, pin D2
pinMode(D2, INPUT);
- Reads the state of the Arduino Nano ESP32 pin by using digitalRead() function.
int inputState = digitalRead(D2);
Touch Sensor - Arduino Nano ESP32 Code
The below code reads the state of the touch sensor and print it to the Serial Monitor.
/*
* This Arduino Nano ESP32 code was developed by newbiely.com
*
* This Arduino Nano ESP32 code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-nano-esp32/arduino-nano-esp32-touch-sensor
*/
#define SENSOR_PIN D2 // The Arduino Nano ESP32 pin connected to the sensor's SIGNAL pin of touch sensor
void setup() {
// Initialize the Serial to communicate with the Serial Monitor.
Serial.begin(9600);
// initialize the ESP32's pin as an input
pinMode(SENSOR_PIN, INPUT);
}
void loop() {
// read the state of the the input pin:
int state = digitalRead(SENSOR_PIN);
// print state to Serial Monitor
Serial.println(state);
}
Detailed Instructions
- If this is the first time you use Arduino Nano ESP32, see how to setup environment for Arduino Nano ESP32 on Arduino IDE.
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to Arduino Nano ESP32 board by clicking Upload button on Arduino IDE
- Touch your finger to the sensor and release.
- Check out the result on the Serial Monitor. It looks like the below:
COM6
0
0
0
1
1
1
1
1
1
0
0
Autoscroll
Clear output
9600 baud
Newline
How to detect the state change from LOW to HIGH
/*
* This Arduino Nano ESP32 code was developed by newbiely.com
*
* This Arduino Nano ESP32 code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-nano-esp32/arduino-nano-esp32-touch-sensor
*/
#define SENSOR_PIN D2 // The Arduino Nano ESP32 pin connected to the sensor's SIGNAL pin of touch sensor
int prev_state = LOW; // The previous state from the input pin
int touch_state; // The current reading from the input pin
void setup() {
// Initialize the Serial to communicate with the Serial Monitor.
Serial.begin(9600);
// initialize the ESP32's pin as an input
pinMode(SENSOR_PIN, INPUT);
}
void loop() {
// read the state of the the input pin:
touch_state = digitalRead(SENSOR_PIN);
if (prev_state == LOW && touch_state == HIGH)
Serial.println("The sensor is touched");
// save the the last state
prev_state = touch_state;
}