Arduino Nano ESP32 - LDR Module
The LDR light sensor module can sense and measure light around it. It has two outputs: a digital output that can be either LOW or HIGH, and an analog output.
In this tutorial, we will learn how to use an Arduino Nano ESP32 and an LDR light sensor module together to detect and measure the amount of light. Here's what we'll cover:
- How to connect the LDR light sensor module to an Arduino Nano ESP32.
- How to program the Arduino Nano ESP32 to detect light by reading the digital signal from the LDR light sensor module.
- How to program the Arduino Nano ESP32 to measure the light level by reading the analog signal from the LDR light sensor module.
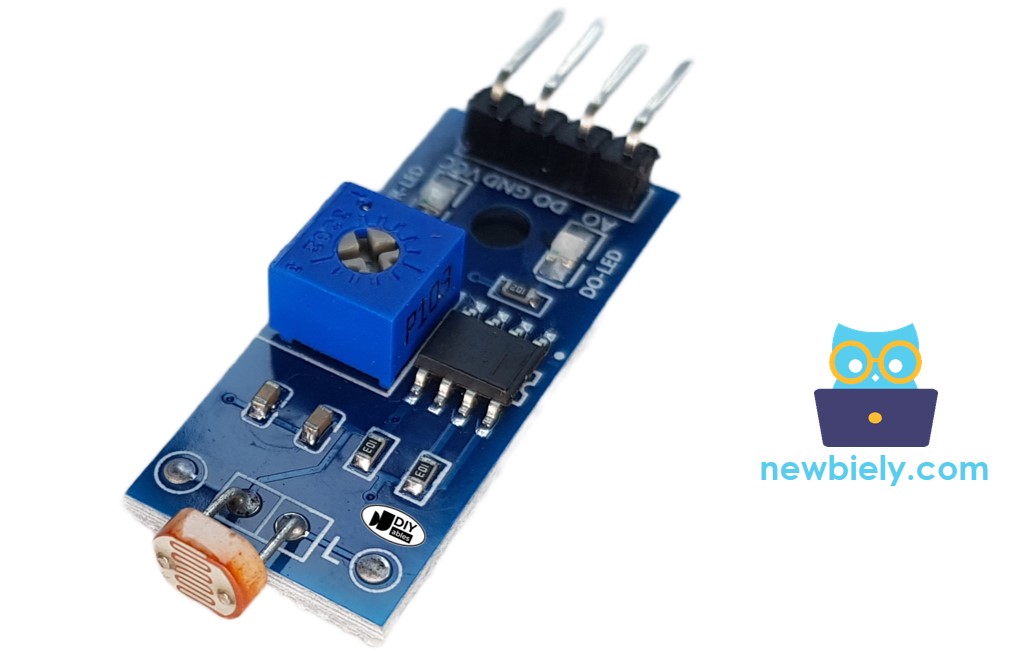
Afterward, you can change the code to make an LED or a light bulb turn on (using a relay) when it senses light.
If you're interested in a light sensor in its raw form, I suggest exploring the tutorial for the Arduino Nano ESP32 - Light Sensor.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of LDR Light Sensor Module
The LDR light sensor module can be used to find out if there is light or how much light there is in the area around it. It has a digital output pin and an analog output pin for different options.
Pinout
The LDR light sensor module has four pins:
- VCC pin: Connect this pin to the power source (between 3.3V to 5V).
- GND pin: Connect this pin to the ground (0V).
- DO pin: This is a digital output pin. It gives a HIGH signal when it's dark and LOW when it's light. You can adjust the threshold between dark and light using a built-in potentiometer.
- AO pin: This is an analog output pin. The value decreases as the light gets brighter and increases as the light gets darker.
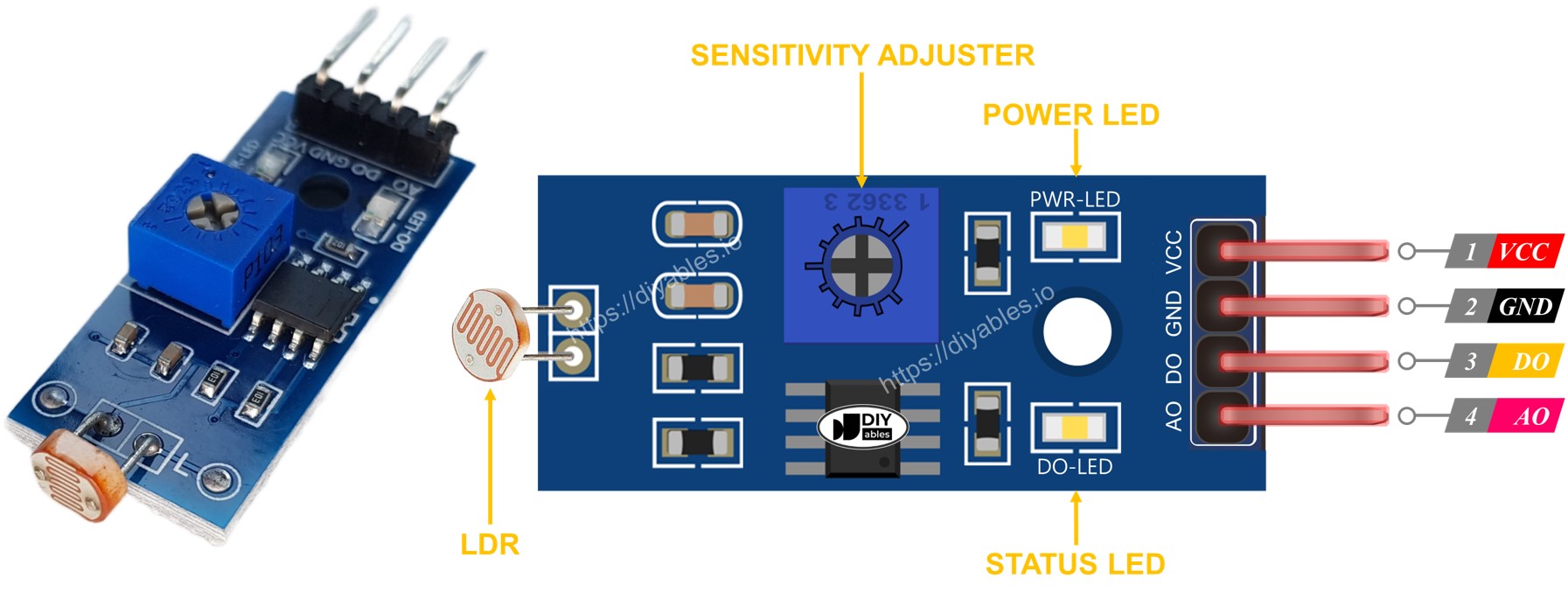
Additionally, the LDR light sensor module is equipped with two LED indicators:
- The PWR-LED indicator shows the power status.
- The DO-LED indicator reflects the state of light on the DO pin: it illuminates when there is light and remains off when it is dark.
How It Works
Regarding the DO pin:
- The LDR light sensor module has a potentiometer that allows you to adjust the sensitivity or threshold for detecting light.
- When the light intensity in the surrounding environment is above the set threshold (considered as light), the output of the sensor on the DO pin becomes LOW, and the DO-LED turns on.
- When the light intensity in the surrounding environment is below the set threshold (considered as dark), the output of the sensor on the DO pin becomes HIGH, and the DO-LED turns off.
Regarding the AO pin:
- The value read from the AO pin is inversely proportional to the light intensity in the surrounding environment. In other words, as the light intensity increases (brighter), the value on the AO pin decreases.
- Similarly, as the light intensity decreases (darker), the value on the AO pin increases.
It's important to note that adjusting the potentiometer does not affect the value on the AO pin.
Wiring Diagram
Since the light sensor module has two outputs, you can choose to use one or both of them, depending on what you need.
- The wiring diagram between Arduino Nano ESP32 and the LDR light sensor module when using DO only.
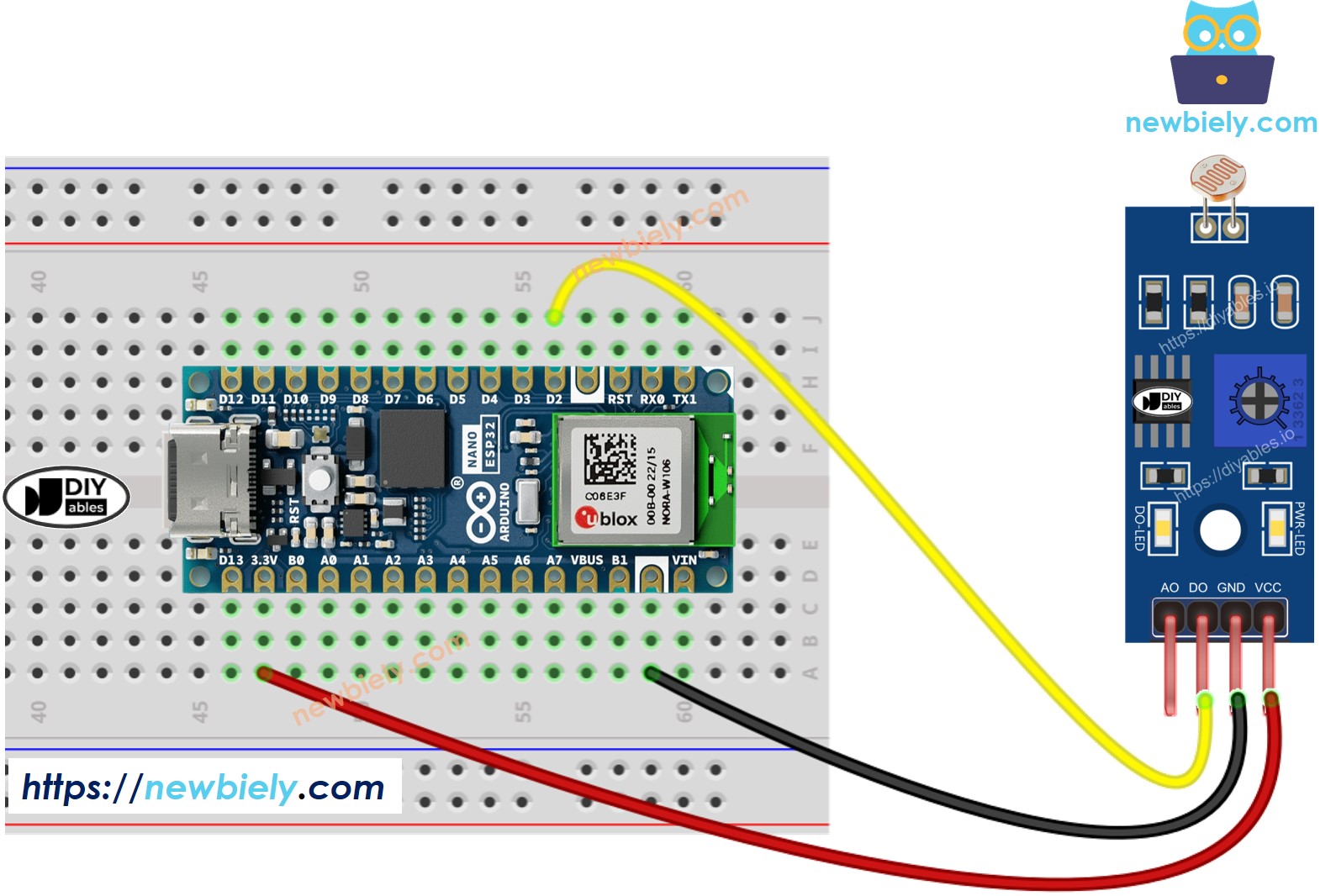
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between Arduino Nano ESP32 and the LDR light sensor module when using AO only.
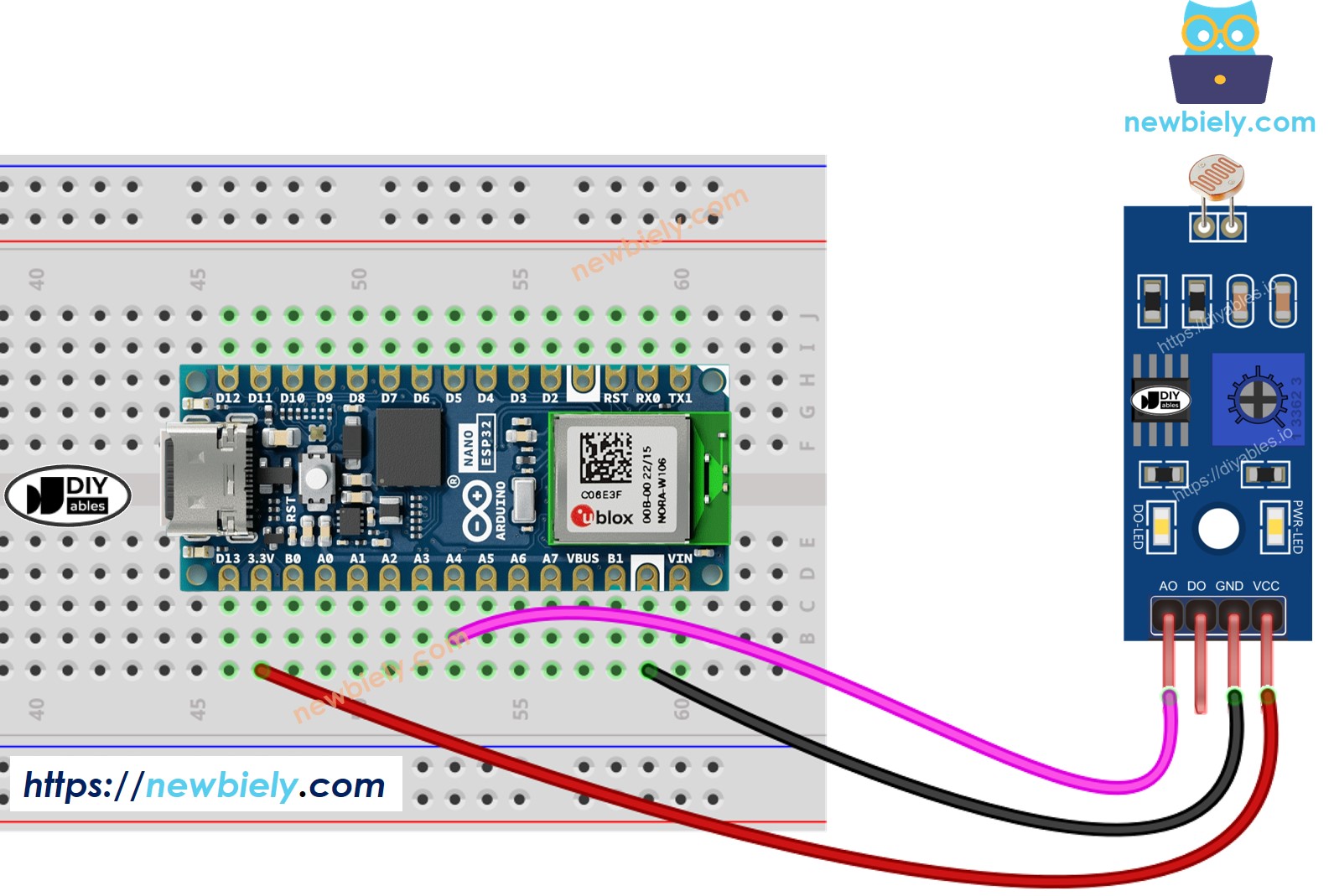
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between Arduino Nano ESP32 and the LDR light sensor module when using both AO an DO.
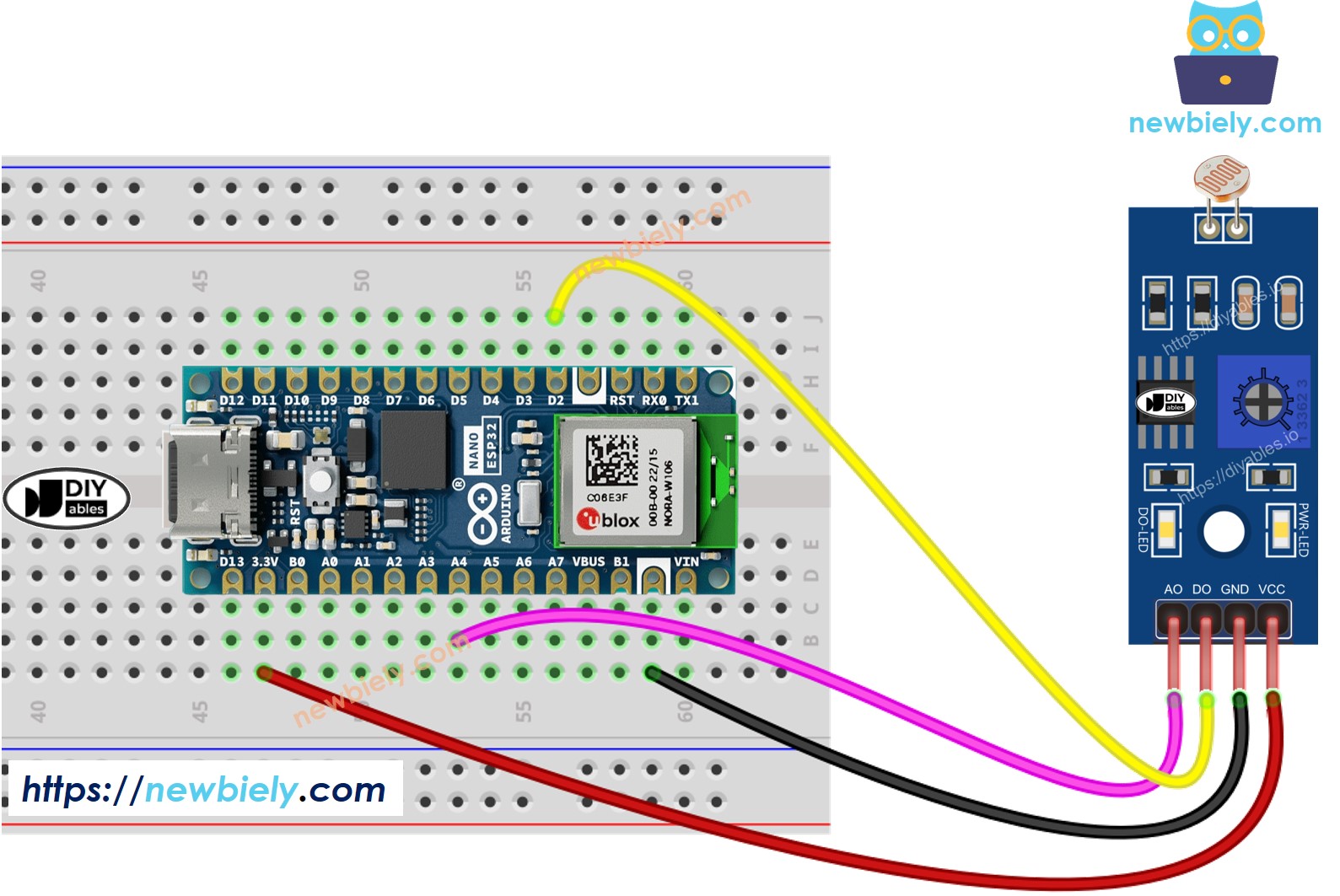
This image is created using Fritzing. Click to enlarge image
Arduino Nano ESP32 Code - Read value from DO pin
Detailed Instructions
To get started with Arduino Nano ESP32, follow these steps:
- If you are new to Arduino Nano ESP32, refer to the tutorial on how to set up the environment for Arduino Nano ESP32 in the Arduino IDE.
- Wire the components according to the provided diagram.
- Connect the Arduino Nano ESP32 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano ESP32 board and its corresponding COM port.
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino Nano ESP32
- Cover and uncover the LDR light sensor module by your hand or something
- Check out the result on the Serial Monitor.
If you observe that the LED status remains constantly on or off, regardless of the presence of light, you have the option to adjust the potentiometer. This adjustment allows you to finely tune the light sensitivity of the sensor.
Furthermore, the code can be modified according to your requirements. For instance, you can program the LED to activate or the light to turn on when light is detected. Additionally, you have the flexibility to make a servo motor rotate. Detailed instructions and tutorials on these customization options can be found at the end of this guide.
Arduino Nano ESP32 Code - Read value from AO pin
Detailed Instructions
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino Nano ESP32
- Cover and uncover the LDR light sensor module by your hand or something
- Check out the result on the Serial Monitor.
※ NOTE THAT:
This tutorial uses the analogRead() function to read values from an ADC (Analog-to-Digital Converter) connected to a sensor or component. The Arduino Nano ESP32's ADC is suitable for projects that do not require high accuracy. However, for projects needing precise measurements, keep the following in mind:
- The Arduino Nano ESP32's ADC is not perfectly accurate and might require calibration for correct results. Each Arduino Nano ESP32 board can vary slightly, so calibration is necessary for each individual board.
- Calibration can be challenging, especially for beginners, and might not always yield the exact results you want.
For projects requiring high precision, consider using an external ADC (e.g ADS1115) with the Arduino Nano ESP32 or using another Arduino, such as the Arduino Uno R4 WiFi, which has a more reliable ADC. If you still want to calibrate the Arduino Nano ESP32's ADC, refer to the ESP32 ADC Calibration Driver.