Arduino UNO R4 - LCD Keypad Shield
In this guide, we’ll explore how to use Arduino Uno R4 with the LCD Keypad Shield, a cool little gadget that mixes a 16x2 LCD screen with six buttons (Right, Up, Down, Left, Select, and Reset). You’ll learn how it works, how to hook it up to your Arduino Uno R4, and how to code it step-by-step. We’ve got wiring image, and code examples to make it super easy to follow!
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
What’s the LCD Keypad Shield?
This LCD Keypad shield is a combo of:
- A 16x2 LCD (shows 2 rows of 16 characters) that uses the LiquidCrystal library.
- 5 buttons (Up, Down, Left, Right, Select) all wired to one pin (A0).
- A Reset button to restart your Arduino Uno R4.
- A tiny knob (potentiometer) to tweak the screen’s contrast.
Pinout
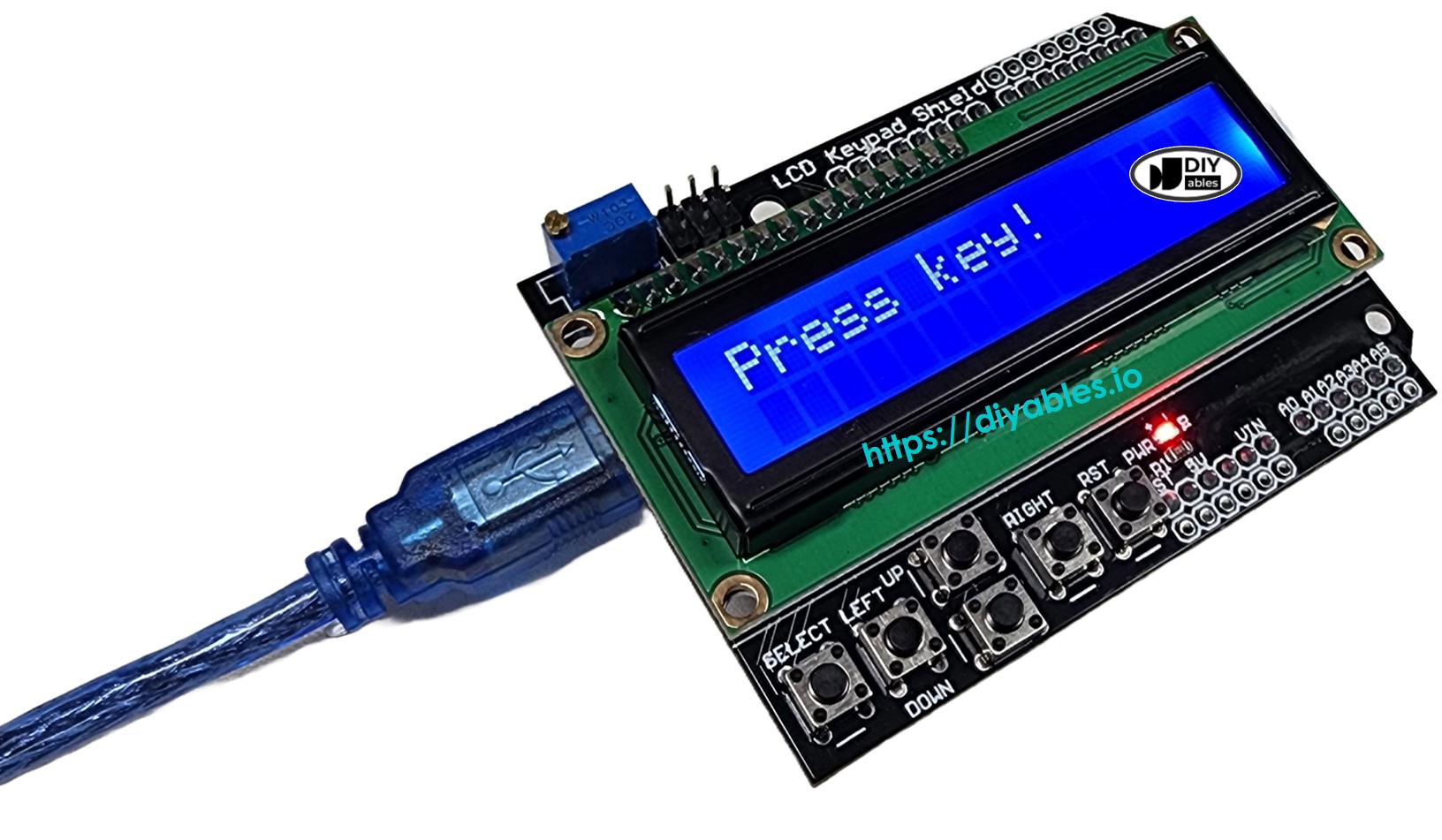
The following table shows how the LCD Keypad Shield connects to the Arduino Uno R4 when stacked on top of it.
Shield Pin | Function | Arduino Uno R4 Pin |
---|---|---|
DB4 | Data | 4 |
DB5 | Data | 5 |
DB6 | Data | 6 |
DB7 | Data | 7 |
RS | Register Select | 8 |
E | Enable | 9 |
Analog A0 | Button Input | A0 |
Reset Button: Press it, and your Arduino Uno R4 starts over.
Knob (Potentiometer): Twist it if the screen’s too faint or too dark.
Wiring Diagram
No complicated wiring here! Just stack the LCD Keypad Shield right onto your Arduino Uno R4. The pins match up on their own.
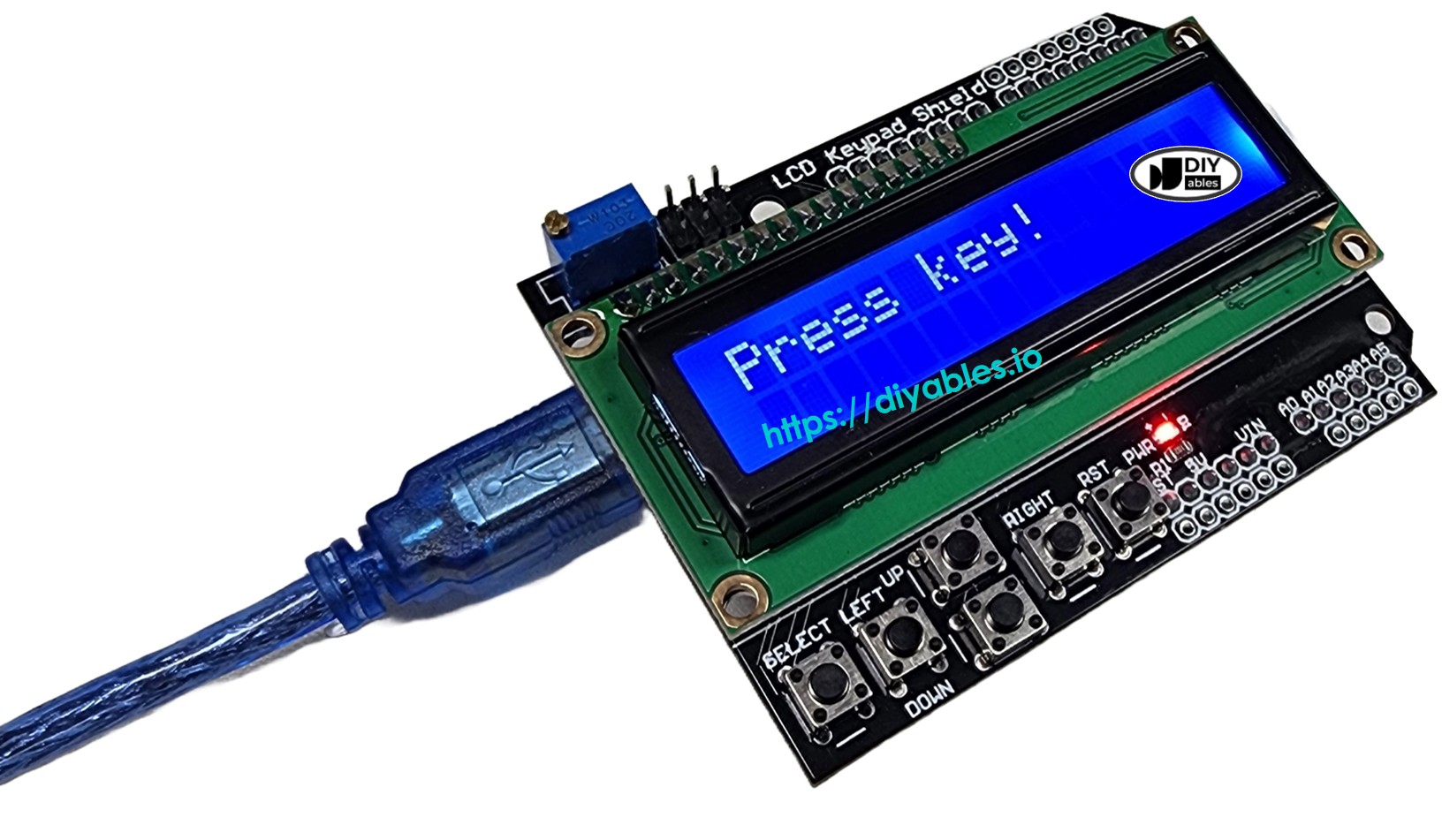
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
Arduino Uno R4 Code
Detailed Instructions
- Stack the LCD Keypad Shield on your Arduino Uno R4
- Plug the Arduino Uno R4 into your computer with the USB cable.
- Open Arduino IDE, pick your board and port in the IDE.
- Copy the code above, paste it in to the Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino Uno R4
- Press the shield’s buttons one by one.
- Watch the LCD show which one you pressed
If Blank Screen? Try This:
- Double-check the shield’s plugged in tight.
- Twist the knob to fix the contrast.
- Make sure the code’s right and the Arduino Uno R4’s powered up.
Bonus: Cleaner Code
Want your code to look pro? Here’s a fancier version with neat functions:
Extra Help
Need more LCD tricks? Check out this Arduino LiquidCrystal LCD tutorial for more cool ideas.
That’s it! You’re ready to play with your LCD Keypad Shield. Have fun building!