Arduino Nano ESP32 - Water Leak Email Notification
This guide will show you how to use Arduino Nano ESP32 to detect water leaks and immediately send email alerts. We will explain how to set up the sensor, provide code examples, and demonstrate how to link with email services. This is useful for keeping your home or office protected from water damage and is ideal for those who like DIY projects and want to enhance their water leak detection systems.
Or you can buy the following kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
We provide clear guides on Water Sensors and Gmail. Each guide includes detailed instructions and simple steps on setting up the hardware, understanding how it works, and connecting and programming the Arduino Nano ESP32. To find out more, please click these links.
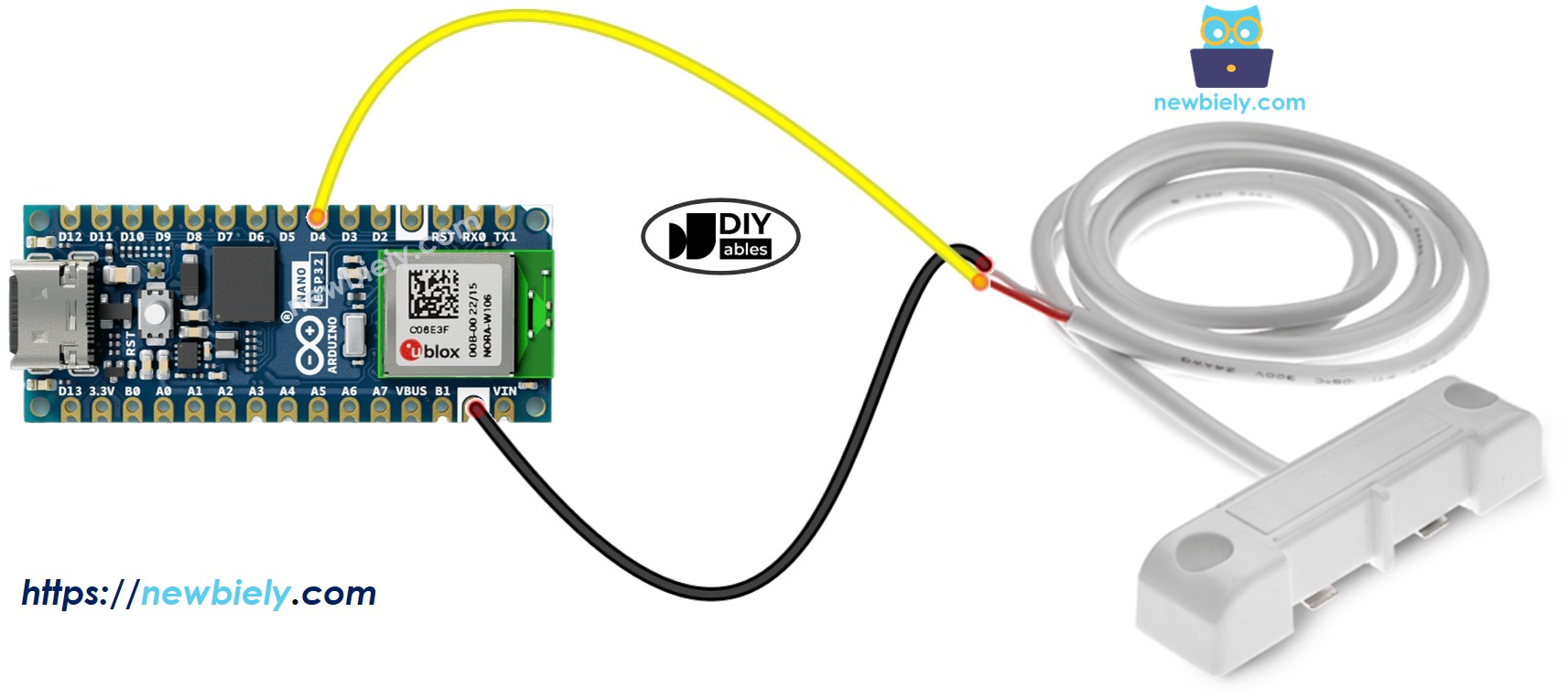
This image is created using Fritzing. Click to enlarge image
#include <WiFi.h>
#include <ESP_Mail_Client.h>
#define WIFI_SSID "YOUR_WIFI_SSID"
#define WIFI_PASSWORD "YOUR_WIFI_PASSWORD"
#define SENDER_EMAIL "xxxxxx@gmail.com"
#define SENDER_PASSWORD "xxxx xxxx xxxx xxxx"
#define RECIPIENT_EMAIL "xxxxxx@gmail.com"
#define SMTP_HOST "smtp.gmail.com"
#define SMTP_PORT 587
#define WATER_SENSOR_PIN D4
int water_state;
int prev_water_state;
SMTPSession smtp;
void setup() {
Serial.begin(9600);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
Serial.print("Connecting to Wi-Fi");
while (WiFi.status() != WL_CONNECTED) {
Serial.print(".");
delay(300);
}
Serial.println();
Serial.print("Connected with IP: ");
Serial.println(WiFi.localIP());
Serial.println();
pinMode(WATER_SENSOR_PIN, INPUT_PULLUP);
water_state = digitalRead(WATER_SENSOR_PIN);
}
void loop() {
prev_water_state = water_state;
water_state = digitalRead(WATER_SENSOR_PIN);
if (prev_water_state == HIGH && water_state == LOW) {
Serial.println("Water leakage is detected!");
String subject = "Email Notification from ESP32";
String textMsg = "This is an email sent from ESP32.\n";
textMsg += "Water leakage is detected";
gmail_send(subject, textMsg);
}
}
void gmail_send(String subject, String textMsg) {
MailClient.networkReconnect(true);
smtp.debug(1);
smtp.callback(smtpCallback);
Session_Config config;
config.server.host_name = SMTP_HOST;
config.server.port = SMTP_PORT;
config.login.email = SENDER_EMAIL;
config.login.password = SENDER_PASSWORD;
config.login.user_domain = F("127.0.0.1");
config.time.ntp_server = F("pool.ntp.org,time.nist.gov");
config.time.gmt_offset = 3;
config.time.day_light_offset = 0;
SMTP_Message message;
message.sender.name = F("ESP32");
message.sender.email = SENDER_EMAIL;
message.subject = subject;
message.addRecipient(F("To Whom It May Concern"), RECIPIENT_EMAIL);
message.text.content = textMsg;
message.text.transfer_encoding = "base64";
message.text.charSet = F("utf-8");
message.priority = esp_mail_smtp_priority::esp_mail_smtp_priority_low;
message.addHeader(F("Message-ID: <abcde.fghij@gmail.com>"));
if (!smtp.connect(&config)) {
Serial.printf("Connection error, Status Code: %d, Error Code: %d, Reason: %s\n", smtp.statusCode(), smtp.errorCode(), smtp.errorReason().c_str());
return;
}
if (!smtp.isLoggedIn()) {
Serial.println("Not yet logged in.");
} else {
if (smtp.isAuthenticated())
Serial.println("Successfully logged in.");
else
Serial.println("Connected with no Auth.");
}
if (!MailClient.sendMail(&smtp, &message))
Serial.printf("Error, Status Code: %d, Error Code: %d, Reason: %s\n", smtp.statusCode(), smtp.errorCode(), smtp.errorReason().c_str());
}
void smtpCallback(SMTP_Status status) {
Serial.println(status.info());
if (status.success()) {
Serial.println("----------------");
Serial.printf("Email sent success: %d\n", status.completedCount());
Serial.printf("Email sent failed: %d\n", status.failedCount());
Serial.println("----------------\n");
for (size_t i = 0; i < smtp.sendingResult.size(); i++) {
SMTP_Result result = smtp.sendingResult.getItem(i);
Serial.printf("Message No: %d\n", i + 1);
Serial.printf("Status: %s\n", result.completed ? "success" : "failed");
Serial.printf("Date/Time: %s\n", MailClient.Time.getDateTimeString(result.timestamp, "%B %d, %Y %H:%M:%S").c_str());
Serial.printf("Recipient: %s\n", result.recipients.c_str());
Serial.printf("Subject: %s\n", result.subject.c_str());
}
Serial.println("----------------\n");
smtp.sendingResult.clear();
}
}
To get started with Arduino Nano ESP32, follow these steps:
Connec the water sensor to the Arduino Nano ESP32 board according to the provided diagram.
Connect the Arduino Nano ESP32 board to your computer using a USB cable.
Launch the Arduino IDE on your computer.
Select the Arduino Nano ESP32 board and its corresponding COM port.
Open the Library Manager by clicking Library Manager icon on the left side of the Arduino IDE.
Search for ESP Mail Client and locate the ESP Mail Client by Mobizt.
Click on the Install button to get the ESP Mail Client library.
Copy the provided code and paste it into the Arduino IDE.
Change your WiFi credentials details by editing WIFI_SSID and WIFI_PASSWORD in the code.
Put your email and password in place of the sender's by updating SENDER_EMAIL and SENDER_PASSWORD.
Edit the recipient's email address in the code by changing RECIPIENT_EMAIL. It can be the same as the sender’s email.
※ NOTE THAT:
The sender's email must be a Gmail account.
Use the App password from the previous step as the sender’s password.
The recipient’s email can be any type of email.
Click the Upload button in Arduino IDE to send the code to Arduino Nano ESP32.
Open the Serial Monitor.
Pour water onto the water sensor.
Check the Serial Monitor to see the result.
Water leakage is detected
#### Email sent successfully
> C: Email sent successfully
----------------
Message sent success: 1
Message sent failed: 0
----------------
Message No: 1
Status: success
Date/Time: May 27, 2024 04:42:50
Recipient: xxxxxx@gmail.com
Subject: Email Notification from Arduino Nano ESP32
----------------