Arduino Nano ESP32 - Keypad 1x4
In this guide, we will learn how to use a 1x4 keypad with an Arduino Nano ESP32. We will cover the following details:
- How to connect a 1x4 keypad to Arduino Nano ESP32.
- How to program Arduino Nano ESP32 to detect which keys are pressed on a 1x4 keypad.
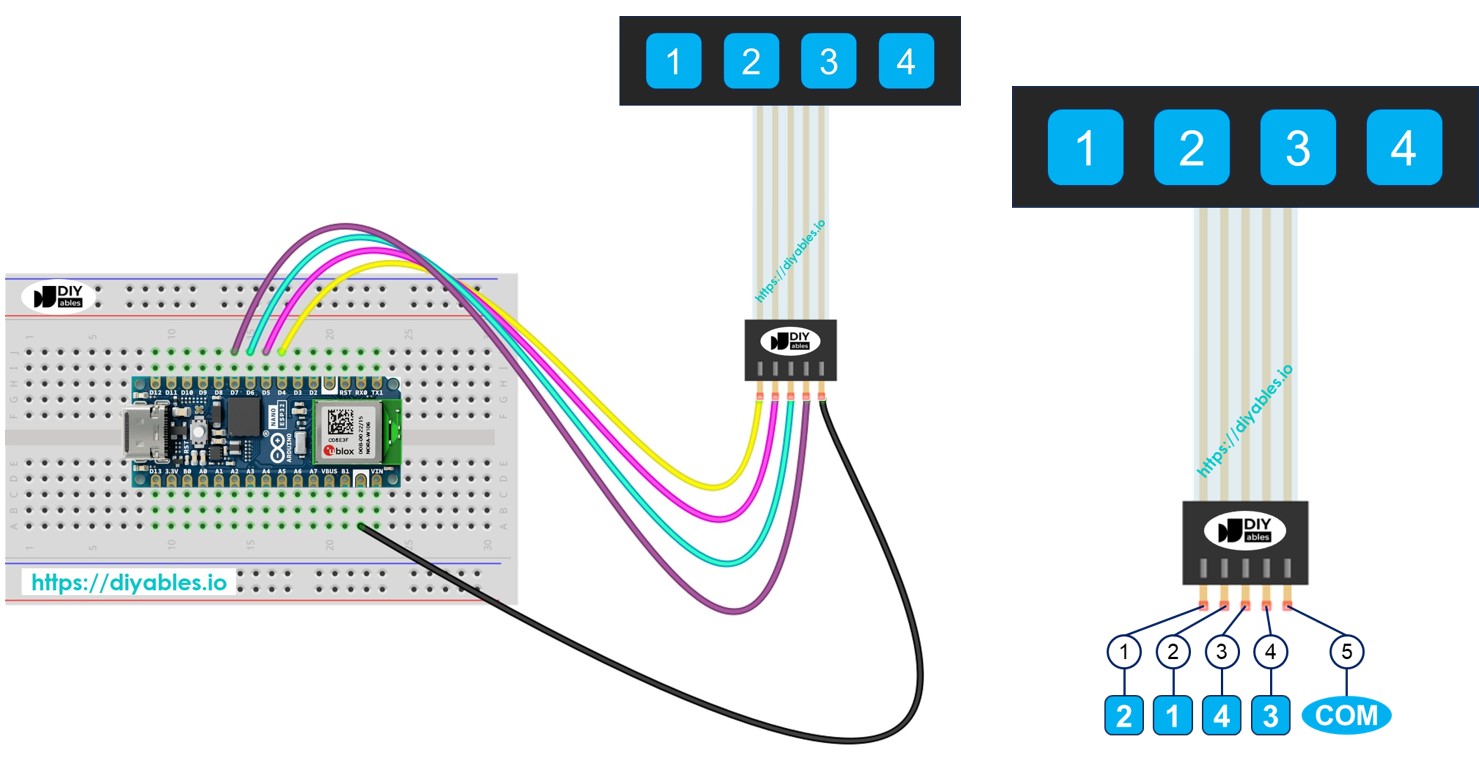
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Keypad 1x4
A 1x4 keypad has four buttons in a row. People use it to enter passwords, move through menus, or control devices.
Pinout
The 1x4 keypad has 5 pins. The arrangement of these pins does not match the order of the key labels.
- Pin 1 is connected to key 2
- Pin 2 is connected to key 1
- Pin 3 is connected to key 4
- Pin 4 is connected to key 3
- Pin 5 connects to all keys and is common
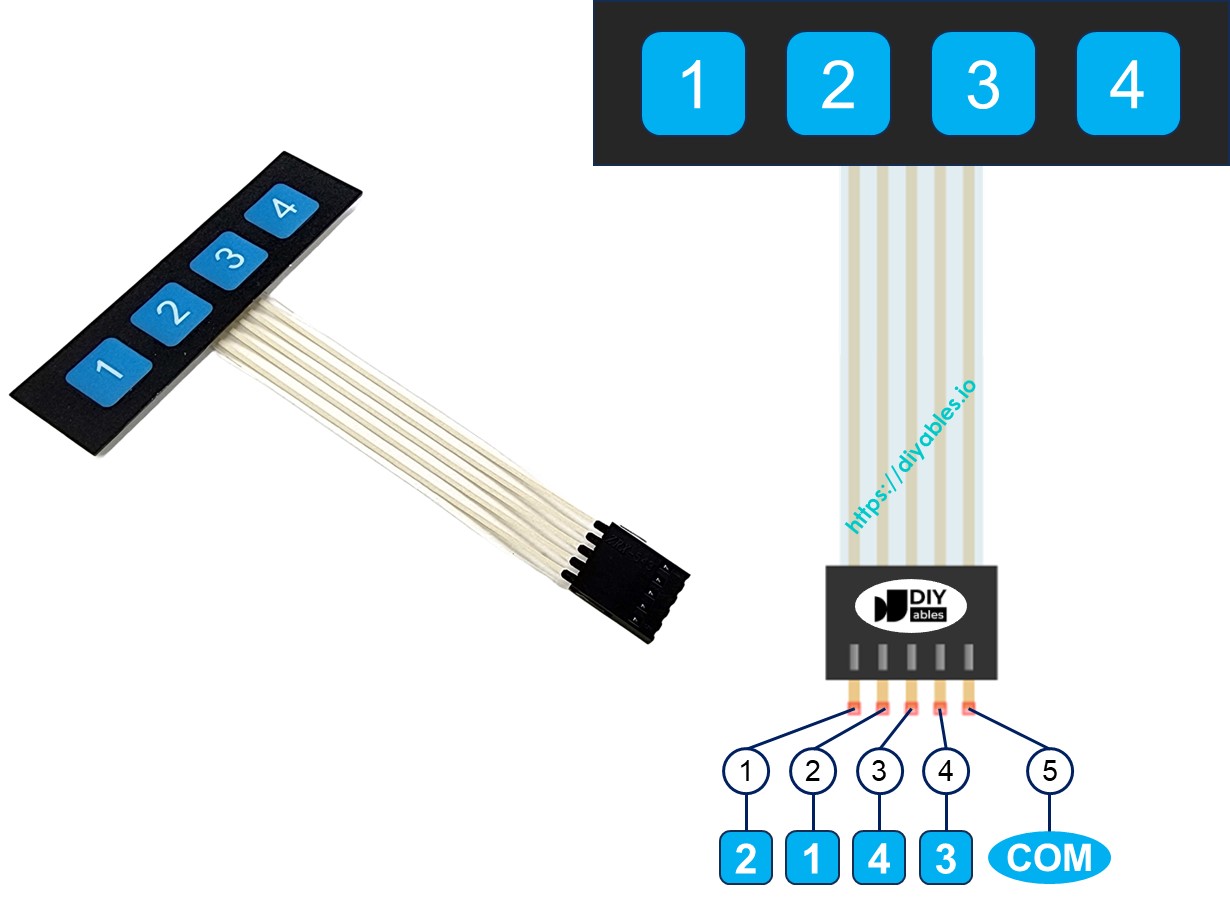
Wiring Diagram
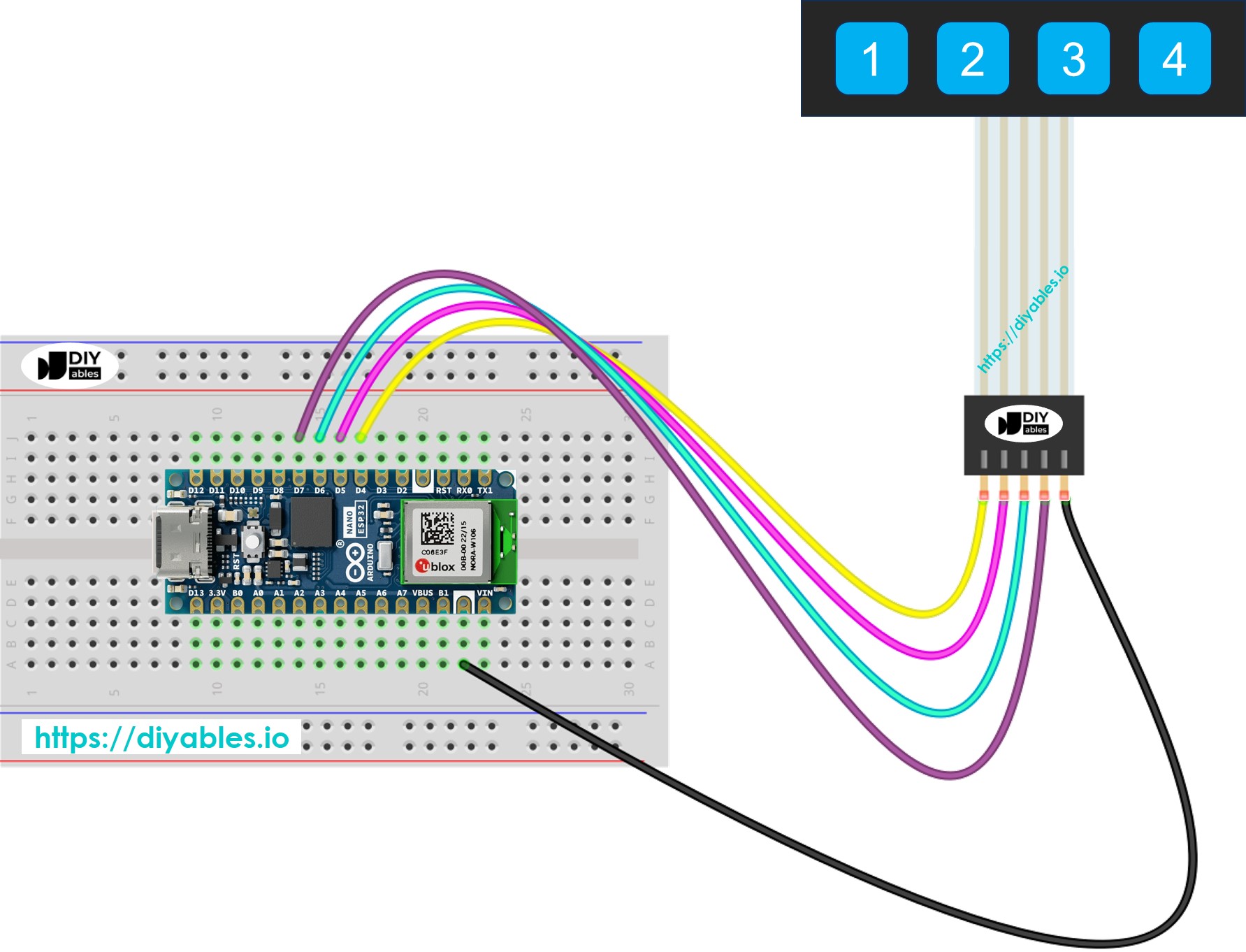
This image is created using Fritzing. Click to enlarge image
Arduino Nano ESP32 Code
Every key on the 1x4 keypad acts as a button, so we can use the digitalRead() function to check each key's status. Like other buttons, we need to address the bouncing issue, where one press might register as several presses. To prevent this, it's important to debounce each key. Debouncing four keys at once without interrupting other code parts can be tough. Luckily, the ezBbutton library makes this easier.
Detailed Instructions
To get started with Arduino Nano ESP32, follow these steps:
- If you are new to Arduino Nano ESP32, refer to the tutorial on how to set up the environment for Arduino Nano ESP32 in the Arduino IDE.
- Connect Arduino Nano ESP32 to the 1x4 keypad
- Connect the Arduino Nano ESP32 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano ESP32 board and its corresponding COM port.
- Click on the Libraries icon on the left side of the Arduino IDE.
- Search for ezButton and locate the button library from Arduino Nano ESP32GetStarted.com
- Click the Install button to add the ezButton library.
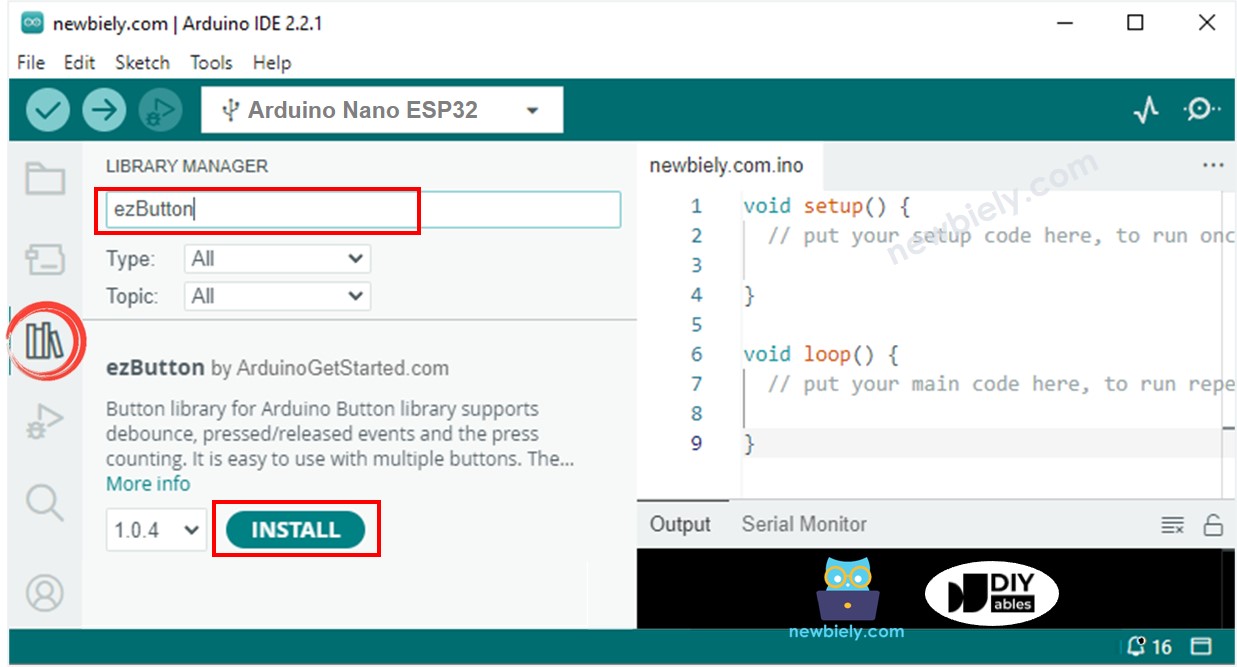
- Copy the code and open it in Arduino IDE
- Click the Upload button in Arduino IDE to upload the code to your Arduino Nano ESP32
- Open the Serial Monitor
- Press each key on the 1x4 keypad one at a time
- Check the results on the Serial Monitor