Arduino Nano - Button - Debounce
When a button is pressed or released, or a switch is toggled, newbies often assume that its state is changed from LOW to HIGH or HIGH to LOW. In reality, this is not always the case. Due to mechanical and physical characteristics, the state of the button (or switch) may be quickly toggled between LOW and HIGH multiple times in response to a single event. This phenomenon is known as chattering. Chattering can cause a single press to be read as multiple presses, resulting in malfunction in certain applications.
The method to eliminate this problem is referred as debouncing or debounce. This tutorial instructs you how to do it when using the button with Arduino Nano. We will learn though the below steps:
Arduino Nano code without debouncing a button.
Arduino Nano code with debouncing a button.
Arduino Nano code with debouncing a button by using the ezButton library.
Arduino Nano code with debouncing for multiple buttons.
Or you can buy the following sensor kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
If you are not familiar with buttons (pinout, functionality, programming, etc.), the following tutorials can help:
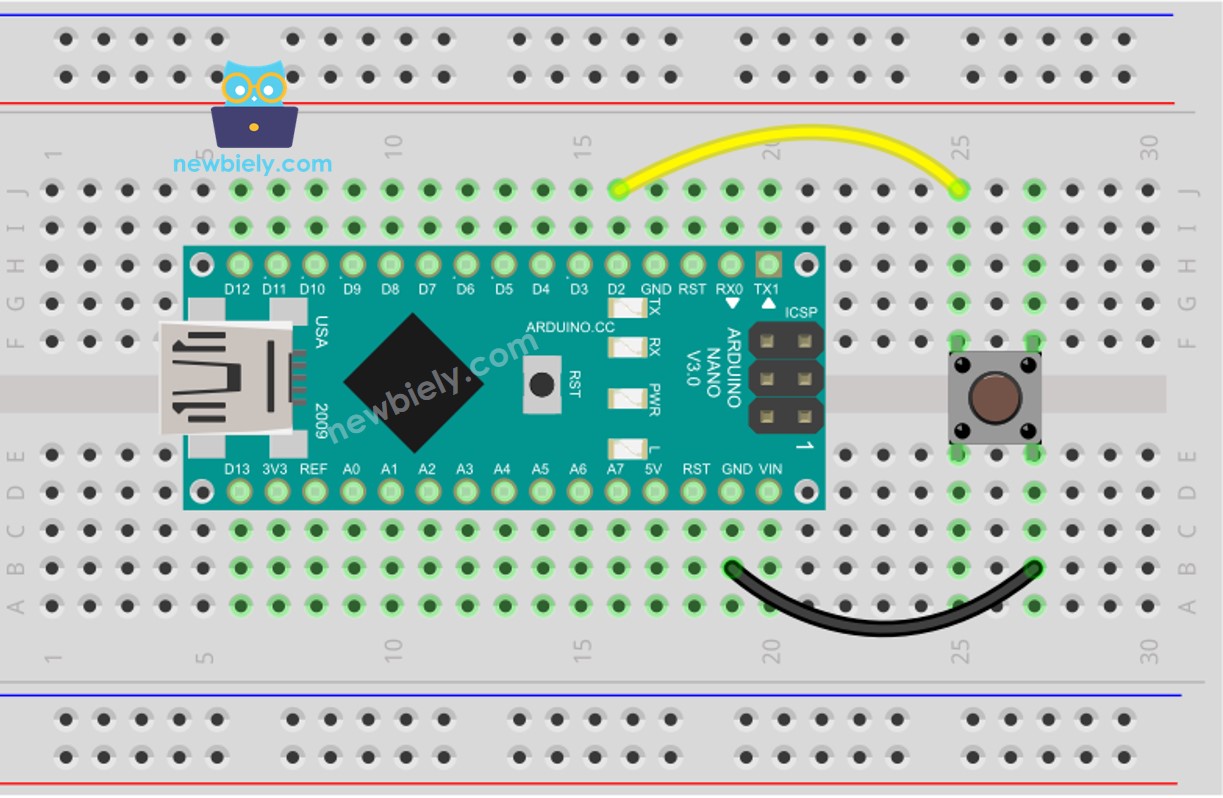
This image is created using Fritzing. Click to enlarge image
Let us observe and contrast the Arduino Nano code for both WITH and WITHOUT debounce, as well as their respective behaviors.
Before delving into the concept of debouncing, take a look at the code without debouncing and observe its behavior.
Attach an USB cable to the Arduino Nano and your PC.
Launch the Arduino IDE, choose the correct board and port.
Copy the code below and open it in the Arduino IDE.
#define BUTTON_PIN 2
int prev_button_state = LOW;
int button_state;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
button_state = digitalRead(BUTTON_PIN);
if(prev_button_state == HIGH && button_state == LOW)
Serial.println("The button is pressed");
else if(prev_button_state == LOW && button_state == HIGH)
Serial.println("The button is released");
prev_button_state = button_state;
}
Open the Serial Monitor.
Press and hold the button for a few seconds, then release it.
Try several times.
Check out the result in the Serial Monitor.
The button is pressed
The button is pressed
The button is pressed
The button is released
The button is released
Sometime, you only pressed and released the button once. Nevertheless, Arduino Nano interprets it as multiple presses and releases. This is the chattering phenomenon mentioned at the beginning of the tutorial. Let's see how to fix it in the next part.
The below code applies the method called debounce to prevent the chattering phenomenon.
#define BUTTON_PIN 2
const int DEBOUNCE_DELAY = 50;
int lastSteadyState = LOW;
int lastFlickerableState = LOW;
int button_state;
unsigned long lastDebounceTime = 0;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
button_state = digitalRead(BUTTON_PIN);
if (button_state != lastFlickerableState) {
lastDebounceTime = millis();
lastFlickerableState = button_state;
}
if ((millis() - lastDebounceTime) > DEBOUNCE_DELAY) {
if (lastSteadyState == HIGH && button_state == LOW)
Serial.println("The button is pressed");
else if (lastSteadyState == LOW && button_state == HIGH)
Serial.println("The button is released");
lastSteadyState = button_state;
}
}
Copy the code above and open it with the Arduino IDE.
Click the Upload button on the Arduino IDE to compile and upload the code to the Arduino Nano.
Open the Serial Monitor.
Continue to press the button for a few seconds, then release it.
Check out the result on the Serial Monitor.
The button is pressed
The button is released
As you can observe, you pushed and released the button only once. Arduino Nano detects it as a single push and release. The noise is eliminated.
We have designed a library, ezButton, to make it simpler for those who are just starting out, particularly when dealing with multiple buttons. You can find out more about the ezButton library here.
#include <ezButton.h>
ezButton button(2);
void setup() {
Serial.begin(9600);
button.setDebounceTime(50);
}
void loop() {
button.loop();
if(button.isPressed())
Serial.println("The button is pressed");
if(button.isReleased())
Serial.println("The button is released");
}
#include <ezButton.h>
ezButton button1(6);
ezButton button2(7);
ezButton button3(8);
void setup() {
Serial.begin(9600);
button1.setDebounceTime(50);
button2.setDebounceTime(50);
button3.setDebounceTime(50);
}
void loop() {
button1.loop();
button2.loop();
button3.loop();
if(button1.isPressed())
Serial.println("The button 1 is pressed");
if(button1.isReleased())
Serial.println("The button 1 is released");
if(button2.isPressed())
Serial.println("The button 2 is pressed");
if(button2.isReleased())
Serial.println("The button 2 is released");
if(button3.isPressed())
Serial.println("The button 3 is pressed");
if(button3.isReleased())
Serial.println("The button 3 is released");
}
The schematic for the code above: The illustration of the wiring for the code:. The visual representation of the wiring for the code:
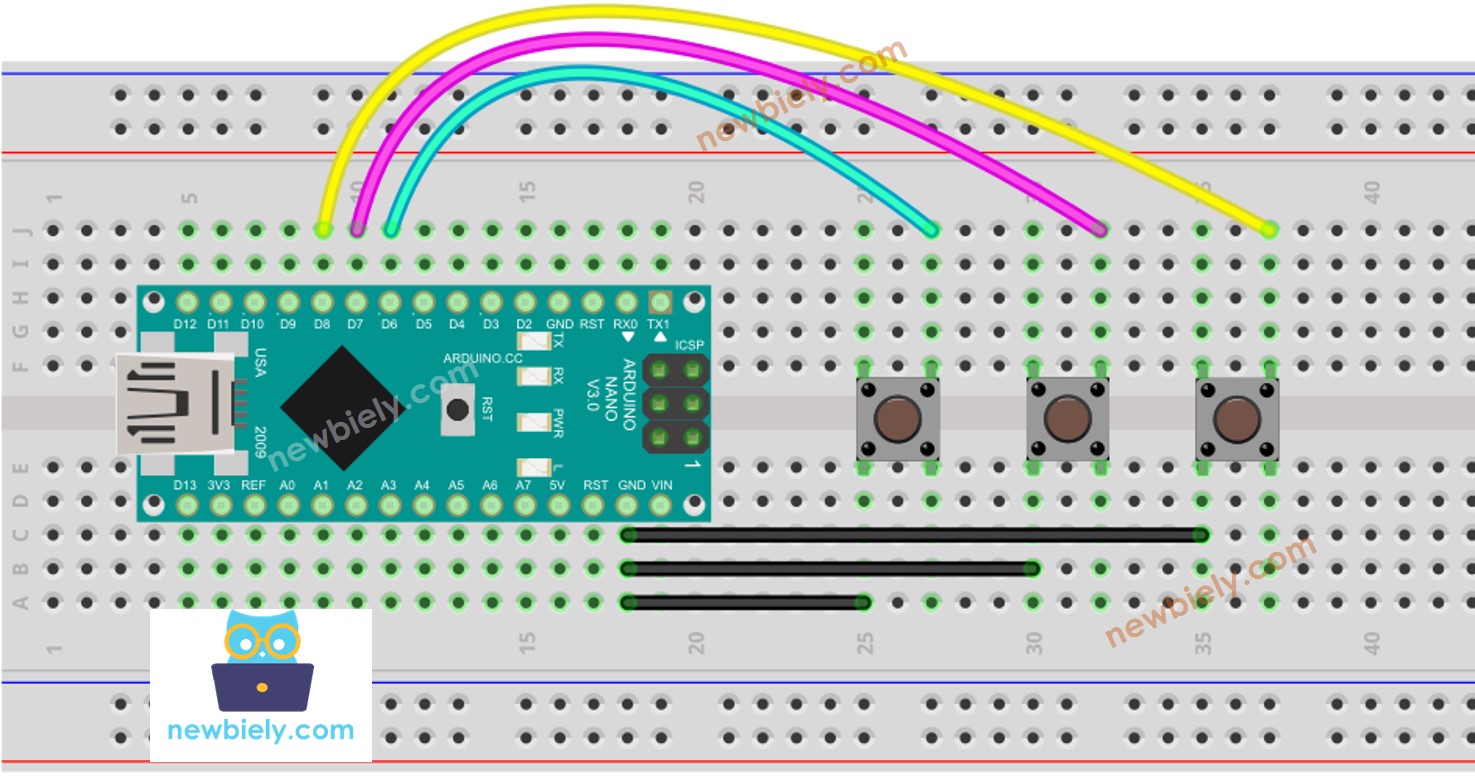
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
The debounce technique can be utilized with a switch, touch sensor, and more.