Arduino Nano - BLE
This tutorial instructs you how to use Arduino Nano to control BLE HM-10 Module. In detail, we will learn:
- The utilization of Bluetooth Low Energy (BLE) technology with Arduino Nano
- Sending data from Arduino Nano to a smartphone app via BLE
- Receiving data on Arduino Nano from a smartphone app through BLE
- Controlling Arduino Nano from a smartphone app with BLE.
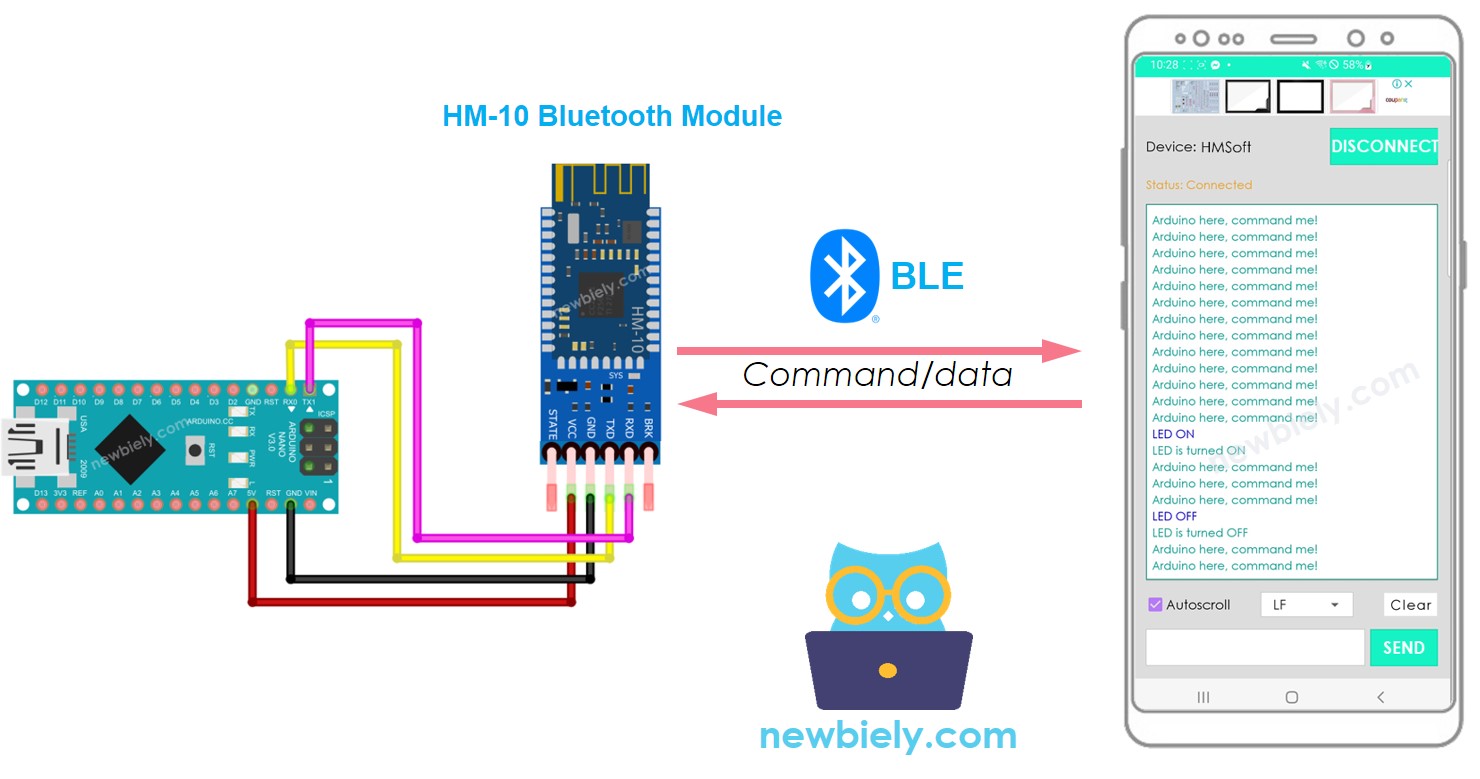
The purpose of this tutorial is to:
- Exchange data between an Arduino Nano and a smartphone app via BLE.
- Utilize the HM-10 Bluetooth Low Energy module.
- Showcase the Bluetooth Serial Monitor app on Android, but any Android/iOS app can be used.
- Manipulate various devices connected to Arduino Nano through the smartphone app, with examples such as an LED and servo motor.
It is important to take into account that this tutorial is focused on Bluetooth Low Energy (BLE, Bluetooth 4.0). If you need information regarding Classic Bluetooth (Bluetooth 2.0), please refer to the similar tutorial Arduino Nano - Bluetooth.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of HM-10 Bluetooth Module
The HM-10 is a Serial BLE module which acts as a Serial to Bluetooth Low Energy converter. It has the capability to:
- Receive data from the Serial RX pin and transmit it to a paired device like a smartphone over BLE
- Receive data from BLE (from the paired device) and send it to the Serial TX pin.
When an Arduino Nano is used to communicate with a smartphone app (Android/iOS), the following steps take place:
- The Arduino Nano is connected to the HM-10 Bluetooth module via the Serial/SoftwareSerial pins
- The HM-10 Bluetooth module is paired with the smartphone app
- Data is sent from the Arduino Nano to the smartphone app by sending it to the Serial/SoftwareSerial
- Data is received from the smartphone app by the Arduino Nano by reading it from the Serial/SoftwareSerial
- No extra BLE code is necessary on the Arduino Nano.
The BLE HM-10 Module Pinout
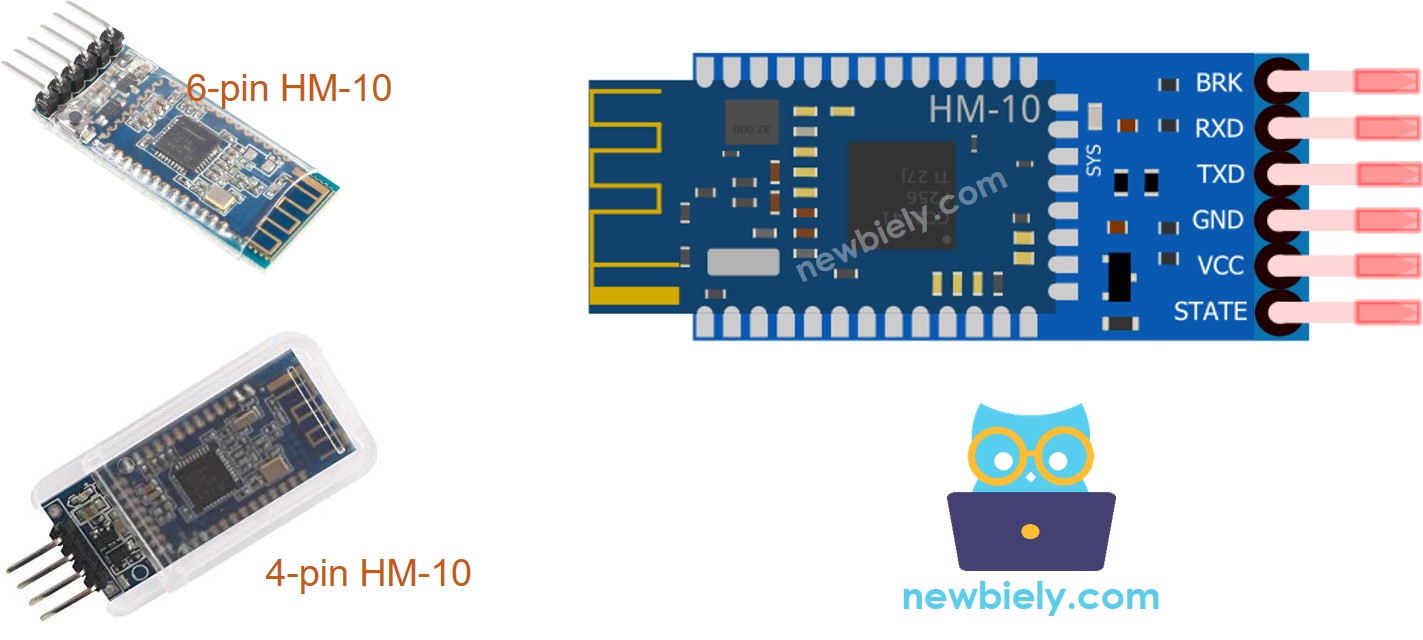
The HM-10 BLE Module has 6 pins:
- BKR pin: This pin is used to control the behavior of the module. Beginners should ignore this pin.
- RX pin: This is a serial data pin, which should be connected to the TX pin of Arduino Nano. Data received from this pin will be sent to Bluetooth.
- TX pin: This is a serial data pin, which should be connected to the RX pin of Arduino Nano. Data received via BLE will be sent to this pin as serial data.
- GND pin: This is a power pin, which should be connected to the GND of the power source.
- VCC pin: This is a power pin, which should be connected to 3.3V of Supply voltage.
- STATE pin: This pin indicates the working states:
- Blink in standby mode - repeat 500ms pulse;
- On in connection state - high level.
- Only four pins are required for the HM-10, which are VCC, GND, RX, and TX.
- Some producers make the HM-10 with only these four pins.
※ NOTE THAT:
Overview of Bluetooth Serial Monitor App
To use the Bluetooth Serial Monitor App, you must first connect your Arduino Nano board to an HM-10 Bluetooth module. Then, download and install the app on your smartphone. Finally, open the app and establish a connection with the HM-10 Bluetooth module.
Once the steps are finished, you will be able to transmit and receive data from the Arduino Nano as if you were using the Serial Monitor in the Arduino IDE, without having to make any changes to the existing Arduino Nano code or adding any Bluetooth-specific code.
Wiring Diagram
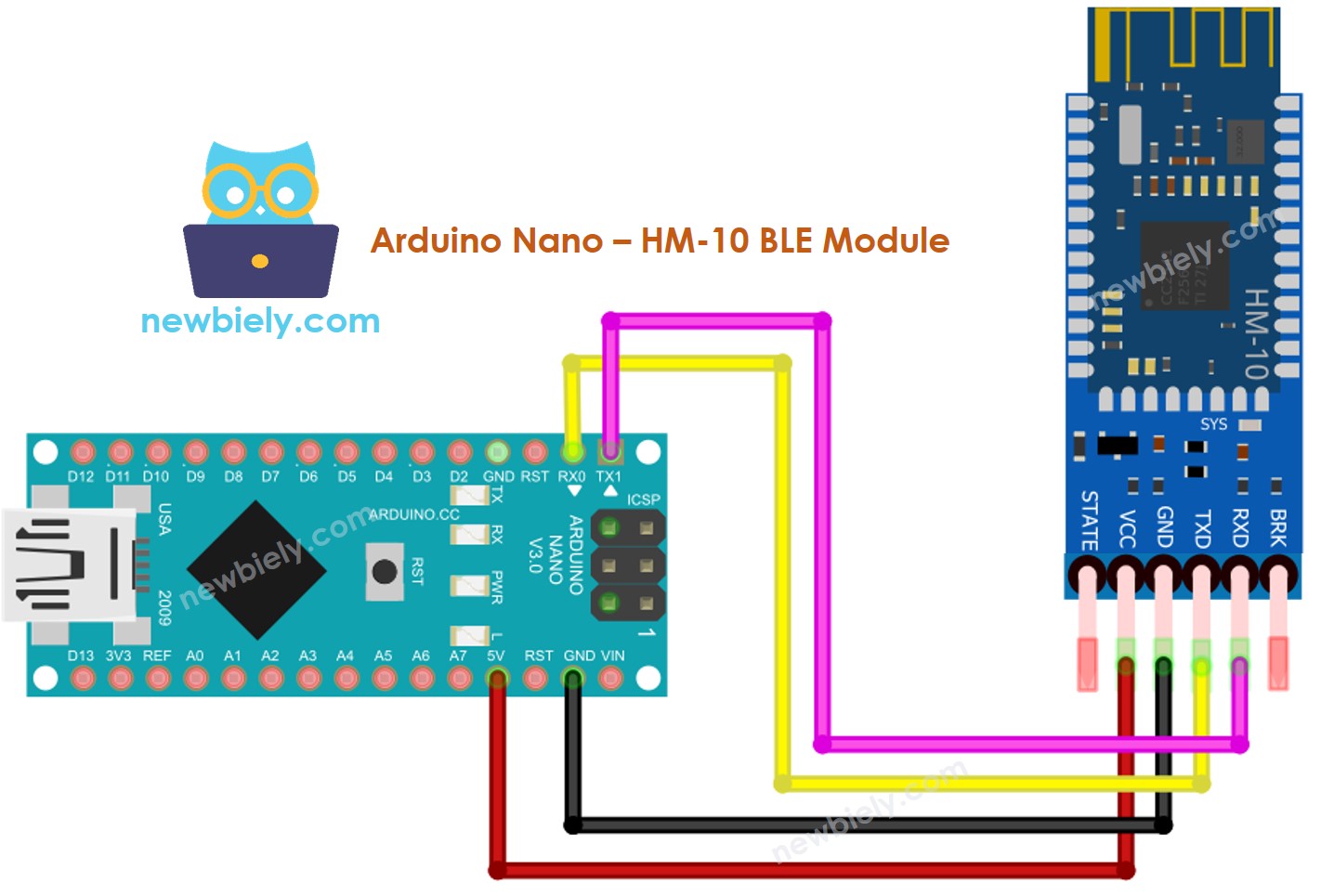
This image is created using Fritzing. Click to enlarge image
The Table of Wiring. A Table for Wiring
Arduino Nano Pins | HM-10 Bluetooth Pins |
---|---|
RX (Pin 0) | TX |
TX (Pin 1) | RX |
5V | VCC |
GND | GND |
BKR (NOT connected) | |
STATE (NOT connected) |
※ NOTE THAT:
The Arduino Nano code can utilize other pins by substituting the Serial object with an alternative like Serial1, Serial2, or SoftwareSerial (if available).
How To Program For Bluetooth
No specific Bluetooth programming is required, only Serial programming is necessary.
Arduino Nano sends data to Bluetooth App on Smartphone
To send data from an Arduino Nano board to a Bluetooth application on a smartphone, the following Arduino Nano code can be utilized. This example shows transmitting the message “Arduino here, command me!” from the Arduino Nano to the smartphone app every one second.
Detailed Instructions
- Scan for available devices and select the HM-10 module.
To use the code and establish a connection between an Arduino Nano board and a smartphone using BLE, follow these steps:
- Download and install the Bluetooth Serial Monitor App on your smartphone.
- As per the wiring diagram, connect the HM-10 Bluetooth module to the Arduino Nano board.
- Open the Arduino IDE, copy the code provided, and upload it to the Arduino Nano board. If you experience difficulty uploading the code, disconnect the TX and RX pins from the Bluetooth module, upload the code, and then reconnect the RX/TX pins.
- Launch the Serial Monitor on the Arduino IDE.
- On your smartphone, open the Bluetooth Serial Monitor App and switch to the BLE mode.
- Look for available devices and select the HM-10 module.
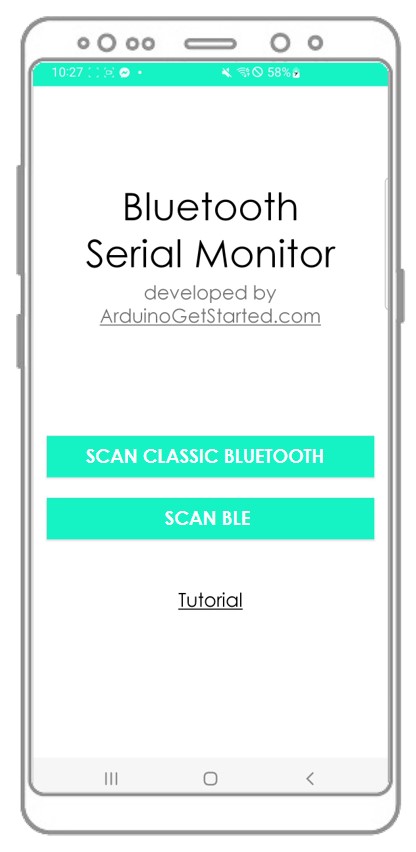
- Connect the smartphone to the HM-10 Bluetooth module.
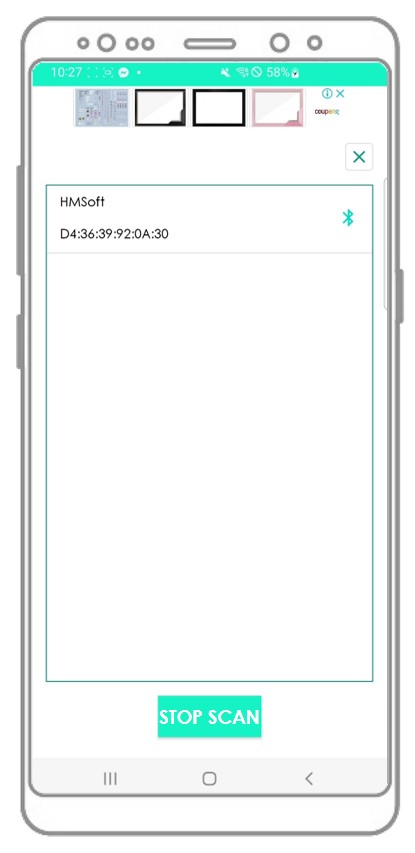
- Take a look at the outcome on the Android App.
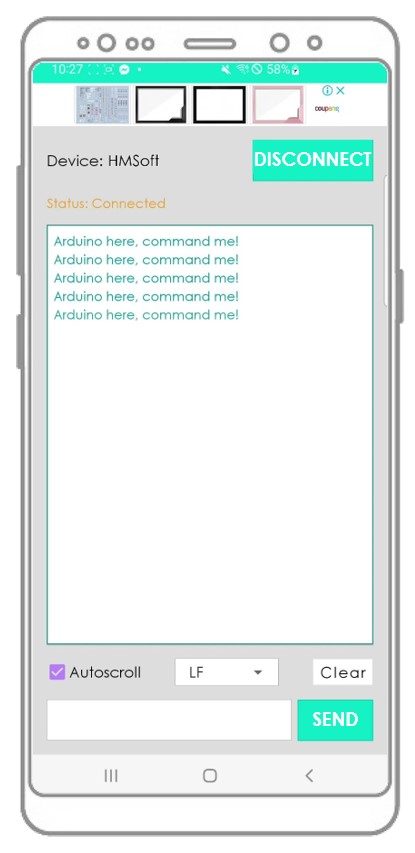
- Take a look at the output displayed in the Serial Monitor of the Arduino IDE.
If you take the necessary steps and execute the code, you will see that the data shown on the Serial Monitor of the Arduino IDE and on the Android application will be the same.
Bluetooth App Send data To Arduino Nano
The code below carries out the following tasks:
- Sending data from the Bluetooth app to the Arduino Nano board
- The Arduino Nano board reading the incoming data and then sending a response back to the Bluetooth device.
Detailed Instructions
Here are the steps to use the code with Arduino Nano and an Android app:
- Launch the Arduino IDE and copy the code provided.
- Click the Upload button to transfer the code to the Arduino Nano board.
- Open the Serial Monitor from the Arduino IDE.
- Start the Android App and pair it with the HM-10 Bluetooth module following the instructions given in a previous example.
- After connecting, type "LED ON" or "LED OFF" in the Android app and press the "SEND" button.
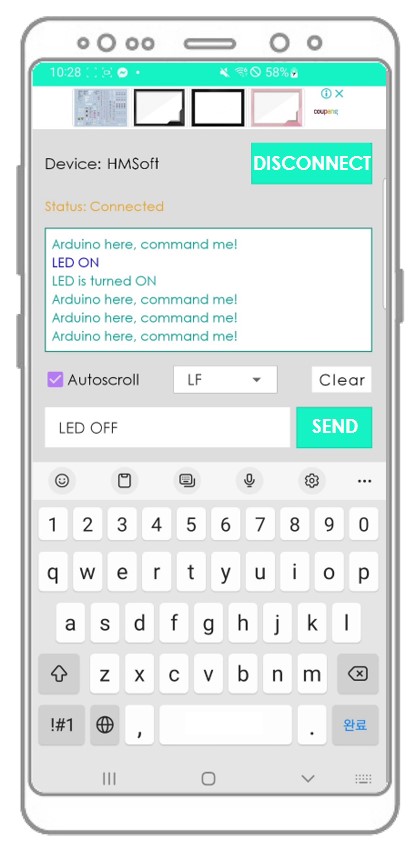
- The Arduino Nano board will receive the data and print a response to the Serial port. Afterwards, this data will be transmitted to the Bluetooth app. Lastly, the result can be viewed on the Android app.
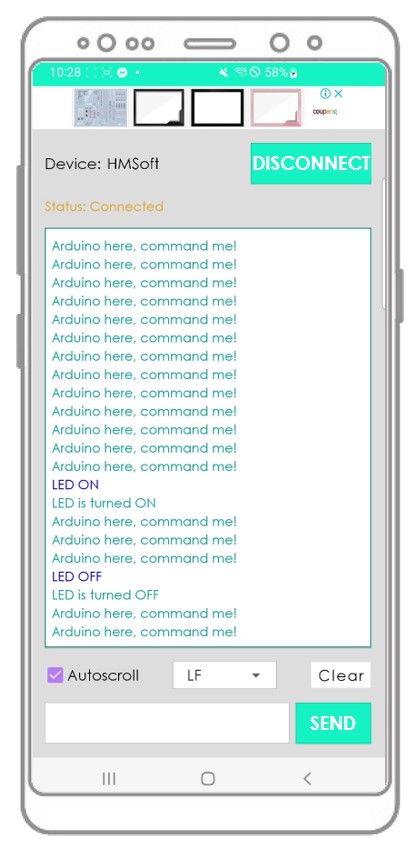
- Examine the output displayed on the Serial Monitor of the Arduino IDE.
Once you have finished the steps above,. you will observe that the data displayed on the Serial Monitor of the Arduino IDE and the Android app match.
Arduino Nano Code - Control LED with smartphone App via BLE
The following Arduino Nano example code makes use of commands “ON” and “OFF” obtained through the Bluetooth Serial Monitor App to switch on/off an integrated LED.
You can find further information on the instructions in the tutorial titled Arduino Nano controls LED via Bluetooth/BLE. For a more detailed look at the instructions, refer to the Arduino Nano controls LED via Bluetooth/BLE tutorial.
Arduino Nano Code - Control Servo Motor with smartphone App via BLE
- The Arduino Nano code below is used to receive an angle value from the Bluetooth Serial Monitor App. 2. This angle value is then used to control the angle of a servo motor.
You can get a more detailed look at the instructions in the Arduino Nano controls Servo Motor via Bluetooth/BLE tutorial. For a closer look at the instructions, please check out the Arduino Nano controls Servo Motor via Bluetooth/BLE tutorial. If you would like to have a better understanding of the instructions, please refer to the Arduino Nano controls Servo Motor via Bluetooth/BLE tutorial.
If you find the Bluetooth Serial Monitor app helpful, please rate it 5 stars on Play Store. Your appreciation is much appreciated!