Arduino Nano - Serial Monitor
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Serial Monitor
Serial Monitor is one of the tools in Arduino IDE which is used for two distinct functions:
- From Arduino Nano to PC: Data is received from Arduino Nano and displayed on the screen. This is usually done for debugging and monitoring.
- From PC to Arduino: Commands are sent from PC to Arduino Nano.
To use Serial Monitor, we must connect Arduino Nano and PC via a USB cable. This same cable is also used to upload code to the Arduino Nano. Data is exchanged between Serial Monitor and Arduino Nano through this USB cable.
How To Use Serial Monitor
Open Serial Monitor
Click the Serial Monitor icon on the right side of Arduino IDE.
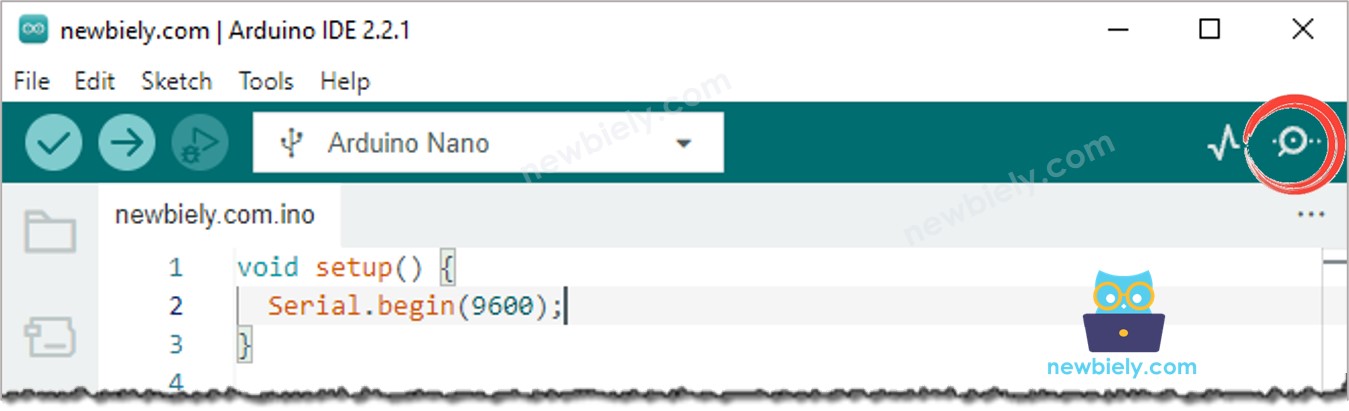
Items on Serial Monitor
Print to the console: show information that has been received from the Arduino Nano.
Checkbox for Autoscroll: Option to choose between automatic scrolling and not scrolling.
*Checkbox to enable display of timestamp before data shown on Serial Monitor.
*ii. Clear Output Button: Erase all text from the output console.
*. 1. Selection of baud rate: Choose the communication speed (baud rate) between Arduino Nano and PC. 2. This value must match the one used in the Arduino Nano code (in the Serial.begin() function).
※ NOTE THAT:
When we choose a baud rate, even if the value remains unchanged, Arduino Nano is reset. Consequently, this is one method of resetting Arduino Nano.
*. Box for entering text:. A user can type characters into it to be sent to an Arduino Nano.
- Ending selection:
- Choose the characters to be added to the data sent to Arduino:
- No line ending: no additional characters
- Newline: add a newline (LF, or '\n') character
- Carriage return: add a carriage return (CR, or '\r') character
- Both NL and CR: add both newline and carriage return (CRLF, or '\r\n') characters
*. 1. Pressing the Send button:. 2. This will cause the Serial Monitor to transmit the data in the textbox, along with the terminating characters, to the Arduino Nano.
Arduino Nano To PC
Set the baud rate and initiate the Serial port using the Serial.begin() function to transmit data from Arduino Nano to PC.
- Using Serial.println():
- Send data to the Serial Monitor utilizing one of these functions: Serial.print(), Serial.println(), Serial.write().
- For instance, to send “Hello World!” to the Serial Monitor, use Serial.println():
Example Use
In this example, we will transmit “ArduinoGetStarted.com” from Arduino Nano to the Serial Monitor at an interval of one second.
Detailed Instructions
- Copy the code and open it with the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to the Arduino Nano.
- Open the Serial Monitor.
- Set the baurate to 9600.
- Check the output on the Serial Monitor.
- Attempt to substitute the Serial.println() function with Serial.print() function.
PC To Arduino Nano
How to send data from PC to Aduino and read it on Arduino Nano
Type your text in the Serial Monitor and then press the Send button.
Set the baud rate and initiate the Serial port by using the following Arduino Nano code:
- Read data from Serial port
Retrieve data from the Serial port by using the following Arduino Nano code:
- Process data
Process the data with the help of the following Arduino Nano code:
- Determine if data is present or not.
- Utilize one of the following functions to read data from Serial port: Serial.read(), Serial.readBytes(), Serial.readBytesUntil(), Serial.readString(), Serial.readStringUntil(). As an example:
Example Use
In this example, we will be sending commands from Serial Monitor to Arduino Nano. The commands are as follows:
- “ON”: the LED will be switched on
- “OFF”: the LED will be switched off
These commands will be used to control a built-in LED.
How can Arduino Nano recognize a full command? For example, when we send “OFF”, how can Arduino Nano differentiate between “O”, “OF”, and “OFF”?
When sending a command, we will append a newline character ('\n') by selecting the “newline” option on the Serial Monitor. Arduino Nano will read data until it encounters the newline character. In this case, the newline character is referred to as a delimiter.
Detailed Instructions
- Copy the code and open it with Arduino IDE.
- Click the Upload button on Arduino IDE to upload the code to Arduino Nano.
- Open the Serial Monitor.
- Choose a baurate of 9600 and select the newline option.
- Type “ON” or “OFF” and then press the Send button.
- Check the built-in LED on the Arduino Nano board. It will be either ON or OFF.
- Additionally, we can observe the LED's state on the Serial Monitor.
- Type the commands “ON” or “OFF” a few times.