Arduino Nano - Flame Sensor
The flame sensor is a nifty device that picks up on infrared emissions from a flame, making it a useful tool for fire detection. Sometimes referred to as an infrared flame sensor or fire sensor, it outputs signals in two flavors: digital (LOW/HIGH) and analog.
In this guide, we'll walk through the process of utilizing an Arduino Nano alongside a flame sensor to detect and gauge the intensity of flames. We'll cover the basics, including:
- How to hook up the flame sensor to your Arduino Nano.
- How to program the Arduino Nano to spot flames and fires by interpreting the digital signals from the flame sensor.
- How to program the Arduino Nano to assess the flame's intensity by reading the analog signals from the flame sensor.
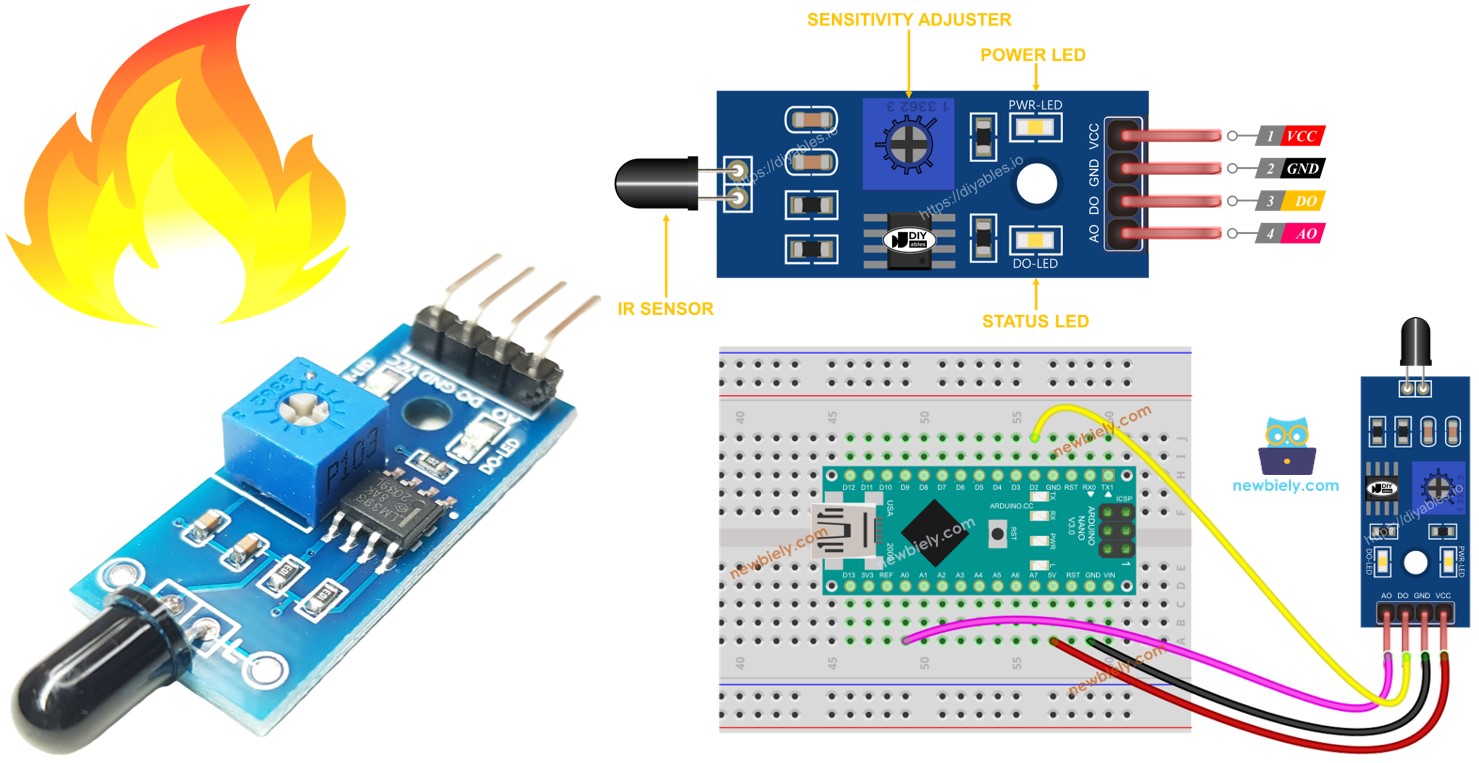
Afterward, you can modify the code to activate a warning horn (via a relay) when it detects fire.
Hardware Preparation
1 | × | Arduino Nano | |
1 | × | USB A to Mini-B USB cable | |
1 | × | Flame Sensor | |
1 | × | Jumper Wires | |
1 | × | (Optional) 9V Power Adapter for Arduino Nano | |
1 | × | (Recommended) Screw Terminal Adapter for Arduino Nano |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of Flame Sensor
The infrared flame sensor serves as a handy tool for identifying the presence of a flame or gauging the emitted infrared level from the flame. As a result, it becomes a reliable detector for fires. The flame sensor offers dual options through a digital output pin and an analog output pin.
These sensors are carefully crafted to selectively hone in on specific wavelengths of infrared radiation associated with flames. This design minimizes the chances of false alarms triggered by other sources of infrared radiation like human body heat or artificial lighting. However, it's crucial to note that, like any sensor, they do have their limitations, and in certain conditions, there might be instances of false positives or false negatives.
Pinout
The flame sensor includes four pins:
- VCC pin: It needs to be connected to VCC (3.3V to 5V).
- GND pin: It needs to be connected to GND (0V).
- DO pin: It is a digital output pin. It is HIGH if the flame is not detected and LOW if detected. The threshold value for flame detection can be adjusted using a built-in potentiometer.
- AO pin: It is an analog output pin. The output value decreases as the infraed level is decreased, and it increases as infraed level is increased.
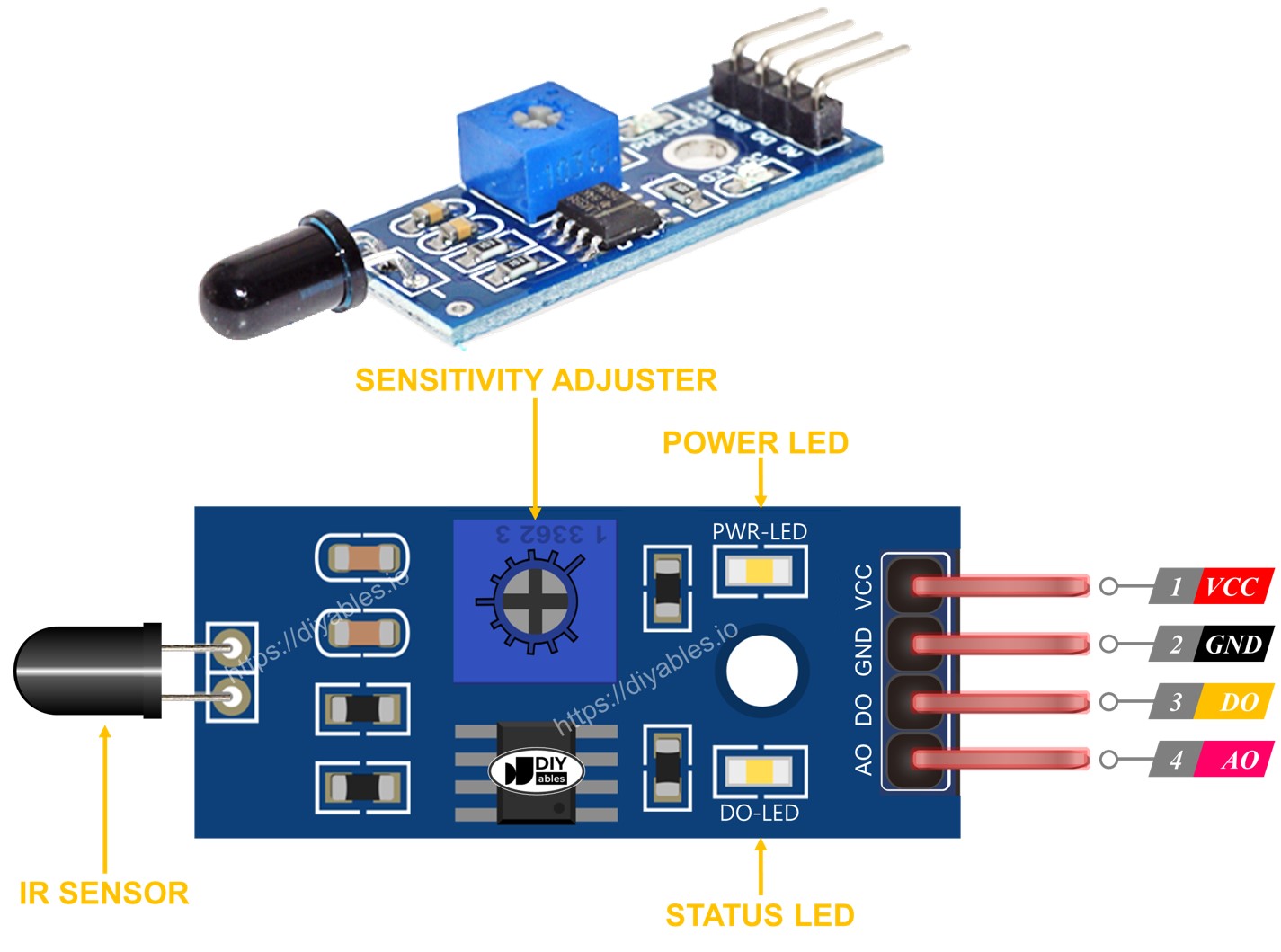
Furthermore, it has two LED indicators:
- One PWR-LED indicator for power.
- One DO-LED indicator for the flame state on the DO pin: it is on when flame is present.
How It Works
Regarding the DO pin:
- The module includes a built-in dial to adjust the infrared sensitivity.
- If the infrared strength surpasses the set threshold, indicating a flame detection, the sensor's output pin goes low, turning on the DO-LED.
- Conversely, if the infrared intensity falls below the threshold, signaling no flame detection, the output pin goes high, turning off the DO-LED.
For the AO pin:
- The AO pin registers higher values with increased infrared intensity in the surrounding area.
- Conversely, lower infrared intensity in the surroundings results in lower values being read from the AO pin.
It's important to note that the dial setting doesn't impact the readings on the AO pin.
Wiring Diagram
Since the flame sensor module has two outputs, you can choose to use one or both of them, depending on what you need.
- The wiring diagram between Arduino Nano and the flame sensor when using DO only.
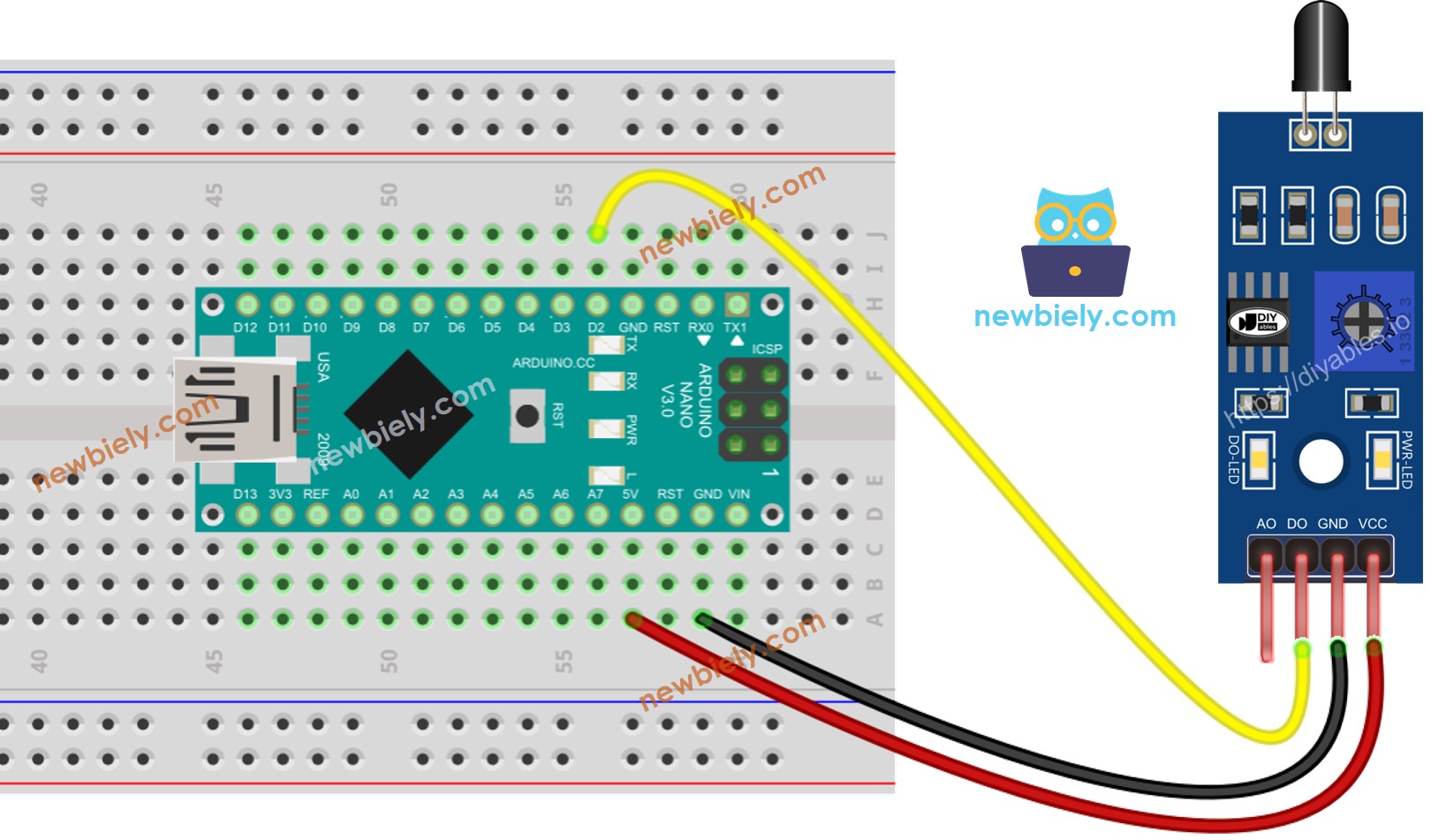
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between Arduino Nano and the flame sensor when using AO only.
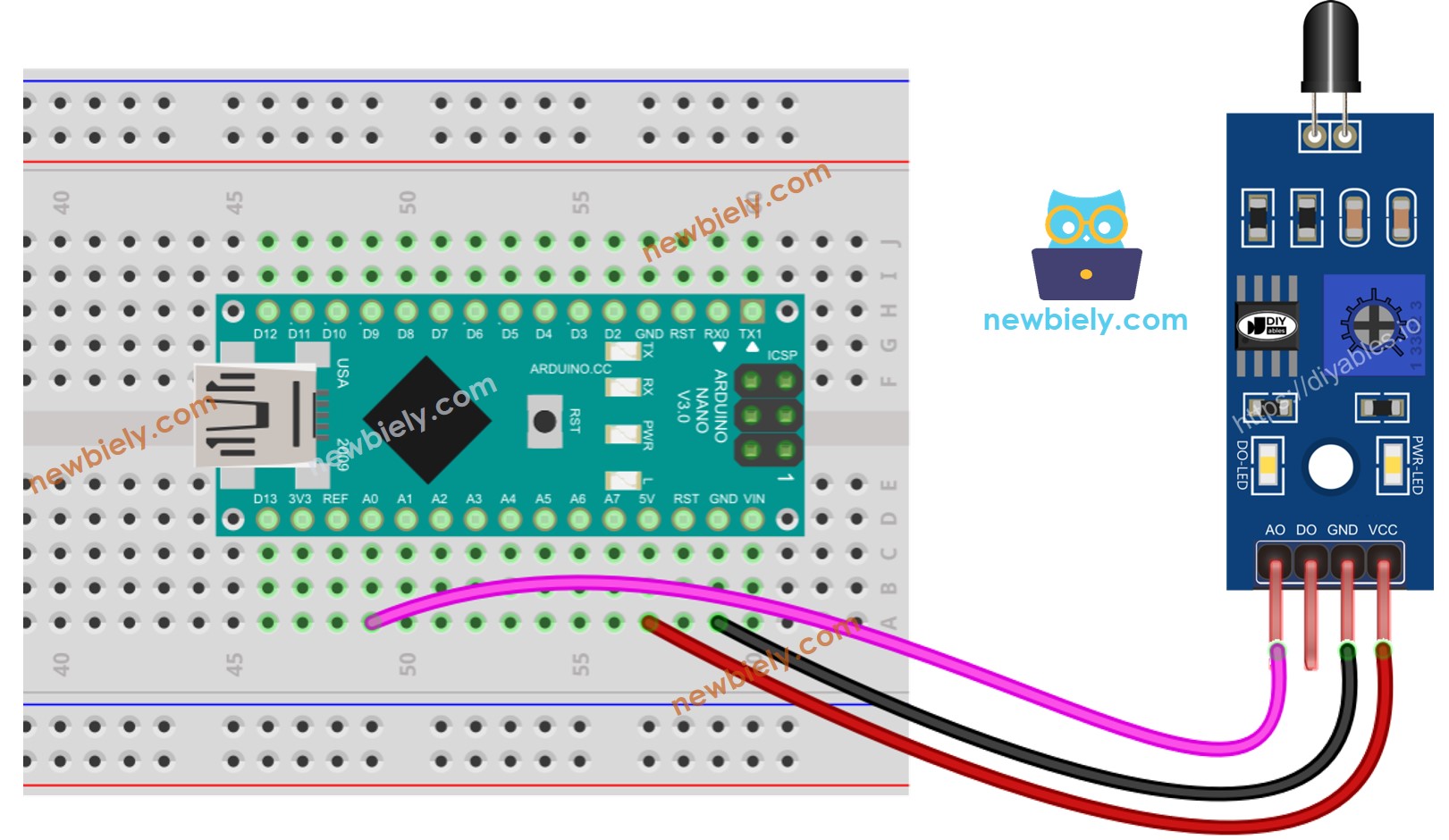
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between Arduino Nano and the flame sensor when using both AO an DO.
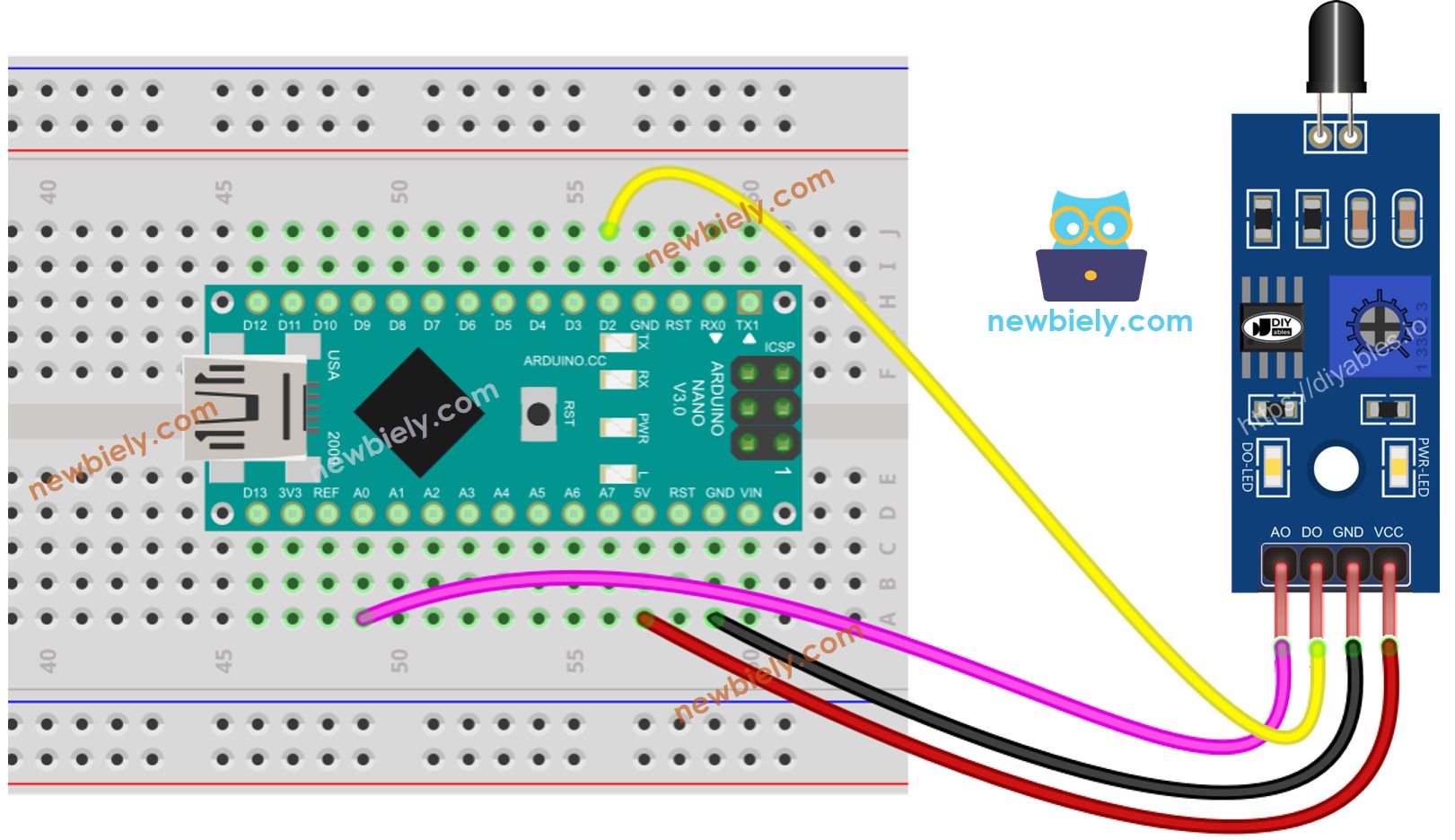
This image is created using Fritzing. Click to enlarge image
Arduino Nano Code - Read value from DO pin
Detailed Instructions
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino Nano
- Direct the flame sensor to a flame.
- Check out the result on the Serial Monitor.
Please keep in mind that if you notice the LED status remaining on constantly or off even when the sensor faces to a flame, you can adjust the potentiometer to fine-tune the sensitivity of the sensor.
Arduino Nano Code - Read value from AO pin
Detailed Instructions
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino Nano
- Direct the flame sensor to a flame.
- Check out the result on the Serial Monitor.