Arduino Nano - Actuator
This tutorial instructs you how to use Arduino Nano to control Linear Actuator. In detail, we will learn:
- How a linear actuator works
- How to program Arduino Nano to make a linear actuator extend or retract
- How to program Arduino Nano to control the speed of a linear actuator
There are two kinds of linear actuators:
- Linear actuator without feedback: It is usually used to extend/retract to the maximum. it can not be controlled to a exact position.
- Linear actuator with feedback: The feedback signal allows it to be controlled to extend/retract to a exact position.
This tutorial is for linear actuator without feedback. If you are interested in learning about linear actuator with feedback, please refer to the Arduino Nano - Actuator with Feedback tutorial.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of Linear Actuator
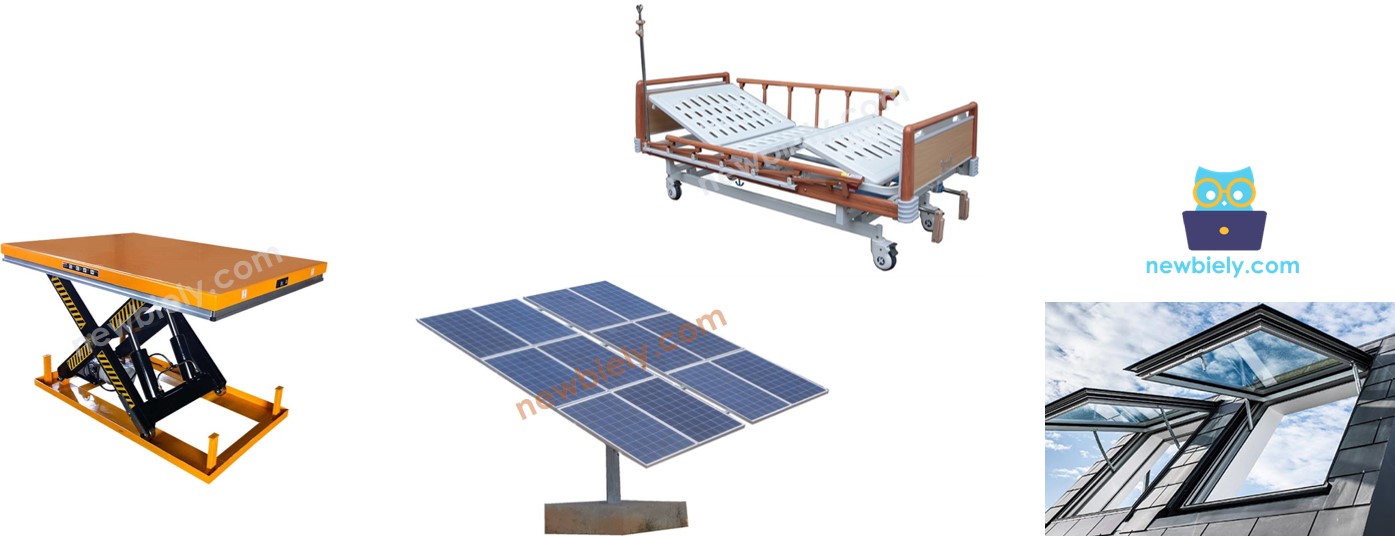
Linear Actuator Pinout
A Linear Actuator has two wires, typically:
- The Positive wire is usually red
- The Negative wire is usually black
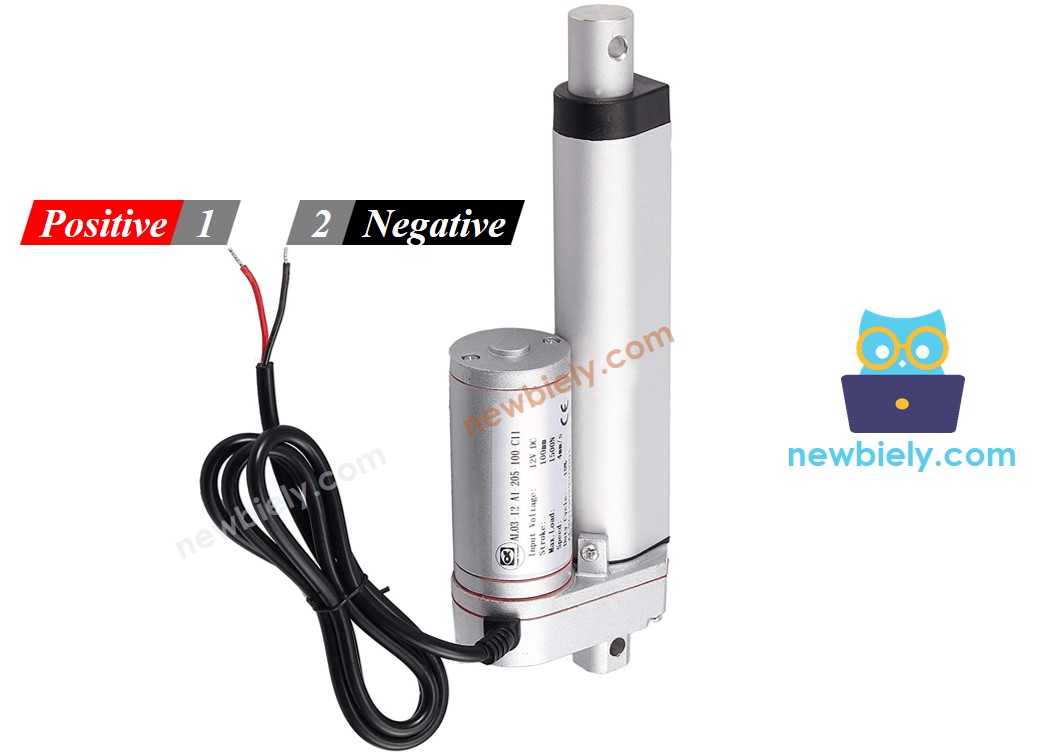
How It Works
When purchasing a linear actuator, it is essential to be aware of the voltage requirement. For instance, let us consider a 12V linear actuator.
When you power the 12V linear actuator by a 12V power source:
- Connect 12V to the positive wire and GND to the negative wire: the linear actuator full-speed extends until it reaches the limit.
- Connect 12V to the negative wire and GND to the positive wire: the linear actuator full-speed retracts until it reaches the limit.
When power is cut to the actuator, while it is in the process of extending or retracting, the movement will cease.
※ NOTE THAT:
For DC motor, servo motor, and stepper motor without gearing, when a load is present, if the power is cut off, they are unable to maintain their position. In contrast, an actuator can keep its position even when the power is no longer supplied while it is carrying a load.
If the voltage of the power supply to a linear actuator is below 12V, the linear actuator will still extend and retract, but not at its maximum speed. Changing the voltage of the power source can alter the speed of the linear actuator, but this method is not usually used due to the difficulty in controlling the voltage. A more practical approach is to keep the voltage of the power source fixed and control the speed of the linear actuator using a PWM signal. The higher the duty cycle of the PWM, the faster the linear actuator will extend and retract.
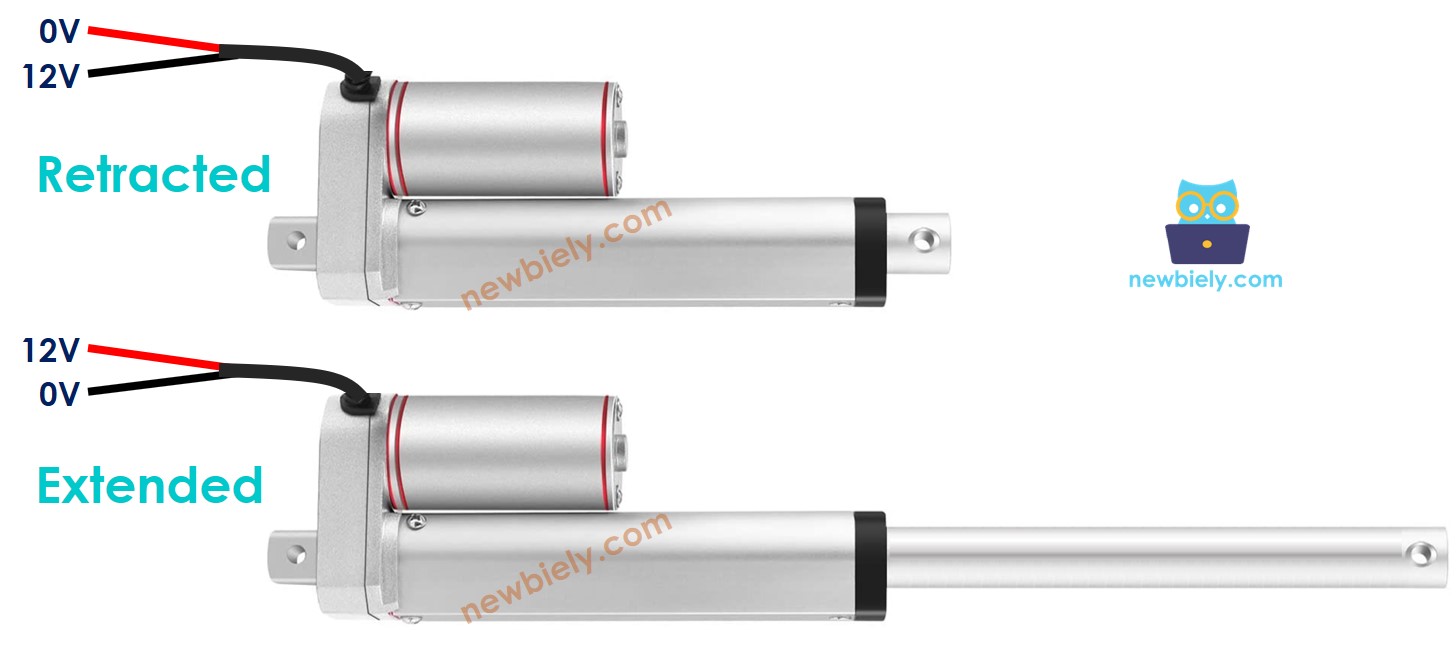
How to control a linear actuator using Arduino Nano
Controlling a linear actuator involves:
- Extending the linear actuator at its highest velocity.
- Retracting the linear actuator at its highest velocity.
- (Optional) Regulating the extending/retracting speed.
Arduino Nano can generate a signal to control the linear actuator, but it has low voltage and current. Therefore, a hardware driver is necessary to be placed between Arduino Nano and the linear actuator in order to:
- Amplify the control signal from Arduino Nano
- Receive another control signal from Arduino Nano to switch the polarity of the power supply for direction control.
※ NOTE THAT:
- This tutorial can be used with any type of linear actuator. 12V linear actuator is just one example.
- When controlling a 5V linear actuator, even though the Arduino Nano pin outputs 5V (which is the same as the linear actuator voltage), a driver is still required between the Arduino Nano and linear actuator because the Arduino Nano pin does not provide enough current for the linear actuator.
There are numerous types of chips and modules such as L293D and L298N that can be used for linear actuator drivers. For this tutorial, we will be utilizing the L298N driver.
※ NOTE THAT:
You can also use relays as a driver. However, it needs four relays to control a single linear actuator (both extend and retract).
Overview of L298N Driver
The L298N Driver can be utilized to regulate a linear actuator, DC motor, and stepper motor. This tutorial instructs you how to use it to control a linear actuator.
L298N Driver Pinout
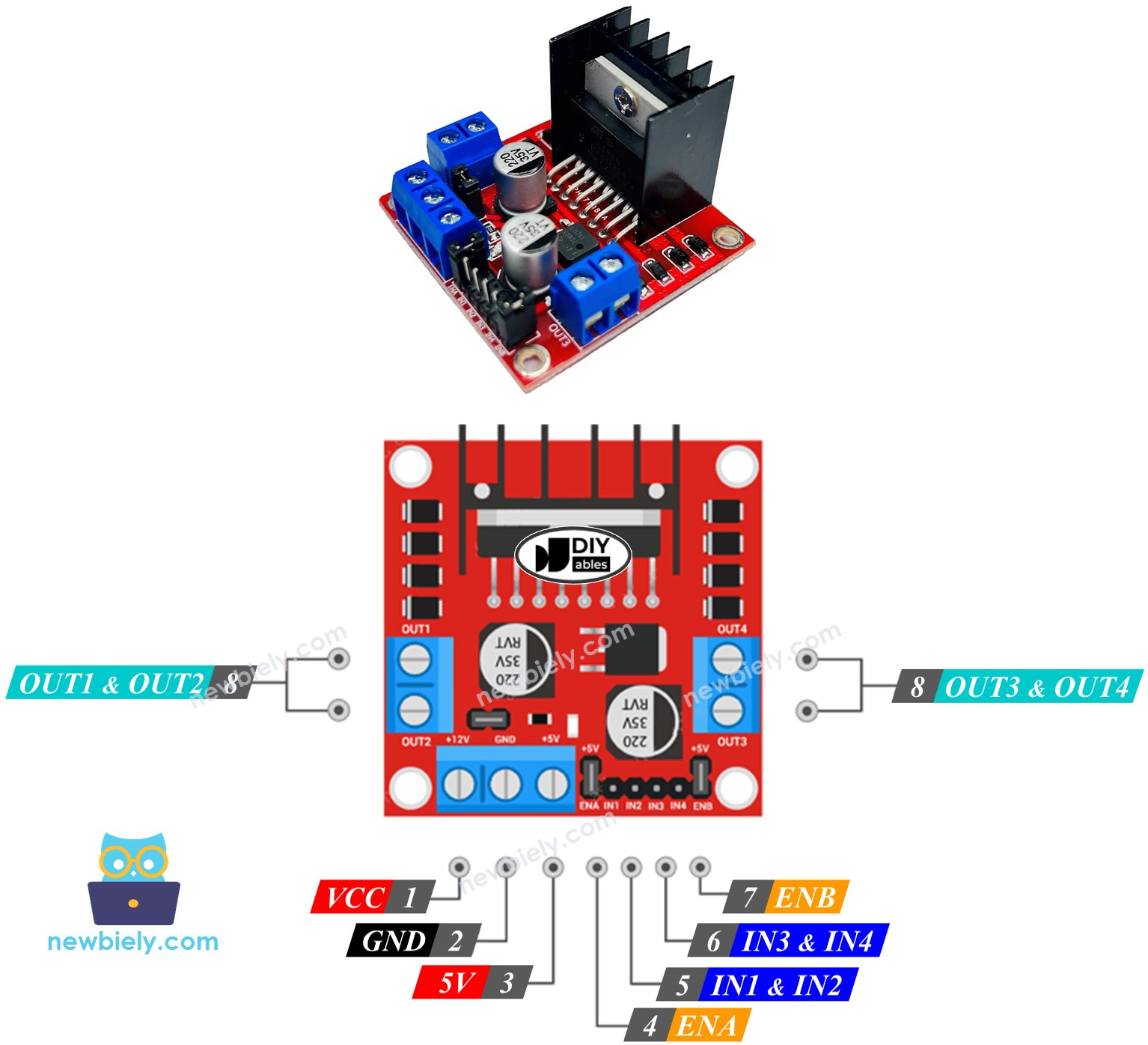
The L298N Driver has two channels, labelled as A and B. This allows it to independently control two linear actuators simultaneously. Suppose linear actuator A is connected to channel A and linear actuator B is connected to channel B. The L298N Driver has 13 pins in total.
The common pins for both channels:
- VCC pin: This pin provides power for the linear actuator and can range from 5 to 35V.
- GND pin: This is a common ground pin and should be connected to 0V (GND).
- 5V pin: This pin supplies power for the L298N module and can be supplied by 5V from an Arduino Nano.
Channel A pins:
- ENA pins: These pins are used to adjust the speed of linear actuator A. By removing the jumper and connecting this pin to a PWM input, we can control the extending/retracting speed of linear actuator A.
- IN1 & IN2 pins: These pins are used to determine the moving direction of a linear actuator. When one of them is HIGH and the other is LOW, the linear actuator will extend or retract. If both the inputs are either HIGH or LOW, the linear actuator will cease movement.
- OUT1 & OUT2 pins: These pins are connected to linear actuator A.
Channel B pins:
- ENB pins: They are used to control the speed of linear actuator B. By removing the jumper and connecting this pin to a PWM input, we can adjust the speed of extension/retraction of linear actuator B.
- IN3 & IN4 pins: These pins are utilized for controlling the direction of a linear actuator. When one of them is set to HIGH and the other to LOW, the linear actuator will extend or retract. If both the inputs are either HIGH or LOW, the linear actuator will cease to move.
- OUT3 & OUT4 pins: These pins are connected to a linear actuator.
The L298N driver has two power inputs:
- One for the linear actuator (VCC and GND pins) with a voltage range of 5 to 35V.
- One for the internal operation of the L298N module (5V and GND pins) with a voltage range of 5 to 7V.
Remove all the jumpers from the L298N driver for simplicity.
We can manage two linear actuators separately simultaneously by utilizing an Arduino Nano and an L298N Driver. For controlling each linear actuator, we require just three pins from Arduino Nano.
※ NOTE THAT:
The remainder of this tutorial will focus on controlling a linear actuator using channel A. Controlling the other linear actuator is similar.
How To Control Linear Actuator
This tutorial instructs you how to manage a Linear Actuator using an L298N driver and Arduino Nano.
Wiring Diagram
Take away all three jumpers from the L298N module prior to connecting the wires.
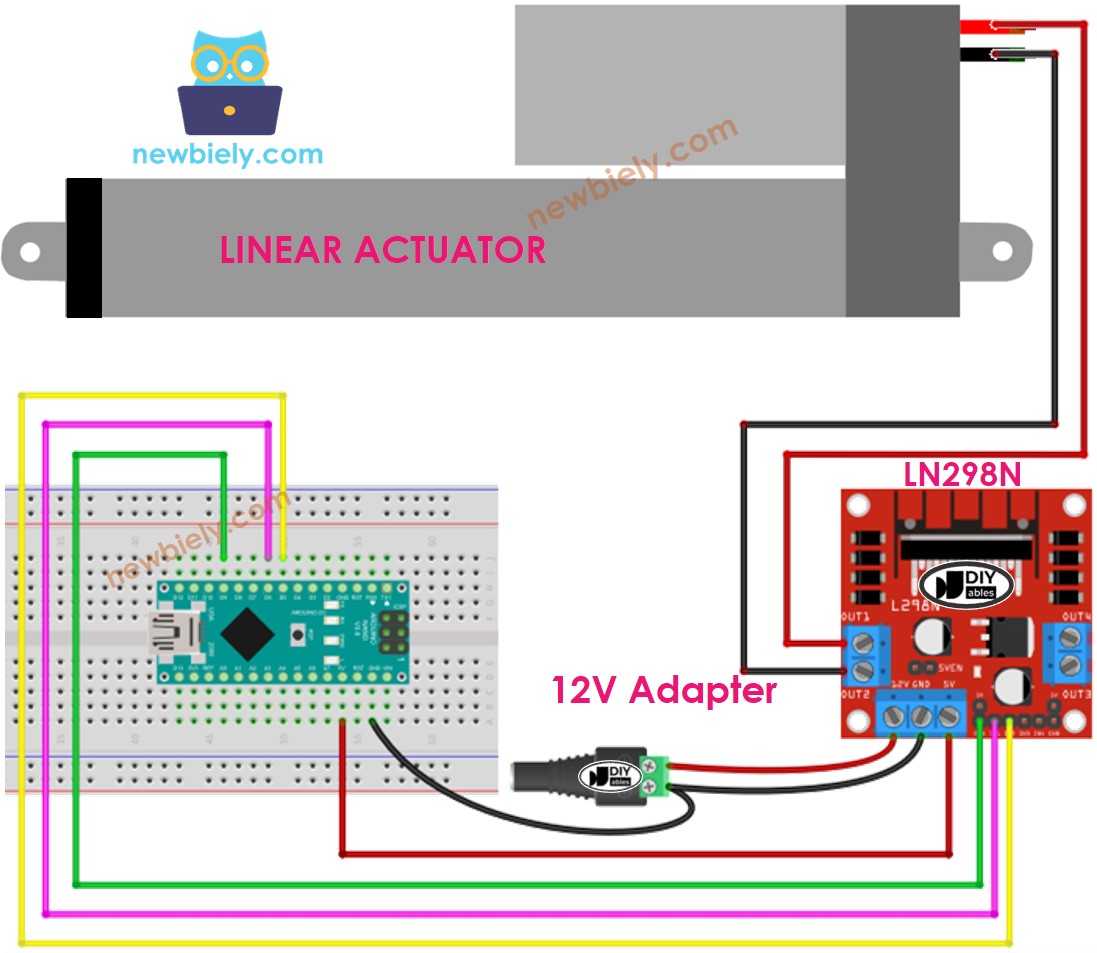
This image is created using Fritzing. Click to enlarge image
How To Make Linear Actuator Expand/Retract
The Linear Actuator's moving direction can be managed by providing a logic HIGH/LOW to IN1 and IN2 pins. The table below explains how to control the direction in both channels.
IN1 pin | IN2 pin | Direction |
---|---|---|
LOW | LOW | Linear Actuator A stops |
HIGH | HIGH | Linear Actuator A stops |
HIGH | LOW | Linear Actuator A extends |
LOW | HIGH | Linear Actuator A retracts |
- Increase the length of Linear Actuator A.
- Reverse the Linear Actuator A
※ NOTE THAT:
The orientation of the linear actuator can be reversed by connecting the OUT1 & OUT2 pins to two pins of the actuator in a different order. To do this, it is necessary to either switch the OUT1 & OUT2 pins or alter the control signal on the IN1 and IN2 pins in the code.
How To Stop Linear Actuator from Extending or Retracting
The linear actuator will cease extending/retracting when it reaches the limit. Additionally, we can program it to stop extending/retracting before it has reached the limit.
- Cut off the power
There are two methods to halt linear actuator:
- Reduce the speed to 0
- Disconnect the power supply
- Sets IN1 IN2 pins to the same value, either LOW or HIGH.
Or
How To Control the Speed of Linear Actuator via L298N Driver
It is easy to manage the speed of the linear actuator. Instead of setting ENA pin to HIGH, we can create a PWM signal to the ENA pin. This can be done by:
- Linking an Arduino Nano pin to the ENA of the L298N
- Utilizing the analogWrite() function to generate a PWM signal to the ENA pin. The L298N Driver will amplify the PWM signal to the linear actuator.
The speed is a range from 0 to 255. If the speed is 0, the linear actuator ceases movement. When the speed is 255, the linear actuator extends/retracts at its highest velocity.
Arduino Nano Example Code
The code:
- Increases the linear actuator to its maximum speed
- Halts the linear actuator
- Decreases the linear actuator to its maximum speed
- Halts the linear actuator
Detailed Instructions
Take out all three jumpers from the L298N module.
Copy the code and open it in the Arduino IDE.
Click the Upload button in the Arduino IDE to upload the code to the Arduino Nano.
You will observe:
- The linear actuator will extend until it reaches its limit, then it will stop.
- The linear actuator will stay in this position for a certain amount of time.
- The linear actuator will then retract until it reaches its limit, then it will stop.
- The linear actuator will remain in this position for a certain amount of time.
- This process will repeat itself.