Arduino Nano - RFID - Relay
This tutorial instructs you how to use an Arduino Nano, RFID NFC RC522 module to control a relay. You can further extend this tutorial by using the relay to control motors, actuators, door lock, light bulb, and more.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of RFID/NFC RC522 Module and Relay
If you are unfamiliar with the RFID/NFC RC522 Module and relay (pinout, how it works, how to program ...), you can find out more in the following tutorials:
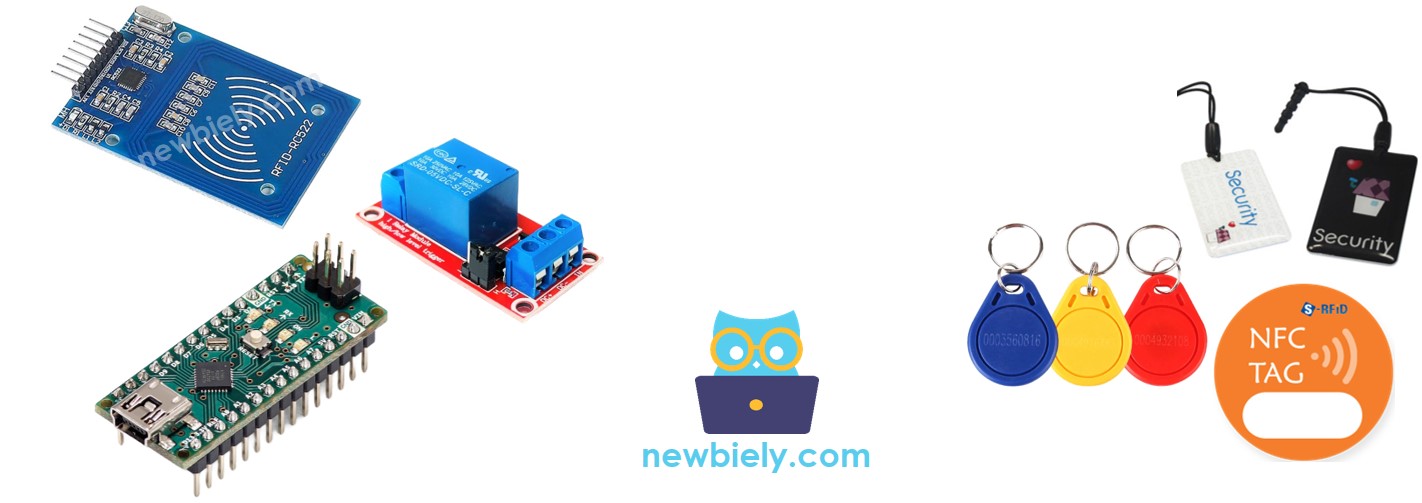
How It Works
- The RFID/NFC reader reads the UID from the tag when a user taps it.
- Arduino Nano then obtains the UID from the reader.
- It compares this UID with the UIDs that have been predefined in the Arduino Nano code.
- If the UID matches one of the predefined UIDs, the Arduino Nano will activate the relay.
Wiring Diagram
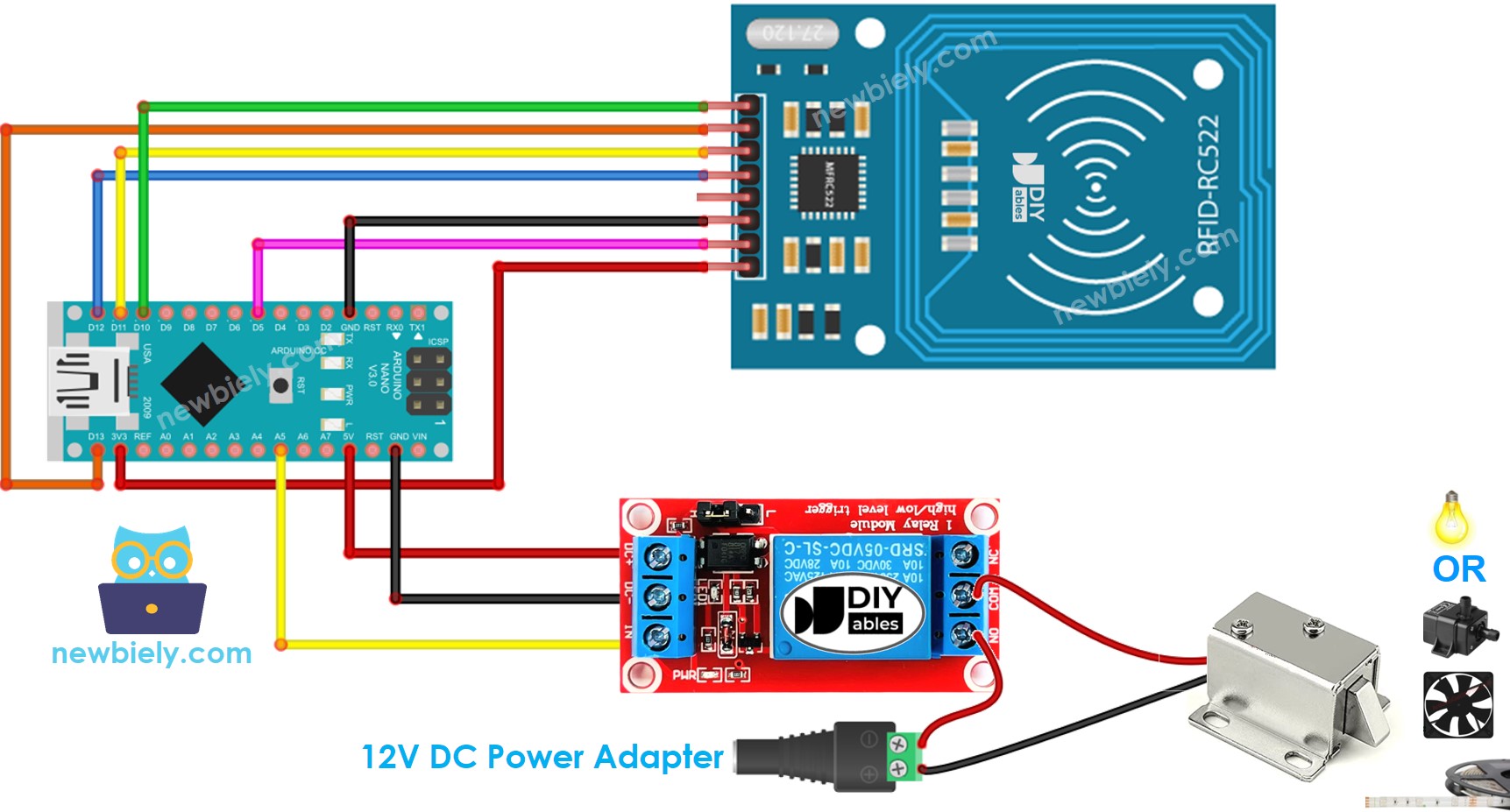
This image is created using Fritzing. Click to enlarge image
To simplify the connection, the pins of the RC522 module are directly connected to the pins of the Arduino. However, in certain cases, this can cause the Arduino to malfunction since its output pins generate 5V, while the pins of the RC522 module operate normally at 3.3V. Therefore, it is advisable to regulate the voltage between the pins of the Arduino and the RC522 module. For further details, please refer to the Arduino Nano - RFID RC522 tutorial. The diagram below illustrates how to regulate 5V to 3.3V using resistors:
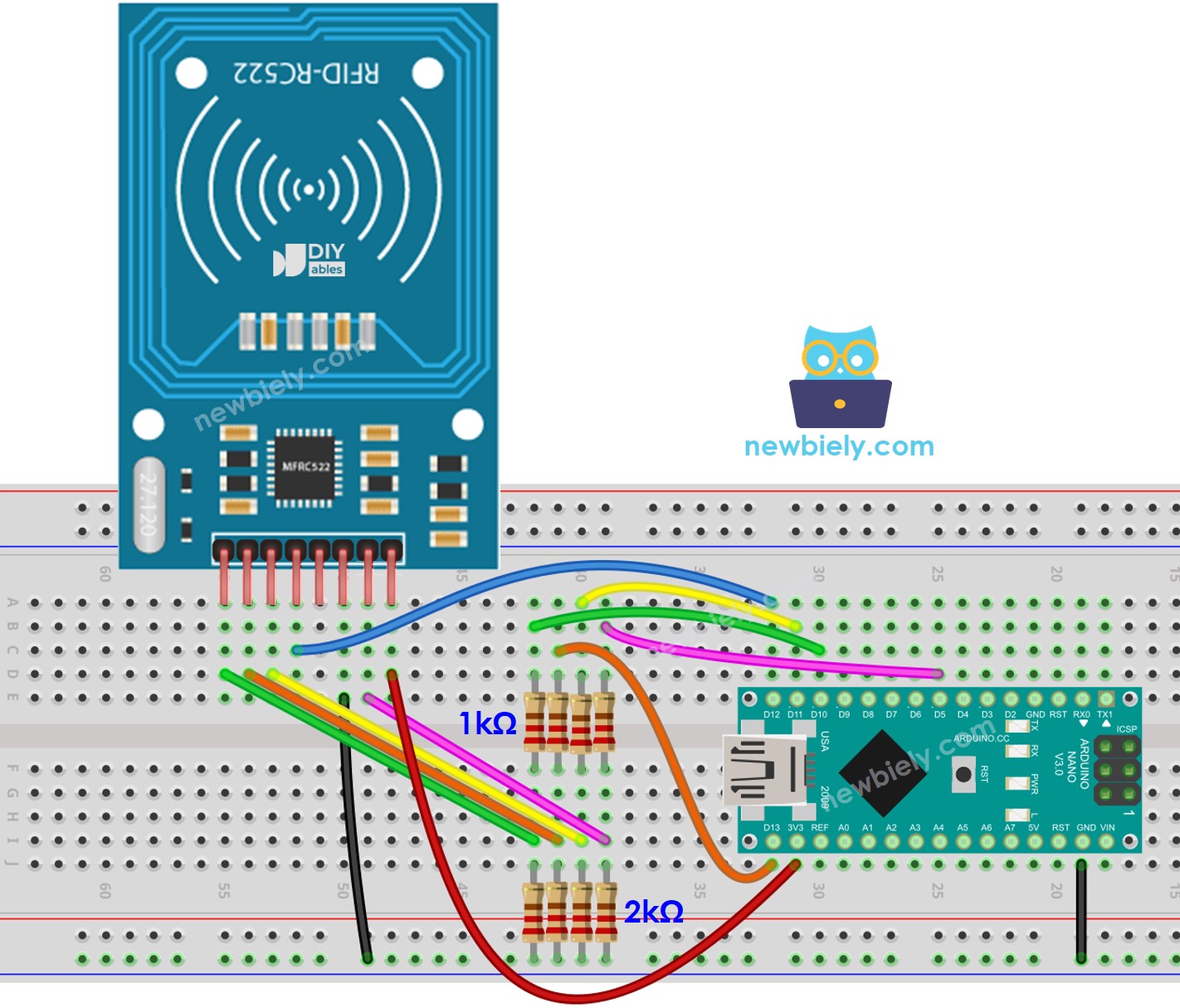
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
※ NOTE THAT:
The arrangement of pins may differ depending on the manufacturer. ALWAYS refer to the labels printed on the module. The image above displays the pinout of modules from DIYables manufacturer.
Arduino Nano Code - Single RFID/NFC Tag
Detailed Instructions
To discover the UID of an RFID/NFC tag, the first step is to upload code to Arduino Nano and open the Serial Monitor. Then, tap the tag on the RFID-RC522 module and view the UID on the Serial Monitor.
After obtaining the UID:
- Alter line 18 of the code to reflect the UID, for example changing byte authorizedUID[4] = {0xFF, 0xFF, 0xFF, 0xFF}; to byte authorizedUID[4] = {0x3A, 0xC9, 0x6A, 0xCB};
- Upload the updated code to the Arduino Nano
- Place an RFID/NFC tag on the RFID-RC522 module
- Check out the output on the Serial Monitor
- Place an RFID/NFC tag on the RFID-RC522 module and observe the output on the Serial Monitor.
※ NOTE THAT:
- To ensure testing is straightforward, the active time is set to 2 seconds; however, it should be increased for practical use/demonstration.
- Installation of the MFRC522 library is necessary. For more information, please refer to the Arduino Nano - RFID/NFC RC522 tutorial.