Arduino Nano - Traffic Light
This tutorial instructs you how to use an Arduino Nano to control a traffic light module. Specifically, we'll cover the following aspects:
- Establishing the connection between the traffic light module and the Arduino Nano
- Programming the Arduino Nano to oversee the RGB traffic light module
- Implementing Arduino Nano programming to regulate the RGB traffic light module without relying on the delay() function.
Hardware Preparation
1 | × | Arduino Nano | |
1 | × | USB A to Mini-B USB cable | |
1 | × | Traffic Light Module | |
1 | × | Jumper Wires | |
1 | × | (Optional) 9V Power Adapter for Arduino Nano | |
1 | × | (Recommended) Screw Terminal Adapter for Arduino Nano |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you. We appreciate your support.
Overview of Traffic Light Module
Pinout
A traffic light module comprises four pins:
- GND pin: Connect this pin to the GND of Arduino Nano.
- R pin: Controls the red light; connect this pin to a digital output of Arduino Nano.
- Y pin: Manages the yellow light; connect this pin to a digital output of Arduino Nano.
- G pin: Governs the green light; connect this pin to a digital output of Arduino Nano.
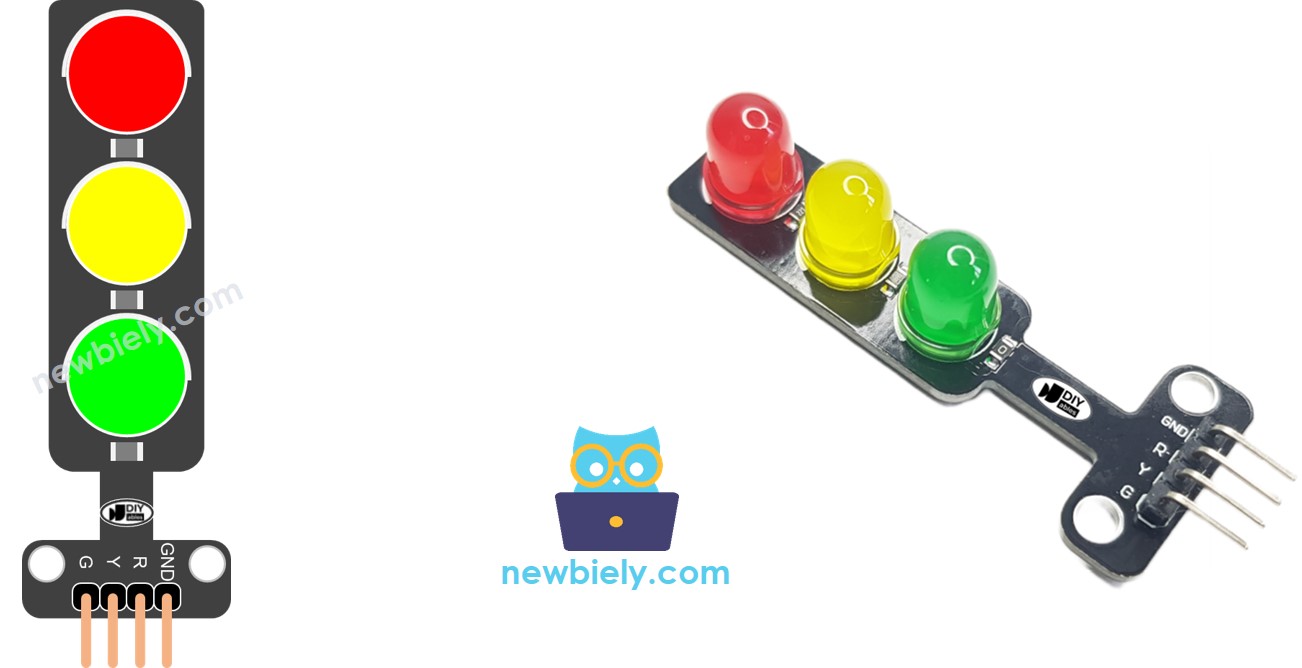
How It Works
Wiring Diagram
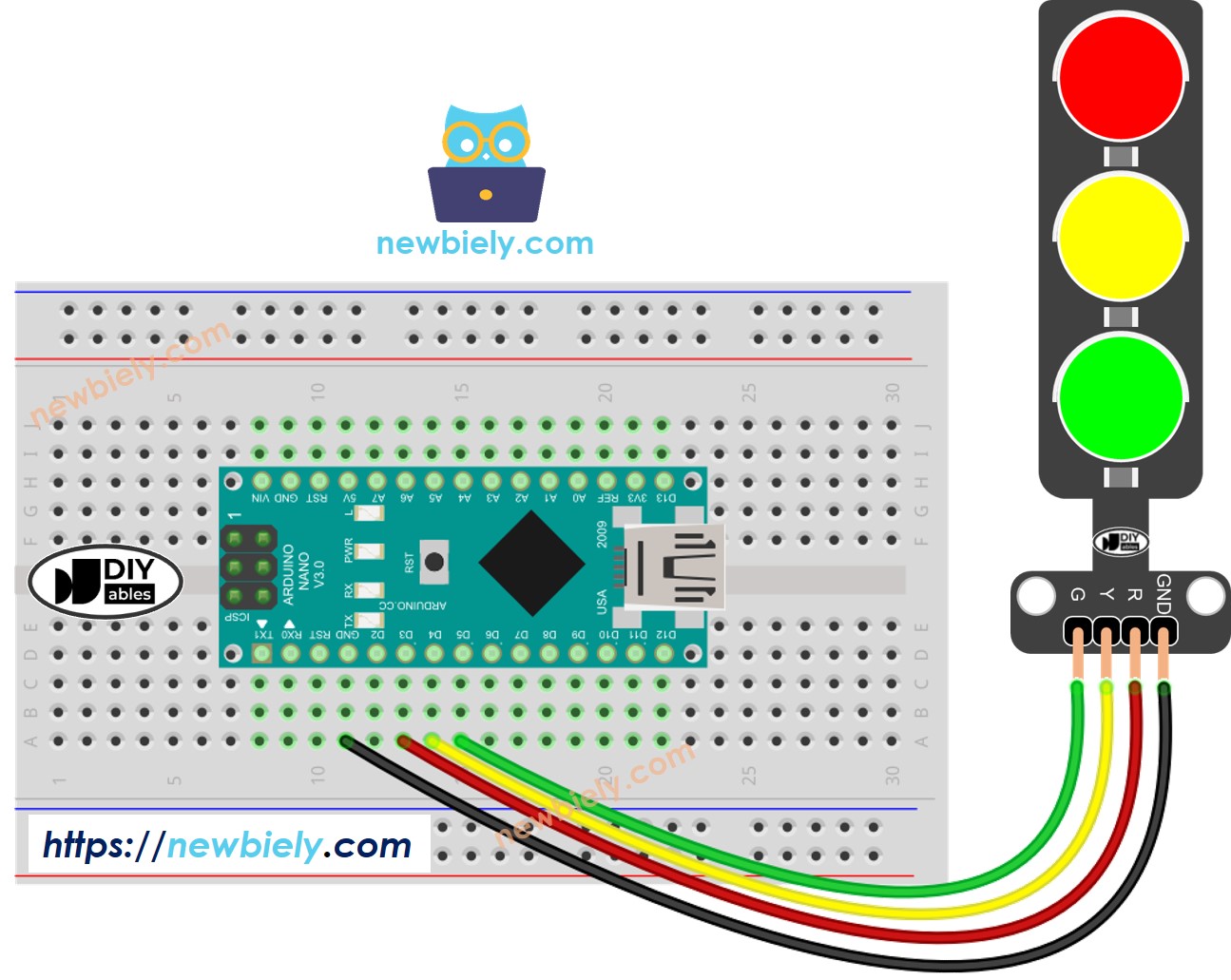
This image is created using Fritzing. Click to enlarge image
How To Program For Traffic Light module
- Configure an Arduino Nano's pins to the digital output mode by using pinMode() function
pinMode(PIN_RED, OUTPUT);
pinMode(PIN_YELLOW, OUTPUT);
pinMode(PIN_GREEN, OUTPUT);
- Program to turn ON red light by using digitalWrite() function:
digitalWrite(PIN_RED, HIGH); // turn on RED
digitalWrite(PIN_YELLOW, LOW); //
digitalWrite(PIN_GREEN, LOW);
delay(RED_TIME); // keep red led on during a period of time
Arduino Nano Code
/*
* This Arduino Nano code was developed by newbiely.com
*
* This Arduino Nano code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-nano/arduino-nano-traffic-light
*/
#define PIN_RED 3 // The Arduino Nano pin connected to R pin of traffic light module
#define PIN_YELLOW 4 // The Arduino Nano pin connected to Y pin of traffic light module
#define PIN_GREEN 5 // The Arduino Nano pin connected to G pin of traffic light module
#define RED_TIME 4000 // RED time in millisecond
#define YELLOW_TIME 4000 // YELLOW time in millisecond
#define GREEN_TIME 4000 // GREEN time in millisecond
void setup() {
pinMode(PIN_RED, OUTPUT);
pinMode(PIN_YELLOW, OUTPUT);
pinMode(PIN_GREEN, OUTPUT);
}
// The loop function repeats indefinitely
void loop() {
// red light on
digitalWrite(PIN_RED, HIGH); // turn on
digitalWrite(PIN_YELLOW, LOW); // turn off
digitalWrite(PIN_GREEN, LOW); // turn off
delay(RED_TIME); // keep red light on during a period of time
// yellow light on
digitalWrite(PIN_RED, LOW); // turn off
digitalWrite(PIN_YELLOW, HIGH); // turn on
digitalWrite(PIN_GREEN, LOW); // turn off
delay(YELLOW_TIME); // keep yellow light on during a period of time
// green light on
digitalWrite(PIN_RED, LOW); // turn off
digitalWrite(PIN_YELLOW, LOW); // turn off
digitalWrite(PIN_GREEN, HIGH); // turn on
delay(GREEN_TIME); // keep green light on during a period of time
}
Detailed Instructions
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino Nano
- Check out the traffic light module
It's important to note that the exact workings of a traffic light can vary depending on the specific design and technology used in different regions and intersections. The principles described above provide a general understanding of how traffic lights operate to manage traffic and enhance safety on the roads.
The code above demonstrates individual light control. Now, let's enhance the code for better optimization.
Arduino Nano Code Optimization
- Let's improve the code by implementing a function for light control.
/*
* This Arduino Nano code was developed by newbiely.com
*
* This Arduino Nano code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-nano/arduino-nano-traffic-light
*/
#define PIN_RED 3 // The Arduino Nano pin connected to R pin of traffic light module
#define PIN_YELLOW 4 // The Arduino Nano pin connected to Y pin of traffic light module
#define PIN_GREEN 5 // The Arduino Nano pin connected to G pin of traffic light module
#define RED_TIME 2000 // RED time in millisecond
#define YELLOW_TIME 1000 // YELLOW time in millisecond
#define GREEN_TIME 2000 // GREEN time in millisecond
#define RED 0 // Index in array
#define YELLOW 1 // Index in array
#define GREEN 2 // Index in array
const int pins[] = { PIN_RED, PIN_YELLOW, PIN_GREEN };
const int times[] = { RED_TIME, YELLOW_TIME, GREEN_TIME };
void setup() {
pinMode(PIN_RED, OUTPUT);
pinMode(PIN_YELLOW, OUTPUT);
pinMode(PIN_GREEN, OUTPUT);
}
// The loop function repeats indefinitely
void loop() {
// red light on
trafic_light_on(RED);
delay(times[RED]); // keep red light on during a period of time
// yellow light on
trafic_light_on(YELLOW);
delay(times[YELLOW]); // keep yellow light on during a period of time
// green light on
trafic_light_on(GREEN);
delay(times[GREEN]); // keep green light on during a period of time
}
void trafic_light_on(int light) {
for (int i = RED; i <= GREEN; i++) {
if (i == light)
digitalWrite(pins[i], HIGH); // turn on
else
digitalWrite(pins[i], LOW); // turn off
}
}
- Let's improve the code by using a for loop.
/*
* This Arduino Nano code was developed by newbiely.com
*
* This Arduino Nano code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-nano/arduino-nano-traffic-light
*/
#define PIN_RED 3 // The Arduino Nano pin connected to R pin of traffic light module
#define PIN_YELLOW 4 // The Arduino Nano pin connected to Y pin of traffic light module
#define PIN_GREEN 5 // The Arduino Nano pin connected to G pin of traffic light module
#define RED_TIME 2000 // RED time in millisecond
#define YELLOW_TIME 1000 // YELLOW time in millisecond
#define GREEN_TIME 2000 // GREEN time in millisecond
#define RED 0 // Index in array
#define YELLOW 1 // Index in array
#define GREEN 2 // Index in array
const int pins[] = {PIN_RED, PIN_YELLOW, PIN_GREEN};
const int times[] = {RED_TIME, YELLOW_TIME, GREEN_TIME};
void setup() {
pinMode(PIN_RED, OUTPUT);
pinMode(PIN_YELLOW, OUTPUT);
pinMode(PIN_GREEN, OUTPUT);
}
// The loop function repeats indefinitely
void loop() {
for (int light = RED; light <= GREEN; light ++) {
trafic_light_on(light);
delay(times[light]); // keep light on during a period of time
}
}
void trafic_light_on(int light) {
for (int i = RED; i <= GREEN; i ++) {
if (i == light)
digitalWrite(pins[i], HIGH); // turn on
else
digitalWrite(pins[i], LOW); // turn off
}
}
- Let's improve the code by using millis() function intead of delay().
/*
* This Arduino Nano code was developed by newbiely.com
*
* This Arduino Nano code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-nano/arduino-nano-traffic-light
*/
#define PIN_RED 3 // The Arduino Nano pin connected to R pin of traffic light module
#define PIN_YELLOW 4 // The Arduino Nano pin connected to Y pin of traffic light module
#define PIN_GREEN 5 // The Arduino Nano pin connected to G pin of traffic light module
#define RED_TIME 2000 // RED time in millisecond
#define YELLOW_TIME 1000 // YELLOW time in millisecond
#define GREEN_TIME 2000 // GREEN time in millisecond
#define RED 0 // Index in array
#define YELLOW 1 // Index in array
#define GREEN 2 // Index in array
const int pins[] = { PIN_RED, PIN_YELLOW, PIN_GREEN };
const int times[] = { RED_TIME, YELLOW_TIME, GREEN_TIME };
unsigned long last_time = 0;
int light = RED; // start with RED light
void setup() {
pinMode(PIN_RED, OUTPUT);
pinMode(PIN_YELLOW, OUTPUT);
pinMode(PIN_GREEN, OUTPUT);
trafic_light_on(light);
last_time = millis();
}
// The loop function repeats indefinitely
void loop() {
if ((millis() - last_time) > times[light]) {
light++;
if (light >= 3)
light = RED; // new circle
trafic_light_on(light);
last_time = millis();
}
// TO DO: your other code
}
void trafic_light_on(int light) {
for (int i = RED; i <= GREEN; i++) {
if (i == light)
digitalWrite(pins[i], HIGH); // turn on
else
digitalWrite(pins[i], LOW); // turn off
}
}