Arduino Nano - Button
This tutorial instructs you how to use Arduino Nano with button. In detail, we will learn:
How to connect the button to Arduino Nano
How to program Arduino Nano to read the state of the button
How to program Arduino Nano to detect the pressing and releasing events from button.
How to prevent the floating input problem when using the button with Arduino Nano.
How to prevent the chattering problem when using the button with Arduino Nano.
The button is referred to as a pushbutton, tactile button or momentary switch. It is a fundamental component and is frequently used in Arduino projects. It is straightforward to use. However, it can be confusing for beginners because of mechanical, physical aspects and the various ways it can be used. This tutorial makes it easier for beginners.
Or you can buy the following sensor kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
Beginners typically encounter two common difficulties when using a button:
1. Floating input problem:.
Symptom: The input pin's read value does not correspond with the button's pressed state.
Cause: The input pin does not have a pull-up or pull-down resistor in use.
Solution: Use a pull-up or pull-down resistor, and the steps to do so will be explained in this tutorial.
2. Chattering phenomenon:.
Symptom: Although the button is pressed only once, the Arduino code detects multiple presses.
Cause: The mechanical and physical problems are causing the button (or switch) state to rapidly toggle between LOW and HIGH multiple times.
Only applications that requires precise detection of the number of presses should take this into consideration.
※ NOTE THAT:
Do not confuse the button with the following:
The push button, also referred to as a pushbutton, tactile button, or momentary switch, is a type of switch that closes when the button is pressed and held, and opens when released. Various types of push buttons exist, which can be broadly categorized into two groups:
Nevertheless, these pins are linked together in pairs. Consequently, only two of the four pins need to be used, which are not connected internally.
There are four possible ways to connect to the button, two of which are symmetrical (as seen in the image).
? Why is it that only two pins of a button are utilized, when it has four?
⇒ To ensure that it is securely mounted on the PCB (printed circuit board) and can withstand any pressing force.
image source: diyables.io
When the button is not pressed, pin A and pin B are not connected.
However, when the button is pressed, pin A and pin B become connected.
One button's pin is connected to either VCC or GND. The other pin of the same button is connected to a pin on an Arduino Nano board. By examining the state of an Arduino Nano pin set as an input, we can determine whether or not a button has been pressed.
The connection between the button and Arduino Nano, as well as the configuration of the Arduino's pin, will determine the relationship between the button state and the pressing state.
There are two ways to use a button with Arduino:
One button's pin is connected to VCC, the other is connected to an Arduino's pin with a pull-down resistor
When the button is pressed, the Arduino's pin state will be HIGH. If not, the Arduino's pin state will be LOW
An external resistor is required in this case.
One button's pin is connected to GND, the other is connected to an Arduino's pin with a pull-up resistor
When the button is pressed, the Arduino's pin state will be LOW. If not, the Arduino's pin state will be HIGH
We can use either an internal or external resistor. The internal resistor is already built into Arduino Nano, and can be set via Arduino Nano code.
※ NOTE THAT:
If we do NOT use any external pull-down/pull-up resistor, the state of the input pin is “floating” when the button is NOT pressed. This means the state can be HIGH or LOW (unstable, unfixed), resulting in incorrect detection.
The worst practice: Initializes the Arduino pin as an input (pinMode(BUTTON_PIN, INPUT)) without utilizing any external pull-down or pull-up resistors, .
The best practice: initializes the Arduino pin as an internal pull-up input (by using pinMode(BUTTON_PIN, INPUT_PULLUP)). It does NOT need to use any external pull-down/pull-up resistor.
For the sake of simplicity for those just starting out, this tutorial uses the most straightforward approach: initializing the Arduino Nano pin as an internal pull-up input without requiring an external resistor. There is no need for the beginners to worry about how to connect the pull-up/pull-down resistor. All they have to do is use the Arduino Nano code.
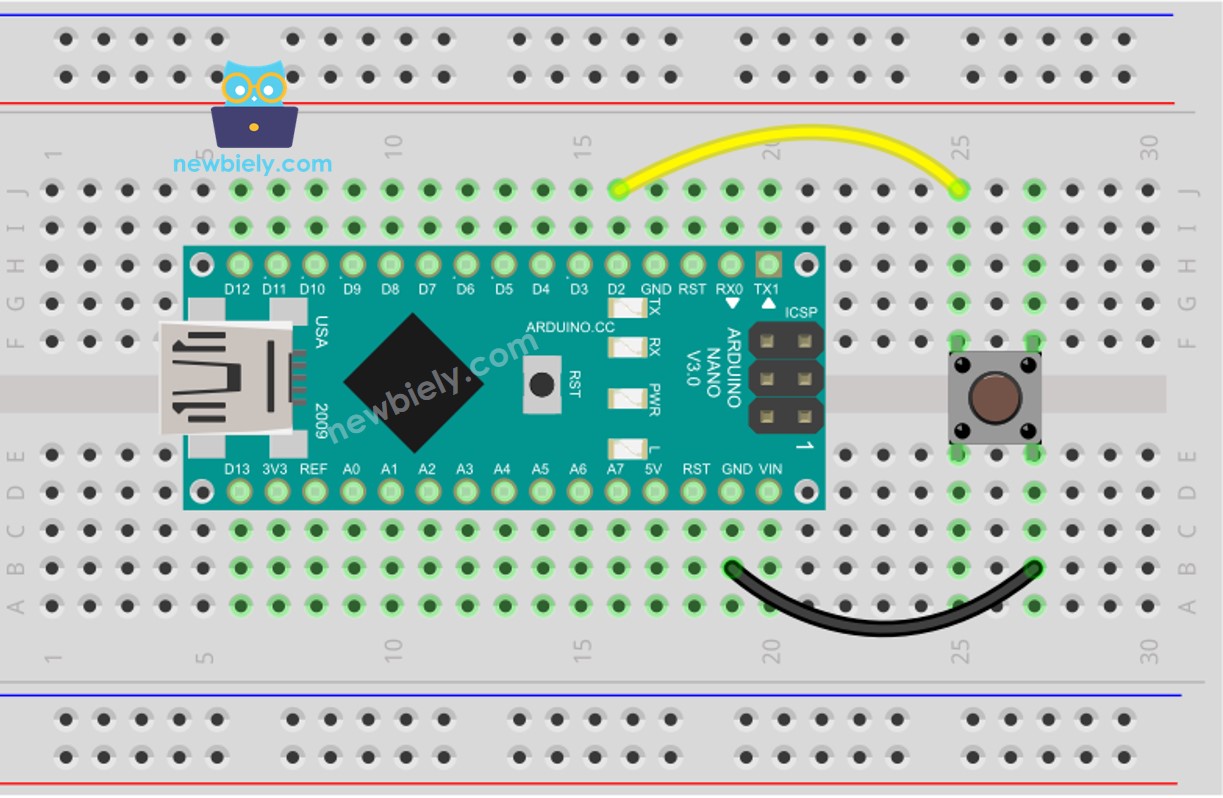
This image is created using Fritzing. Click to enlarge image
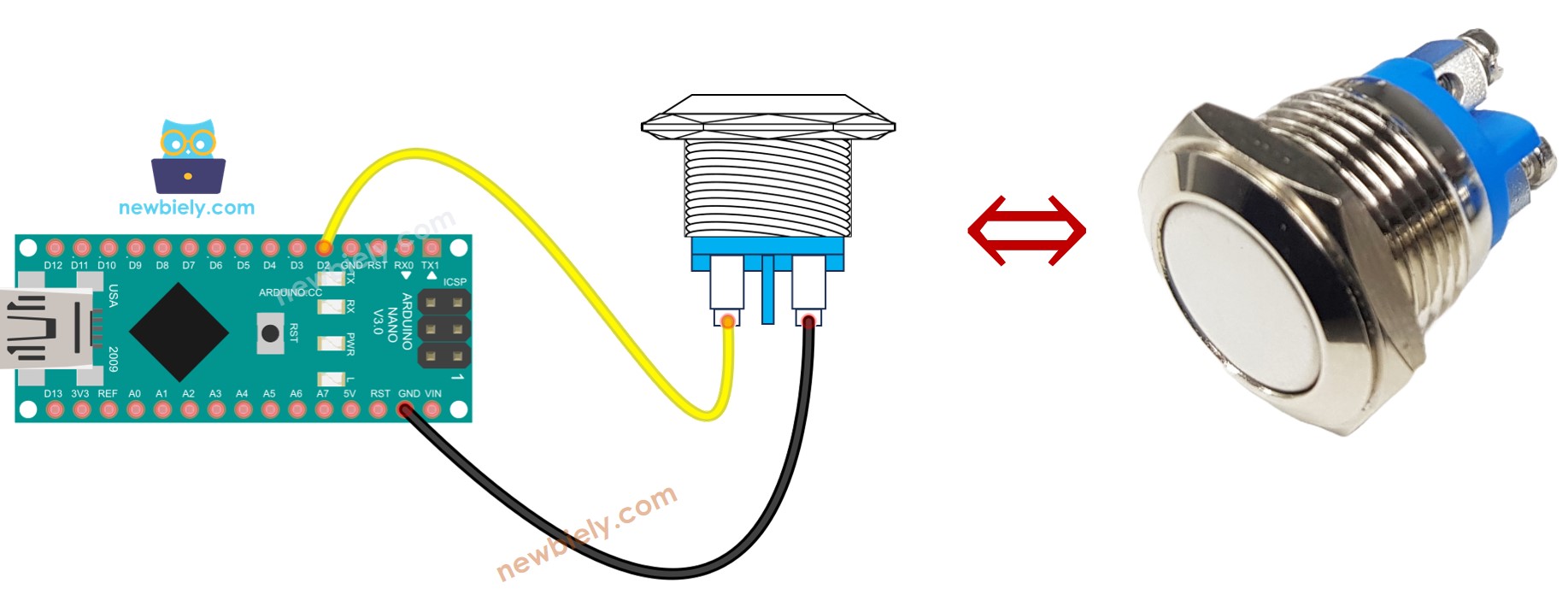
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Use the
pinMode() function to set the Arduino Nano pin as an internal pull-up input.
For instance, pin 2:
pinMode(2, INPUT_PULLUP);
Utilizes the
digitalRead() function to ascertain the state of the Arduino Nano pin.
int button_state = digitalRead(BUTTON_PIN);
※ NOTE THAT:
Two common use cases exist:
The first: If the input state is HIGH, perform one action. If the input state is LOW, take the opposite action.
The second: If the input state changes from LOW to HIGH (or HIGH to LOW), do something.
We select one of these based on the application. For example, when using a button to control an LED:
If we want the LED to be ON when the button is pressed and OFF when the button is NOT pressed, we SHOULD use the first use case.
If we want the LED to be toggled between ON and OFF each time we press the button, we SHOULD use the second use case.
const int BUTTON_PIN = 2;
int prev_button_state = HIGH;
int button_state;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
button_state = digitalRead(BUTTON_PIN);
if(prev_button_state == LOW && button_state == HIGH)
Serial.println("The state changed from LOW to HIGH");
prev_button_state = button_state;
}
Connect your Arduino Nano to a computer using a USB cable.
Launch the Arduino IDE, select the correct board and port.
Copy the code below and open it in the Arduino IDE.
#define BUTTON_PIN 2
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
int button_state = digitalRead(BUTTON_PIN);
Serial.println(button_state);
}
1 is HIGH, 0 is LOW.
Check out the line-by-line explanation contained in the comments of the source code!
Let's modify the code so that it can recognize press and release events.
#define BUTTON_PIN 2
int prev_button_state = LOW;
int button_state;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
button_state = digitalRead(BUTTON_PIN);
if(prev_button_state == HIGH && button_state == LOW)
Serial.println("The button is pressed");
else if(prev_button_state == LOW && button_state == HIGH)
Serial.println("The button is released");
prev_button_state = button_state;
}
The button is pressed
The button is released
※ NOTE THAT:
Even if you only press and release the button once, the output in the Serial Monitor may display multiple pressed and released events. This is the typical behavior of the button and is known as the "chattering phenomenon". To solve this problem, please refer to the Arduino Nano - Button Debounce tutorial.
※ NOTE THAT:
We have developed a library, ezButton, to simplify the process for those just starting out, particularly when dealing with multiple buttons. You can find out more about the ezButton library here.
When the button is pressed, the LED will be turned on.
When the button is not pressed, the LED will be turned off.
Each time the button is pressed, the LED will switch between ON and OFF.
What are the occasions when it is appropriate to use a pull-down/pull-up resistor for an input pin? What are the times when it is not suitable to utilize a pull-down/pull-up resistor for an input pin?
If the sensor has either closed or open states, a pull-up or pull-down resistor is needed to make them become LOW and HIGH. Examples of such sensors include push-button, switch, and magnetic contact switch (door sensor).
On the other hand, if the sensor has two defined voltage levels (LOW and HIGH), no pull-up or pull-down resistor is required. Examples of such sensors are motion sensor and touch sensor.