Arduino Nano - Bluetooth LED Matrix
This tutorial instructs you how to use Arduino Nano to control an LED matrix display with a smartphone through either Bluetooth or BLE.
We will have the option to select between two different modules: HC-05 for Classic Bluetooth (Bluetooth 2.0) and HM-10 for Bluetooth Low Energy (BLE, Bluetooth 4.0). The tutorial will provide step-by-step instructions for both modules with Arduino Nano.
To send messages from the smartphone to the Arduino Nano, we will use the Bluetooth Serial Monitor App. Once the message is received by Arduino Nano, it will be displayed on the LED matrix display.
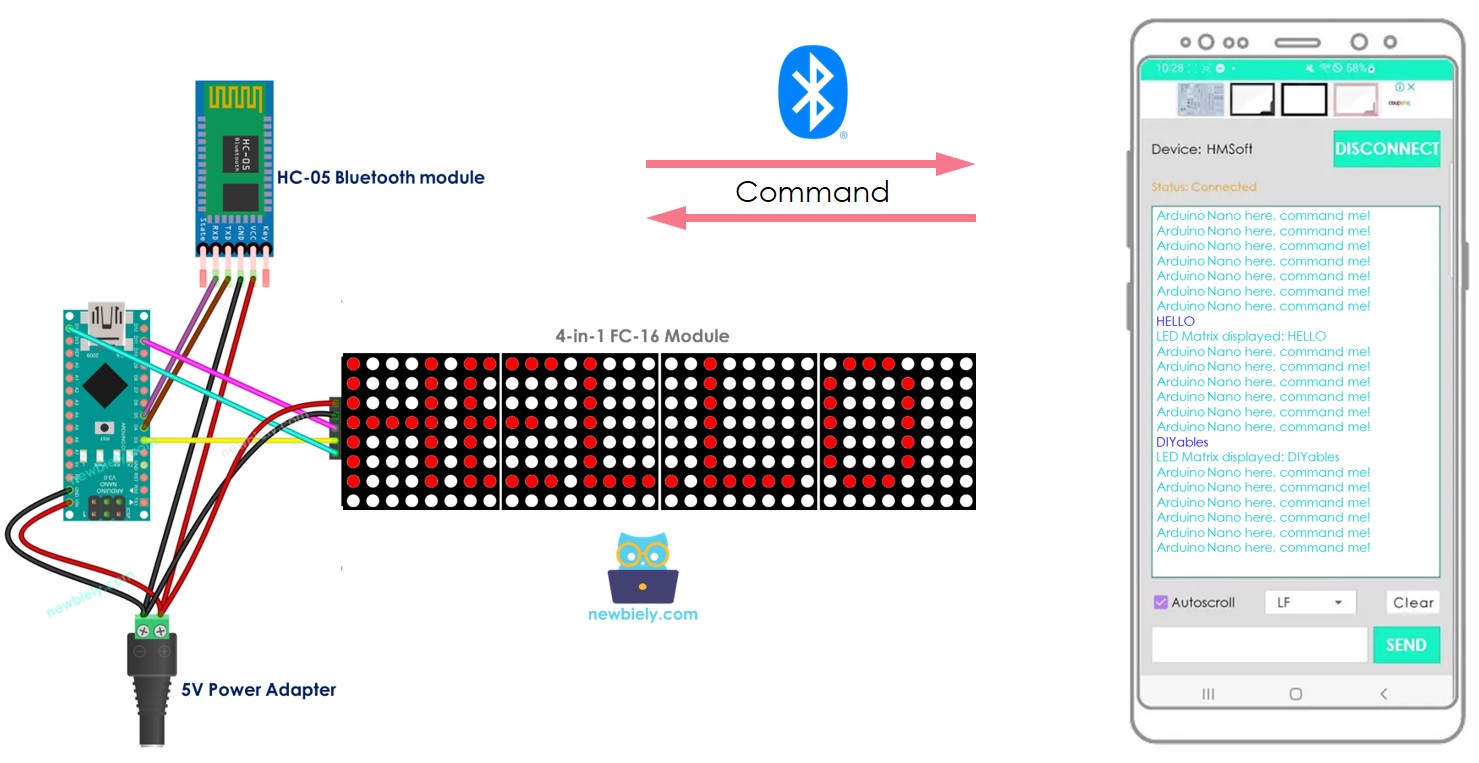
In detail, we will learn:
- How to connect the LED matrix display and HC-05 Classic Bluetooth module (Bluetooth 2.0) to Arduino Nano.
- How to connect the LED matrix display and HM-10 Bluetooth Low Engery module (BLE, Bluetooth 4.0) to Arduino Nano.
- How to program Arduino Nano to receive the text from smartphone via Bluetooth or BLE and display it on LED Matrix.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of LED matrix display and Bluetooth Module
Prior to beginning this tutorial, it is suggested that you have a general comprehension of LED matrix displays and Bluetooth modules, including their pinouts, how they operate, and how to program them. If you are not familiar with these concepts, please take a look at the following tutorials for more information:
- Arduino Nano - LED Matrix Display tutorial
- Arduino Nano - Bluetooth tutorial
- Arduino Nano - BLE tutorial
Wiring Diagram
- To control the LED matrix display via Classic Bluetooth, you can use the HC-05 Bluetooth module and connect it to Arduino Nano in accordance with the wiring diagram below:
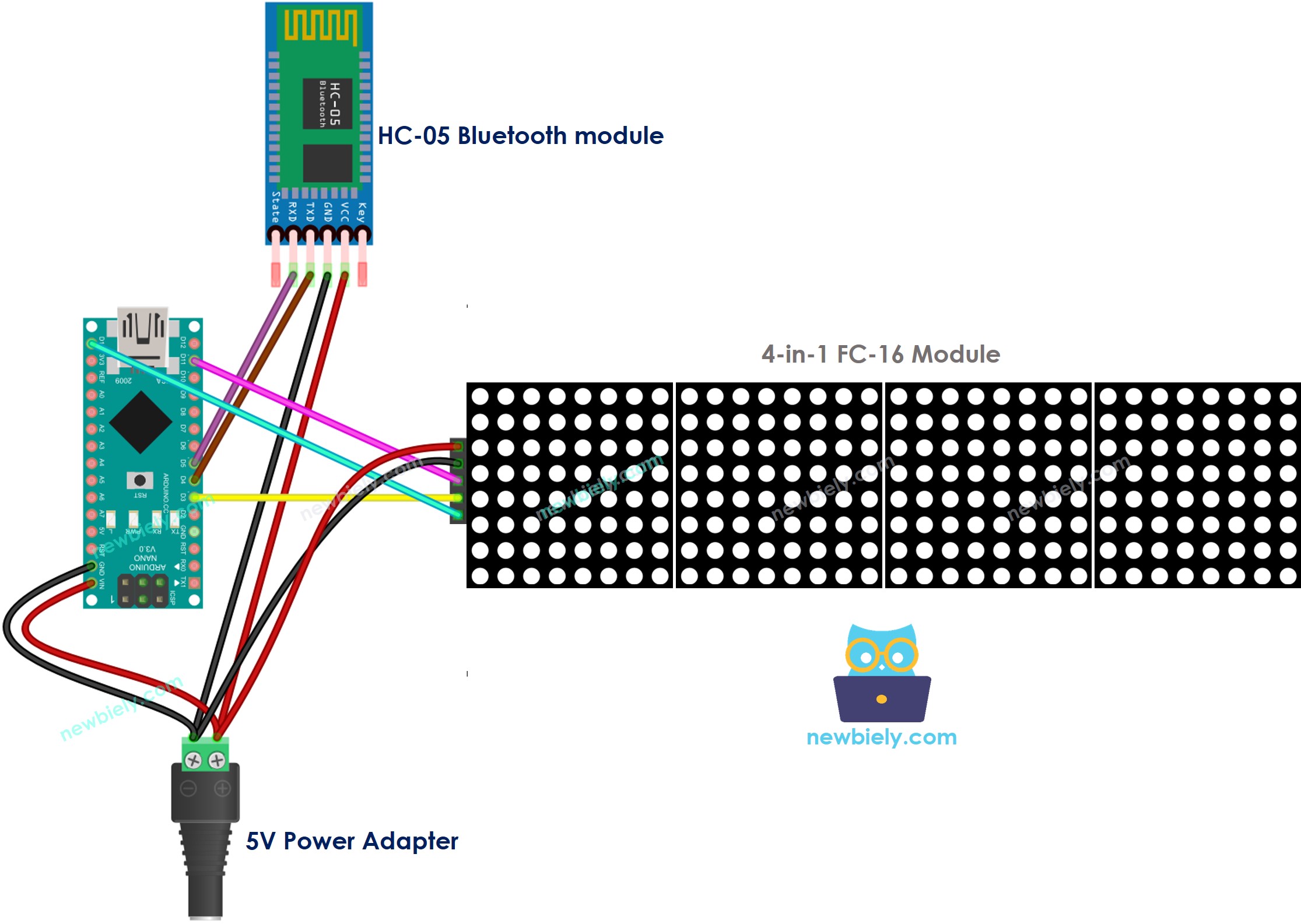
This image is created using Fritzing. Click to enlarge image
- To control the LED matrix display via BLE, the HM-10 Bluetooth module should be linked to Arduino Nano in accordance with the wiring diagram below:
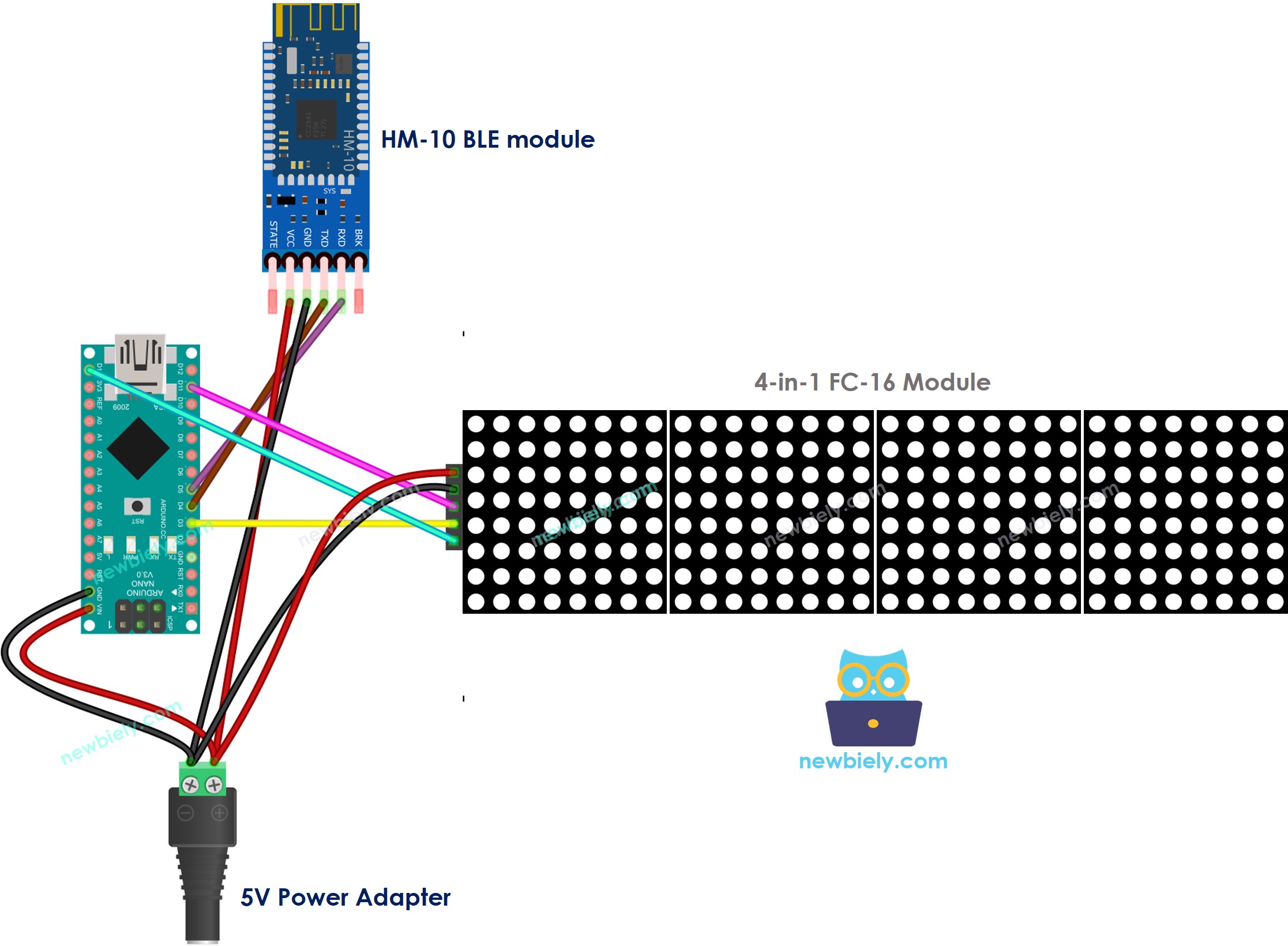
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Arduino Nano Code - controls LED matrix display via Bluetooth/BLE
The code can be used with both the HC-10 Bluetooth module and the HM-10 BLE module. No changes necessary.
Detailed Instructions
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “MD_Parola” and locate the MD_Parola library.
- Then, click the Install button.
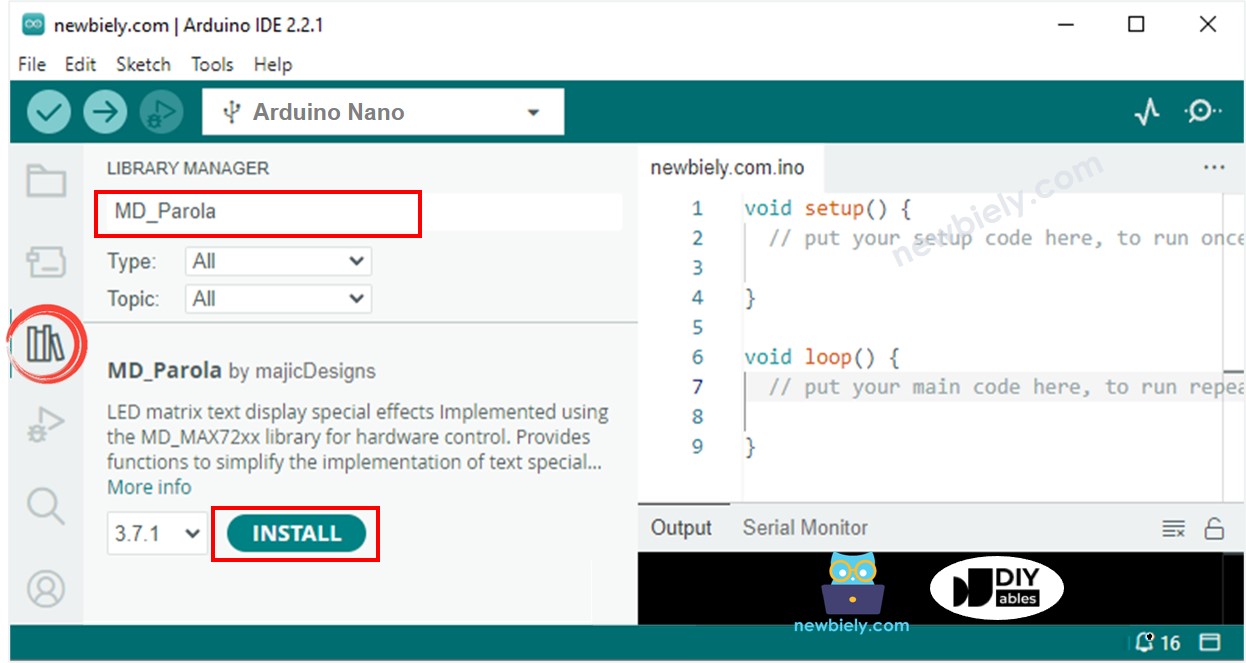
- You will be asked to install the “MD_MAX72XX” library
- Click Install All button to install the dependency.
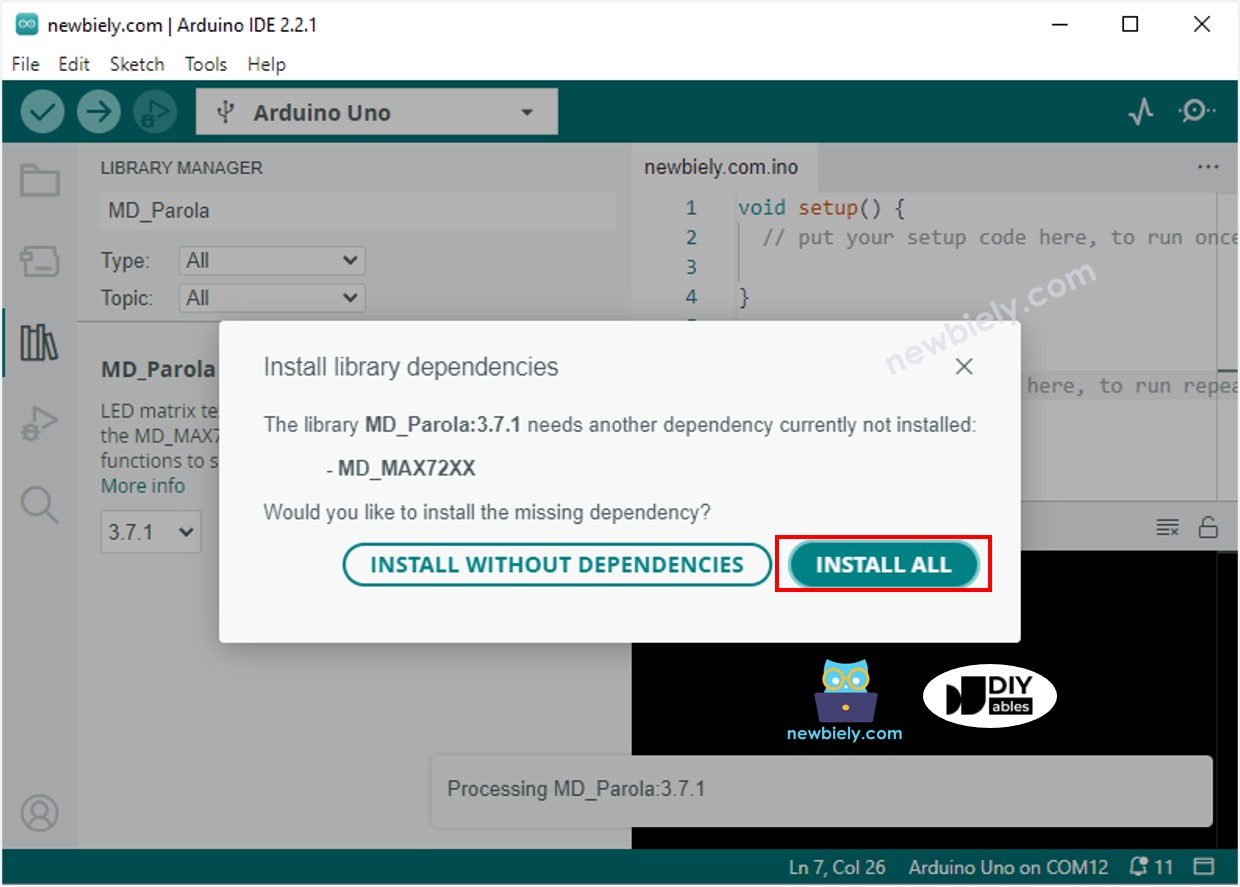
- Download the Bluetooth Serial Monitor App onto your smartphone.
- Take the code given and open it with the Arduino IDE. Upload the code to your Arduino Nano. If you have trouble uploading the code, try disconnecting the TX and RX pins from the Bluetooth module, upload the code, and then reconnect the RX/TX pins again.
- Launch the Bluetooth Serial Monitor App on your smartphone.
- Choose either Classic Bluetooth or BLE, depending on the module you are using.
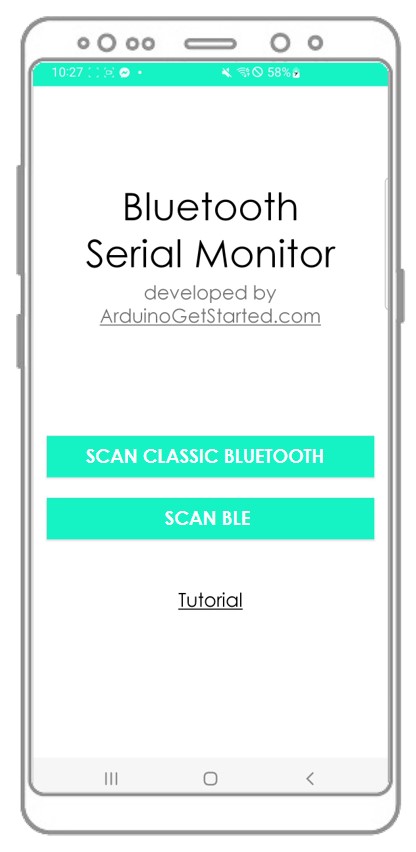
- Connect the Bluetooth App to the HC-05 Bluetooth module or the HM-10 BLE module.
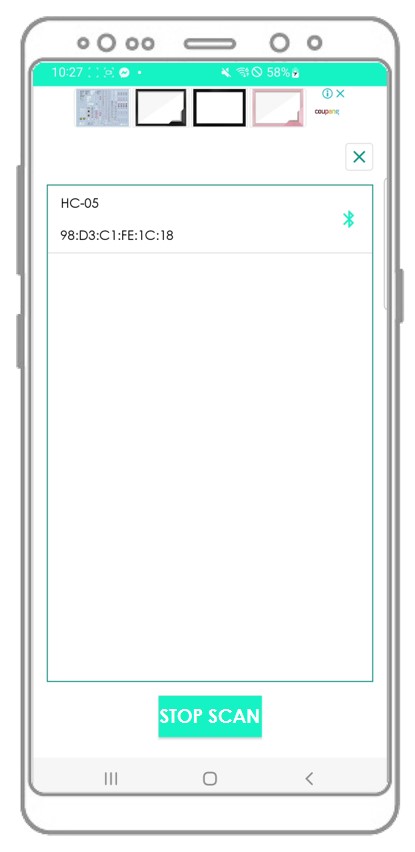
- Type a message, for instance “HELLO”, and then press the Send button to transmit it to the Arduino Nano.
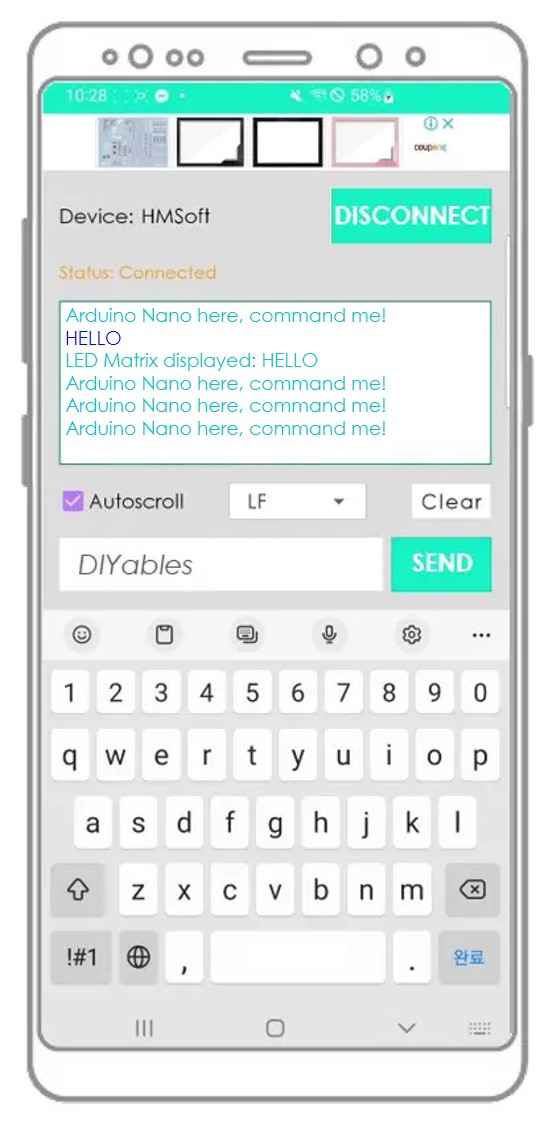
- Examine the message shown on the LED matrix display and the Bluetooth App.
- Confirm the outcome on the Android App.
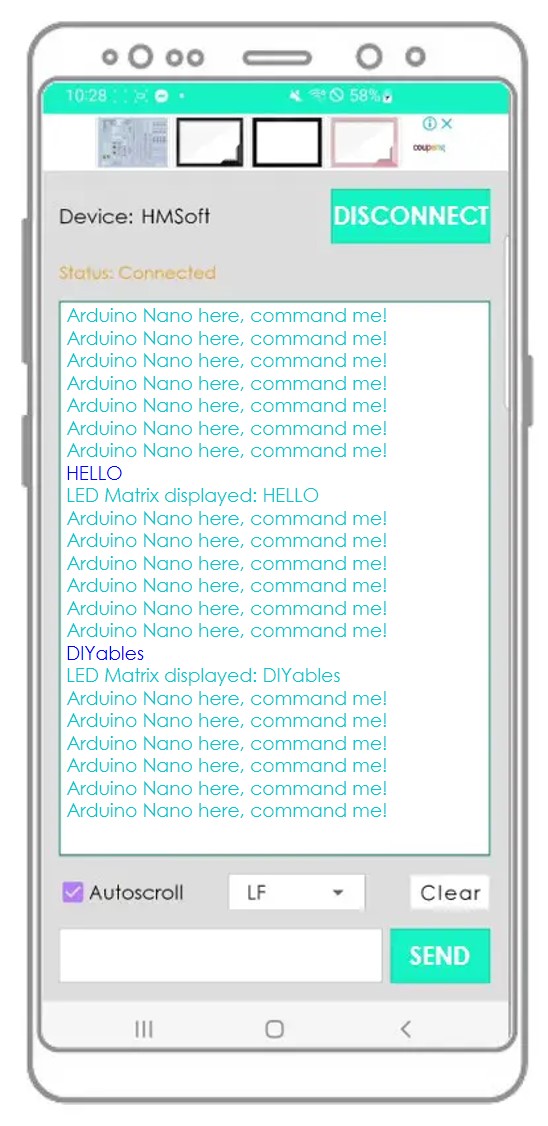
If you have found the Bluetooth Serial Monitor app to be useful, please take a moment to leave a 5-star rating on the Play Store. Your feedback would be greatly appreciated! Thank you!