Arduino Nano - Potentiometer
This tutorial instructs you how to use Arduino Nano with a potentiometer, which is also known as pot, trimmer, variable resistor, rheostat, or rotary angle sensor. In detail, we will learn:
- How potentiometer works.
- Connecting a potentiometer to Arduino Nano.
- Programming Arduino Nano to read the value from the potentiometer and convert it to a controllable value.
Hardware Preparation
1 | × | Arduino Nano | |
1 | × | USB A to Mini-B USB cable | |
1 | × | Potentiometer | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Optional) 9V Power Adapter for Arduino Nano | |
1 | × | (Recommended) Screw Terminal Adapter for Arduino Nano |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of Potentiometer
A rotary potentiometer, also known as a rotary angle sensor, is used to manually alter the value of something. Examples include the volume of a stereo, the brightness of a lamp, and the zoom level of an oscilloscope.
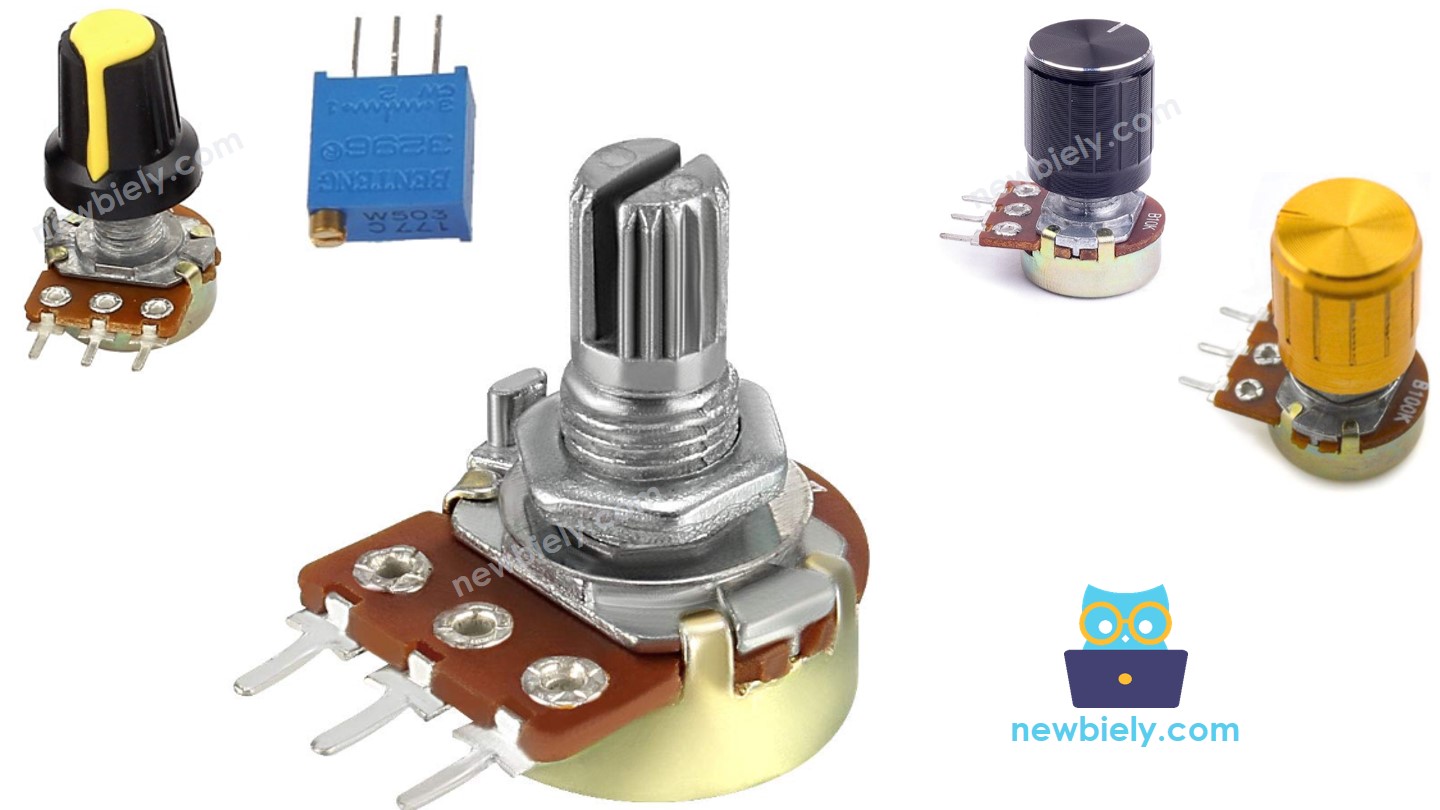
The Potentiometer Pinout
A potentiometer typically has three pins:
- The GND pin should be linked to ground (0V).
- The VCC pin should be connected to VCC (5V or 3.3v).
- The output pin provides the voltage to the Arduino's input pin.
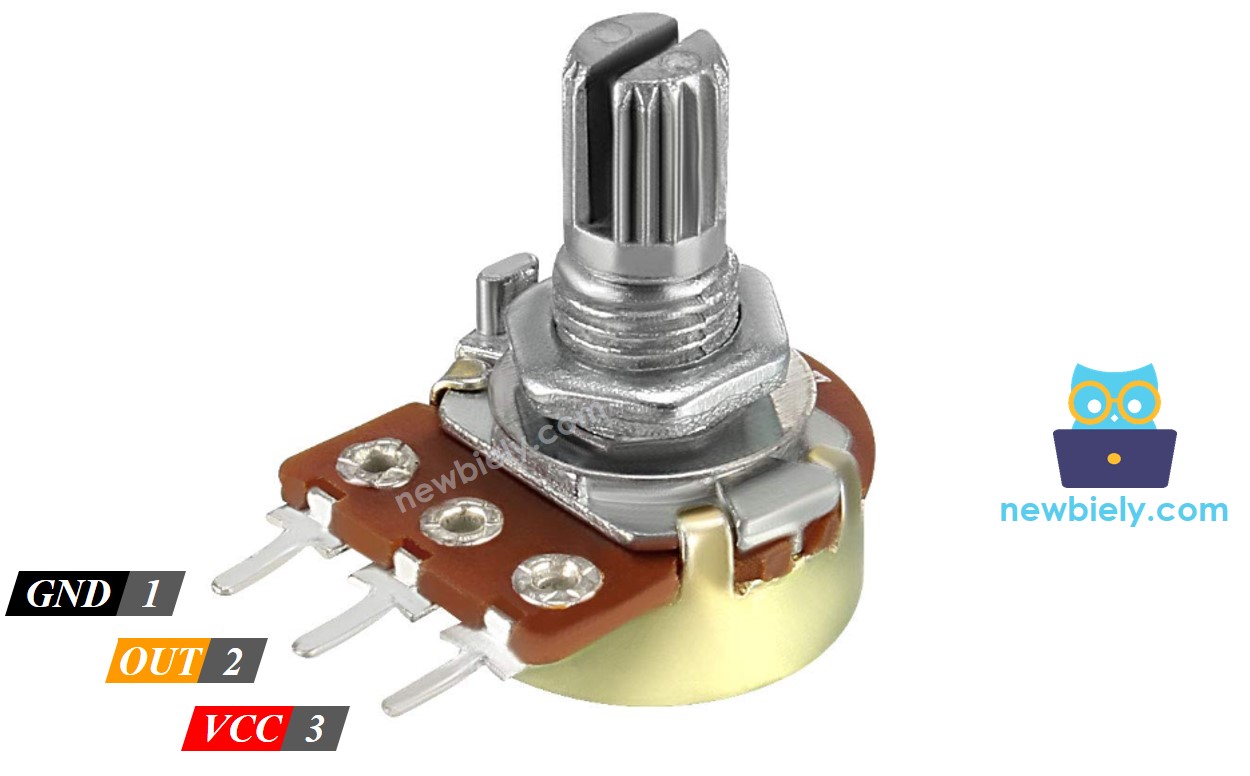
※ NOTE THAT:
The GND and VCC pins can be swapped.
How It Works
The rotatable shaft of the potentiometer has a range from 0° (closest to GND) to the maximum angle (closest to the VCC pin), referred to as ANGLE_MAX.
※ NOTE THAT:
The value of ANGLE_MAX is determined by the manufacturer. Generally, we do not need to consider this value unless we are calculating the angle of rotation (see the use cases section).
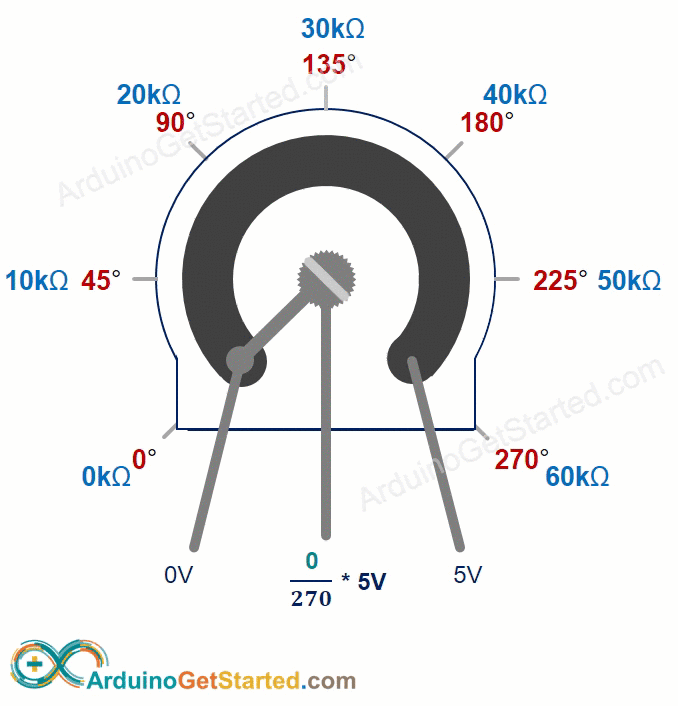
The working principle:
- An user rotates shaft of the potentiometer
- ⇒ The angle of the potentiometer is changed
- ⇒ The resistance of the potentiometer is changed
- ⇒ The voltage in the output pin of the potentiometer is changed
- ⇒ The analog value read by Arduino Nano is changed
Arduino Nano - Rotary Potentiometer
Arduino Nano's pins A0 through A7 are capable of functioning as analog inputs. These analog input pins convert the voltage (ranging from 0 volts to VCC) into integer values (from 0 to 1023), referred to as ADC value or analog value.
We can connect an output pin of the potentiometer to an analog input pin. This allows us to read the analog value from the pin and convert it to a meaningful value.
The value Arduino Nano receives is not an angle or voltage; rather, it is an integer value ranging from 0 to 1023.
Once we have obtained the integer value from the analog input pin, we can rescale it to a different value. Let us consider the applications of this.
Use Cases
- Rescale to the angle of the potentiometer.
- Rescale to the voltage of the potentiometer.
- Rescale to a controllable value, such as volume of a stereo, brightness, or speed of a DC motor - this is the most frequent use.
Rescale Range
FROM | TO | |||
---|---|---|---|---|
Angle | rotated by user | 0° | → | ANGLE_MAX |
Voltage | from potentiometer's pin | 0V | → | VCC |
ADC value | read by Arduino | 0 | → | 1023 |
Other value | converted by Arduino | VALUE_MIN | → | VALUE_MAX |
Wiring Diagram
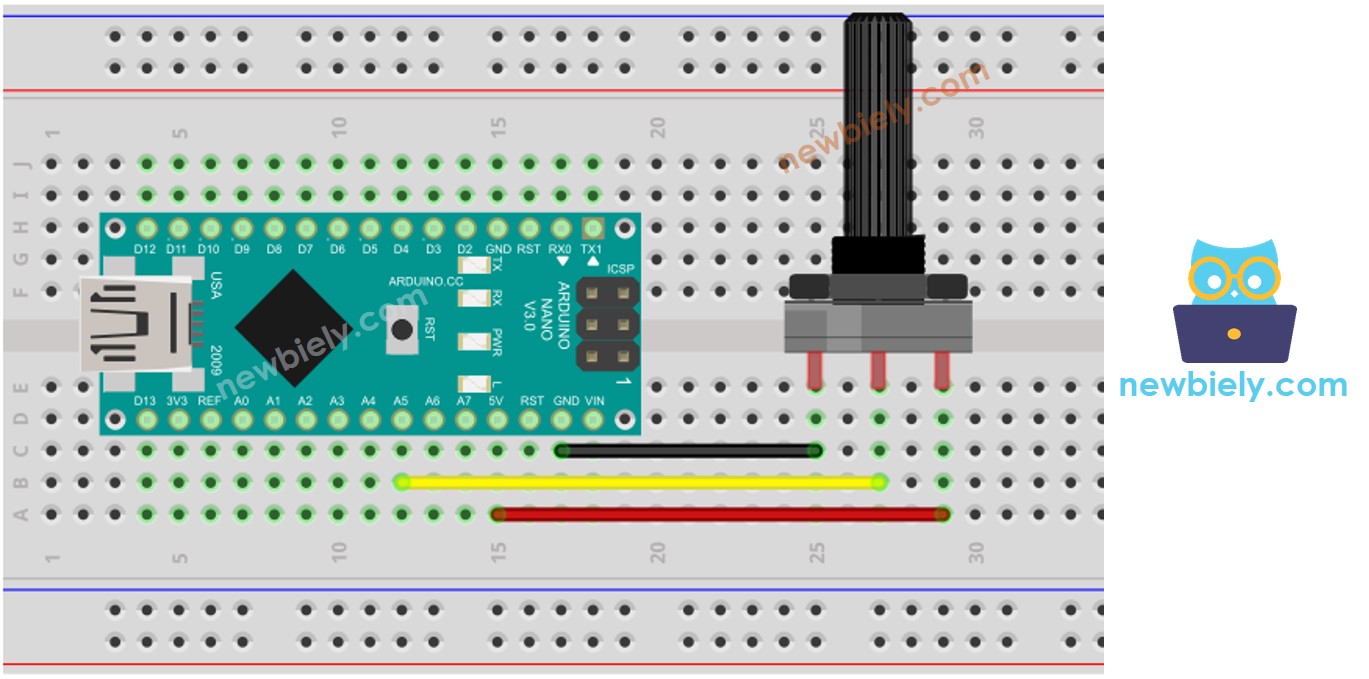
This image is created using Fritzing. Click to enlarge image
How To Program For Potentiometer
- Retrieve the value from an input pin, linked to the output pin of the potentiometer, using the analogRead() function.
- Use the map()function to adjust the potentiometer's angle.
- Adjust the voltage to the potentiometer's level.
- Rescale the value to something that can be managed or adjusted (e.g. volume of a stereo, brightness, speed of a DC motor... )
- For instance, adjusting the brightness of an LED. As stated in this tutorial, the brightness of an LED can be regulated by using a PWM value ranging from 0 (always OFF) to 255 (always ON). Thus, we can map the analog value to the brightness of the LED (from OFF to the brightest) like this:
If you desire to adjust the LED from a dim nightlight to its brightest level,
※ NOTE THAT:
The map() function is only applicable for rescaling an analog value to either the int or long type. If the controllable value is of float type, floatMap() should be used instead of map().
The floatMap() function:. It is a function that takes a list of floats as an argument and returns a new list with the same number of elements, where each element is the result of applying the given function to the corresponding element of the original list.
Arduino Nano Code
Detailed Instructions
- Copy the code and open it with the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to the Arduino Nano.
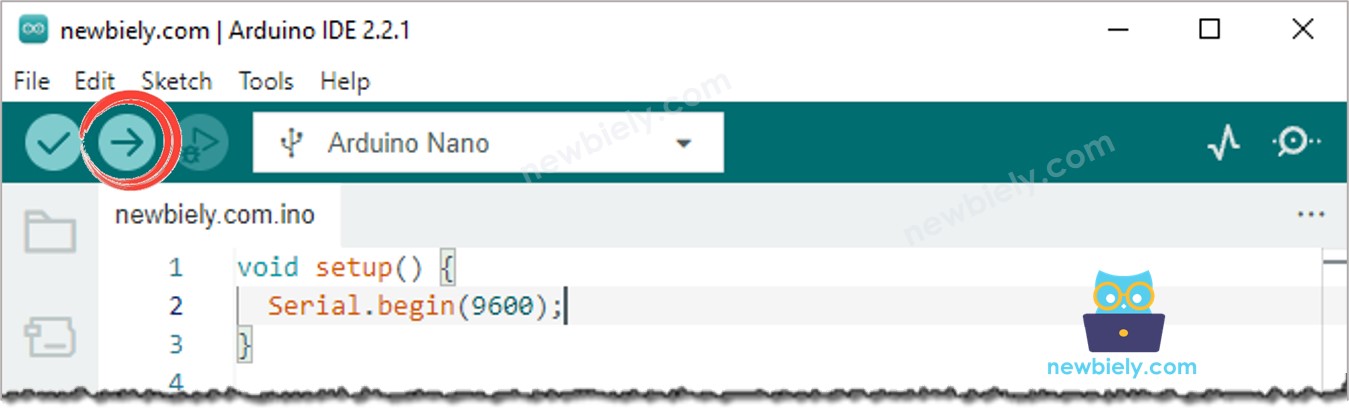
- Open the Serial Monitor.
- Turn the potentiometer.
- Check out the result on the Serial Monitor.
Video Tutorial
Challenge Yourself
Utilize the potentiometer to accomplish one of these projects:
- Manipulate the position of the servo motor. Tip: Check out Arduino Nano - Servo Motor.
- Modify the brightness of LED. Tip: Look at Arduino Nano - Fade Led.
Additional Knowledge
- GND and VCC pins can be swapped without any specific convention. However, one thing to bear in mind is that the voltage value at the output pin will be inverted when these pins are interchanged.