Arduino Nano - Solenoid Lock
This tutorial instructs you how to use Arduino Nano to control the solenoid lock, which is also referred to as the electric strike lock. We can apply this secure cabinets, drawers, and doors.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Solenoid Lock
The Solenoid Lock Pinout
The Solenoid Lock has two wires:
- The Positive (+) wire (red) must be connected to the 12V of the DC power supply
- The Negative (-) wire (black) must be connected to the GND of the DC power supply
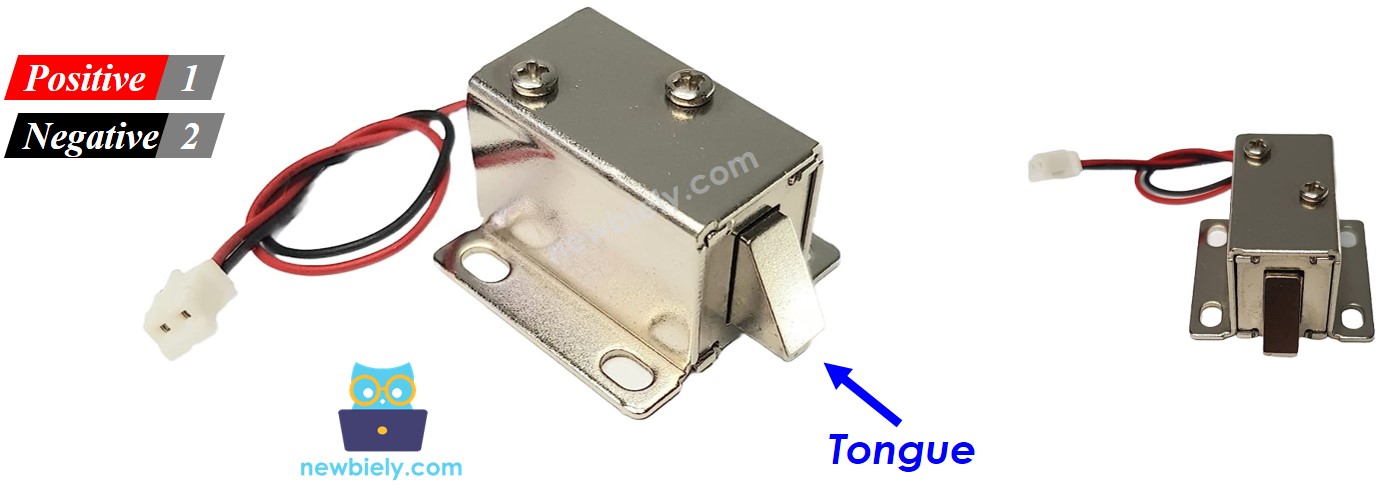
How It Works
- When the Solenoid Lock is powered, the lock tongue is extended and the door is locked.
- When the Solenoid Lock is NOT powered, the lock tongue is retracted and the door is unlocked.
※ NOTE THAT:
The solenoid lock typically requires 12V, 24V or 48V power supply. Consequently, it CANNOT be connected directly to an Arduino Nano pin. A relay must be used to connect it to the Arduino Nano pin.
If we link the solenoid lock to a relay (in the normally open mode):
- When the relay is not activated, the door is unlocked
- When the relay is activated, the door is locked
Connecting Arduino Nano to a relay allows us to program it to control the solenoid lock. To learn more about relays, please refer to the Arduino Nano - Relay tutorial.
Wiring Diagram
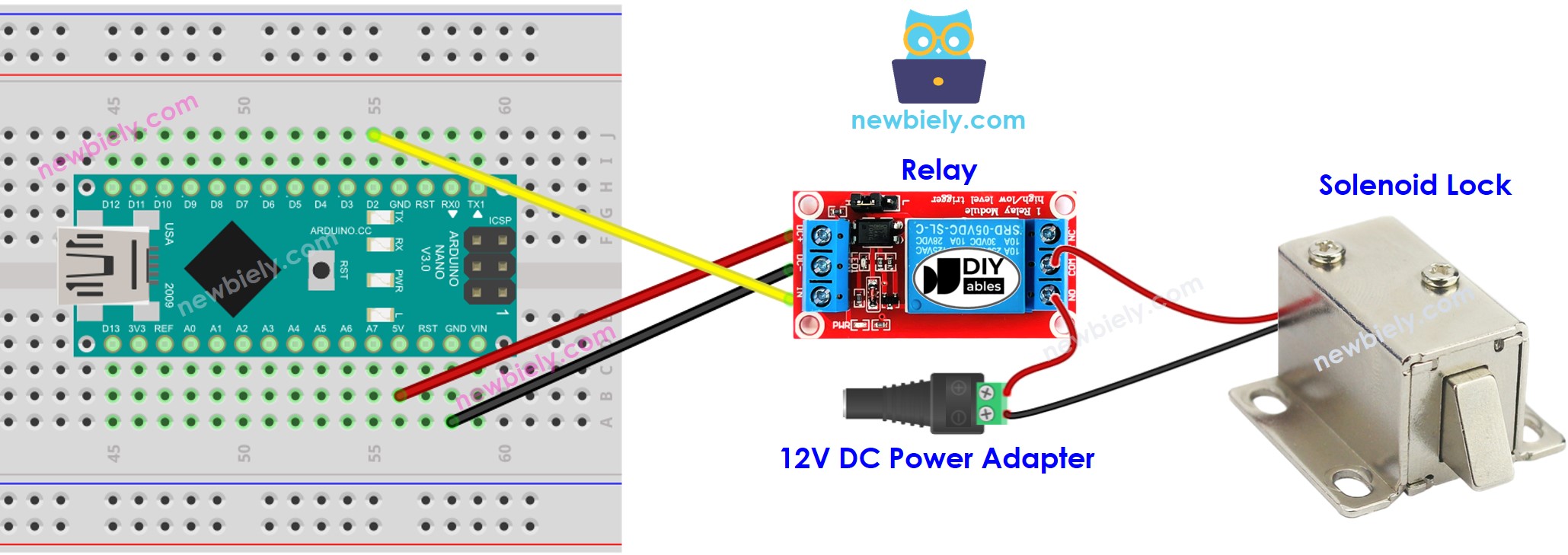
This image is created using Fritzing. Click to enlarge image
Arduino Nano Code
The code below will cause the door to be locked and unlocked every five seconds.
Detailed Instructions
- Copy the code and open it with Arduino IDE.
- Click the Upload button on Arduino IDE to compile and upload the code to Arduino Nano.
- Check out the lock tongue's condition.