Arduino Nano - Serial Plotter
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Serial Plotter
Serial Plotter is a feature of the Arduino IDE. It allows Arduino Nano to read data from temperature, humidity or any other type of sensor and transmit it to Serial Plotter. Serial Plotter will then take this data and display it as a waveform. It can even show multiple sensor readings in the same graph.
Data is exchanged between Serial Plotter and Arduino Nano through a USB cable. This same cable is used to upload code to Arduino Nano. Consequently, in order to use Serial Plotter, it is necessary to connect Arduino Nano and PC with this cable.
Serial Plotter has a selection box to pick the serial baud rate and a graph. The X-axis of the graph shows the time, with 500 points. The time between each point is equivalent to the time between two consecutive Serial.println() function calls, which is usually the same as the loop() function. The Y-axis displays the values received from Arduino Nano, and adjusts itself automatically when the value increases or decreases.
How To Open Serial Plotter
Click the Serial Plotter icon on the right side of Arduino IDE.
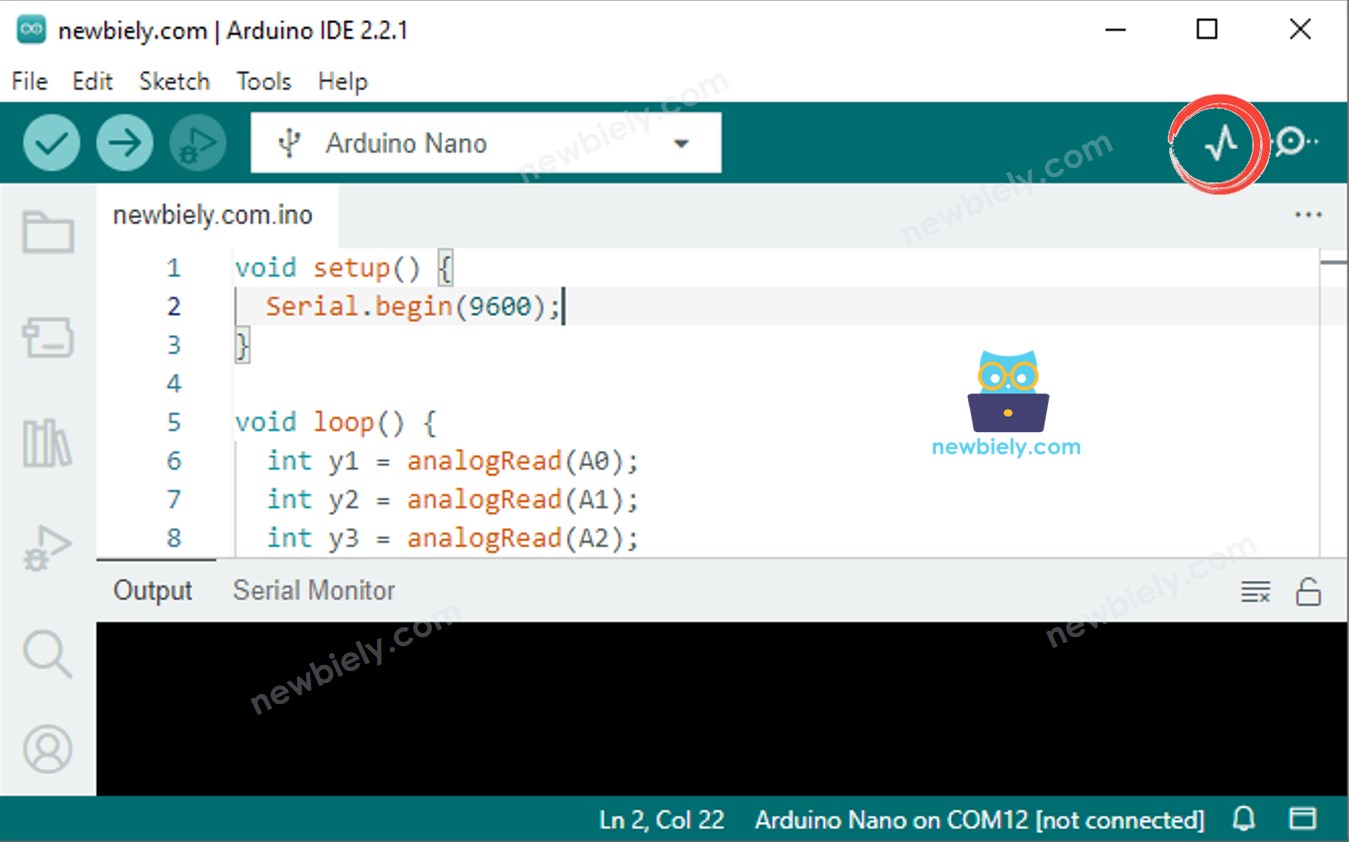
Plotting of Single Line in Graph
To generate a single graph, we need to send the data and end it with the “\r\n” character.
Specifically, we need to use the Serial.println() function.
※ NOTE THAT:
Serial.println() adds “\r\n” characters to the end of data.
Example Code
Take the value from an analog input pin and plot it on Serial Plotter. This is an example of doing so.
Detailed Instructions
- Copy the code and open it with the Arduino IDE.
- Click the Upload button on the Arduino IDE to compile and upload the code to the Arduino Nano.
- Open the Serial Plotter.
- Set the baurate to 9600.
- View the graph on the Serial Plotter.
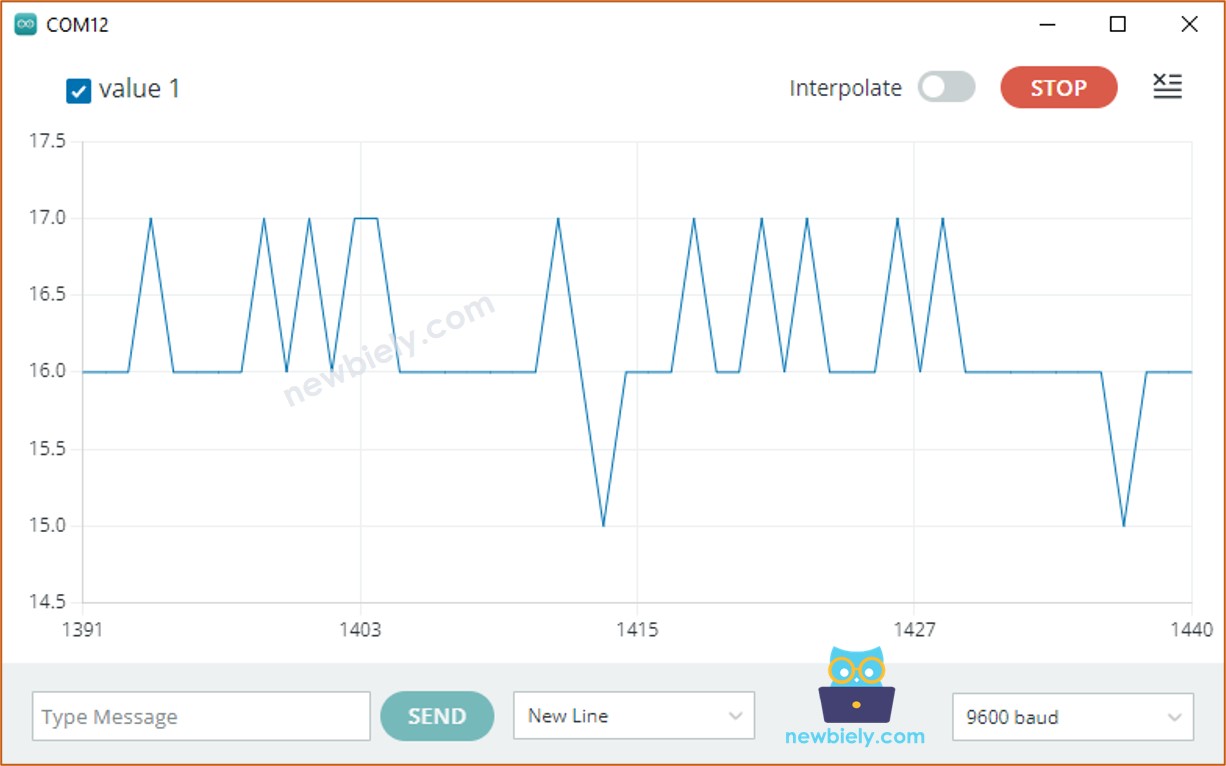
Plotting of Multiple Lines in Graph
If we wish to plot multiple variables, we must separate them from one another with either a “\t” or " " character. The final value MUST be ended with “\r\n” characters.
- Printing the first variable:
- Printing the variables in the middle:
- Printing the final variable:
Example Code
Take the value from four analog input pins and display them on the Serial Plotter.
Using Multiple Graphs:. Creating multiple graphs to display data.
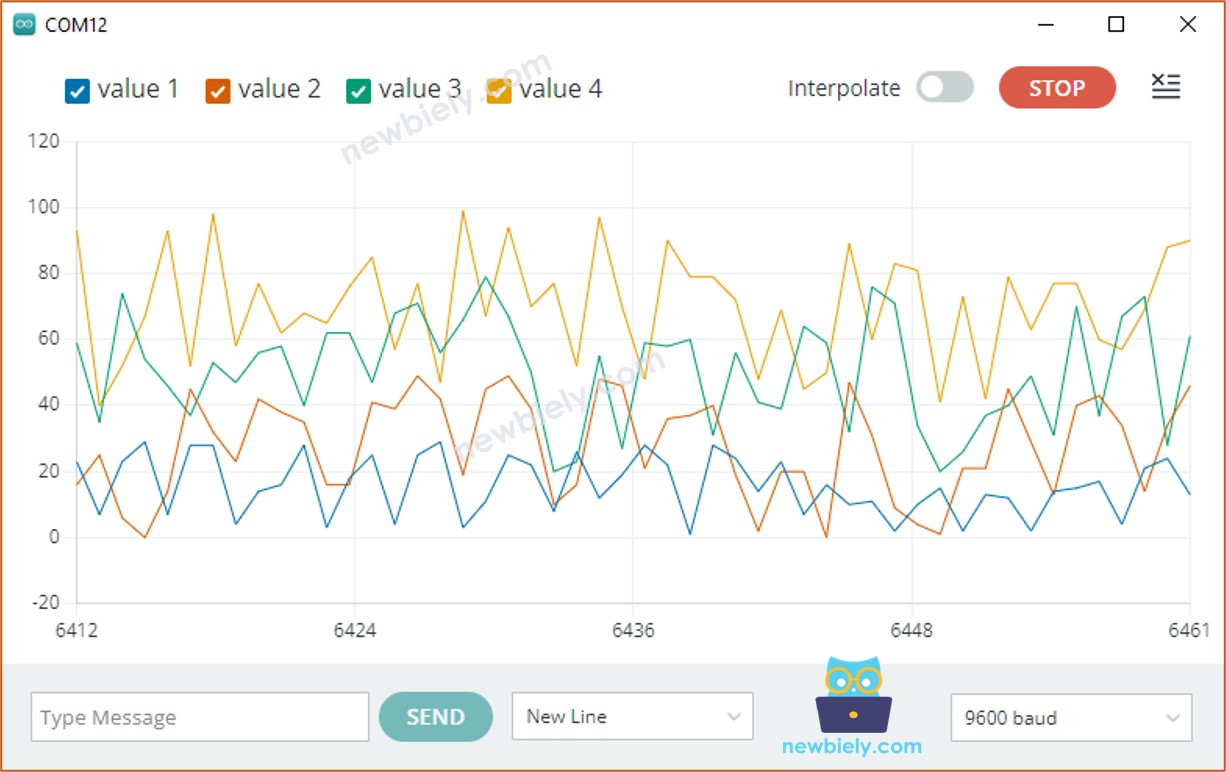
Example of 3 Sine Waveforms
Graph of Multiple Sine Waves:. This graph displays multiple sine waves.
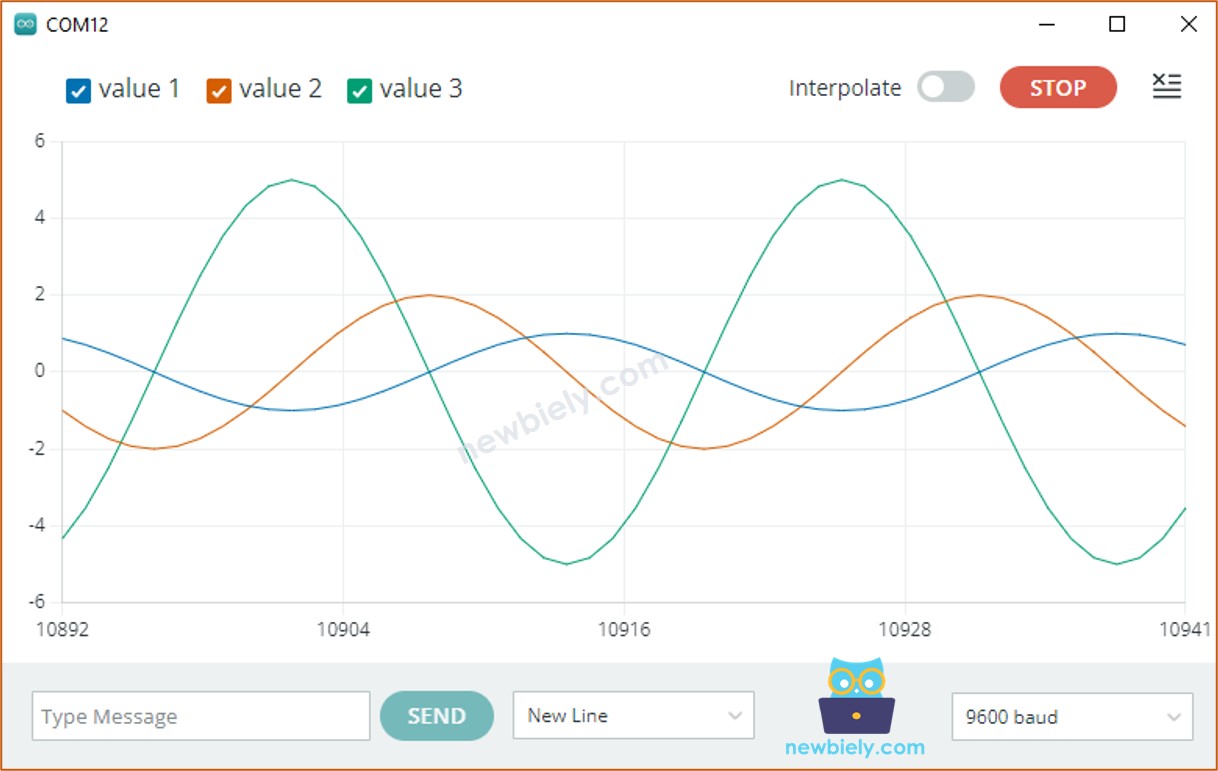