Arduino Nano - DC Motor
This tutorial instructs you how to use Arduino Nano to control DC Motor. In detail, we will learn:
- How DC motor works
- How to use Arduino Nano and L298N driver to control a DC motor
- How to program Arduino Nano to control the speed and direction of a DC motor
- How to program Arduino Nano to control two DC motors simultaneously
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of DC Motor
DC Motor Pinout
DC Motor has two wires, the positive wire is usually red and the negative wire is usually black.
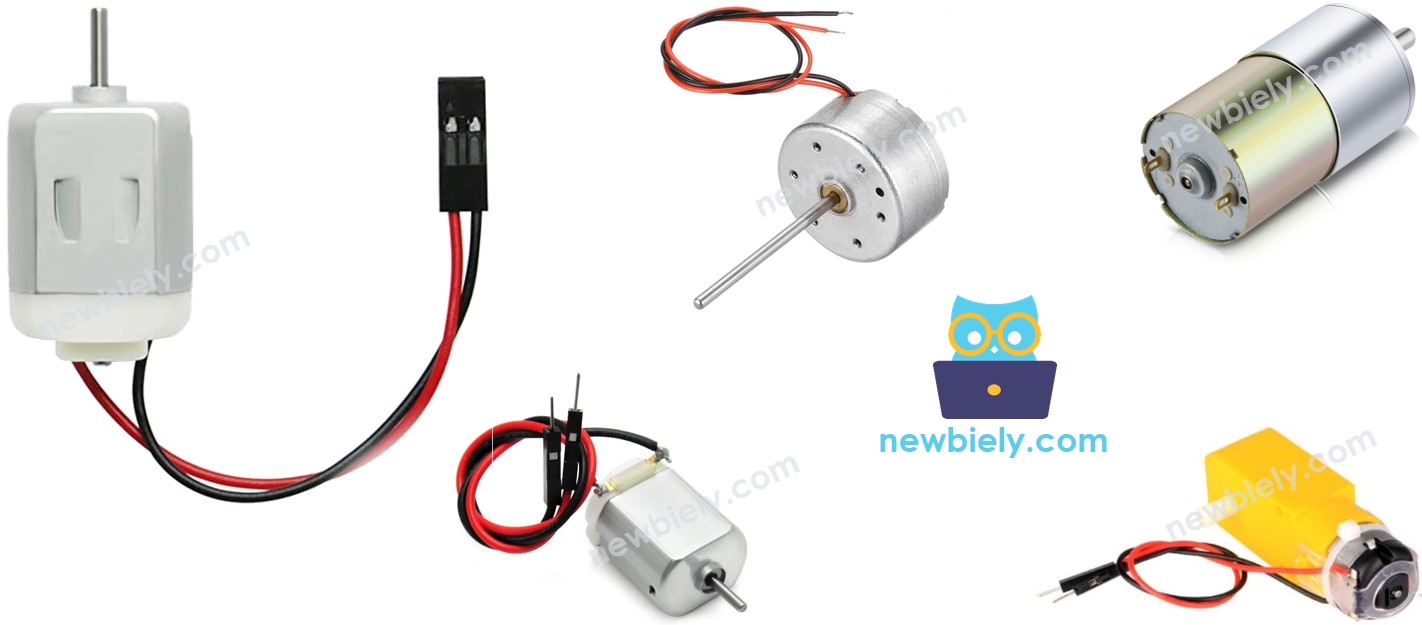
How It Works
When purchasing a DC motor, it is important to be aware of the voltage at which it operates. For instance, let's consider a 12V DC motor.
When you power the 12V DC motor by a 12V power source:
- Connecting 12V and GND to the positive wire and negative wire, respectively: the DC motor rotates at maximum speed in the clockwise direction
- Connecting 12V and GND to the negative wire and positive wire, respectively: the DC motor rotates at maximum speed in the anti-clockwise direction
Swapping the power pole between two wires of the DC motor will reverse its rotation direction. This technique is used to control the direction of the DC motor, not manually but by programming.
If the voltage of the power supply for a DC motor is lower than 12V, the motor will still rotate, but not at its maximum speed. Therefore, by adjusting the voltage of the power source, we can control the speed of the DC motor. Nevertheless, this approach is not typically used due to the difficulty in controlling the voltage of the power source. As an alternative, we keep the voltage of the power source fixed and control the speed of the DC motor through a PWM signal. The higher the duty cycle of the PWM, the faster the DC motor rotates.
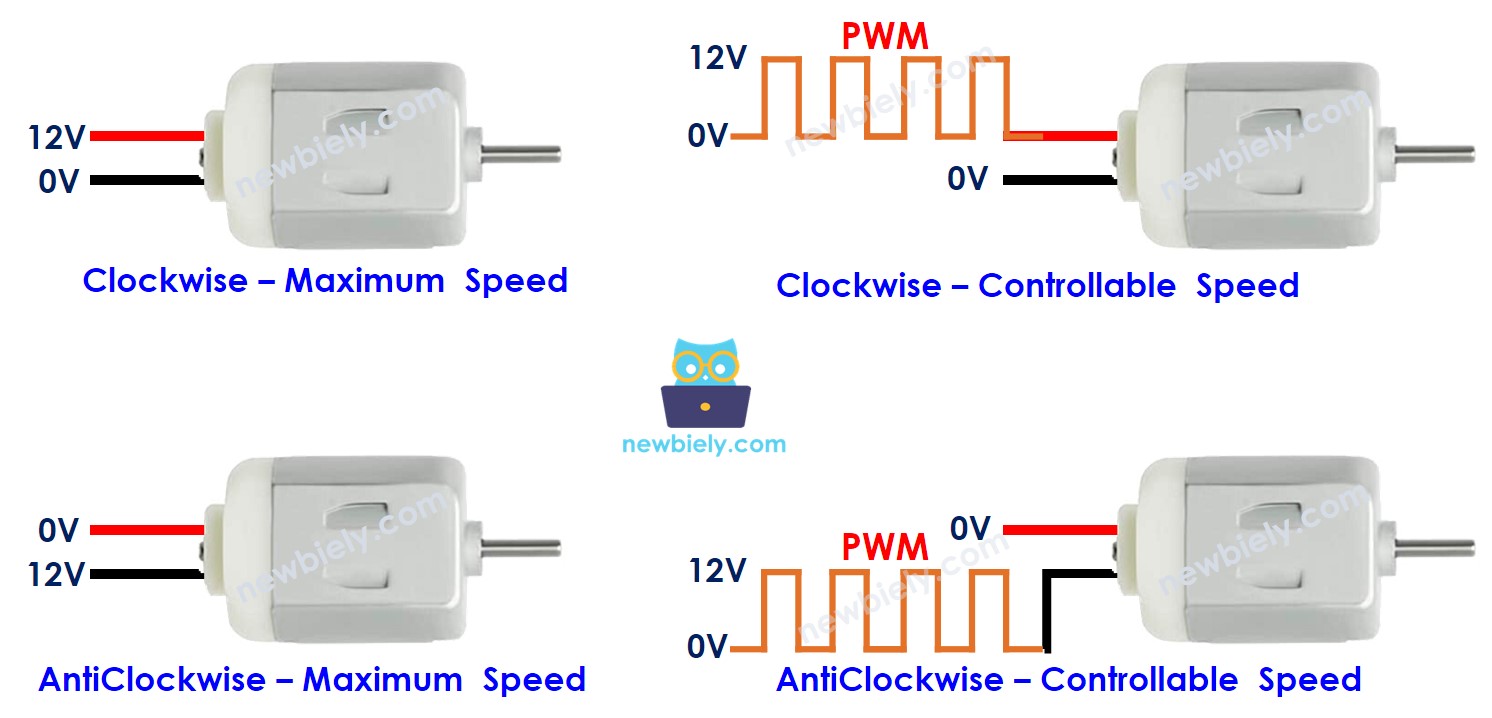
How to control DC motor using Arduino Nano
Controlling a DC motor involves two elements: speed and direction. Arduino Nano can generate a PWM signal, but this signal has low voltage and current, so it cannot be used directly to control the motor. We need to use a hardware driver between Arduino Nano and the motor. The driver performs two tasks:
- Amplifying the PWM signal from Arduino Nano (in terms of current and/or voltage) for speed control
- Receiving the control signal from Arduino Nano to switch the polarity of the power supply for direction control.
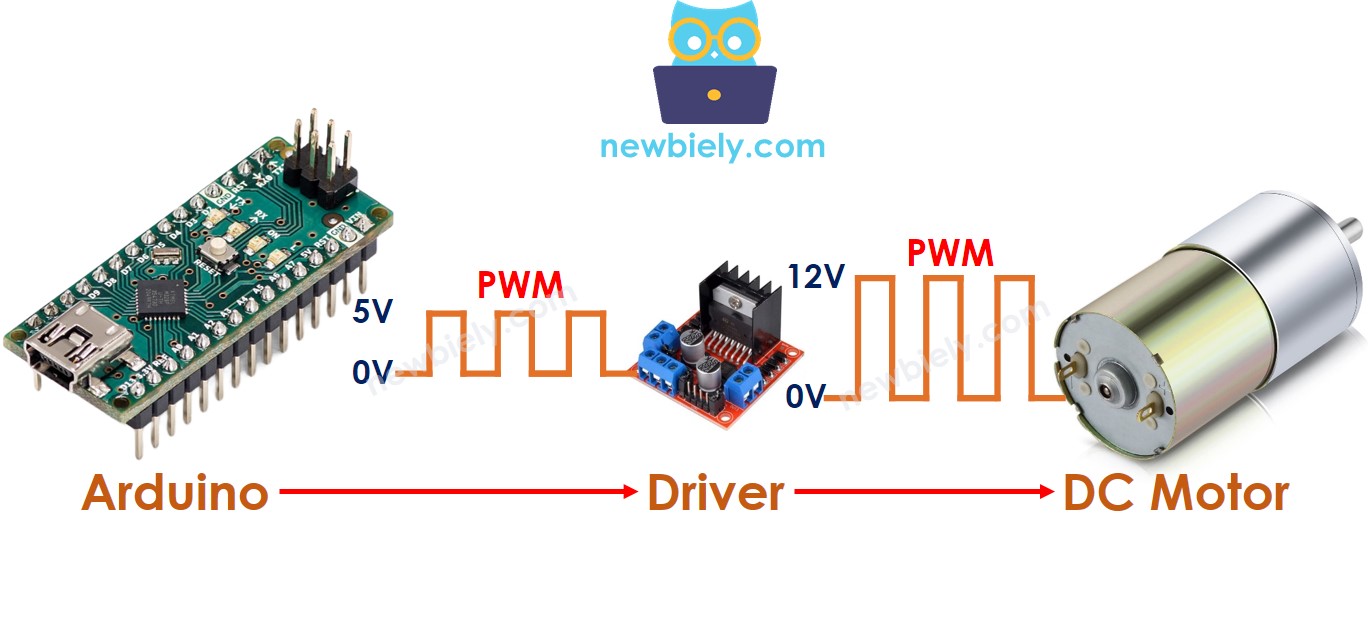
※ NOTE THAT:
- This tutorial can be used for all DC motors. We are taking 12V DC motor as an example.
- When controlling a 5V DC motor, even though the Arduino Nano pin outputs 5V (which is the same voltage as the DC motor), a driver is still required between the Arduino Nano and the DC motor as the Arduino Nano pin does not provide enough current for the DC motor.
- If you use 5V DC motor, you need a 5V power adapter for the motor. If you use 12V DC motor, you need a 12V power adapter for the motor.
There are lots of chips and modules that can work as drivers for DC motors, such as the L293D and L298N. In this guide, we'll be using the L298N driver.
Overview of L298N Driver
The L298N Driver can be used to manage DC motors and stepper motors. This tutorial instructs you how to utilize it to control a DC motor.
L298N Driver Pinout
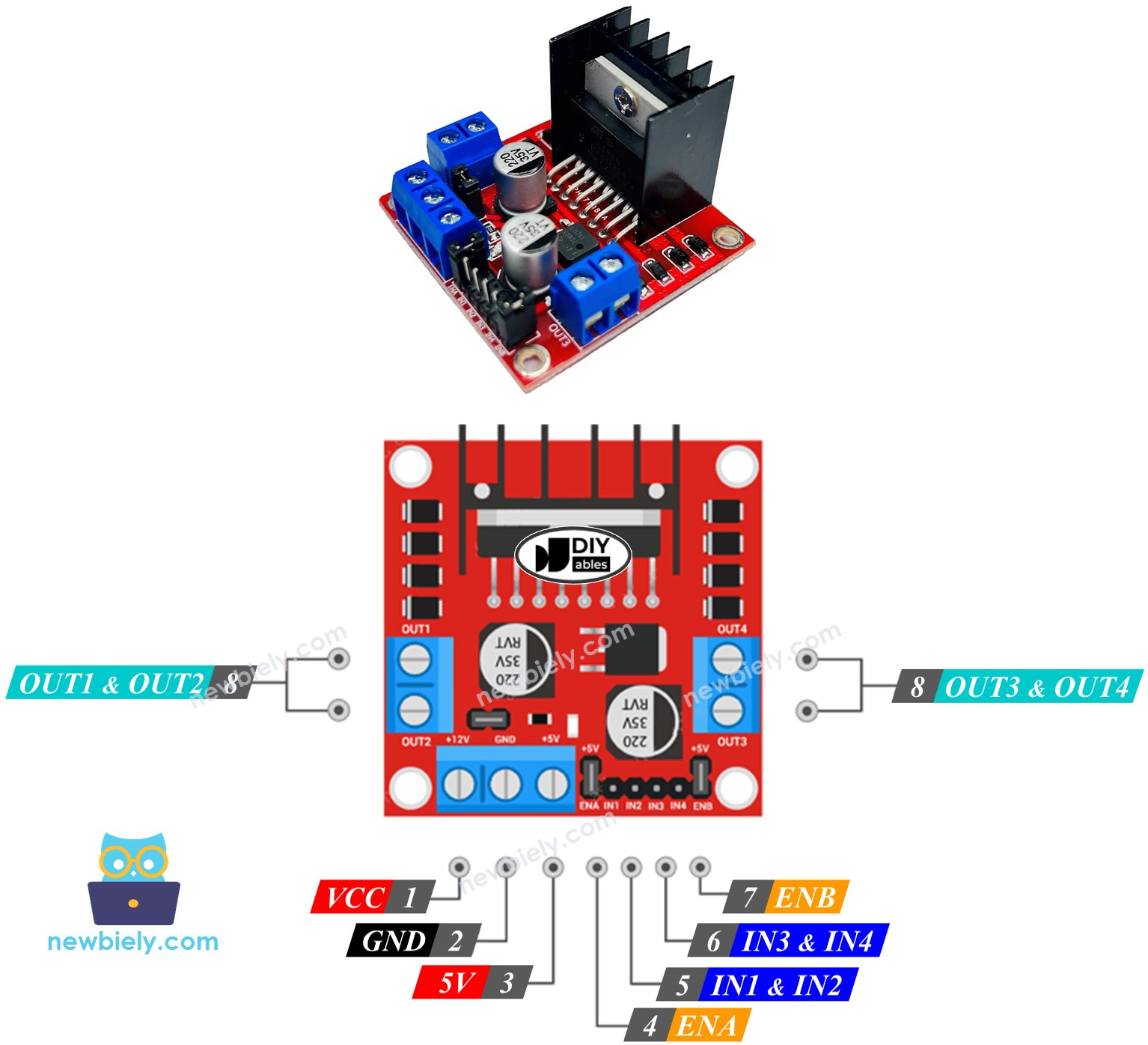
The L298N Driver is capable of controlling two DC motors independently at the same time, referred to as motor A and motor B. This driver has thirteen pins.
The common pins for both motors:
- VCC pin: This pin provides power to the motor, with a voltage range of 5 to 35V.
- GND pin: This pin is a common ground connection that must be connected to 0V (GND).
- 5V pin: This pin supplies power to the L298N module and can be supplied by a 5V source from an Arduino Nano.
Motor A pins (Channel A):
- ENA pins: These pins are used to regulate the speed of Motor A. By taking out the jumper and connecting this pin to a PWM input, it will enable us to control the speed of Motor A.
- IN1 & IN2 pins: These pins are utilized to manage the spinning direction of Motor A. When one of them is HIGH and the other is LOW, Motor A will rotate. If both the inputs are either HIGH or LOW, Motor A will cease.
- OUT1 & OUT2 pins: These pins are connected to Motor A.
Motor B pins (Channel B):
- ENB pins: This pin can be used to control the speed of Motor B. By taking out the jumper and connecting it to the PWM input, we can adjust the speed of Motor B.
- IN3 & IN4 pins: These pins are utilized to manage the spinning direction of Motor B. When one of them is HIGH while the other is LOW, Motor B will spin. If both the inputs are either HIGH or LOW, Motor B will cease to move.
- OUT3 & OUT4 pins: These pins are connected to Motor B.
The L298N driver has two input powers:
- One for the DC motor, with a voltage range of 5 to 35V (VCC and GND pins).
- One for the internal operation of the L298N module, with a voltage range of 5 to 7V (5V and GND pins).
Remove all the jumpers from the L298N driver for simplicity.
We can also control two DC motors separately simultaneously by using an Arduino Nano and an L298N Driver. To manipulate each motor, we only require three pins from Arduino Nano.
※ NOTE THAT:
The remainder of this tutorial will focus on controlling a DC motor using channel A. Similar steps should be followed to control the other DC motor.
How To Control the Speed of DC Motor via L298N Driver
It is easy to control the speed of a DC motor by producing a PWM signal to the ENA pin of L298N. To do this:
- Connect an Arduino Nano pin to the ENA of L298N
- Generate a PWM signal to the ENA pin through analogWrite() function. The L298N Driver will amplify the PWM signal to the DC motor.
The speed is a number ranging from 0 to 255. When the speed is 0, the motor will cease to move. When the speed is 255, the motor will rotate at its fastest rate.
How To Control the Direction of DC Motor via L298N Driver
The rotation of a motor can be managed by giving a logic HIGH/LOW to IN1 and IN2 pins. The table below shows how to control the direction in both channels.
IN1 pin | IN2 pin | Direction |
---|---|---|
LOW | LOW | Motor A stops |
HIGH | HIGH | Motor A stops |
HIGH | LOW | Motor A spins Clockwise |
LOW | HIGH | Motor A spins Anti-Clockwise |
Accordingly:
- Arduino code to make motor A rotate in a clockwise direction.
- Arduino code to make motor A rotate in a counter-clockwise direction.
※ NOTE THAT:
The clockwise/counter-clockwise direction of the DC motor can be reversed by connecting the OUT1 & OUT2 pins to two pins of the motor in an opposite manner.
How To Stop DC Motor Spinning
Two methods for stopping a DC motor:
- Reducing its speed to zero
- Sets the IN1 and IN2 pins to the same value, either LOW or HIGH.
- Or
How to control a DC motor using L298N driver.
Wiring Diagram
Take out all three jumpers from the L298N module before connecting the wires.
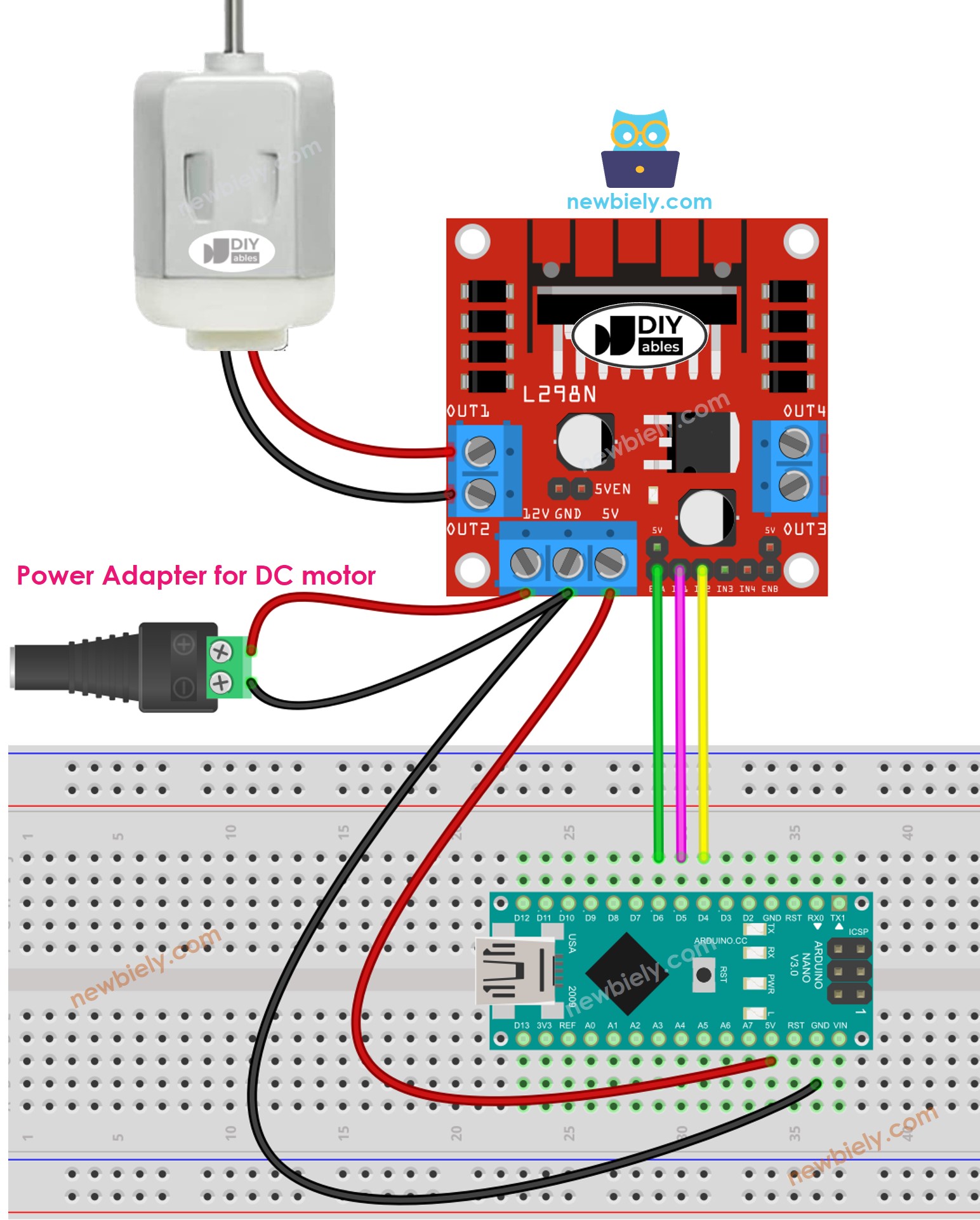
This image is created using Fritzing. Click to enlarge image
Arduino Nano Code
The following code does the followings one by one:
- Increases the speed of a DC motor
- Changes the direction
- Decreases the speed of a DC motor
- Stops the DC motor
Detailed Instructions
- Take out all three jumpers from the L298N module.
- Copy the code and open it in the Arduino IDE.
- Click the Upload button on the Arduino IDE to upload the code to the Arduino Nano.
- You should observe:
- The DC motor will speed up and then rotate at its maximum speed for 1 second.
- The direction of the DC motor will be changed.
- The DC motor will rotate at its maximum speed for 1 second in the opposite direction.
- The DC motor will slow down.
- The DC motor will stop for 1 second.
- This process will be repeated continuously.
※ NOTE THAT:
This tutorial instructs you how to adjust the speed of a DC motor relative to its maximum speed. To control the absolute speed (in revolutions per second), we will need to use a PID controller and an encoder. A separate tutorial will cover controlling the absolute speed of the DC motor.
How to Control two DC Motors using L298N Driver
Coming soon!