Arduino Nano - Stepper Motor
This tutorial instructs you how to use Arduino Nano to control Stepper Motor using L298N Driver. In detail, we will learn:
- How to use Arduino Nano and L298N driver to control a bipolar stepper motor
- How to program Arduino Nano to control the position of the stepper motor
- How to program Arduino Nano to control the speed of the stepper motor
- How to program Arduino Nano to control the direction of the stepper motor
The tutorial is applicable to all types of bipolar stepper motors with four wires. It will use a NEMA 17 stepper motor as an example.
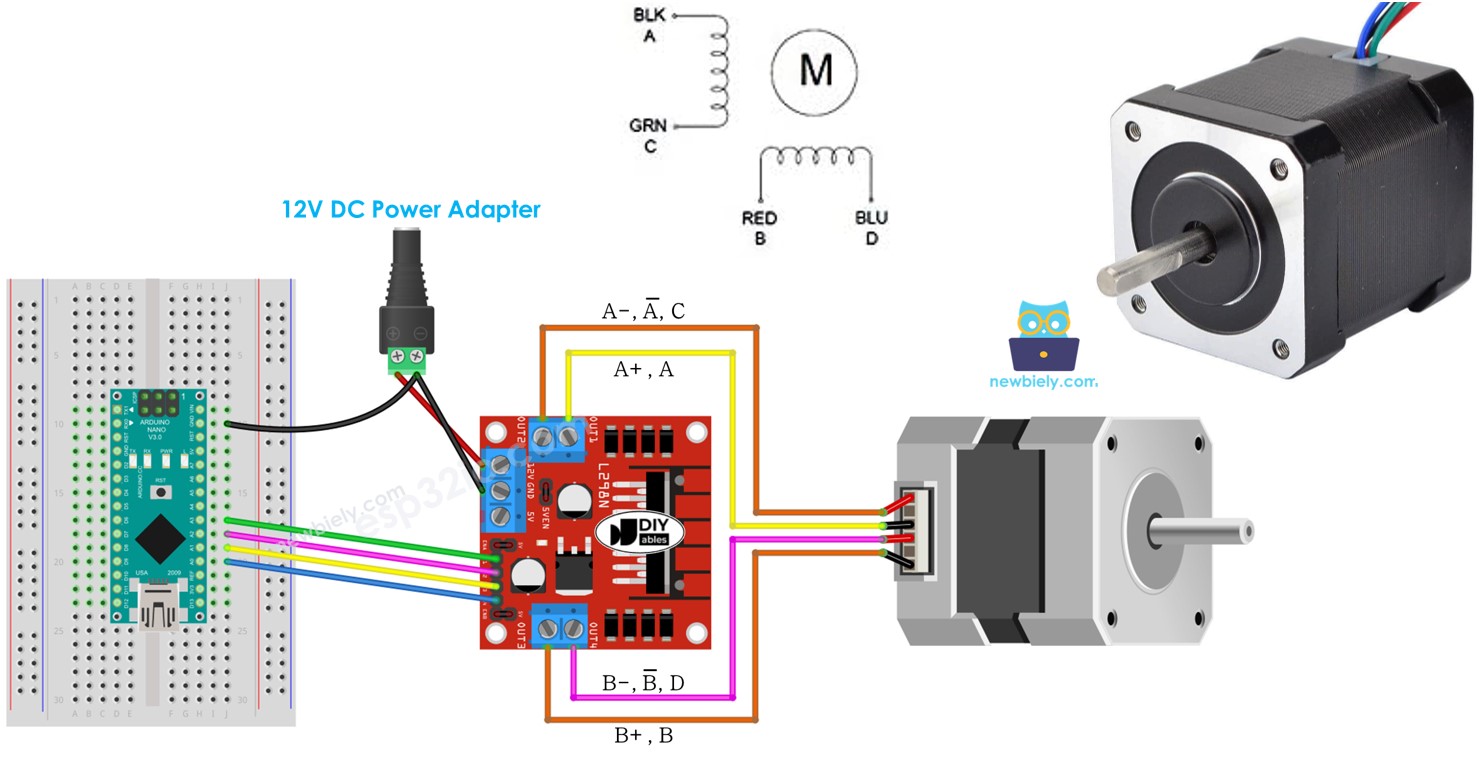
If you want control another type of stepper motor, please check out this Arduino Nano - 28BYJ-48 Stepper Motor tutorial.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Stepper Motor
Two common types of stepper motors exist:
- bipolar: this motor has 4 wires
- unipolar: this motor has either 5 or 6 wires.
For a 6-wire unipolar stepper motor, we can utilize four of the six wires and control it as if it were a bipolar stepper motor.
For a 5-wire unipolar stepper motor, check out the tutorial Arduino Nano - control 28BYJ-48 stepper motor using ULN2003 driver for more information.
The emphasis of this tutorial is solely on the bipolar stepper motor.
Bipolar Stepper Motor pinout
The bipolar Stepper Motor has four pins, and the names of these pins vary depending on the manufacturer. The following table provides some of the most commonly used names:
PIN NO | Naming 1 | Naming 2 | Naming 3 |
---|---|---|---|
1 | A+ | A | A |
2 | A- | A | C |
3 | B+ | B | B |
4 | B- | B | D |
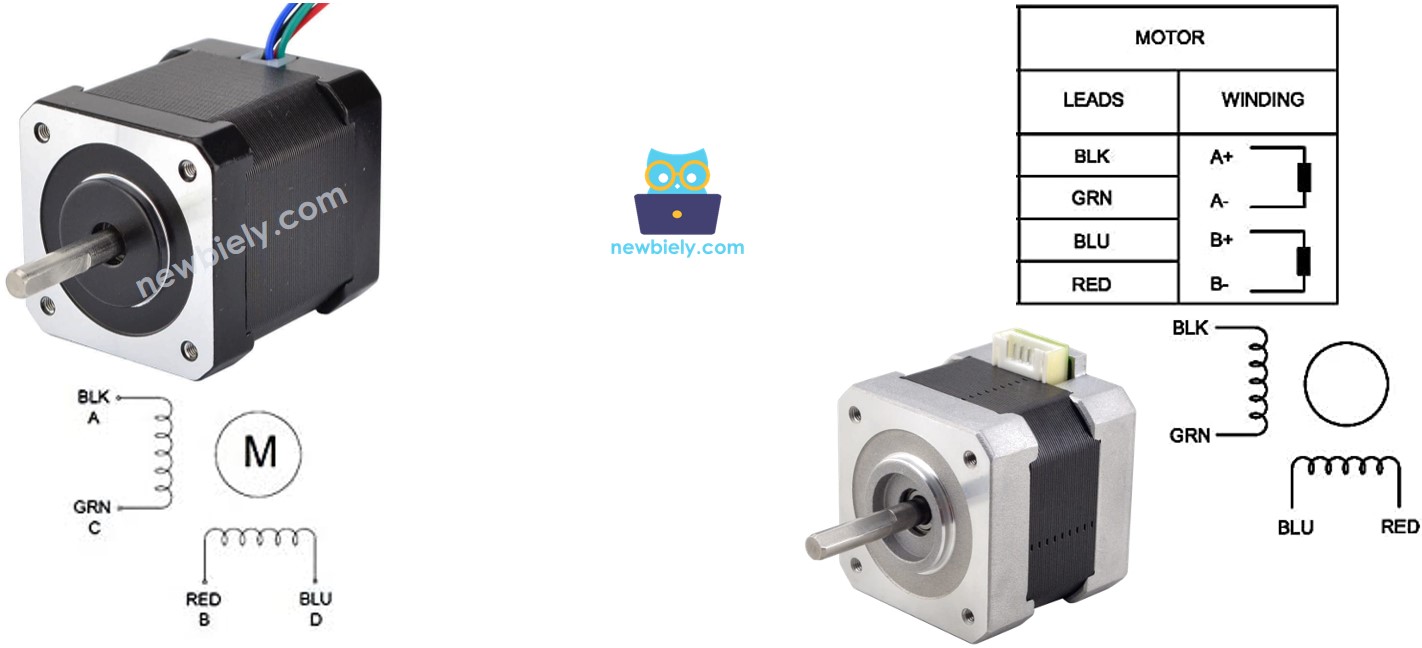
The arrangement of pins, the labels assigned to the wires, and the colors of the wires can differ among manufacturers. You must check the datasheet or manual to find out how the wire color corresponds to the pin name. The image above also displays the specifications of two distinct motors with different wire labeling and coloring.
Steps per Revolution
The specification of the motor specifies the DEG_PER_STEP value. Depending on the control technique, STEP_PER_REVOLUTION can be determined from the following table:
Control method | Steps per Revolution | Real degree per step |
---|---|---|
Full-step | STEP_PER_REVOLUTION = 360 / DEG_PER_STEP | DEG_PER_STEP |
Half-step | STEP_PER_REVOLUTION = (360 / DEG_PER_STEP) * 2 | DEG_PER_STEP / 2 |
Micro-step (1/n) | STEP_PER_REVOLUTION = (360 / DEG_PER_STEP) * n | DEG_PER_STEP / n |
For instance, if the motor's datasheet states a 1.8 degree/step measurement:
Control method | Steps per Revolution | Real degree per step |
---|---|---|
Full-step | 200 steps/revolution | 1.8° |
Half-step | 400 steps/revolution | 0.9° |
Micro-step (1/n) | (200 * n) steps/revolution | (1.8 / n)° |
How to control a stepper motor using Arduino Nano
Arduino Nano can generate signals to control the stepper motor, however these signals do not have the sufficient voltage and/or current that the stepper motor requires. Therefore, a hardware driver is needed between Arduino Nano and the stepper motor. This driver has two functions:
- To amplify the control signals from Arduino Nano in terms of current and voltage
- To protect Arduino Nano from the high current and voltage used to power the stepper motor.
There are numerous types of hardware drivers that can be used to manage stepper motors. One of the most popular hardware drivers for controlling stepper motors is the L298N Driver.
Overview of L298N Driver
A L298N Driver can be utilized to control two DC motors or a stepper motor. This tutorial instructs you how to use it to control the stepper motor.
L298N Driver Pinout
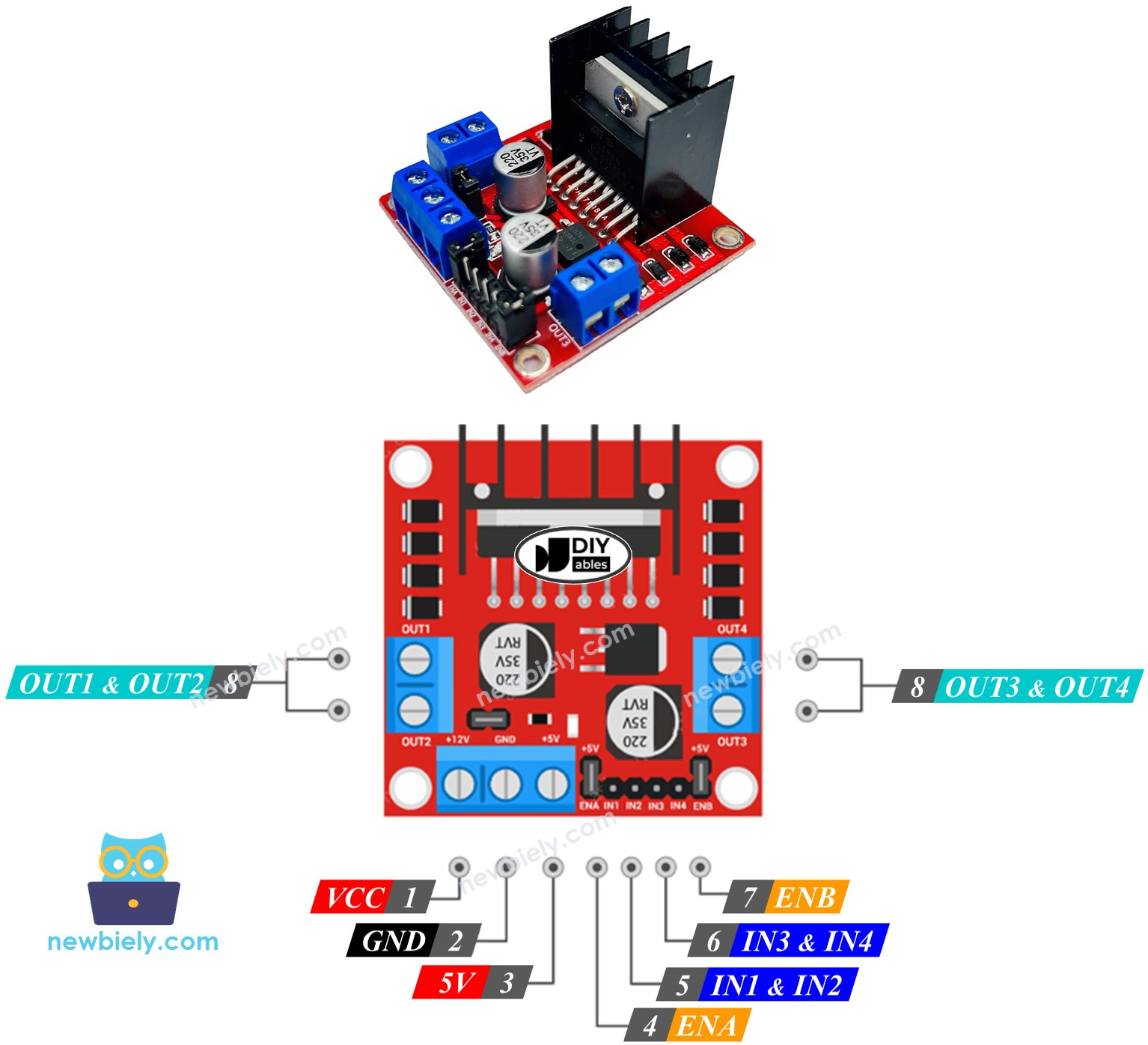
The L298N Driver has 11 pins and three jumpers:
- VCC pin: This supplies power for the motor and can be anywhere between 5 to 35V.
- GND pin: This is a common ground pin and needs to be connected to GND (0V).
- 5V pin: This supplies power for the L298N module and can be supplied by 5V from Arduino Nano.
- IN1, IN2, IN3, IN4 pins: These are connected to Arduino's pins to receive the control signal to control the stepper motor.
- OUT1, OUT2, OUT3, OUT4 pins: These are connected to the stepper motor.
- ENA, ENB jumpers: These are used to enable the stepper motor and both the ENA & ENB jumpers need to be in place.
- 5V-EN jumper: If this is kept in place, the power for the L298N module is got from VCC and nothing needs to be connected to the 5V pin. If it is removed, power needs to be supplied to the L298N module via a 5V pin.
The L298N driver has two input powers:
- One for the stepper motor (VCC and GND pins): ranging from 5 to 35V.
- One for the internal operation of the L298N module (5V and GND pins): ranging from 5 to 7V. If the 5V-EN jumper is kept in place, no connection to this pin is necessary.
Wiring Diagram
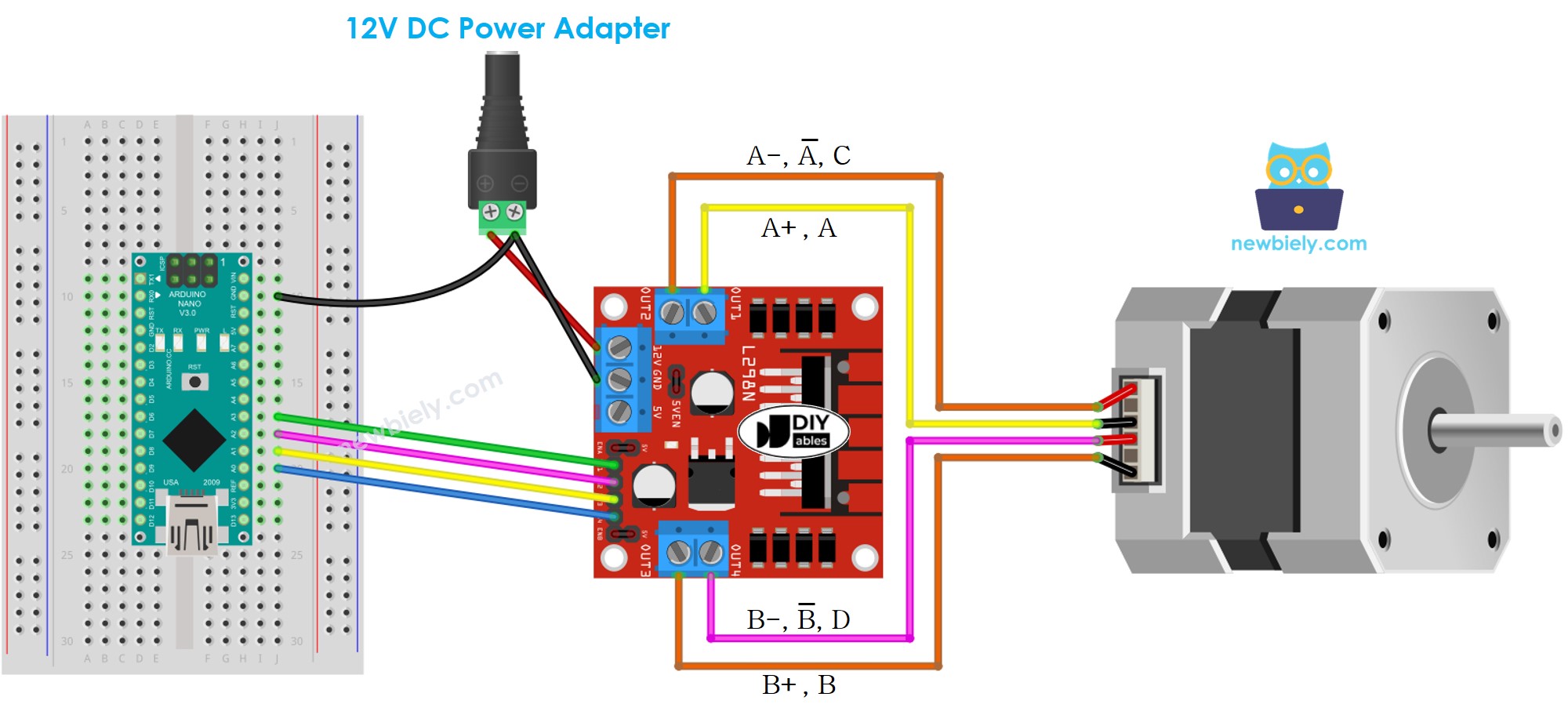
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
※ NOTE THAT:
- Keep the three jumpers on the L298N module in their current positions if the motor's power supply is 12V or lower.
- The pin arrangement on stepper motors can differ between manufacturers. Please refer to the table below for the correct wiring.
Wiring table between Arduino Nano and L298N Driver
Arduino Nano pins | L298N pins |
---|---|
7 | IN1 |
6 | IN2 |
5 | IN3 |
4 | IN4 |
Wiring table between L298N Driver and Stepper motor
Important!: Do not pay attention to the order of the wires in the stepper motor in the diagram above. It is just an illustration. The arrangement of the pins on stepper motors may differ between manufacturers. Make sure that your wiring follows the table below.
L298N pins | Stepper motor pins | Or | Or |
---|---|---|---|
OUT1 | A+ | A | A |
OUT2 | A- | A | C |
OUT3 | B+ | B | B |
OUT4 | B- | B | D |
Before purchasing a stepper motor, it is recommended to review the datasheet, specification, or manual of the stepper motor. Ensure that the information contains the mapping between the pin's color and name. For instance, this stepper motor supplies the mapping as seen in the following image:
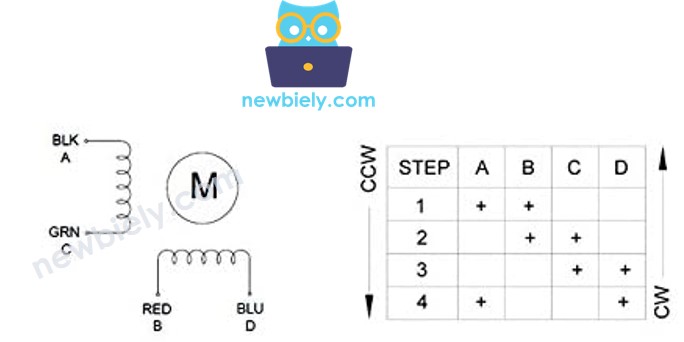
Using the mapping, the wiring table is:
L298N pins | stepper motor pins | wire color |
---|---|---|
OUT1 | A | black wire |
OUT2 | C | green wire |
OUT3 | B | red wire |
OUT4 | D | blue wire |
※ NOTE THAT:
In all the wiring tables presented above between the stepper motor and L298N Driver, it is possible to interchange OUT1 with OUT2, and OUT3 with OUT4. Consequently, there are more ways to do the wiring. Nevertheless, if the swapping is done, the rotation direction of the motors may be altered (from clockwise to anticlockwise, and vice versa).
How to control a Stepper motor using an L298N driver.
Controlling a stepper motor can be a challenging endeavor, particularly when we need to do so without blocking. Fortunately, the AccelStepper library makes it a breeze.
The Arduino IDE contains an integrated Stepper library. Nevertheless, we do not suggest you utilize this library due to the following reasons:
- The library offers a blocking feature. This implies that Arduino Nano is prevented from executing other tasks while it is controlling the stepper motor.
- It does not possess adequate functions.
Instead, we suggest using the AccelStepper library. This library offers:
- Acceleration
- Deceleration
- Full-step and half-step driving
- The capacity to control multiple steppers simultaneously, with individual concurrent stepping for each stepper
- Drawback: Does NOT provide micro-step driving.
How To Control the Position of Stepper Motor via L298N Driver
We can achieve our goal of moving the stepper motor to the desired position by utilizing:
※ NOTE THAT:
The stepper.moveTo() function is non-blocking, which is a great advantage of the library. However, when using this function, there are some points to pay attention to:
- Make sure to call 'stepper.run()' as often as possible, preferably in the void loop() function.
- Avoid using the delay() function while the motor is moving.
- Do not use Serial.print() and Serial.println() functions while the motor is running, as these will slow down the stepper motor.
How To Control the Speed of Stepper Motor via L298N Driver
We can regulate not only the speed, but also the acceleration and deceleration, through the use of some basic functions.
How To Control the Direction of Stepper Motor via L298N Driver
If you wire the motor as indicated, it will rotate in:
- Clockwise direction: when controlling the motor from a lower to a higher position (Position increment)
- Anticlockwise direction: when controlling the motor from a higher to a lower position (Position decrement)
For examples:
- When the current position is 100 and the motor is directed to 200, it rotates in a clockwise direction
- If the current position is -200 and the motor is controlled to -100, it rotates in a clockwise direction
- If the current position is 200 and the motor is regulated to 100, it rotates in an anticlockwise direction
- If the current position is -100 and the motor is managed to -200, it rotates in an anticlockwise direction
※ NOTE THAT:
As previously stated, if you exchange OUT1 with OUT2, or OUT3 with OUT4, the increase of the position could be counterclockwise and the decrease of the position could be clockwise.
How To Stop Stepper Motor
- The stepper motor will halt when it reaches the desired position.
- It is also possible to make it stop immediately by using the stepper.stop() function.
Arduino Nano Code - Stepper Motor Code
The following code:
- Makes the motor rotate one revolution in a clockwise direction
- Pauses the motor for 5 seconds
- Causes the motor to rotate one revolution in an anticlockwise direction
- Halts the motor for 5 seconds
- Repeats the process over and over
Detailed Instructions
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “AccelStepper” and locate the AccelStepper library created by Mike McCauley.
- Press the Install button to complete the installation of the AccelStepper library.
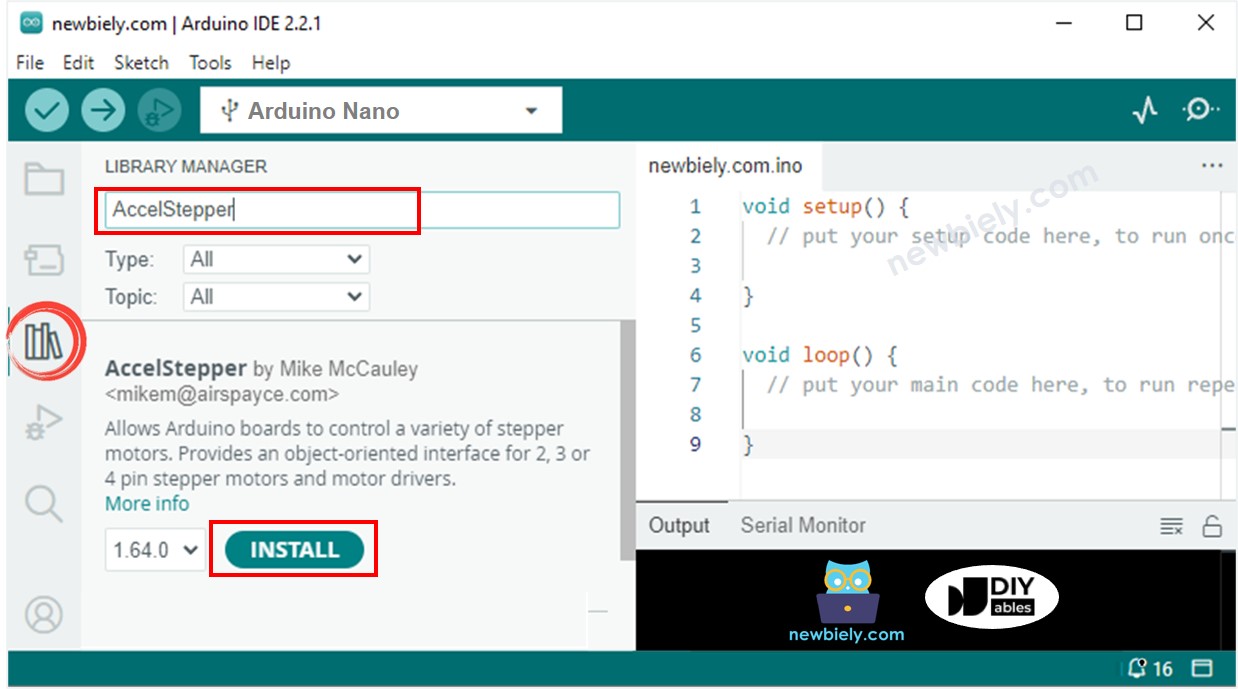
- Copy the code and open it in the Arduino IDE.
- Click the Upload button to upload the code to the Arduino Nano.
- The stepper motor will rotate one revolution in the clockwise direction, pause for 5 seconds, rotate back one revolution in the counterclockwise direction, pause for 5 seconds, and repeat this process.
- Check out the result in the Serial Monitor.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!