Arduino Nano - Cooling System using DS18B20 Temperature Sensor
This tutorial instructs you how to use Arduino Nano to control the temperature with the help of a fan and a DS18B20 temperature sensor.
- When the temperature gets too high, Arduino Nano turns on the cooling fan.
- When the temperature is back to normal, Arduino Nano turns off the cooling fan.
If you would like to use a DHT11 or DHT22 instead of the DS18B20 sensor, please refer to Arduino Nano - Cooling System using DHT Sensor.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Buy Note: Many DS18B20 sensors available in the market are unreliable. We strongly recommend buying the sensor from the DIYables brand using the link provided above. We tested it, and it worked reliably.
Overview of Cooling Fan and DS18B20 Temperature Sensor
The fan in this tutorial requires a 12v power supply. If the power is supplied, the fan will turn on and if not, the fan will be off. To control the fan with Arduino Nano, we must use a relay as an intermediary.
If you are unfamiliar with temperature sensors and fans (including pinouts, how they work, and how to program them), the following tutorials can provide more information:
Wiring Diagram
- Wiring diagram using a breadboard.
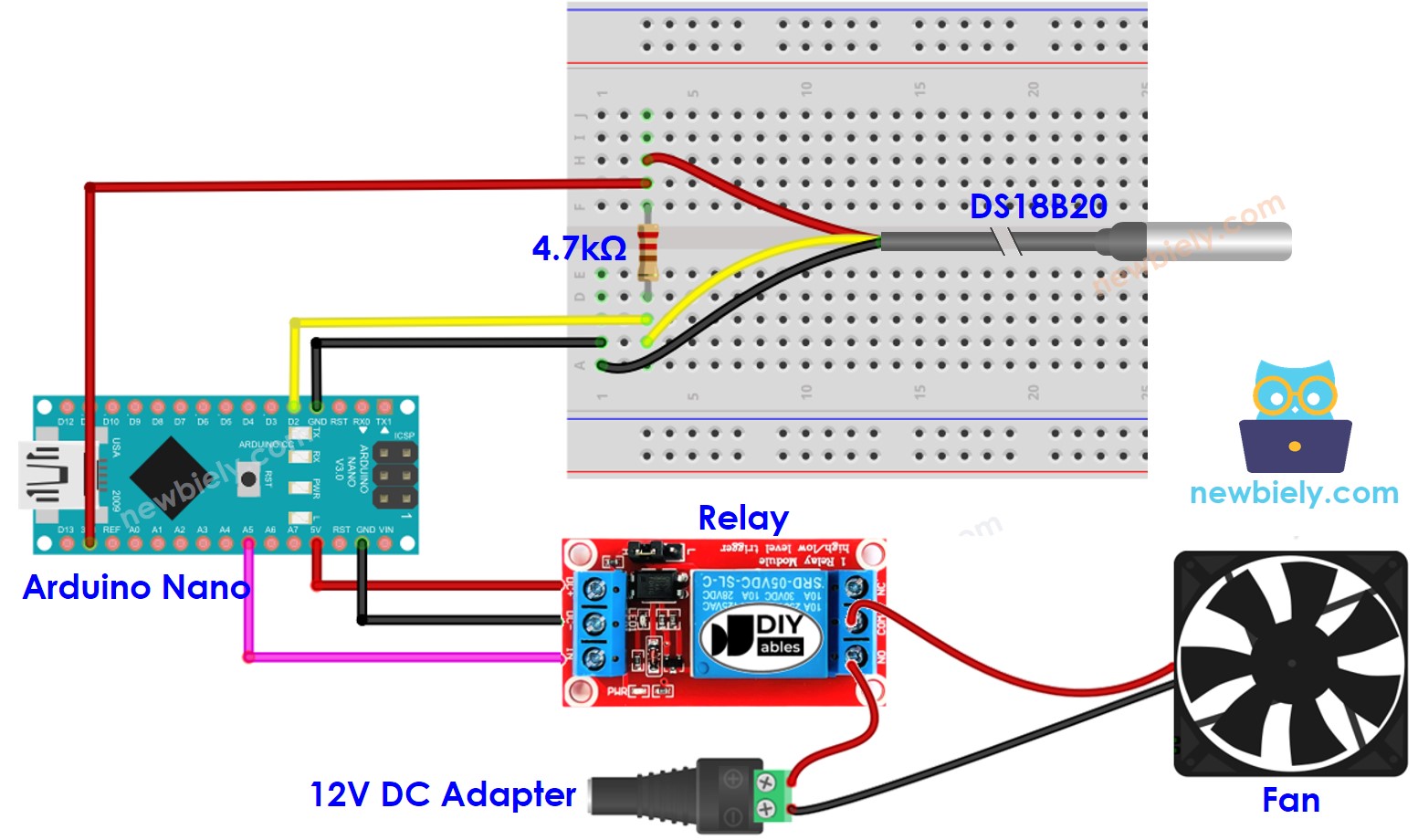
This image is created using Fritzing. Click to enlarge image
- Wiring diagram using a terminal adapter (recommended).
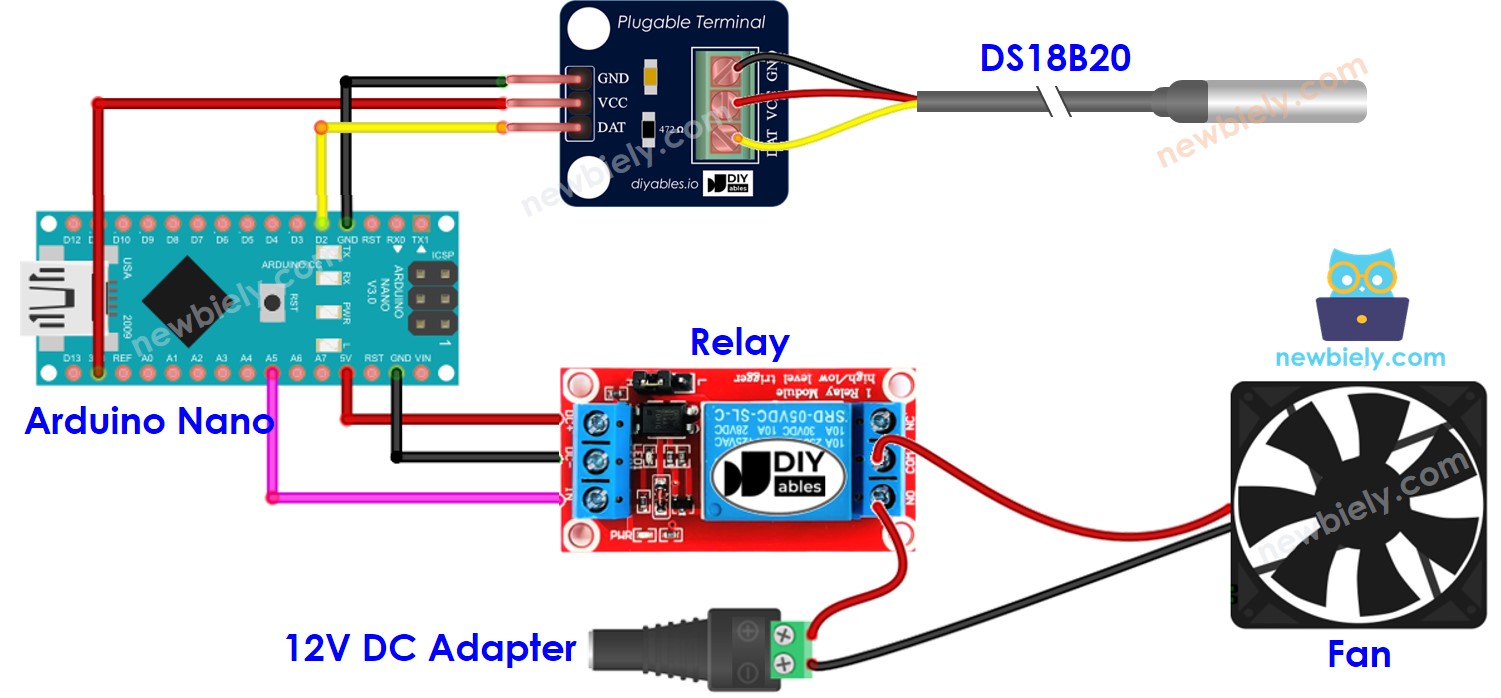
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
We recommend buying a DS18B20 sensor along with its accompanying wiring adapter for a seamless setup. This adapter includes an integrated resistor, removing the need for an additional resistor in the wiring.
How System Works
- The Arduino Nano will take a reading from the temperature sensor.
- If the reading is higher than the upper threshold, the fan will be activated by the Arduino Nano.
- If the reading is lower than the lower threshold, the Arduino Nano will switch off the fan.
This loop is repeated without end.
Arduino Nano Code for Cooling System with DS18B20 sensor
In the code above, when the temperature goes above 25°C, the Arduino Nano will activate the fan. The fan will remain on until the temperature drops below 20°C.
Detailed Instructions
- Connect Arduino Nano to a computer using a USB cable
- Launch the Arduino IDE, choose the correct board and port
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “Dallas” and locate the DallasTemperature library created by Miles Burton.
- Then, press the Install button to add it to your project.
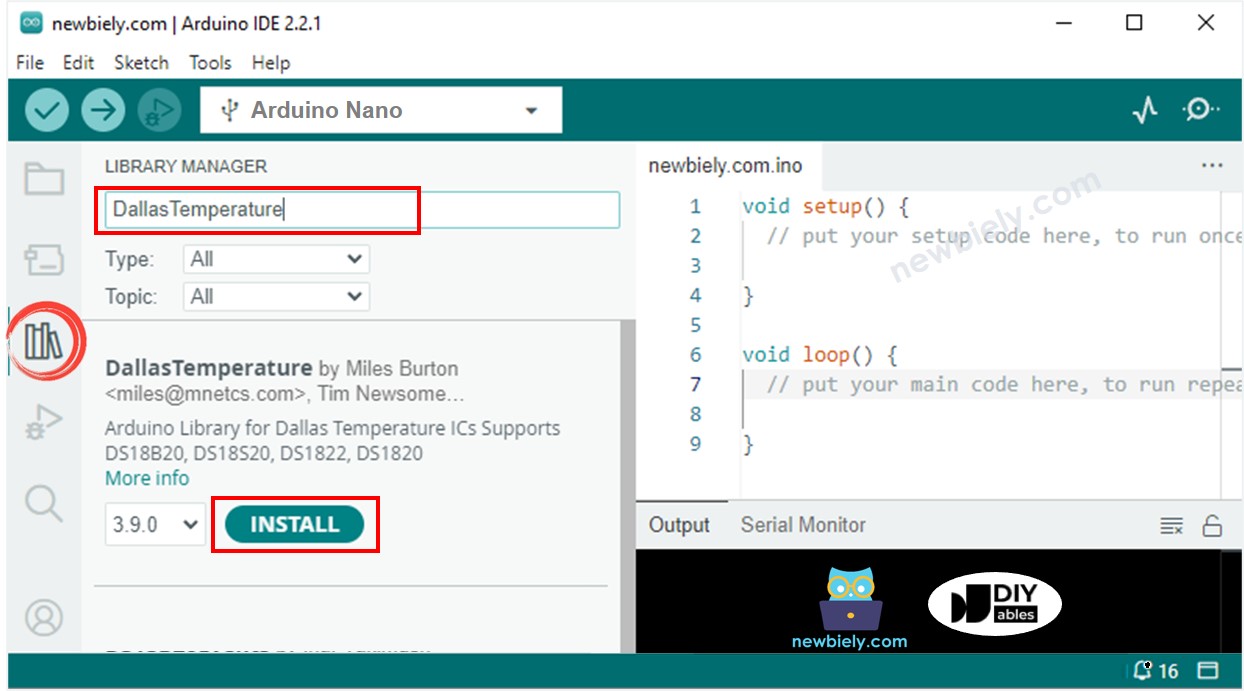
- You will be asked to install the dependency. Click Install All button to install OneWire library.
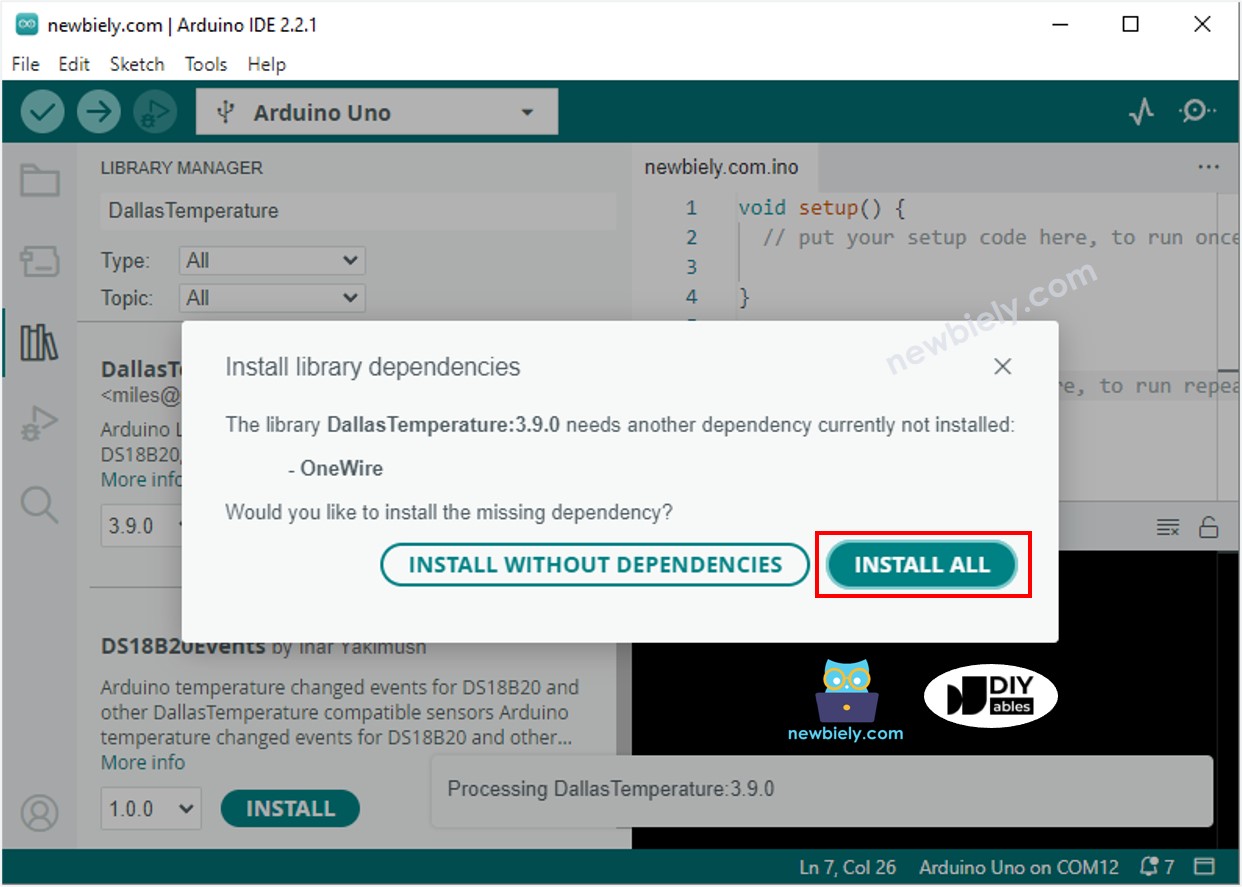
- Copy the code and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to compile and upload the code to the Arduino Nano.
- Change the temperature of the sensor's environment by making it hotter or colder.
- Check the status of the fan on the Serial Monitor.
Advanced Knowledge
This controlling technique is referred to as an on-off controller, which is also known as a signaller or "bang-bang" controller. It is quite easy to implement this method.
An alternative to the traditional temperature control method is the PID controller. This approach provides a more stable desired temperature, however, it is complex and challenging to understand and use. As a result, the PID controller is not widely used for temperature control.