Arduino Nano - Keypad - Relay
This tutorial instructs you how to use a keypad and Arduino Nano to control relay. When the user inputs the correct password on the keypad, the Arduino Nano will activate the relay.
The tutorial also provides the Arduino Nano code that activates a relay for a certain duration and then deactivates it without using the delay() function. Furthermore, the Arduino Nano code is capable of handling multiple passwords.
By connecting relay to an Electromagnetic Lock, a Solenoid Lock, a Linear Actuator, a Heating Element, a Pump, or a Fan ... We can control them using a keypad.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of Keypad and Relay
If you are unfamiliar with keypad and relay (including pinout, operation, programming, etc.), the following tutorials can help:
- Arduino Nano - Keypad tutorial
- Arduino Nano - Relay tutorial
Wiring Diagram
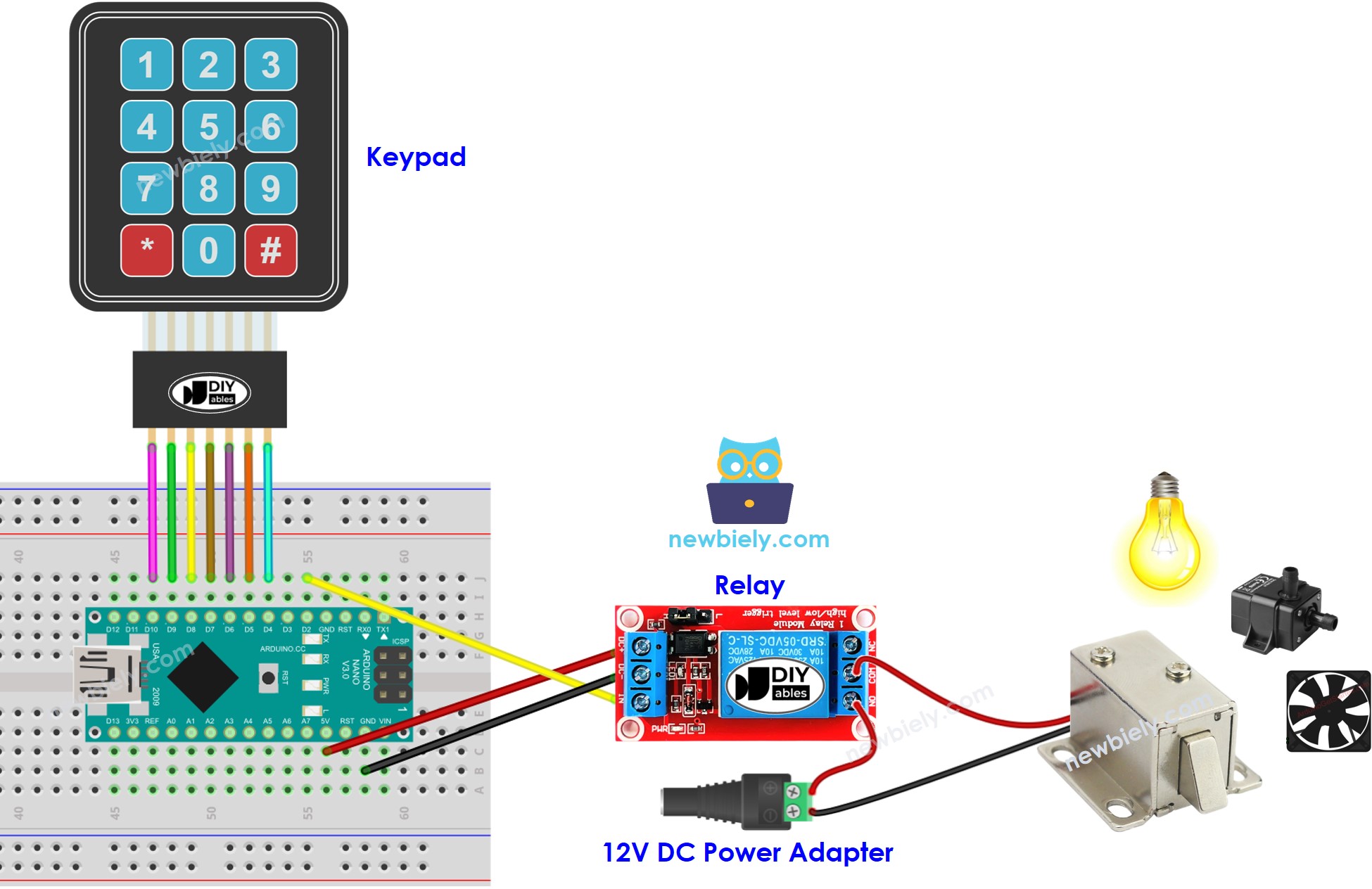
This image is created using Fritzing. Click to enlarge image
Arduino Nano Code - turn relay on if the password is correct
If the password is correct, the following codes will activate a relay.
Detailed Instructions
- Connect a USB cable to the Arduino Nano and PC.
- Open the Arduino IDE, select the correct board and port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “keypad” and locate the keypad library created by Mark Stanley and Alexander Brevig.
- Then, press the Install button to install the keypad library.
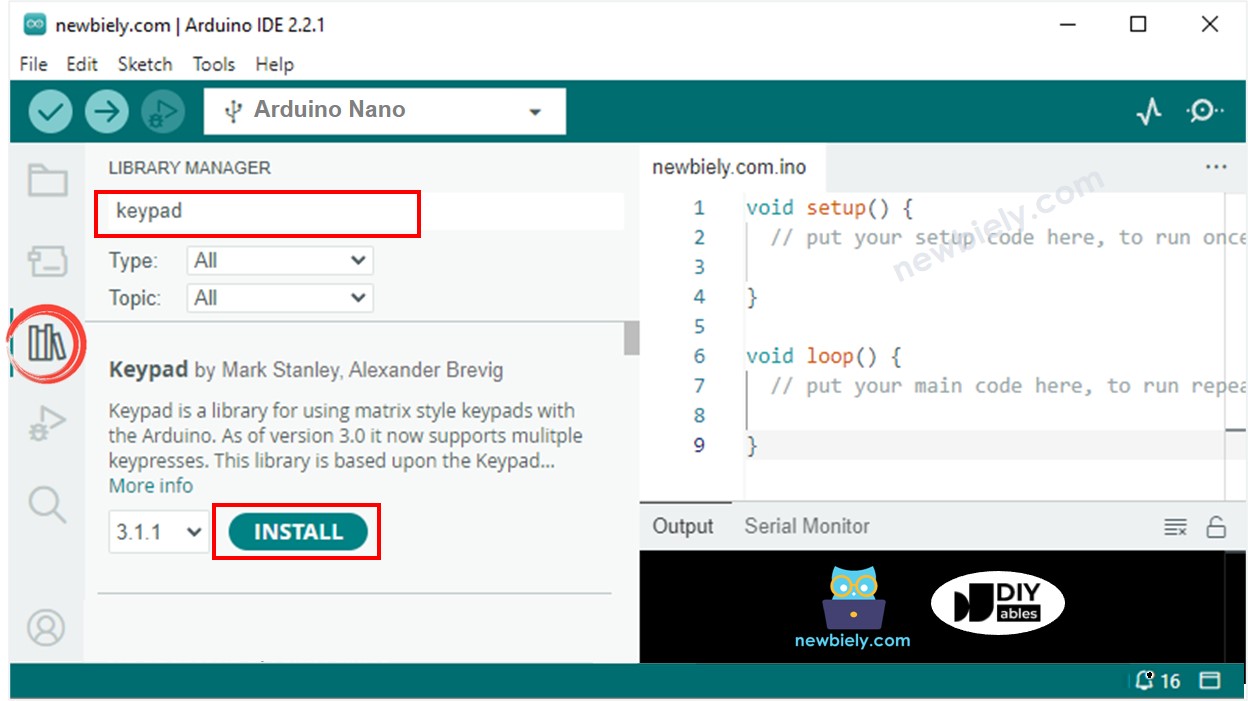
- Search for “ezOutput”, then locate the ezOutput library by ArduinoGetStarted.
- Press the Install button to install the ezOutput library.
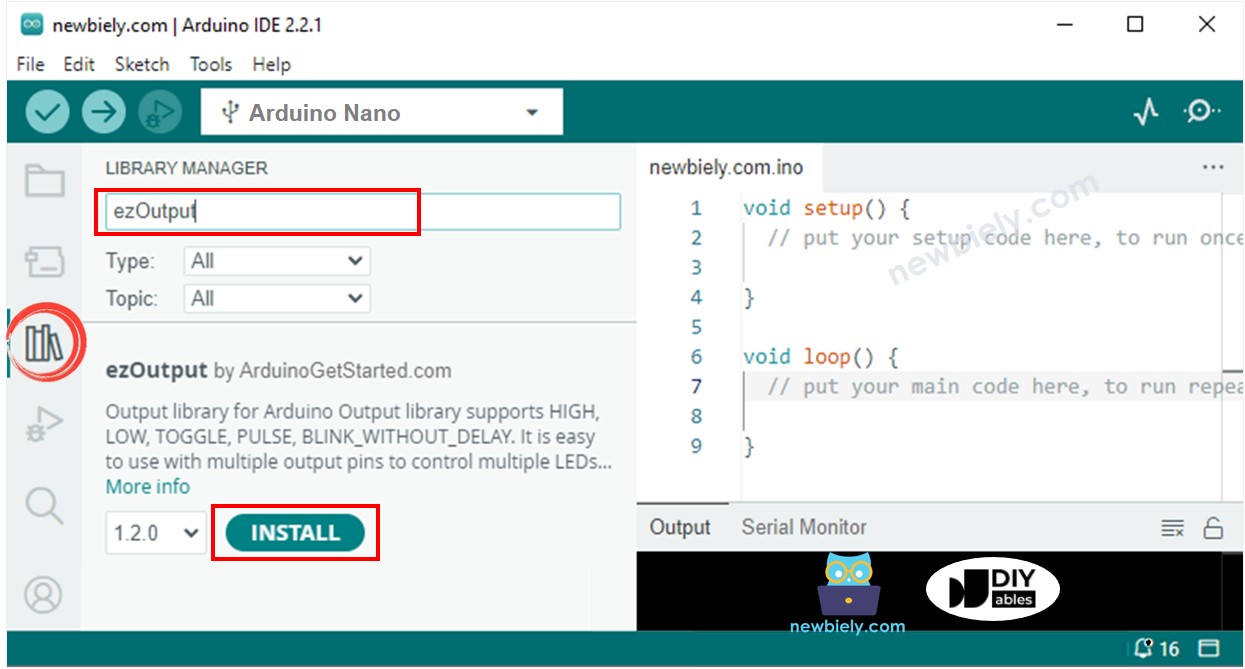
- Copy the code and open it in the Arduino IDE.
- Then, press the Upload button in the Arduino IDE to compile and upload the code to the Arduino Nano.
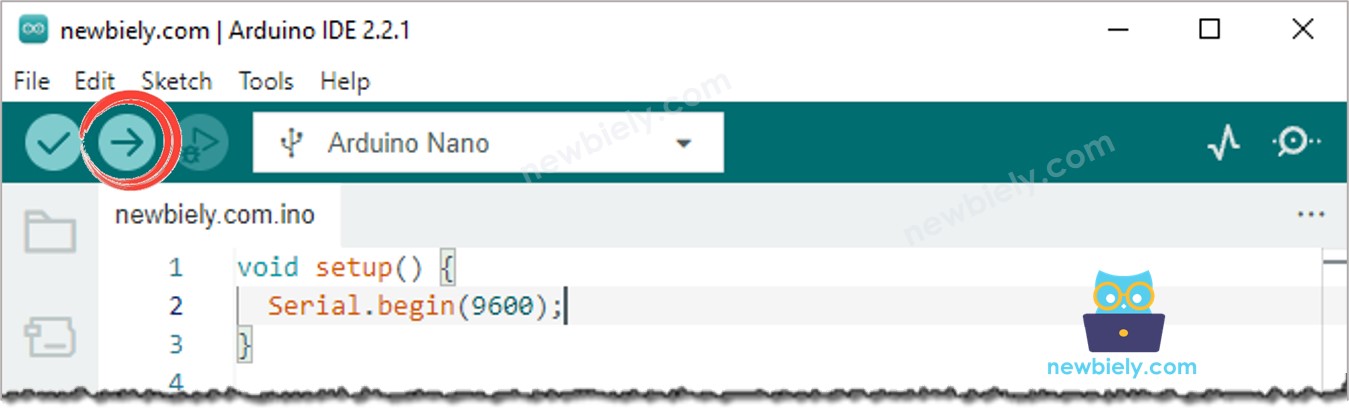
- Enter 1234 followed by the # key and then 9765 followed by the # key.
- Check the result on the Serial Monitor and observe the state of the relay.
Code Explanation
Authorized passwords are pre-defined in the Arduino Nano code. A string is utilized to store the password inputted by users, referred to as the input string. On the keypad, two keys (* and #) are used for special purposes: clearing the password and terminating the password. When a key on the keypad is pressed:
- If the pressed key is not either of the two special keys, it is appended to the input string.
- If the pressed key is *, the input string is cleared. This can be used to start or restart inputting the password.
- If the pressed key is #:
- The Arduino Nano verifies the input password by comparing the input string with the pre-defined passwords. If it matches one of the pre-defined passwords, the relay is turned on.
- Regardless of whether the password is correct or not, the input string is cleared for the next input.
Arduino Nano Code - turn a relay on in a period of time if the password is correct
If the password is correct, the relay will be switched on for 5 seconds. After that period of time, the relay will be deactivated.