Arduino Nano - Button Count - OLED
This tutorial instructs you how to use Arduino Nano and button to count the press events and then display the value on OLED. In detail:
- Arduino Nano counts how many times a button is pressed
- Arduino Nano shows the count number on an OLED display.
- Arduino Nano automatically centers the count number both horizontally and vertically on the OLED display.
In this tutorial, we will be debouncing the button without using the delay() function. For more information, please refer to Why do we need debouncing?
You can modify this to work with different sensors in place of the button.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand, DIYables.
Additionally, some of these links are for products from our own brand, DIYables.
Wiring Diagram
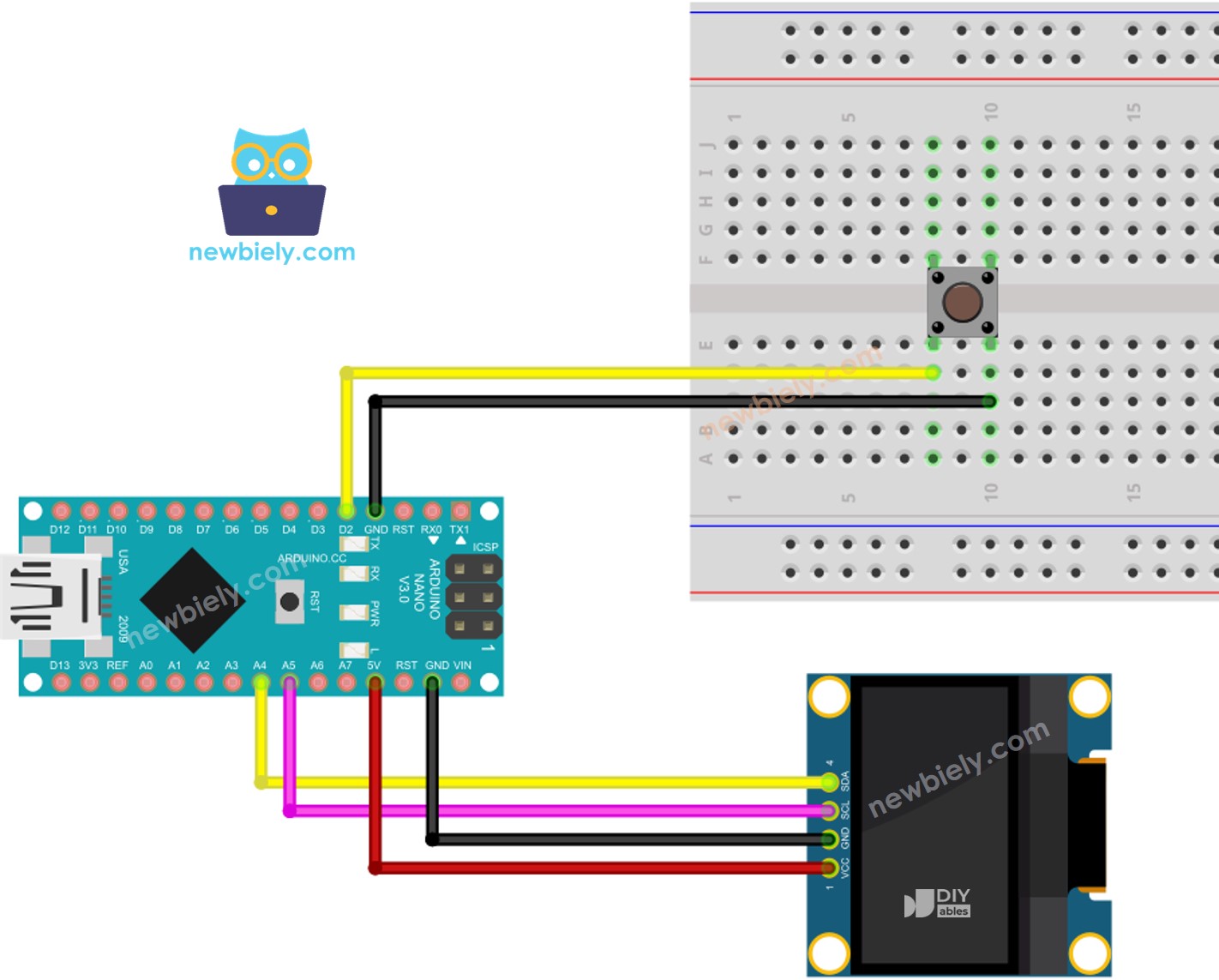
This image is created using Fritzing. Click to enlarge image
Arduino Nano Code - Vertical and Horizontal Center Align on OLED
/*
* This Arduino Nano code was developed by newbiely.com
*
* This Arduino Nano code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-nano/arduino-nano-button-count-oled
*/
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <ezButton.h>
#define OLED_WIDTH 128 // OLED display width, in pixels
#define OLED_HEIGHT 64 // OLED display height, in pixels
Adafruit_SSD1306 oled(OLED_WIDTH, OLED_HEIGHT, &Wire, -1); // create SSD1306 display object connected to I2C
ezButton button(2); // create ezButton object for pin D2
unsigned long prev_count = 0;
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
oled.setTextSize(2); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(0, 10); // position to display
button.setDebounceTime(50); // set debounce time to 50 milliseconds
button.setCountMode(COUNT_FALLING);
}
void loop() {
button.loop(); // MUST call the loop() function first
unsigned long count = button.getCount();
if (prev_count != count) {
Serial.println(count); // print count to Serial Monitor
String text = String(count);
oled_display_center(text);
prev_count != count;
}
}
void oled_display_center(String text) {
int16_t x1;
int16_t y1;
uint16_t width;
uint16_t height;
oled.getTextBounds(text, 0, 0, &x1, &y1, &width, &height);
// center the display both horizontally and vertically
oled.clearDisplay(); // clear display
oled.setCursor((OLED_WIDTH - width) / 2, (OLED_HEIGHT - height) / 2);
oled.println(text); // text to display
oled.display();
}
※ NOTE THAT:
The code below will horizontally and vertically center the text on an OLED display. For more information, please refer to How to vertical/horizontal center on OLED.