Arduino Nano - LED - Fade
This tutorial instructs you how to program Arduino Nano to fade LED. We will go through three examples and compare the differences between them:
- How to program an Arduino Nano to fade LED by using delay() function
- How to program an Arduino Nano to fade LED by using millis() function
- How to program an Arduino Nano to fade LED by utilizing ezLED library
Hardware Preparation
1 | × | Arduino Nano | |
1 | × | USB A to Mini-B USB cable | |
1 | × | LED | |
1 | × | 220 ohm resistor | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Optional) 9V Power Adapter for Arduino Nano | |
1 | × | (Recommended) Screw Terminal Adapter for Arduino Nano |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of LED
The LED Pinout
LED has two pins:
- Cathode(-) pin: must be attached to GND (0V)
- Anode(+) pin: used for controlling LED's state
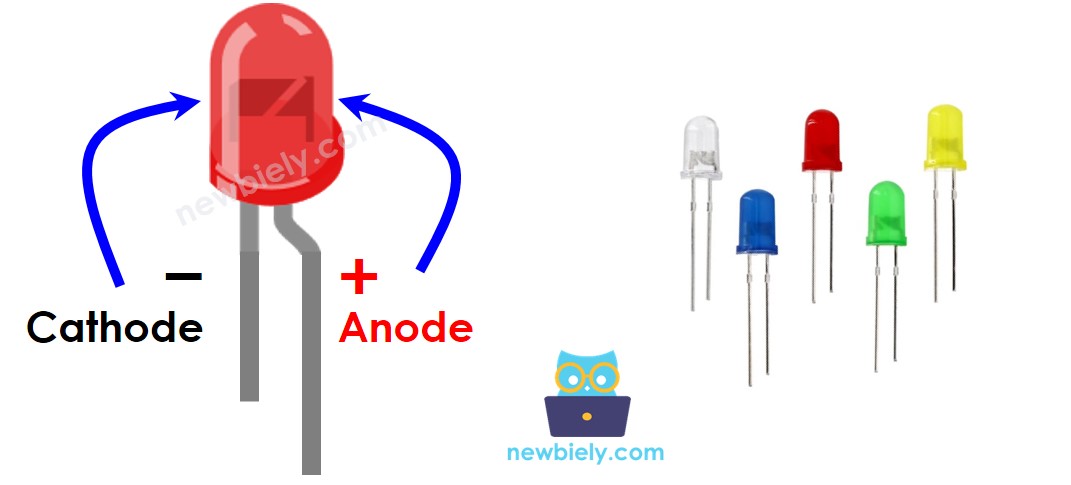
How It Works
Once the cathode(-) is connected to GND:
- If GND is connected to the anode(+), the LED will be OFF.
- If VCC is connected to the anode(+), the LED will be ON.
- If a PWM signal is applied to the anode(+), the brightness of the LED will be adjusted according to the PWM value, which ranges from 0 to 255. A larger PWM value will result in a brighter LED, while a smaller PWM value will result in a darker LED.
- When the PWM value is 0, it is equivalent to GND, thus the LED will be OFF.
- When the PWM value is 255, it is equivalent to VCC, thus the LED will be fully ON.
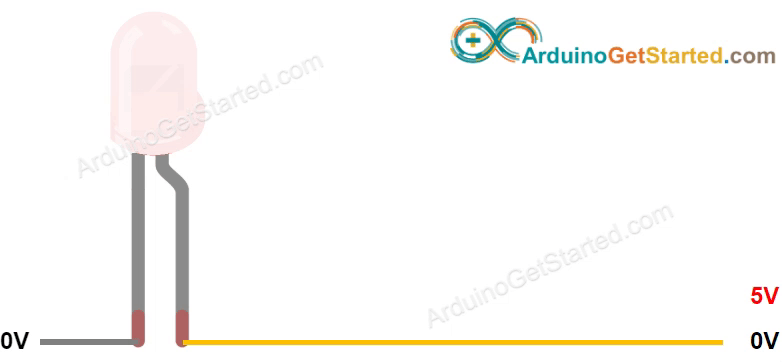
※ NOTE THAT:
For the majority of LEDs, a resistor must be placed between the anode(+) and VCC. The resistor's value is dependent on the LED's specifications.
Arduino Nano - fade LED
Some of the pins on an Arduino Nano can be programmed to generate a PWM signal. To fade an LED, we can connect the anode (+) pin of the LED to an Arduino Nano pin, the cathode (-) to GND, and program the Arduino Nano pin to generate PWM.
Wiring Diagram
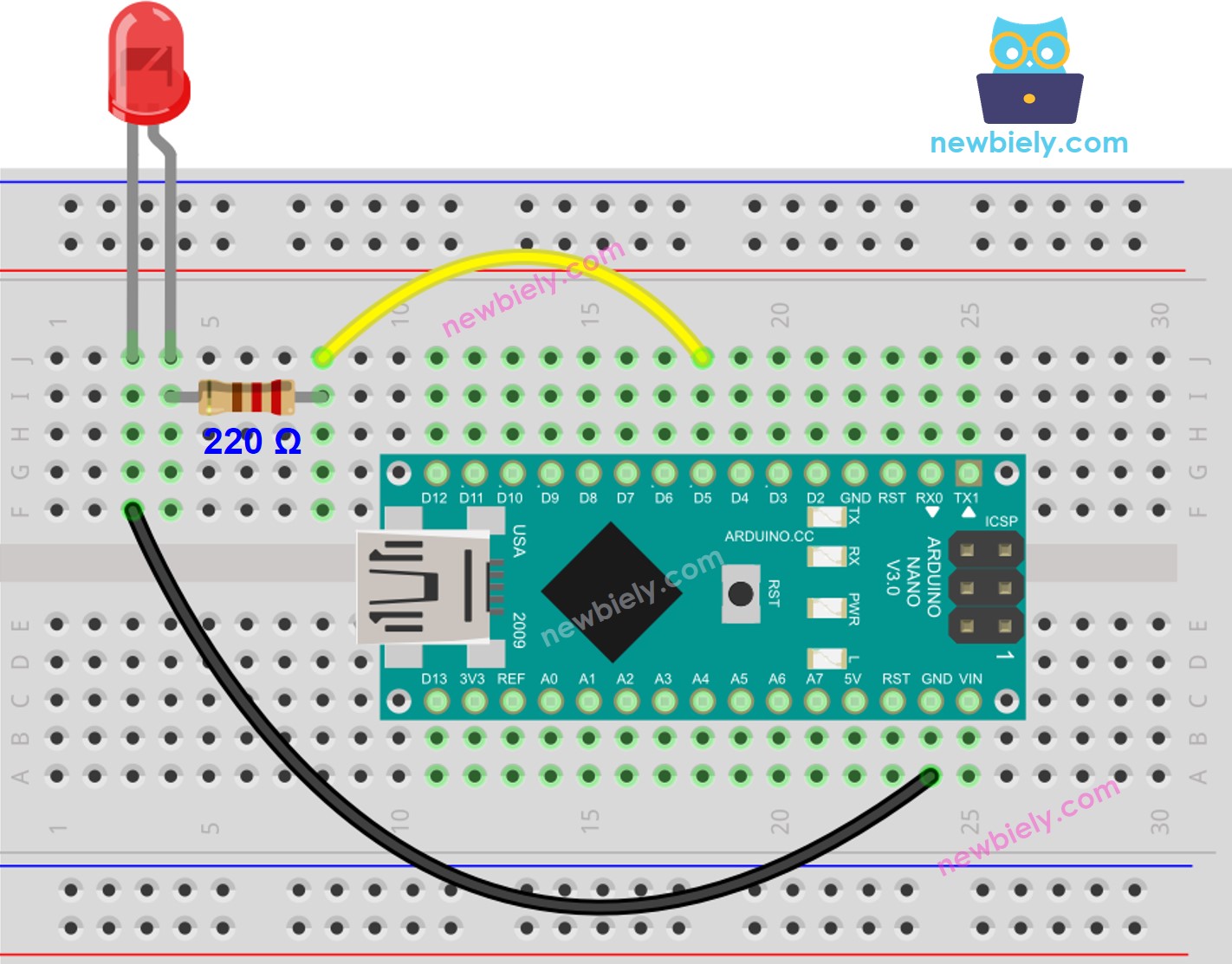
This image is created using Fritzing. Click to enlarge image
How To Program
- Set up a digital output on an Arduino's pin by using the pinMode() function.
- For example, this could be done for pin 5:
- Adjust the brightness of the LED by creating a PWM signal with the analogWrite() function:
The brightness can range from 0 to 255.
Arduino Nano Code - Fade LED
Detailed Instructions
- Attach Arduino Nano to the computer using a USB cable.
- Open the Arduino IDE, select the correct board and port.
- Copy the above code and open it in the Arduino IDE.
- Click the Upload button on Arduino IDE to compile and upload the code to the Arduino Nano board.
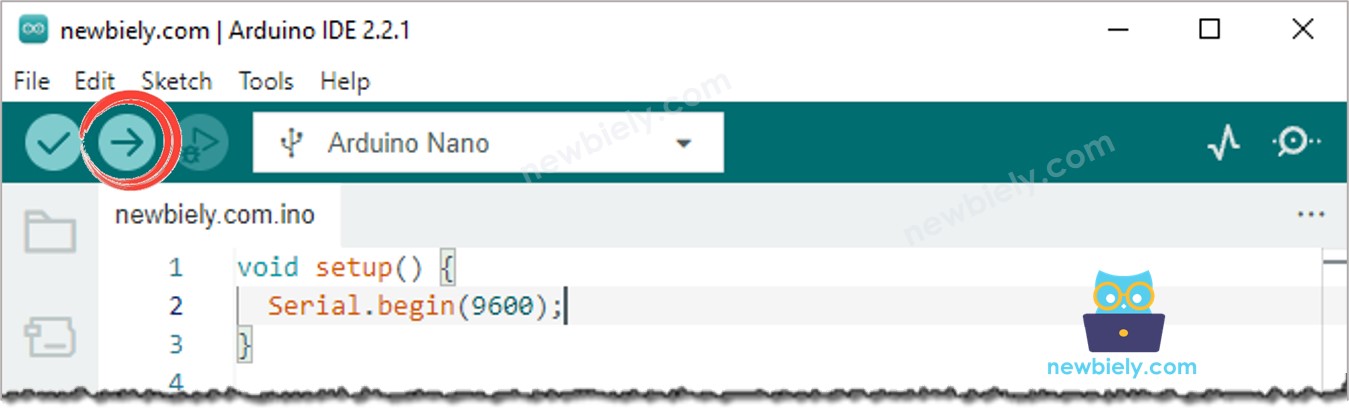
- Check out the luminosity of the LED.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
※ NOTE THAT:
The example above makes use of the delay() function to create a fade-in and fade-out effect. However, this method is not very smooth and prevents other code from running. In the upcoming sections, we will learn how to achieve a smooth fade-in and fade-out without blocking other code by using the millis() function.
How to fade-in LED in a period without using delay()
How to fade-out LED in a period without using delay()
Video Tutorial
Challenge Yourself
Adjust the brightness of the LED with a potentiometer. Hint: Have a look at Arduino Nano - Potentiometer for more information.
Additional Knowledge
- The analogWrite() function in Arduino Nano generates a PWM signal which causes a LED to fade. However, if one has the advanced knowledge to create a custom function that produces a low-frequency PWM signal, the LED will be blinked instead of faded.
- Summary: PWM signals generated by Arduino Nano can be used for a variety of purposes, such as controlling servo motors, DC motors, producing sound with a piezo buzzer, fading LEDs, and blinking LEDs.