Arduino Nano - DHT11 - LCD
This tutorial instructs you how to use Arduino Nano to read the temperature and humidity from DHT11 sensor and display it on LCD I2C.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of DHT11 and LCD
If you are unfamiliar with the DHT11 temperature humidity sensor and LCD (pinout, how it works, how to program ...), then the following tutorials can help:
Wiring Diagram
Arduino Nano - DHT11 and LCD Wiring Diagram
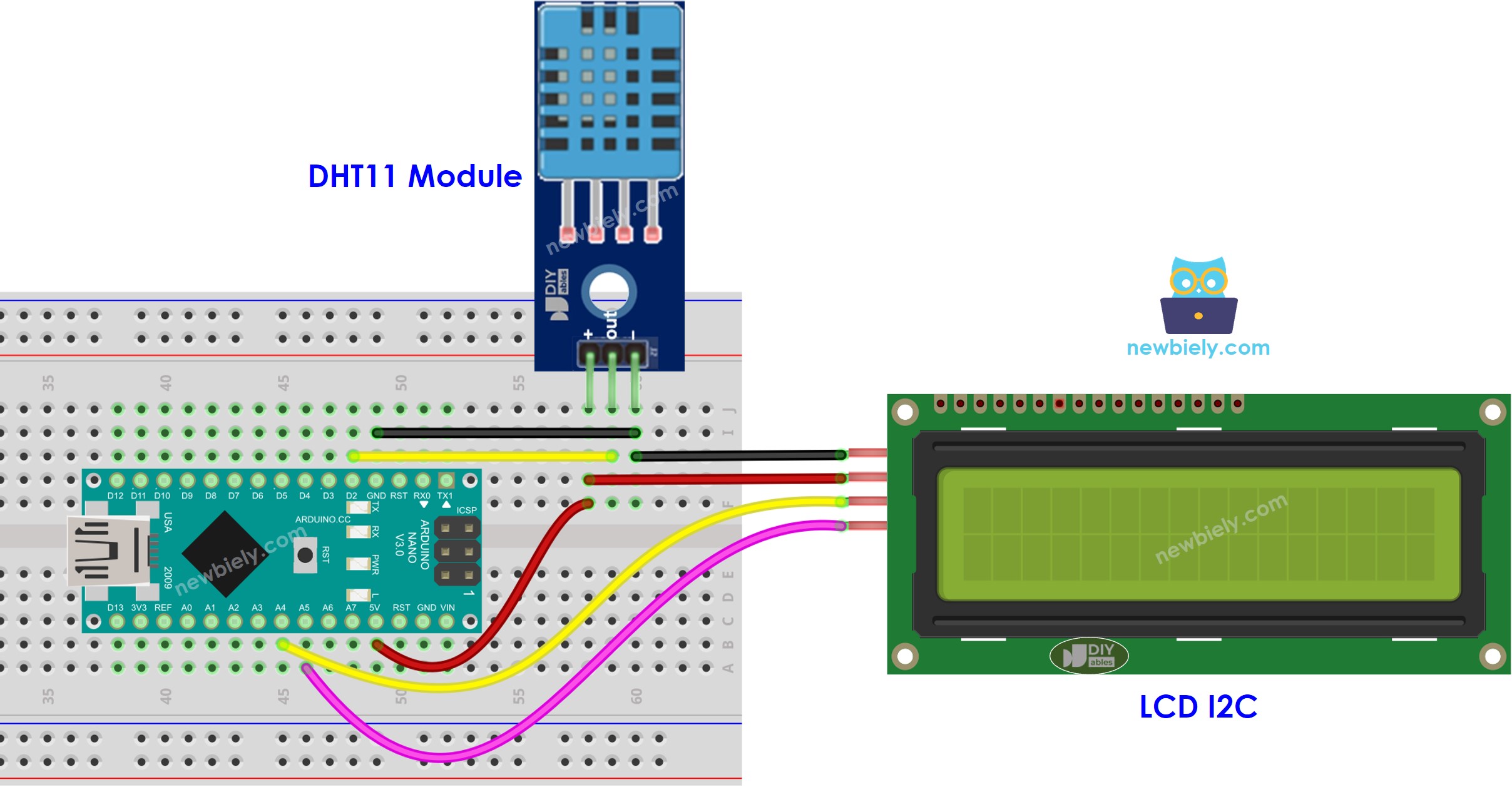
This image is created using Fritzing. Click to enlarge image
Please note that the power from the 5V pin of the Arduino Nano may not be enough for both the DHT11 and the LCD. If you see the LCD display nothing, please add extra power to the LCD and DHT11 as shown in the wiring diagram below.
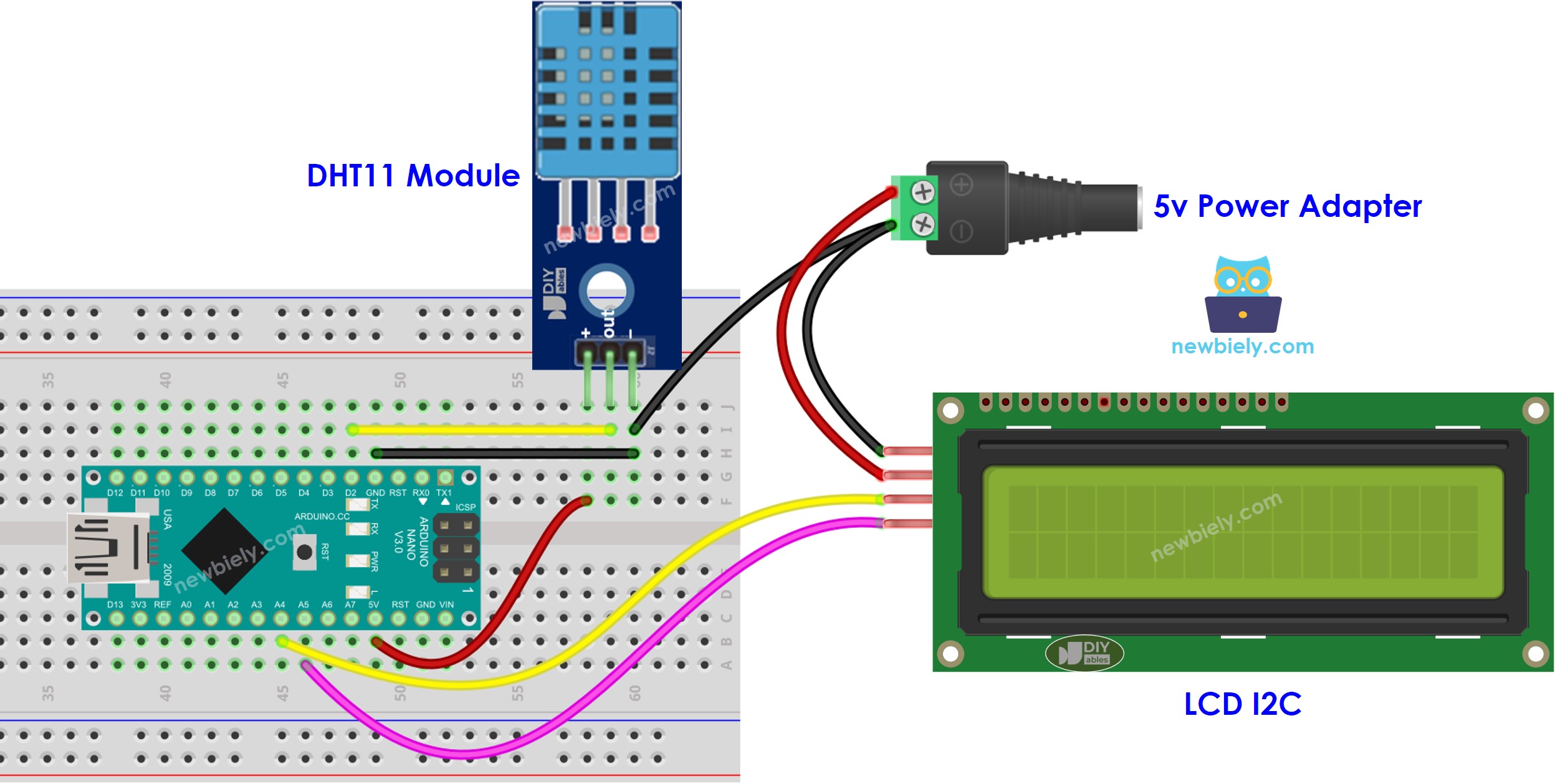
This image is created using Fritzing. Click to enlarge image
Arduino Nano Code - DHT11 Sensor - LCD I2C
※ NOTE THAT:
The address of the LCD can differ depending on the manufacturer. We have used 0x27 in our code, which is specified by DIYables manufacturer.
Detailed Instructions
- Connect an USB cable between the Arduino Nano and the PC.
- Open the Arduino IDE, choose the correct board and port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “DHT” and locate the DHT sensor library by Adafruit.
- Press the Install button to install the library.
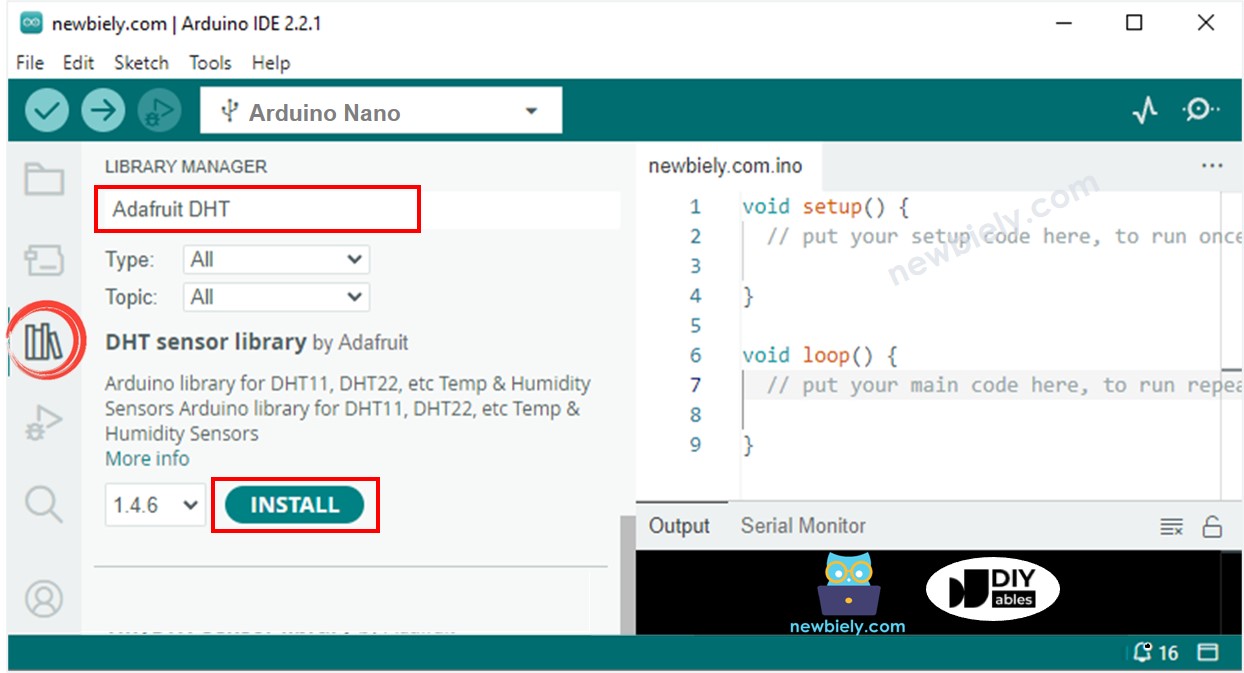
- You will be prompted to install some other library dependencies.
- Click the Install All button to install all necessary library dependencies.
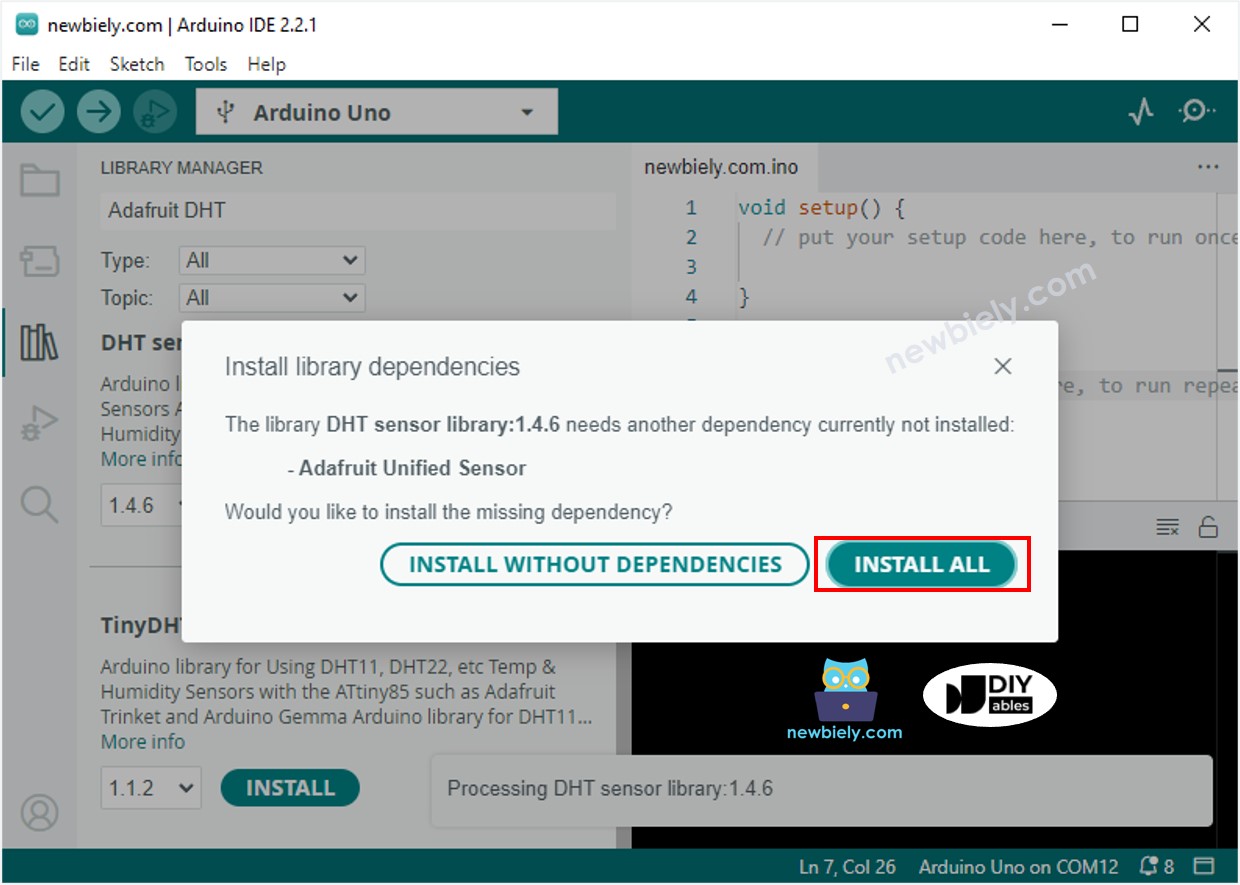
- Search for “LiquidCrystal I2C” and locate the LiquidCrystal_I2C library by Frank de Brabander.
- Then, click the Install button to install the library.
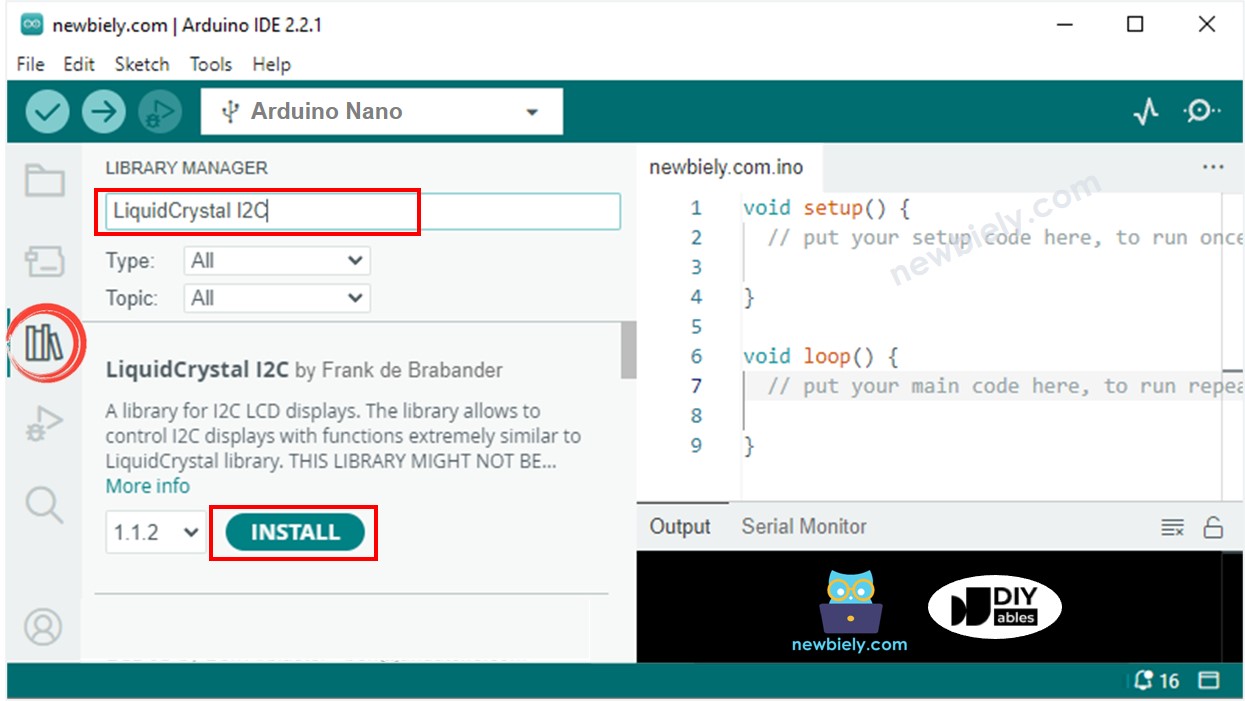
- Copy the code above and open it with the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to the Arduino Nano.
- Change the temperature of the environment around the sensor.
- Check out the result on the LCD.
A grandfather, who was learning through this tutorial to help his grandchild, tested this code with Arduino Nano and sent us the result as follows:
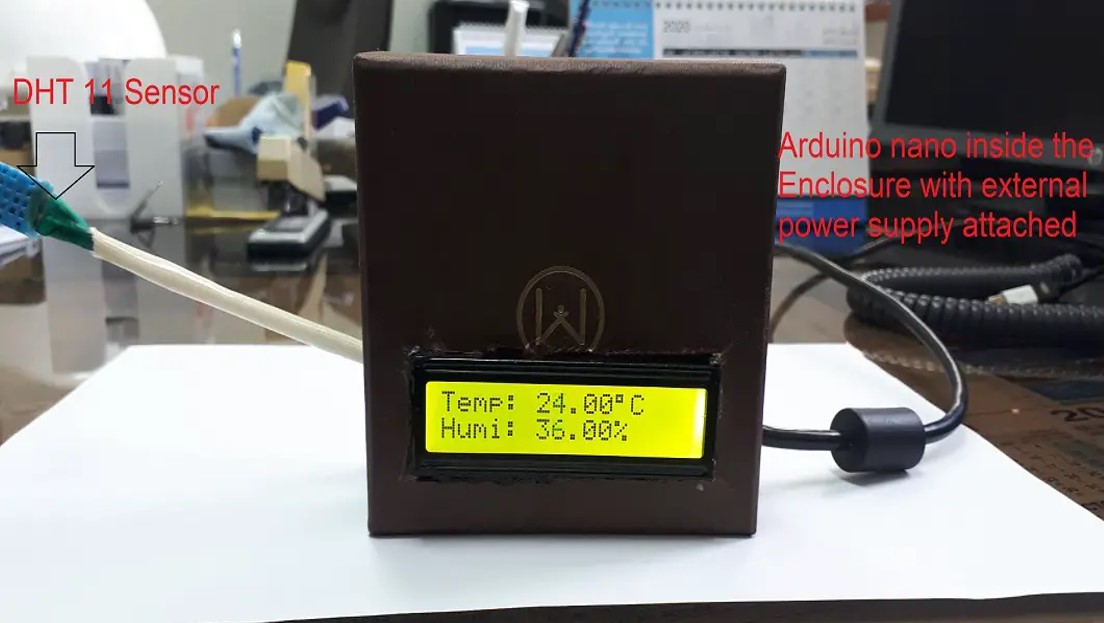
If the LCD does not show anything, check Troubleshooting on LCD I2C for help.