Arduino Nano - Keypad - LCD
This tutorial instructs you how to use Arduino Nano to display the input from keypad on LCD display.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand, DIYables .
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Keypad and LCD
If you are unfamiliar with keypad and LCD (pinout, how it works, how to program ...), the following tutorials can help you:
Wiring Diagram
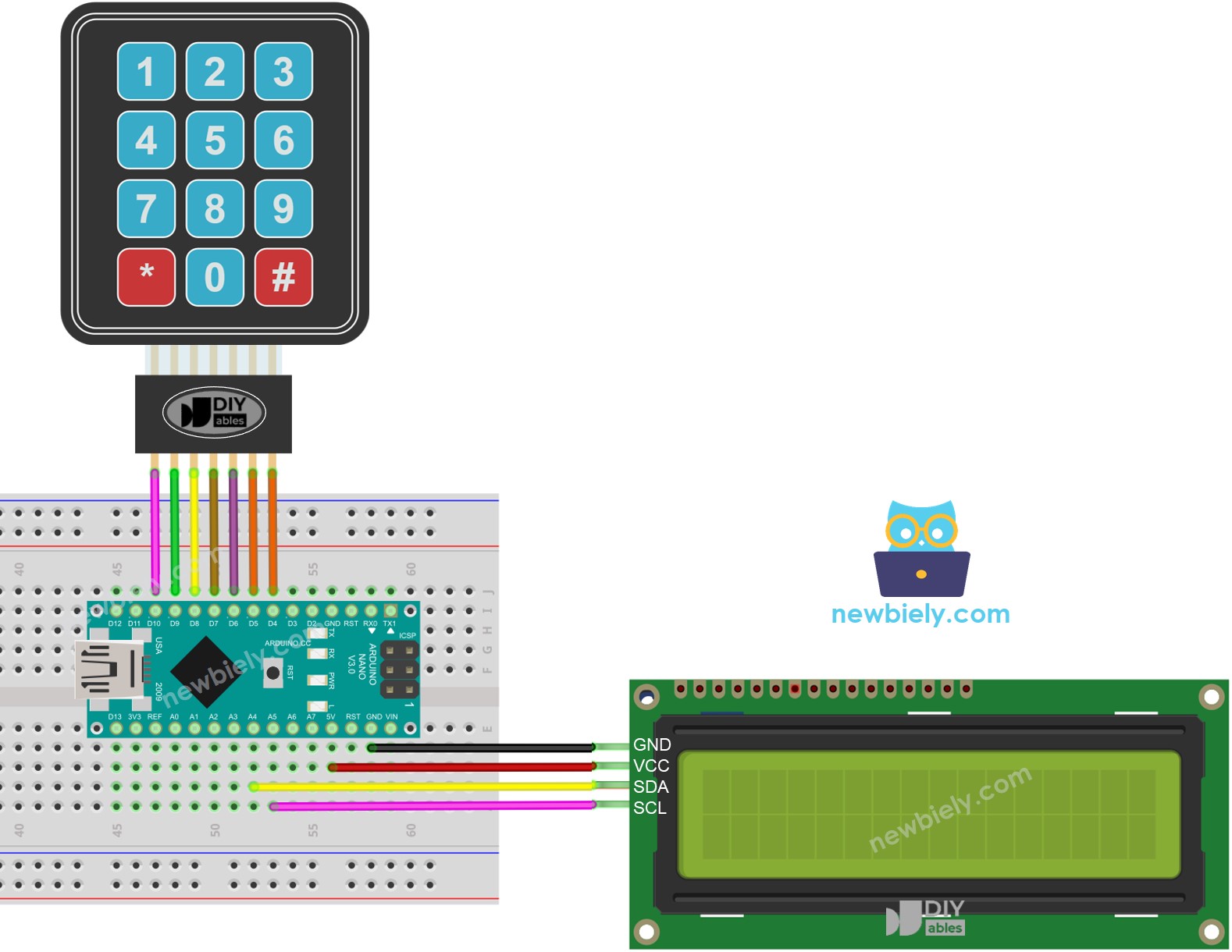
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Arduino Nano Code
/*
* This Arduino Nano code was developed by newbiely.com
*
* This Arduino Nano code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-nano/arduino-nano-keypad-lcd
*/
#include <Keypad.h>
#include <LiquidCrystal_I2C.h>
const int ROW_NUM = 4; //four rows
const int COLUMN_NUM = 3; //four columns
const byte pin_rows[ROW_NUM] = {10, 9, 8, 7}; // The Arduino Nano pin connected to the row pins of the keypad
const byte pin_column[COLUMN_NUM] = {6, 5, 4}; // The Arduino Nano pin connected to the column pins of the keypad
char key_layout[ROW_NUM][COLUMN_NUM] = {
{'1', '2', '3'},
{'4', '5', '6'},
{'7', '8', '9'},
{'*', '0', '#'}
};
Keypad keypad = Keypad( makeKeymap(key_layout), pin_rows, pin_column, ROW_NUM, COLUMN_NUM);
LiquidCrystal_I2C lcd(0x27, 16, 2); // I2C address 0x27 (from DIYables LCD), 16 column and 2 rows
int cursorColumn = 0;
void setup() {
lcd.init(); // Initialize the LCD I2C display
lcd.backlight();
}
void loop() {
char key = keypad.getKey();
if (key) {
lcd.setCursor(cursorColumn, 0); // move cursor to (cursorColumn, 0)
lcd.print(key); // print key at (cursorColumn, 0)
cursorColumn++; // move cursor to next position
if (cursorColumn == 16) { // if reaching limit, clear LCD
lcd.clear();
cursorColumn = 0;
}
}
}
※ NOTE THAT:
The address of the LCD may differ depending on the manufacturer. In our code, we have used 0x27, as specified by DIYables.
Detailed Instructions
- Connect an USB cable to the Arduino Nano and the PC.
- Open the Arduino IDE, select the appropriate board and port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “keypad” and locate the keypad library by Mark Stanley and Alexander Brevig.
- Press the Install button to install the keypad library.
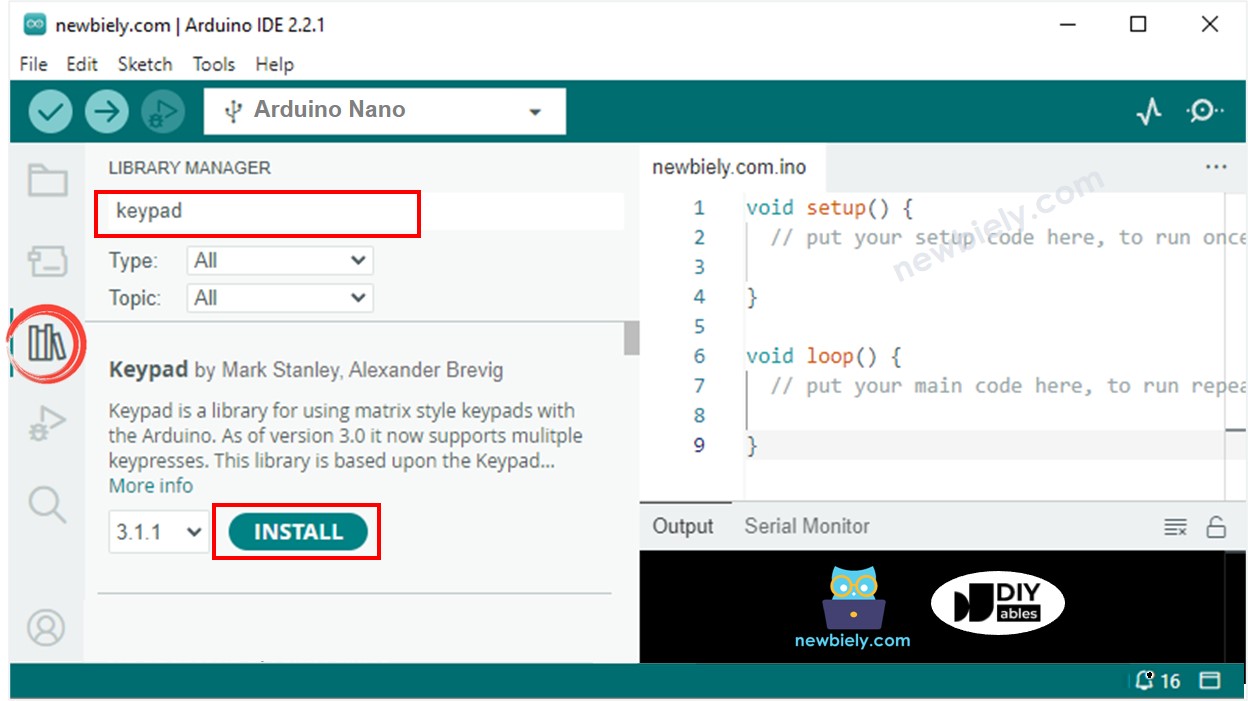
- Search for “LiquidCrystal I2C” and locate the LiquidCrystal_I2C library created by Frank de Brabander.
- Then, click the Install button to install the said library.
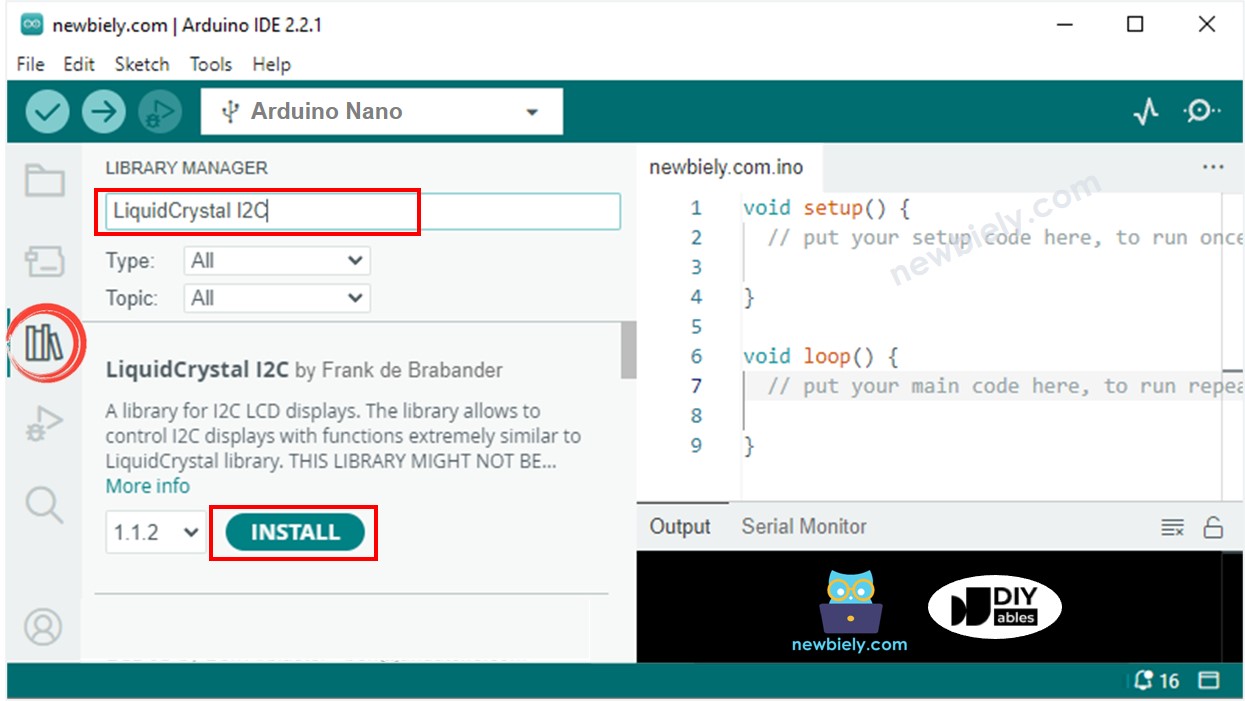
- Copy the code and open it in Arduino IDE.
- Click the Upload button on Arduino IDE to compile and upload the code to Arduino Nano.
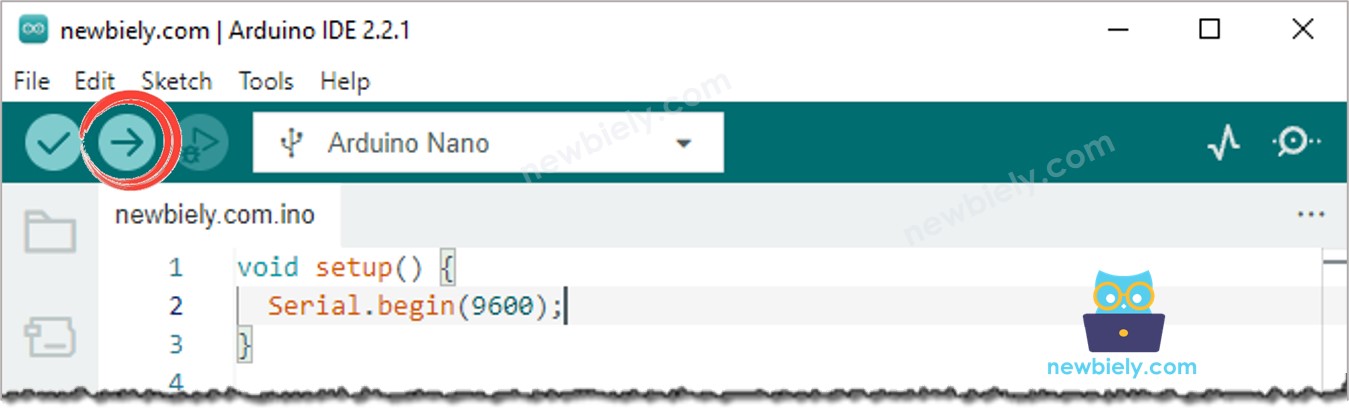
- Press certain keys on the keypad
- Check out the outcome on the LCD display
If the LCD does not show anything, . check out Troubleshooting on LCD I2C for help.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!