Arduino Nano - LDR Module
The LDR light sensor module can sense and measure the light in its surroundings. It has two outputs: one is a digital output that can be either low or high, and the other is an analog output.
This tutorial instructs you how to use an Arduino Nano and an LDR light sensor module to detect and measure the amount of light. We will cover the following:
- Connecting the LDR light sensor module to an Arduino Nano.
- Programming the Arduino Nano to detect light by reading the digital signal from the LDR light sensor module.
- Programming the Arduino Nano to measure the light level by reading the analog signal from the LDR light sensor module.
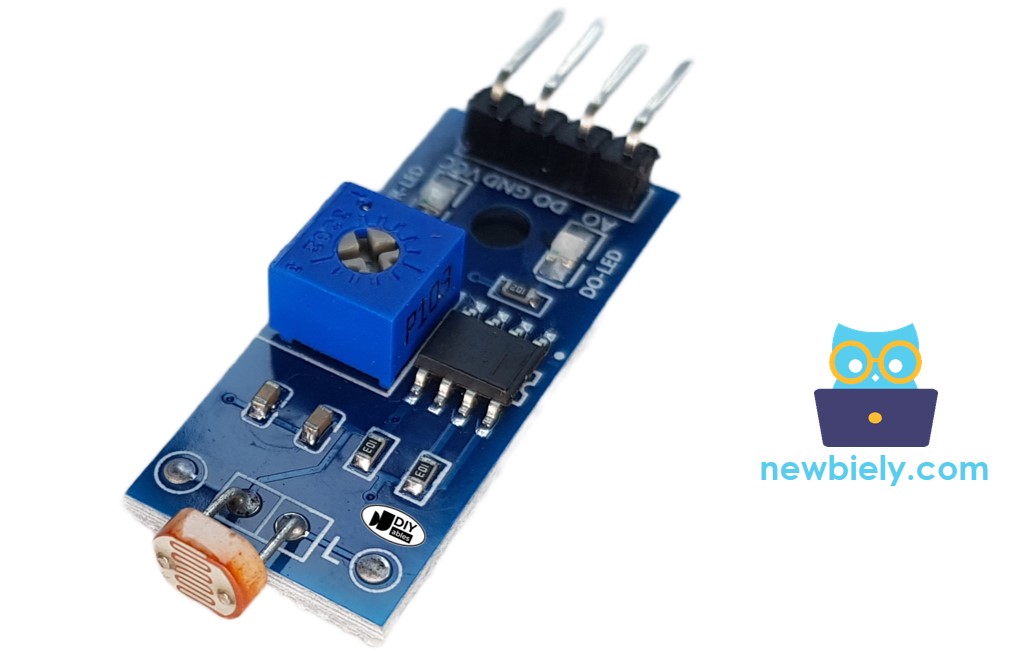
Later on, you can change the code so that when the light is detected, it can turn on an LED or a light bulb by using a relay.
If you prefer a light sensor in its raw form, I suggest exploring the tutorial on the Arduino Nano - Light Sensor.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of LDR Light Sensor Module
The LDR light sensor module can be used to find out if there is light or measure how much light is present in the surrounding area. It offers two choices through a digital output pin and an analog output pin.
Pinout
The LDR light sensor module has four pins with specific functions:
- VCC pin: Connect this pin to a power source (between 3.3V to 5V).
- GND pin: Connect this pin to the ground (0V) of the power source.
- DO pin: This is a digital output pin. When it's dark, the output is HIGH, and when it's light, the output is LOW. You can adjust the darkness-to-lightness threshold using a built-in potentiometer.
- AO pin: This is an analog output pin. The output value decreases as the light gets brighter and increases as the light gets darker.
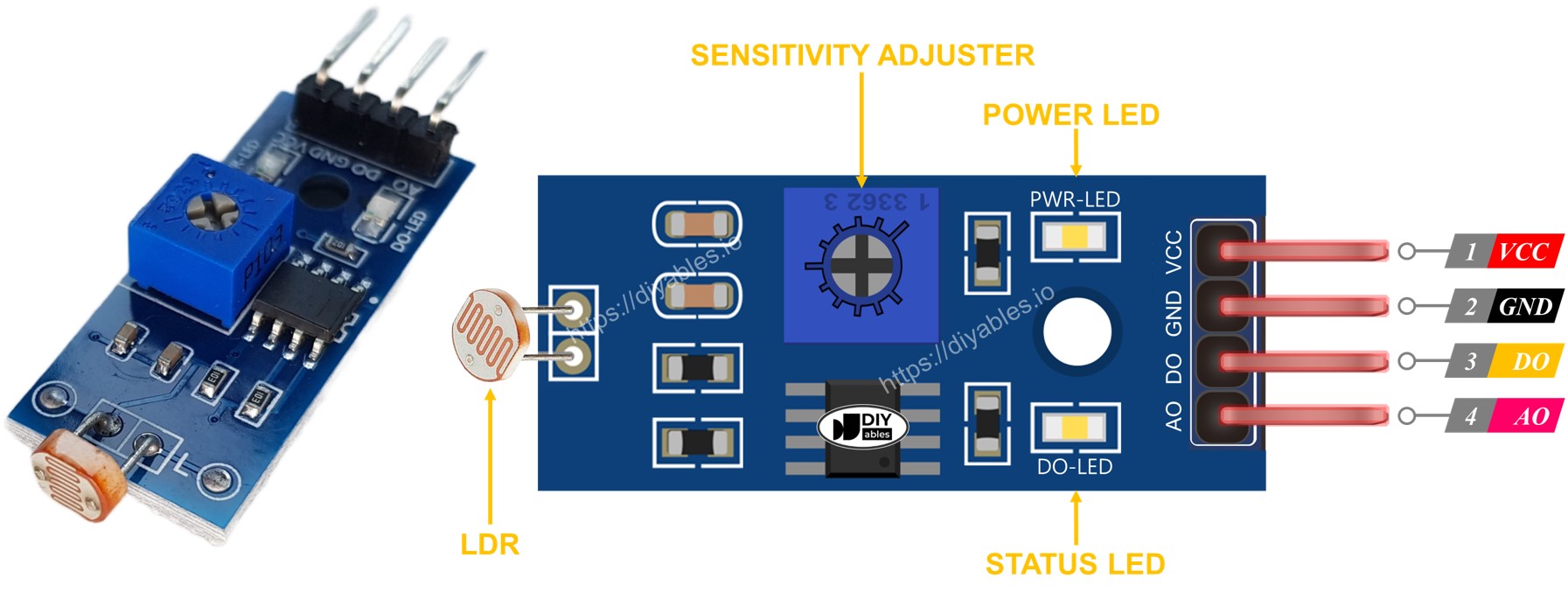
Additionally, the LDR light sensor module includes two LED indicators:
- The PWR-LED indicator shows the power status.
- The DO-LED indicator reflects the light state on the DO pin: it turns on when there is light and turns off when it is dark.
How It Works
Regarding the DO pin:
- The module comes with a built-in potentiometer that allows you to adjust the sensitivity or light threshold.
- When the light intensity in the surroundings is higher than the threshold value (considered as light), the sensor's output pin becomes LOW, and the DO-LED turns on.
- When the light intensity in the surroundings is lower than the threshold value (considered as dark), the sensor's output pin becomes HIGH, and the DO-LED turns off.
Regarding the AO pin:
- The value read from the AO pin decreases as the light intensity in the surroundings increases (more light).
- The value read from the AO pin increases as the light intensity in the surroundings decreases (less light).
Note that adjusting the potentiometer does not affect the value read from the AO pin.
Wiring Diagram
Since the light sensor module has two outputs, you can choose to use one or both of them, depending on what you need.
- The wiring diagram between Arduino Nano and the LDR light sensor module when using DO only.
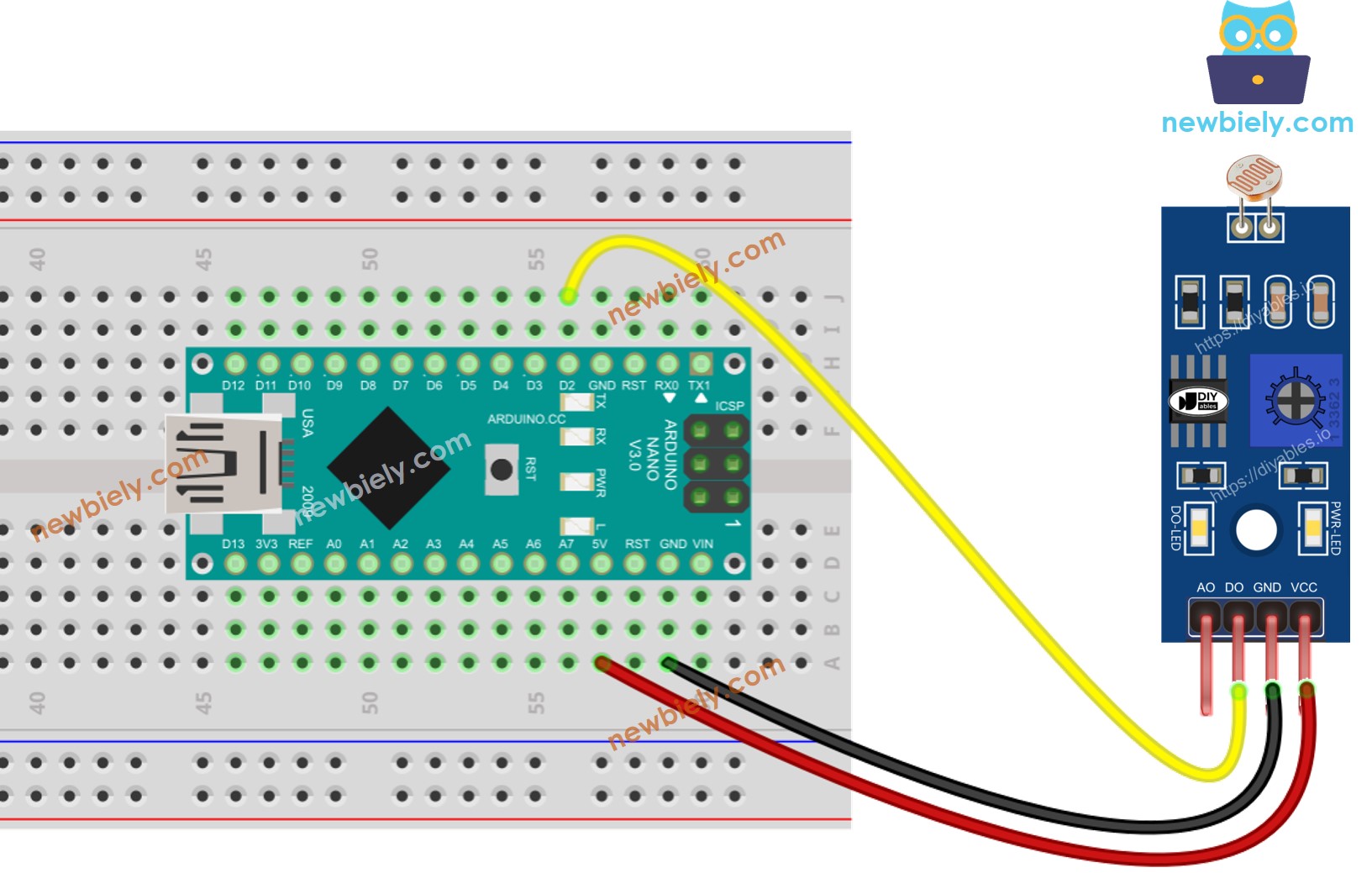
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between Arduino Nano and the LDR light sensor module when using AO only.
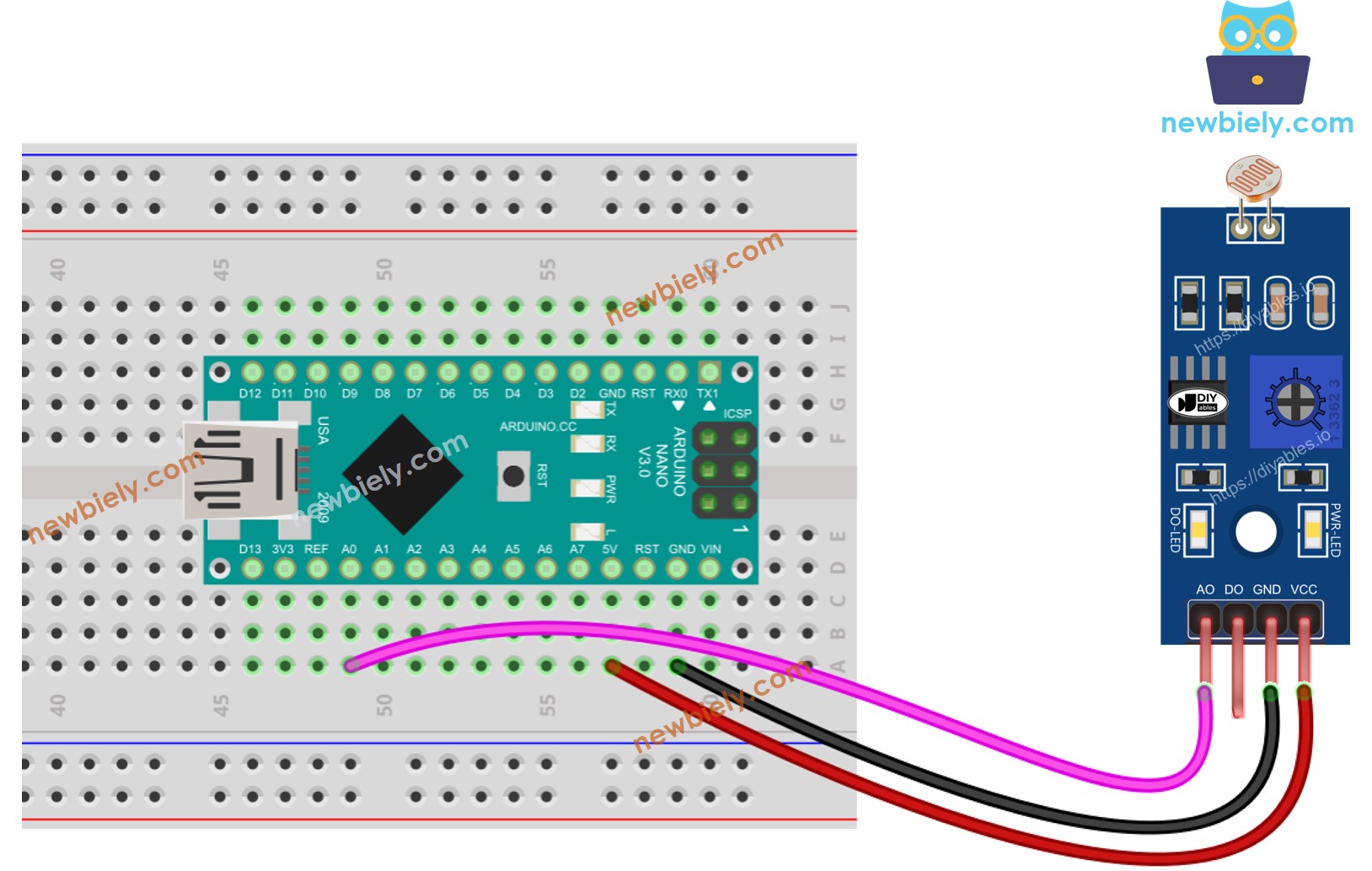
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between Arduino Nano and the LDR light sensor module when using both AO an DO.
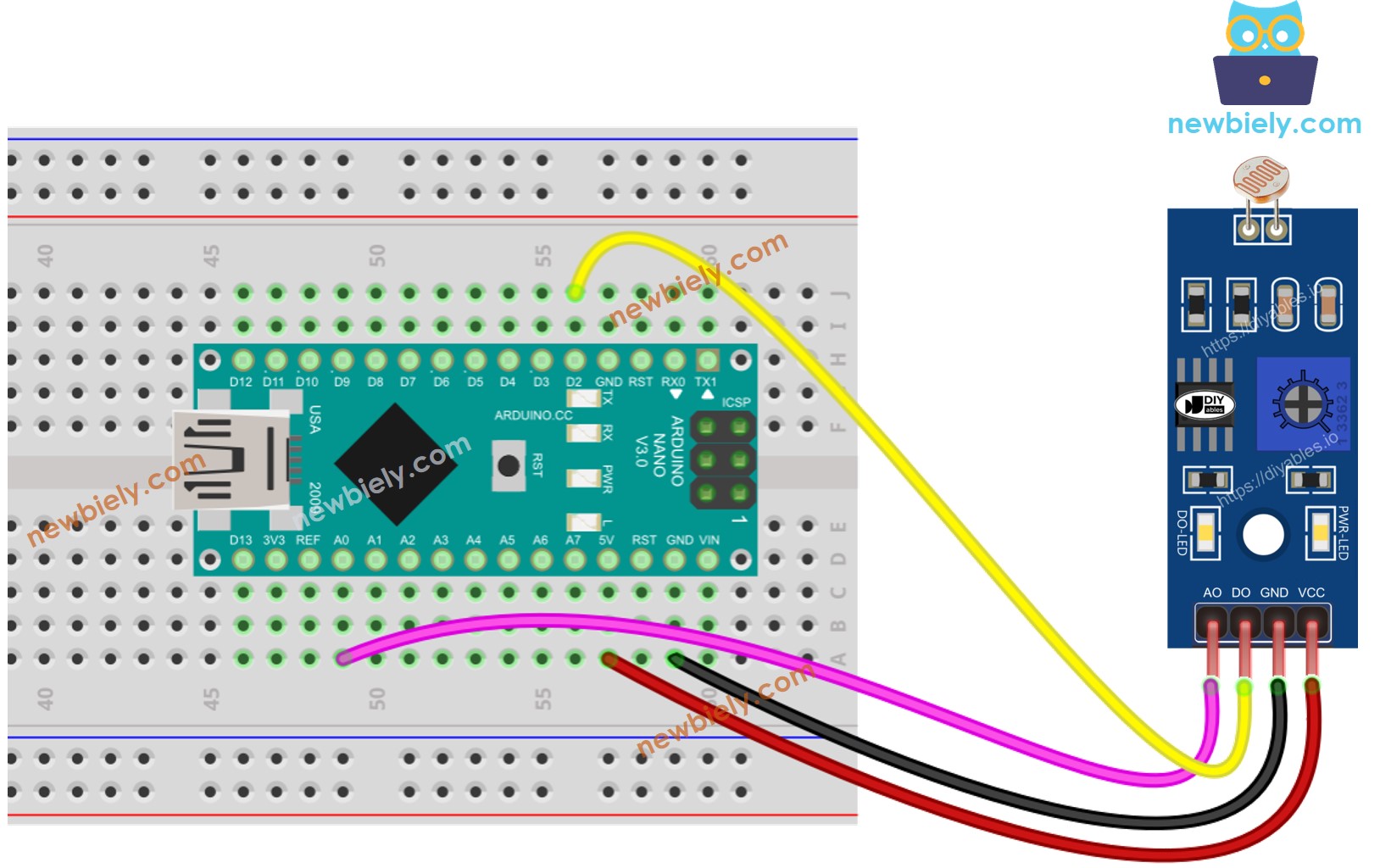
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Arduino Nano Code - Read value from DO pin
Detailed Instructions
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino Nano
- Cover and uncover the LDR light sensor module by your hand or something
- Check out the result on the Serial Monitor.
If you observe that the LED status remains constantly on or off, regardless of the presence of light, you have the option to adjust the potentiometer. This adjustment allows you to finely tune the light sensitivity of the sensor.
Furthermore, it is possible to customize the code to activate an LED or a light when light is detected. Alternatively, you can make a servo motor rotate. For detailed instructions and additional information, please refer to the tutorials provided at the end of this guide.
Arduino Nano Code - Read value from AO pin
Detailed Instructions
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino Nano
- Cover and uncover the LDR light sensor module by your hand or something
- Check out the result on the Serial Monitor.