Arduino Nano Control LED via Bluetooth
This tutorial instructs you how to program Arduino Nano to control a LED through either Bluetooth or BLE.
- To control the LED via Bluetooth, the HC-05 Bluetooth module should be used.
- For controlling the LED via BLE, the HM-10 BLE module is the one to use.
This tutorial gives instructions for both modules.
We will use the Bluetooth Serial Monitor App on a smartphone to send commands to Arduino Nano.
These commands include:
- LED ON which will turn on the LED
- LED OFF which will turn off the LED
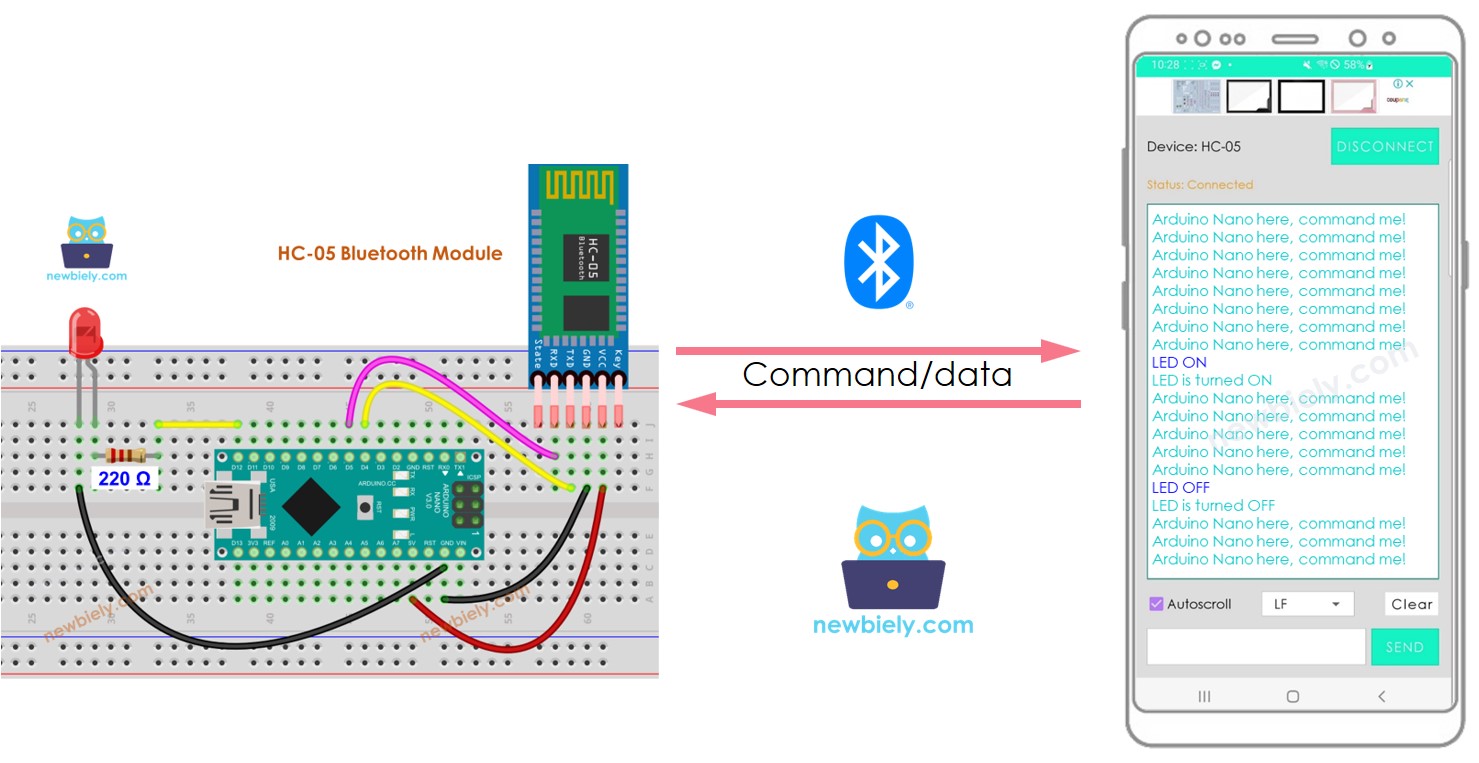
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of LED and Bluetooth Module
If you are unfamiliar with LED and Bluetooth Module (pinout, how it works, how to program ...), the following tutorials can help you:
Wiring Diagram
- If you desire to manage LED through Bluetooth, the HC-05 Bluetooth module should be used in accordance with the wiring diagram below.
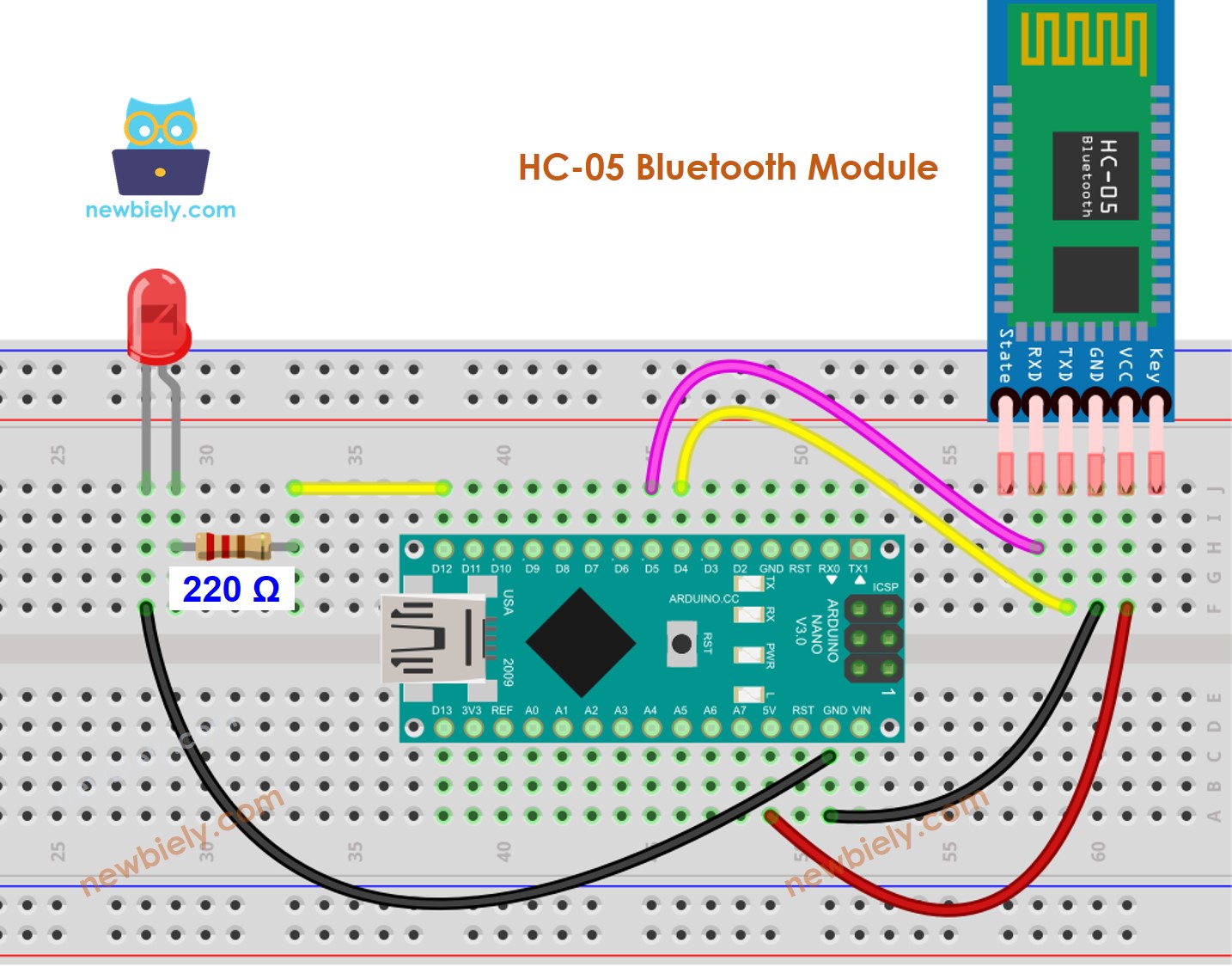
This image is created using Fritzing. Click to enlarge image
- If you wish to manipulate an LED through BLE, the HM-10 BLE module should be utilized in accordance with the wiring diagram below.
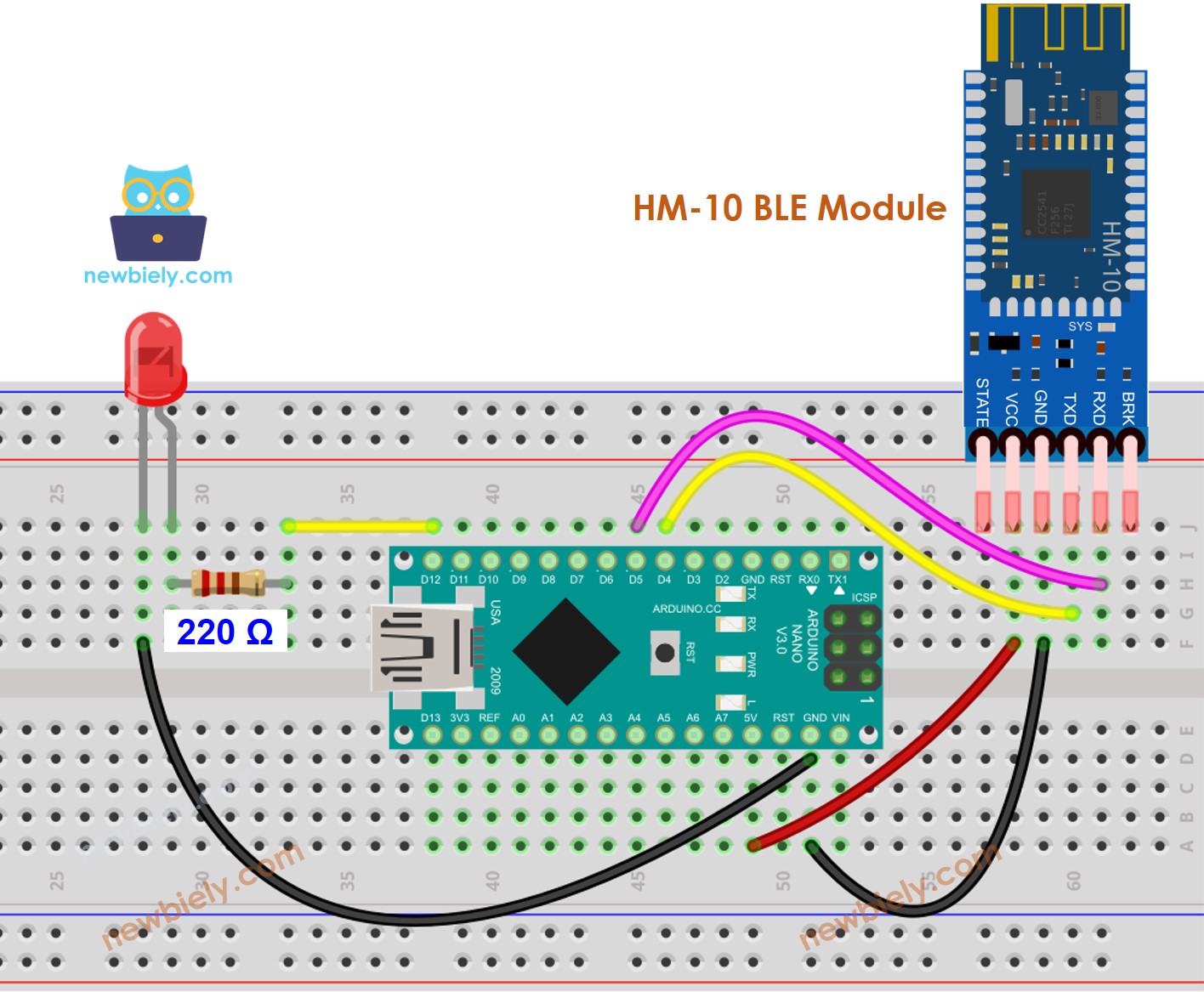
This image is created using Fritzing. Click to enlarge image
Arduino Nano Code - controls LED via Bluetooth/BLE
The code functions for both the HC-10 Bluetooth module and the HM-10 BLE module. It is applicable to both.
Detailed Instructions
- Download the Bluetooth Serial Monitor App to your smartphone.
- Open the code in Arduino IDE and click the Upload button to upload it to the Arduino Nano. If you have trouble uploading, disconnect the TX and RX pins from the Bluetooth module, upload the code, and then reconnect them.
- Launch the Bluetooth Serial Monitor App on your phone and select Classic Bluetooth or BLE according to the module you used.
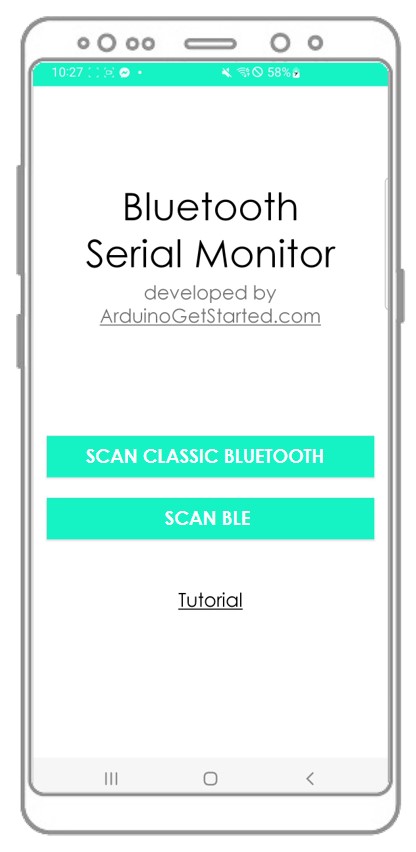
- Connect the Bluetooth App to the HC-05 Bluetooth module or HM-10 BLE module.
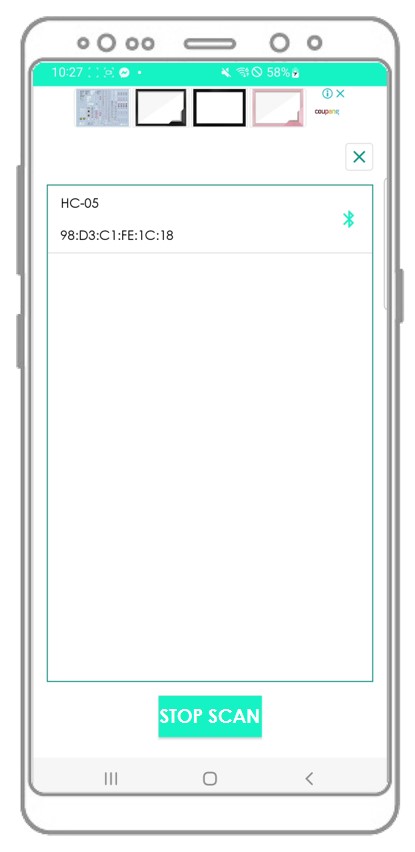
- Enter either “LED ON” or “LED OFF” and press the Send button.
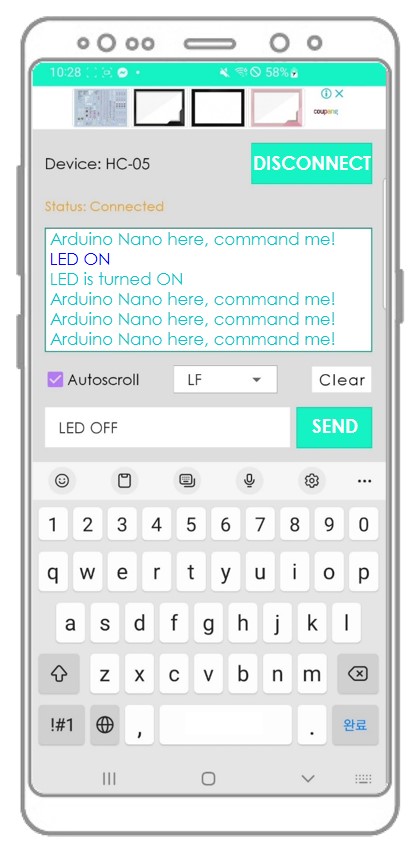
- Check out the LED's state on the Arduino Nano board. It will be either ON or OFF.
- Additionally, we can view the LED's state on the Bluetooth App.
- Finally, check the outcome on the Android App.
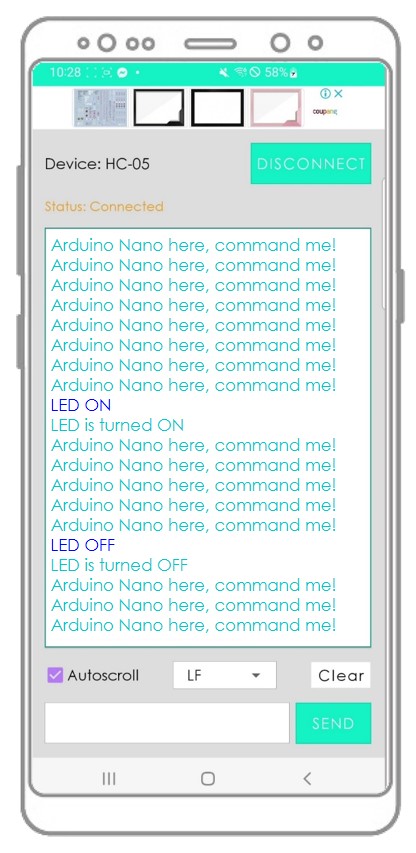
You may be curious as to how Arduino Nano can interpret a full command? For example, when we send “OFF”, how does Arduino Nano determine whether the command is “O”, “OF” or “OFF”?
When sending a command, the Bluetooth App adds a newline character ('\n') by choosing the “newline” option on the App. Arduino Nano will read data until it encounters the newline character. The newline character serves as a command separator.
If you find the Bluetooth Serial Monitor app helpful, kindly give it a 5-star rating on Play Store. We would be grateful for your support. Thank you!