Arduino Nano - Button - Servo Motor
In this tutorial, you will learn how to control a servo motor using an Arduino Nano and a button. When the button is pressed, the servo motor will rotate 90 degrees. If the button is pressed again, the servo motor will return to 0 degrees. This action will repeat continuously.
This tutorial has two sections:
Or you can buy the following sensor kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand, DIYables.
If you are unfamiliar with servo motors and buttons (including pinouts, how they work, and how to program them), the following tutorials can help:
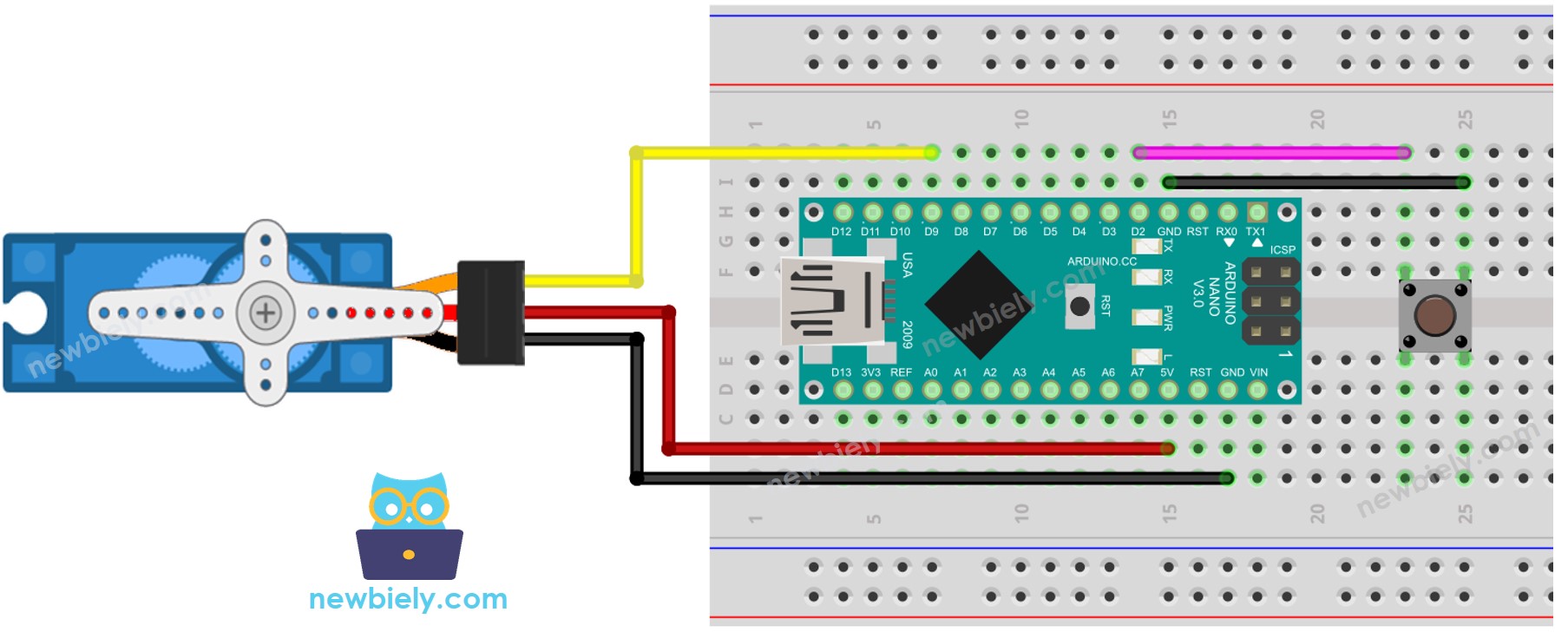
This image is created using Fritzing. Click to enlarge image
It should be noted that the wiring diagram shown above is only suitable for a servo motor with low torque. In case the motor vibrates instead of rotating, an external power source must be utilized to operate the servo motor. The following wiring diagram demonstrates how to connect the servo motor to an external power source.
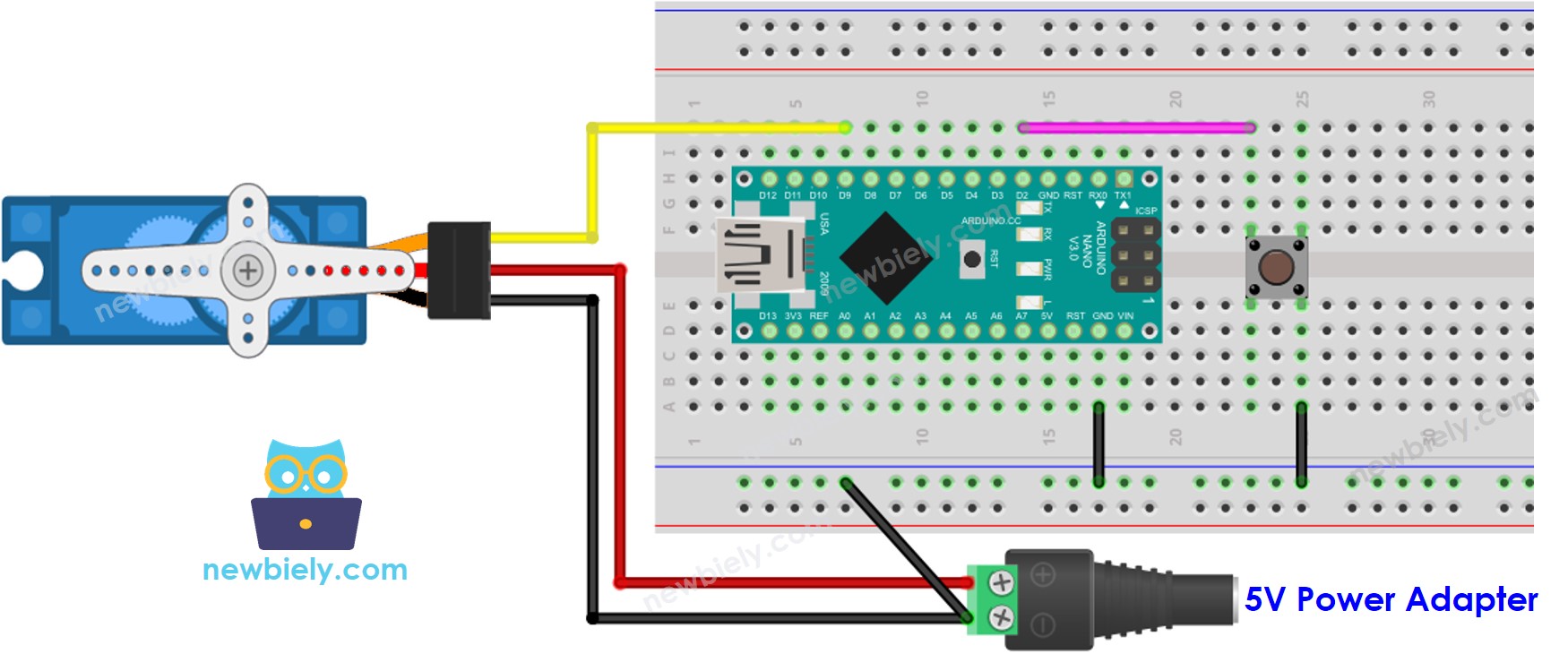
This image is created using Fritzing. Click to enlarge image
Please do not forget to connect GND of the external power to GND of Arduino.
#include <Servo.h>
const int BUTTON_PIN = 2;
const int SERVO_PIN = 9;
Servo servo;
int angle = 0;
int prev_button_state;
int button_state;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
servo.attach(SERVO_PIN);
servo.write(angle);
button_state = digitalRead(BUTTON_PIN);
}
void loop() {
prev_button_state = button_state;
button_state = digitalRead(BUTTON_PIN);
if(prev_button_state == HIGH && button_state == LOW) {
Serial.println("The button is pressed");
if(angle == 0)
angle = 90;
else
if(angle == 90)
angle = 0;
servo.write(angle);
}
}
Plug a USB cable into your Arduino Nano and PC.
Launch the Arduino IDE, select the correct board and port.
Paste the code into the IDE and open it.
Click the Upload button in the IDE to send the code to the Arduino Nano.
Push the button multiple times.
Check out the servo motor's movement.
※ NOTE THAT:
In practice, the code mentioned above does not always work correctly. To ensure it functions properly, we need to debounce for the button. Debouncing the button can be difficult for those new to the topic. Fortunately, with the help of the ezButton library, this task can be made much simpler.
Why is debouncing necessary? See the Arduino Nano - Button Debounce tutorial for more information.
#include <Servo.h>
#include <ezButton.h>
const int BUTTON_PIN = 2;
const int SERVO_PIN = 9;
ezButton button(BUTTON_PIN);
Servo servo;
int angle = 0;
void setup() {
Serial.begin(9600);
button.setDebounceTime(50);
servo.attach(SERVO_PIN);
servo.write(angle);
}
void loop() {
button.loop();
if(button.isPressed()) {
Serial.println("The button is pressed");
if(angle == 0)
angle = 90;
else
if(angle == 90)
angle = 0;
servo.write(angle);
}
}
Install the ezButton library. Refer to
How To for instructions.
Open the code in the Arduino IDE.
Click the Upload button in the Arduino IDE to upload the code to the Arduino Nano.
Push the button multiple times.
Check out the changes in the servo motor.