Arduino Nano - Temperature Humidity Sensor - OLED
This tutorial instructs you how to read the temperature and humidity from a DHT11/DHT22 sensor and display it on an OLED.
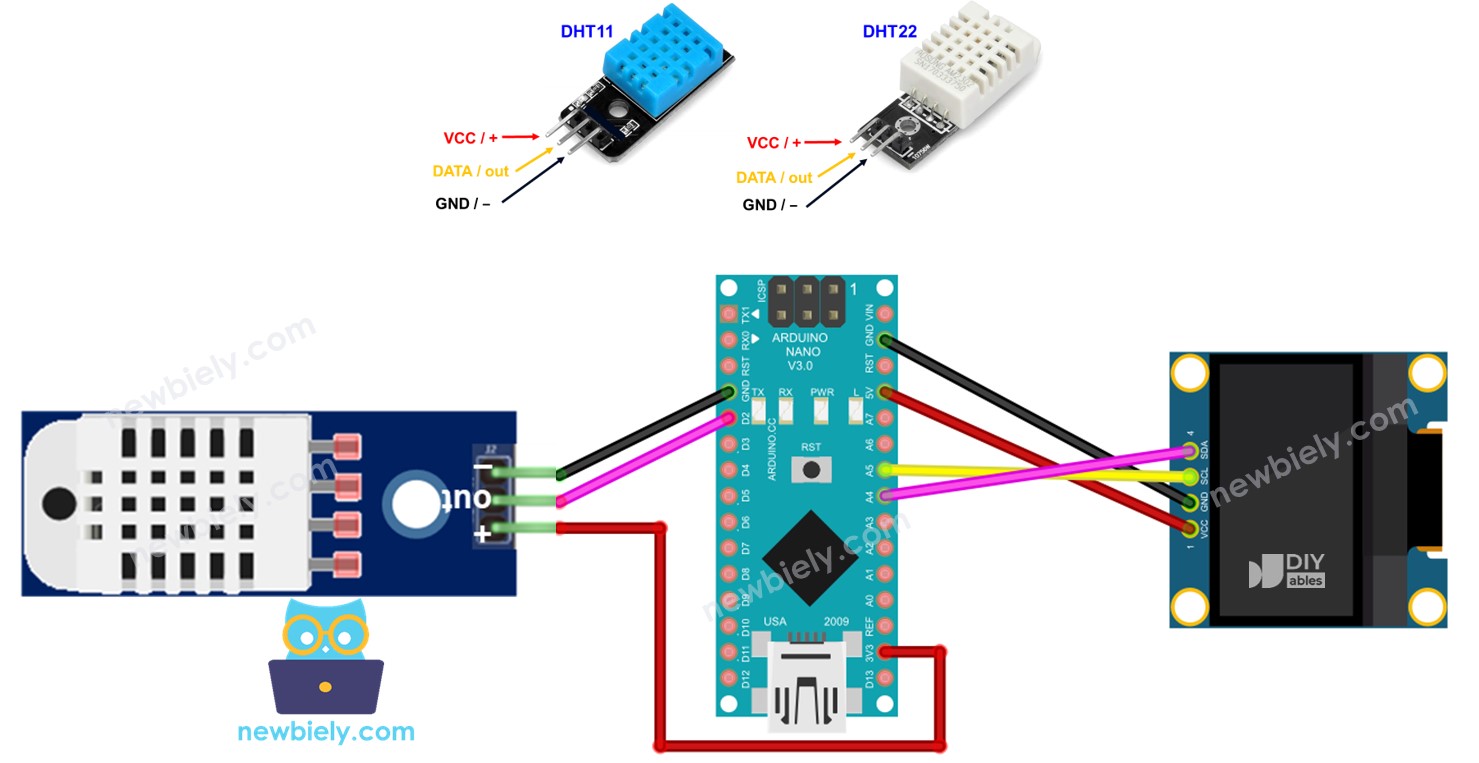
Hardware Preparation
You can use DHT22 sensor instead of DHT11 sensor.
1 | × | Recommended: Screw Terminal Expansion Board for Arduino Nano | |
1 | × | Recommended: Breakout Expansion Board for Arduino Nano | |
1 | × | Recommended: Power Splitter for Arduino Nano |
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of OLED display, DHT11 and DHT22 Temperature Humidity Sensor
If you are unfamiliar with OLED display, DHT11 and DHT22 temperature humidity sensor (pinout, functionality, programming ...), the following tutorials can help you:
- Arduino Nano - OLED tutorial
Wiring Diagram
Arduino Nano - DHT11 Module LCD Wiring
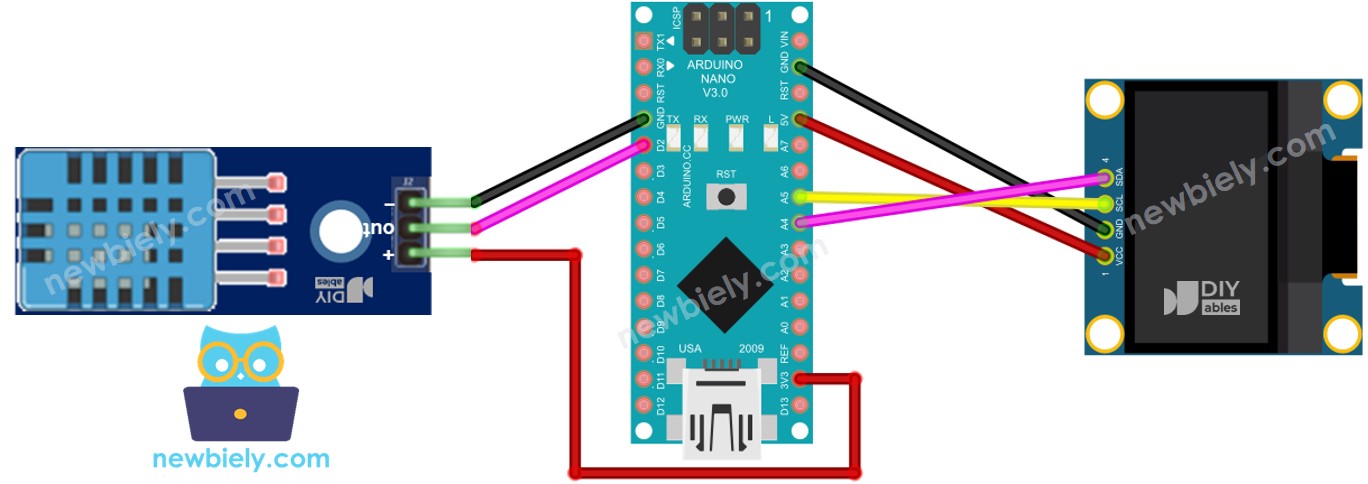
This image is created using Fritzing. Click to enlarge image
Arduino Nano - DHT22 Module LCD Wiring
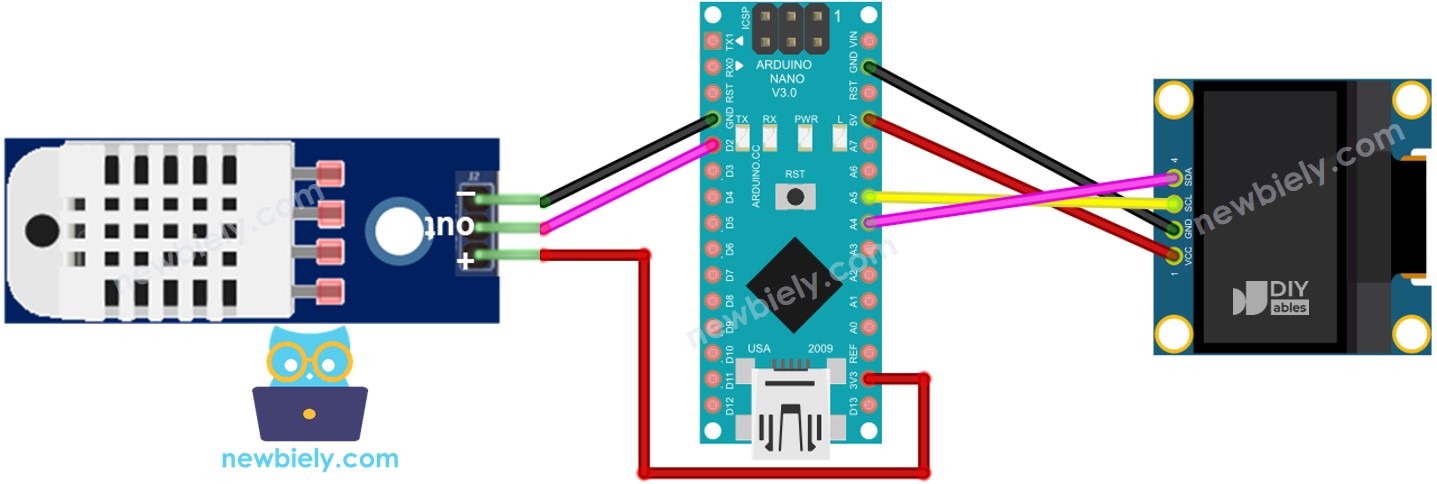
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Arduino Nano Code - DHT11 Sensor - OLED
Detailed Instructions
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Look for “SSD1306” and then locate the SSD1306 library from Adafruit.
- Press the Install button to install the library.
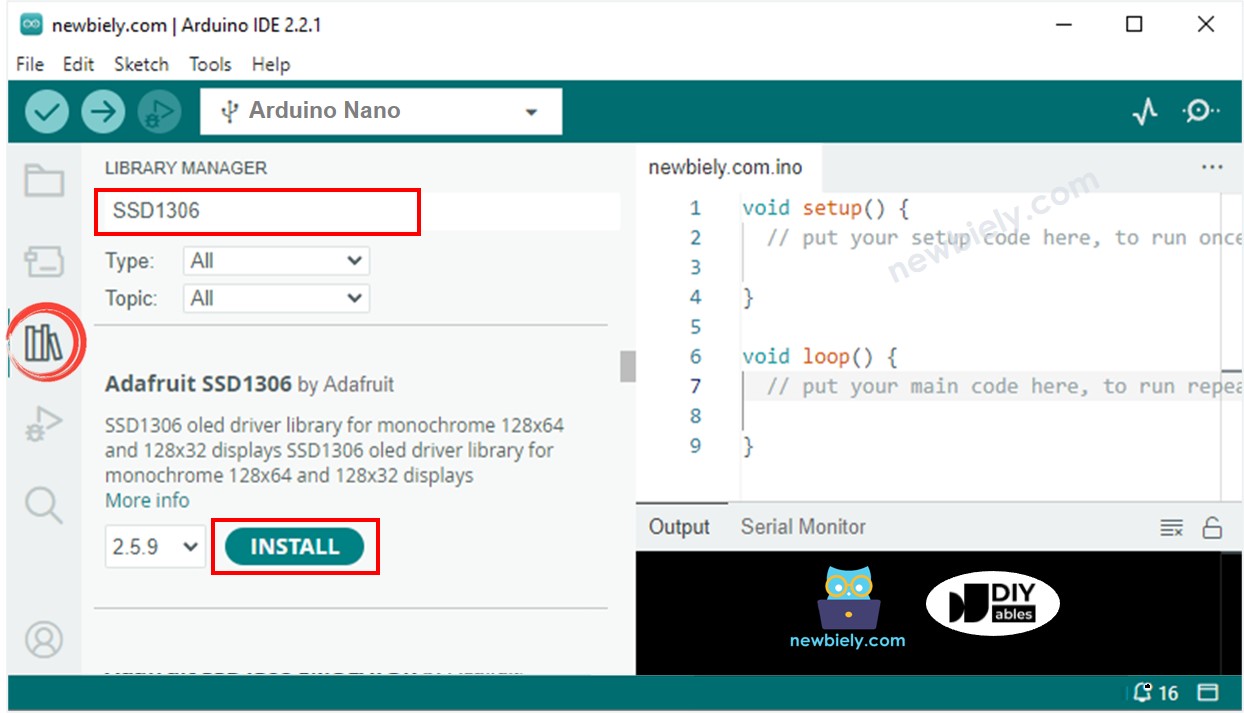
- You will be requested to install some other library dependencies.
- Press the Install All button to complete the installation of all library dependencies.
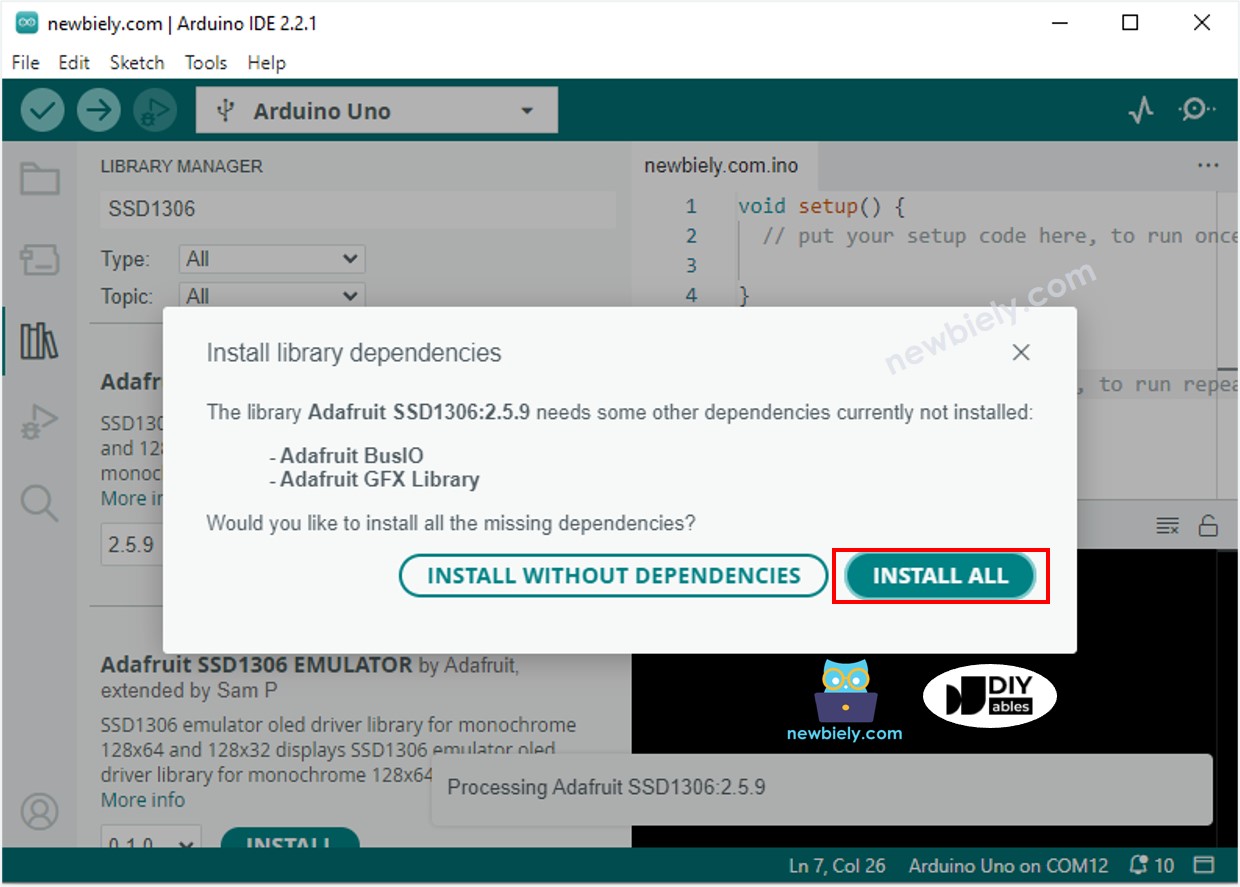
- Search for “DHT” and locate the Adafruit DHT sensor library.
- Press the Install button to install the library.
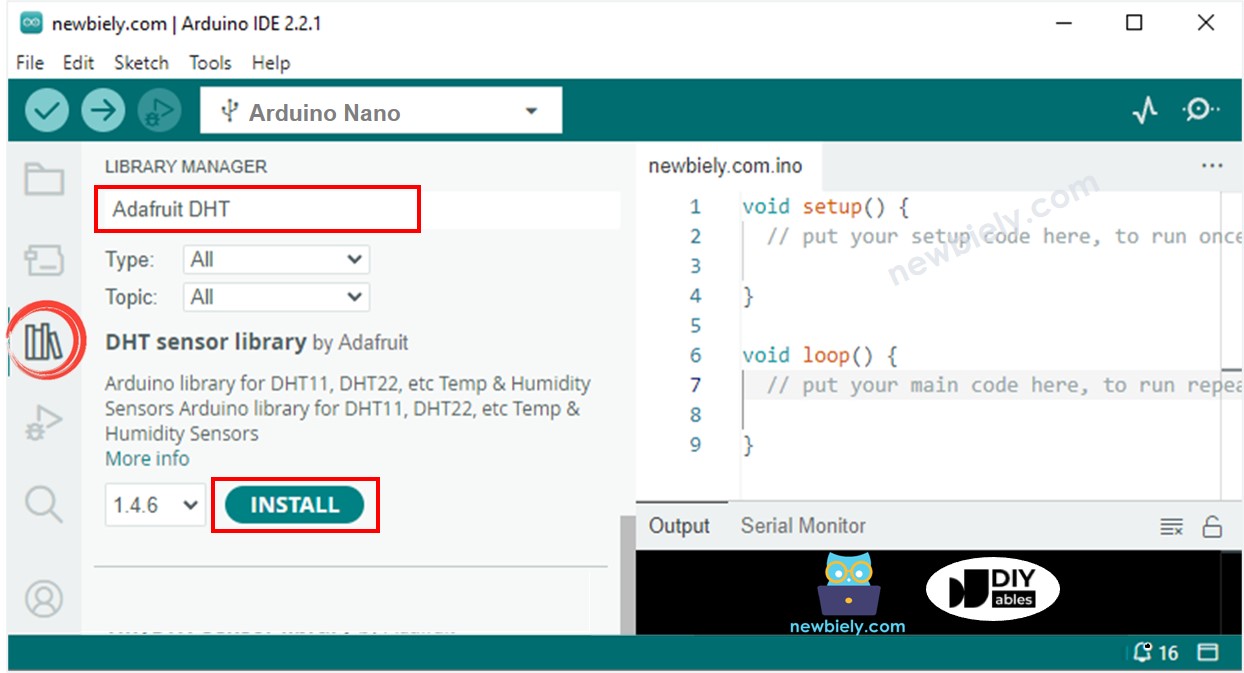
- You will be requested to install some other library dependencies.
- Click the Install All button to install all of the library dependencies.
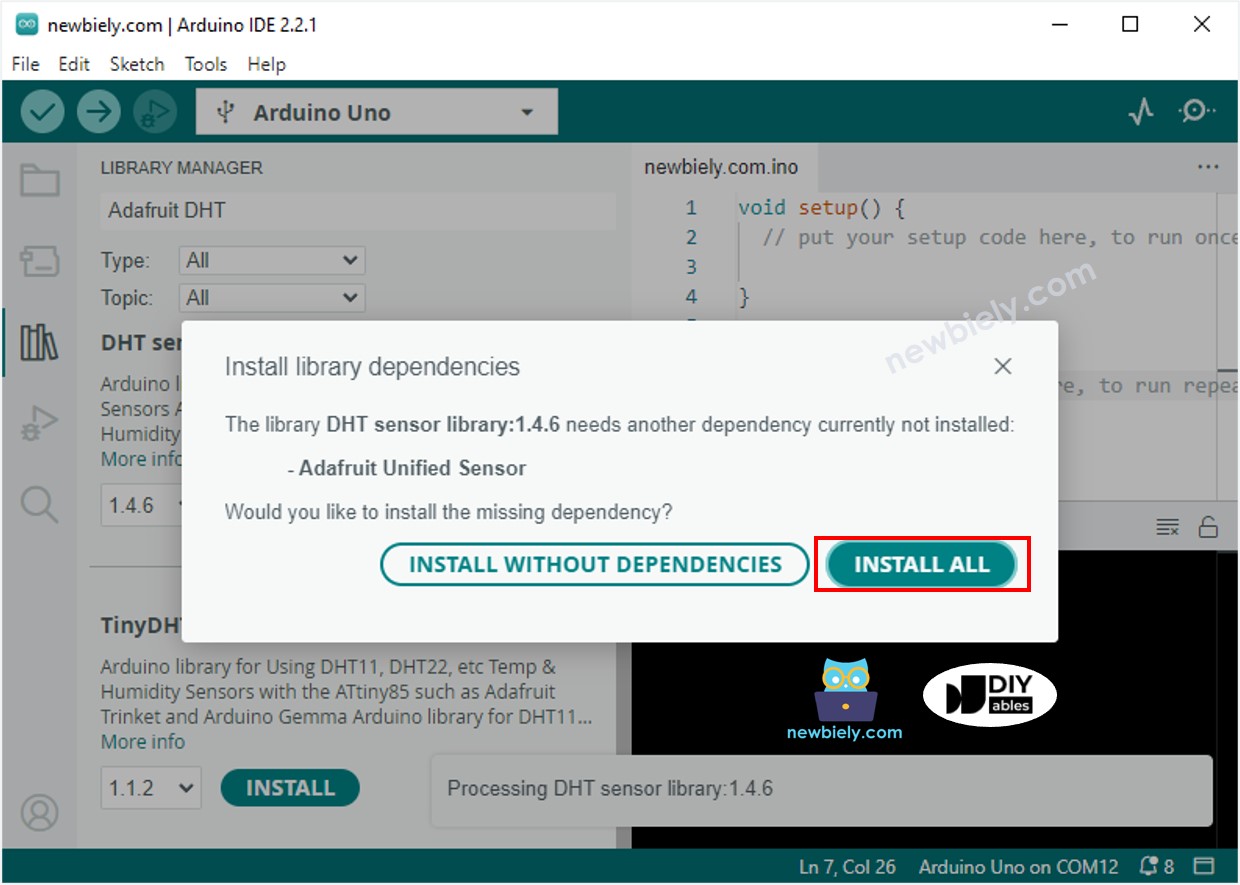
- Copy the code above and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to the Arduino Nano.
- Place the sensor in hot and cold water, or hold it in your hand.
- Check the result on the OLED and Serial Monitor.
※ NOTE THAT:
The code in question automatically centers the text both horizontally and vertically on an OLED display.
Arduino Nano Code - DHT22 Sensor - OLED
※ NOTE THAT:
The code for DHT11 and DHT22 is the same, apart from one line. The library used for both is also the same.