Arduino Nano - Car
This tutorial instructs you how to build a IR-remote-controlled car use Arduino Nano, and IR remote controller.
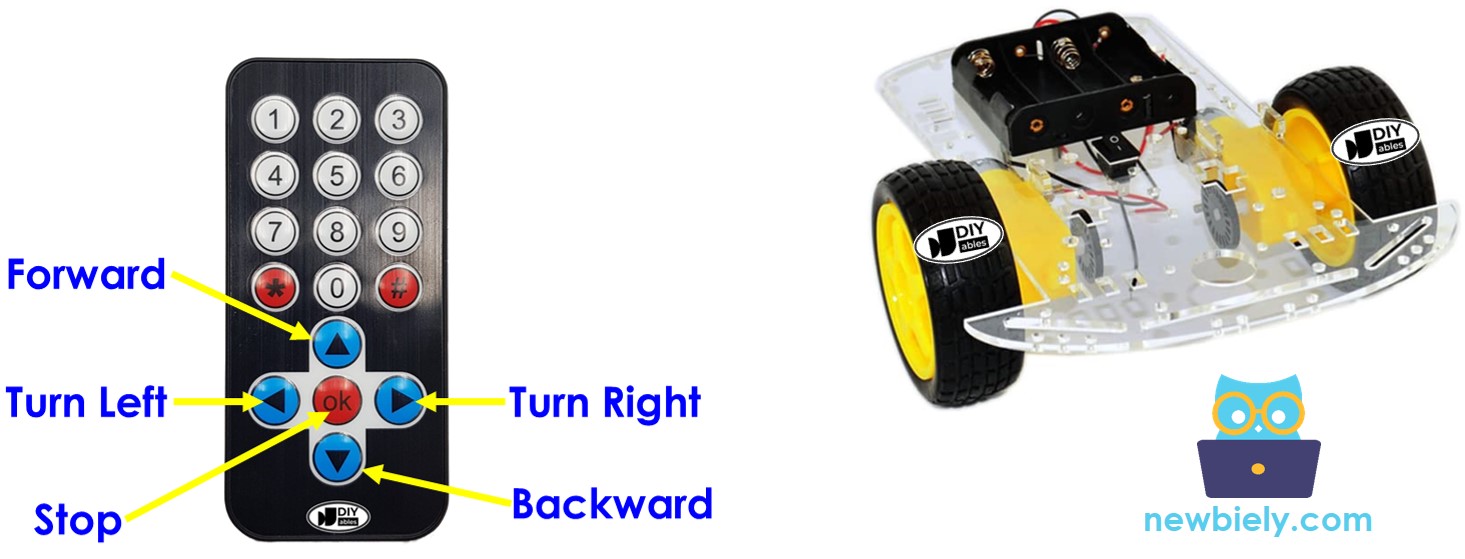
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Robot Car
In the world of Arduino Nano, the robot car is commonly known as the RC car, remote control car, smart car, or DIY car. This nifty creation can be controlled from a distance using either an IR remote controller or a smartphone app via Bluetooth/WiFi. It can make sharp turns to the left or right and move forward or backward with ease.
A 2WD (Two-Wheel Drive) vehicle for Arduino Nano is a small robot car that you can assemble and operate using an Arduino Nano board. It usually consists of the following essential parts:
- Chassis: This is like the car's body, where you attach all the other components.
- Wheels: These are the two wheels that make the car move. They are connected to two DC motors.
- Motors: Two DC motors are used to drive the two wheels.
- Motor Driver: The motor driver board is a crucial component that connects the Arduino Nano to the motors. It takes signals from the Arduino Nano and gives power and control to the motors.
- Arduino Nano Board: This is the car's brain. It reads inputs from sensors and user commands and controls the motors accordingly.
- Power Source: The 2WD car needs a power source, usually batteries and a battery holder, to provide power to the motors and the Arduino Nano board.
- Wireless Receiver: This is an infrared, Bluetooth, or WiFi module that enables wireless communication with a remote control or smartphone.
- Optional Components: Depending on how advanced you want your project to be, you can add various optional parts like sensors (for example, ultrasonic sensors for avoiding obstacles or line-following sensors), and more.
In this tutorial, to make it simple, we will use:
- 2WD Car kit (including Chassis, wheels, motors, battery holder)
- L298N Motor Driver
- IR infrared kit (including IR controller and IR receiver)
Check the hardware list at the top of this page.
How It Works
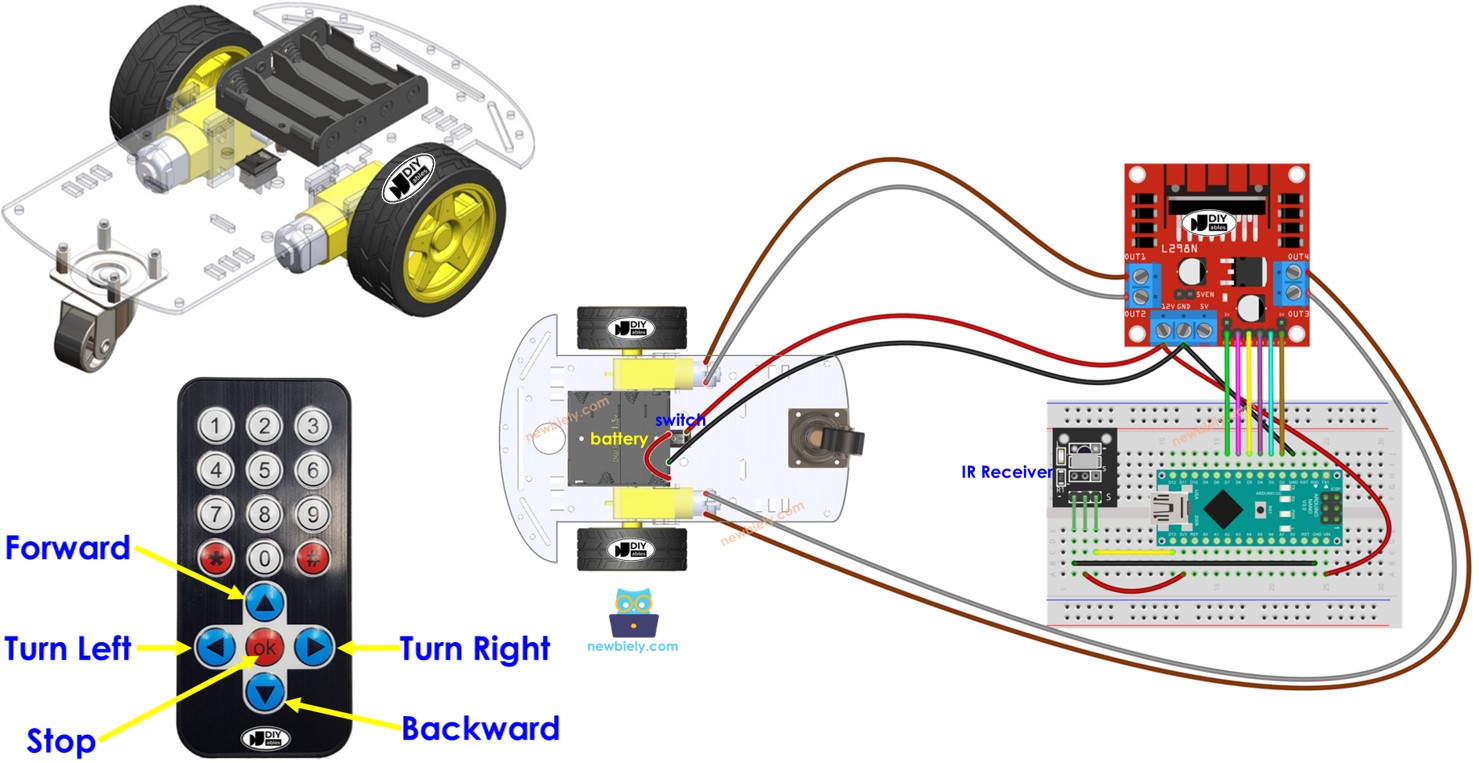
- Arduino Nano connects to the DC motors of the robot car through L298N motor driver module.
- Arduino Nano connects to an IR receiver.
- The battery powers Arduino Nano, DC motors, motor driver, and IR receiver.
- Users press the UP/DOWN/LEFT/RIGHT/OK keys on the IR remote controller.
- Arduino Nano receives the UP/DOWN/LEFT/RIGHT/OK commands through the IR receiver.
- Arduino Nano controls the car to move FORWARD/BACKWARD/LEFT/RIGHT/STOP by controlling the DC motor via the motor driver.
Wiring Diagram
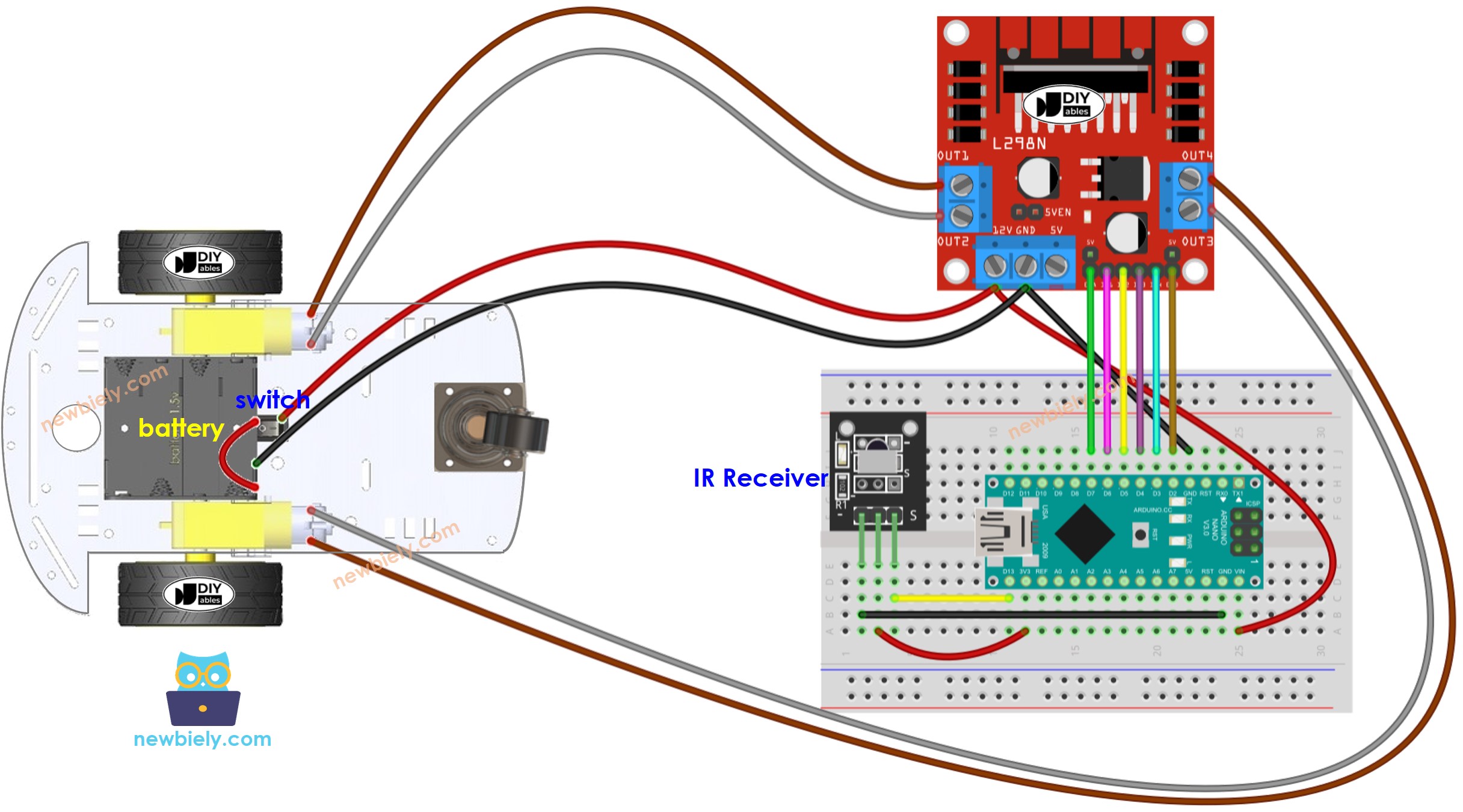
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Usually, we require two sources of power:
- One for the motor (indirectly through the L298N module).
- Another for the Arduino Nano board, L298N module, and IR receiver.
However, there's a way to simplify this and use only one power source for everything. You can achieve this by using four 1.5V batteries (totaling 6V). Here's how:
- Connect the batteries to the L298N module following the diagram above.
- Remove all of three jumpers on the L298N module.
Arduino Nano Code
Detailed Instructions
- Install DIYables_IRcontroller library on Arduino IDE by following this instruction
- Do the wiring as the diagram shown above.
- Disconnect the wire from the Vin pin on the Arduino Nano because we will power Arduino Nano via USB cable when uploading code.
- Flip the car upside down so that the wheels are on top.
- Connect the Arduino Nano to your computer using the USB cable.
- Copy the provided code and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to transfer the code to the Arduino Nano.
- Use the IR remote controller to make the car go forward, backward, left, right, or stop.
- Check if the wheels move correctly according to your commands.
- If the wheels move the wrong way, swap the wires of the DC motor on the L298N module.
- You can also see the results on the Serial Monitor in the Arduino IDE.
- If everything is working fine, disconnect the USB cable from the Arduino Nano, and connect back the wire to Vin pin to power the Arduino Nano from the battery.
- Flip the car back to its normal position with the wheels on the ground.
- Have fun controlling the car!
Code Explanation
Read the line-by-line explanation in comment lines of code!
You can learn more about the code by checking the following tutorials:
- Arduino Nano - DC motor tutorial
You can extend this project by:
- Adding obstacle avoidance sensors to immidilately stop the car if an obstacle is detected.
- Adding function to control the speed of car (see Arduino Nano - DC motor tutorial). The provided code controls car with full speed.