Arduino Nano - Feedback Actuator
with feedback
In a prior tutorial, we discussed the linear actuator without feedback. Now, we will be exploring the feedback linear actuator (also known as the feedback linear actuator). This type of actuator provides information to determine the position of its stroke and then control it. Specifically, we will be looking at:
- The workings of a feedback linear actuator
- How to identify the position of a feedback linear actuator (in millimeters)
- How to control the position of a feedback linear actuator
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Feedback Linear Actuator
A linear actuator with feedback is available, which provides a signal to identify its position and control it. This feedback is in the form of a potentiometer that outputs a voltage value that is proportional to the stroke's position.
Feedback Linear Actuator Pinout
A Feedback Linear Actuator has 5 wires:
- Actuator Positive wire: This wire is used to control the linear actuator by utilizing high voltage (12V, 24V, 48V...).
- Actuator Negative wire: This wire is used to control the linear actuator by utilizing high voltage (12V, 24V, 48V...).
- 5V wire: this wire is used for the feedback potentiometer. Connect this wire to 5V or 3.3V
- GND wire: this wire is used for the feedback potentiometer. Connect this wire to GND
- Potentiometer wire: (also called feedback wire, or output wire) this wire outputs the voltage value in proportion to the stroke's position.
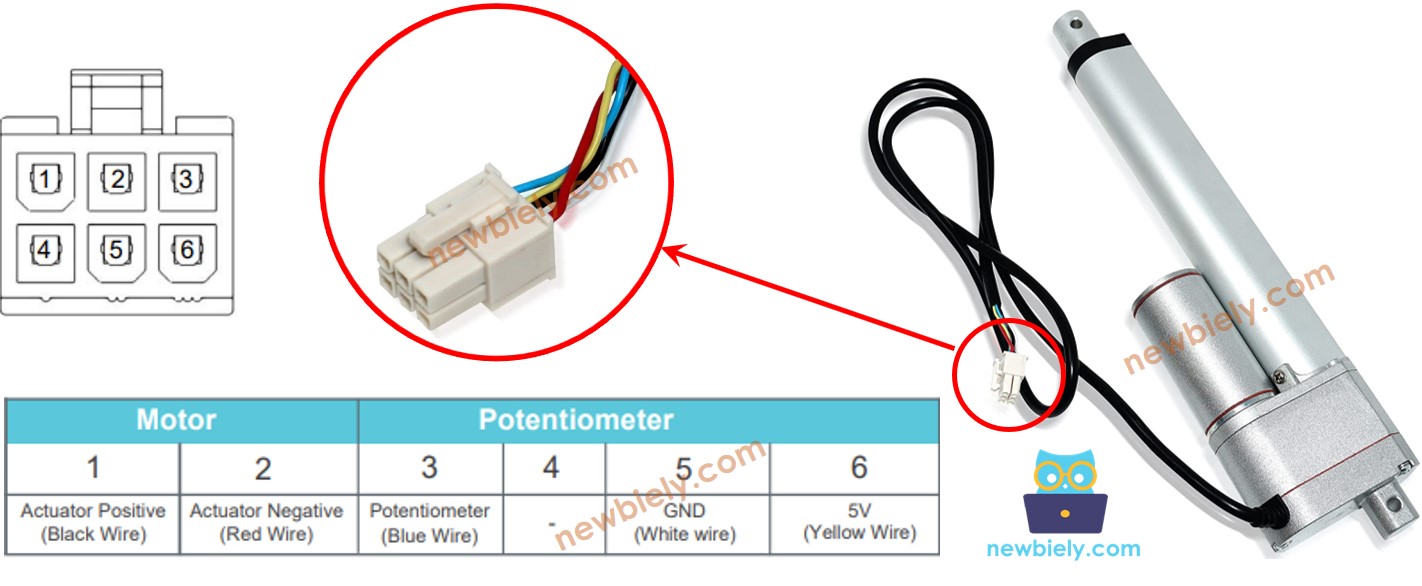
How It Works
If we supply high voltage to the positive and negative wires, the stroke of the actuator will be extended or retracted. Specifically:
- If 12V (12V, 24V, 48V...) is connected to the positive wire and GND to the negative wire, the linear actuator will extend at full speed until it reaches the limit.
- If 12V (12V, 24V, 48V...) is connected to the negative wire and GND to the positive wire, the linear actuator will retract at full speed until it reaches the limit.
- If power is stopped to the actuator (GND to both positive and negative wire), the actuator will cease extending/retracting.
※ NOTE THAT:
- The voltage necessary for operating the actuator is determined by its specifications. To find out the exact voltage value, consult the datasheet or manual.
- Even when power is cut off, the actuator can maintain its position while carrying a load.
The voltage in the potentiometer wire is related to the stroke's position on the actuator. By measuring this voltage, we can determine the stroke's position.
Wiring Diagram
Take out all three jumpers from the L298N module prior to connecting the wires.
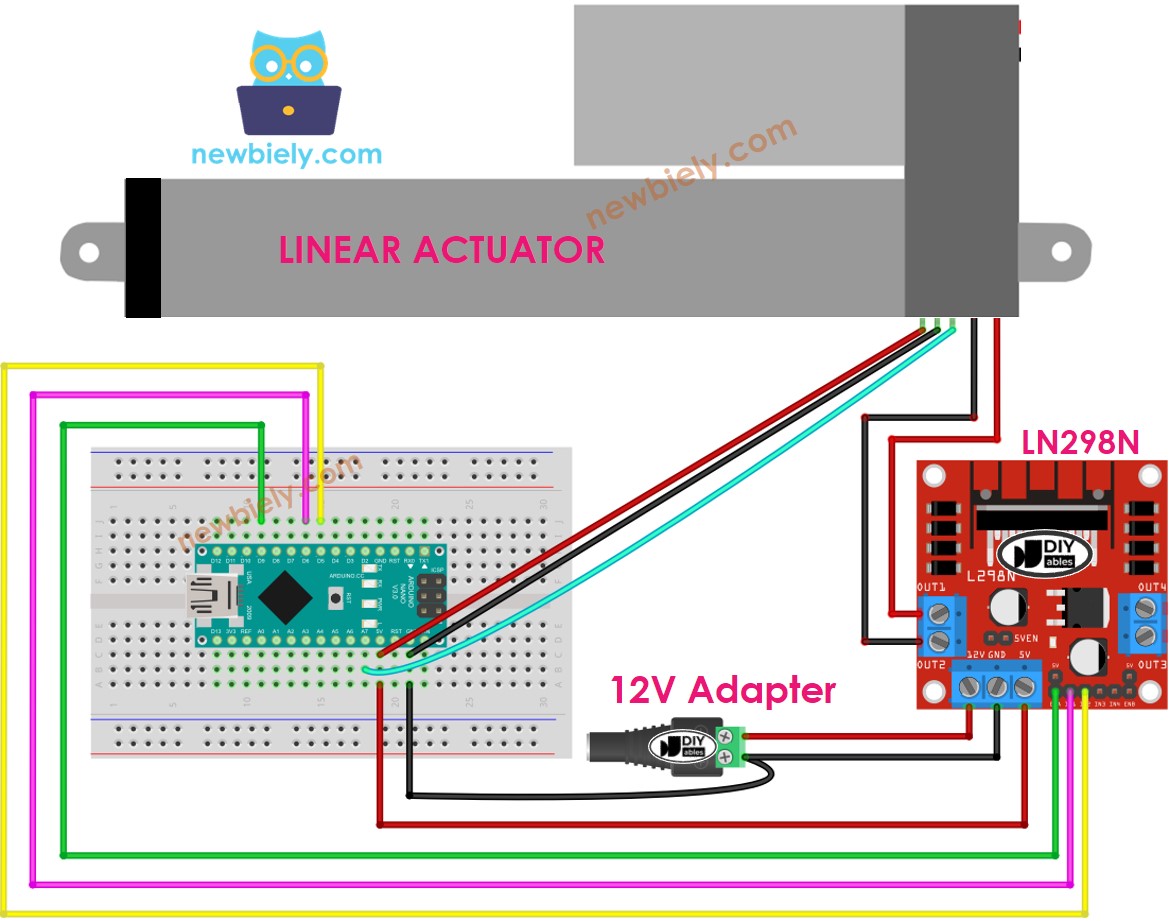
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
How to control extend/retract a linear actuator
Check out the tutorial on Arduino Nano - Actuator at BASE_URL/tutorials/arduino-nano/arduino-nano-actuator.
How to find the position of the linear actuator
This is an example of how to locate the stroke on a linear actuator. It provides an illustration of the process.
Calibration
- Determine the length of the actuator's stroke (in millimeters) by either measuring it with a ruler or consulting the datasheet.
- Run the following code to determine the output values when the linear actuator is fully extended and fully retracted.
- Observe the log on the Serial Monitor as demonstrated in the example below.
Make a note of the following three values and update them in the code below: the minimum value, the maximum value, and IN1_PIN and IN2_PIN. If the minimum and maximum values are swapped, also swap IN1_PIN and IN2_PIN.
Arduino Nano code that calculate the position of the actuator
- Refresh the three calibrated values in the code
- Transfer the code to Arduino Nano
- Check the outcome on Serial Monitor