Arduino Nano - LED
This tutorial instructs you how to use Arduino Nano to control an LED. In detail, we will learn:
- How to connect the LED to Arduino Nano
- How to program Arduino Nano to turn the LED on or off.
- How to program Arduino Nano to blink the LED.
Hardware Preparation
1 | × | Arduino Nano | |
1 | × | USB A to Mini-B USB cable | |
1 | × | LED | |
1 | × | 220 ohm resistor | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Optional) 9V Power Adapter for Arduino Nano | |
1 | × | (Recommended) Screw Terminal Adapter for Arduino Nano |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of LED
The LED Pinout
LED has two pins:
- The Cathode(-) pin: should be connected to the negative of power supply
- The Anode(+) pin: should be connected to the positive of power supply via a resistor
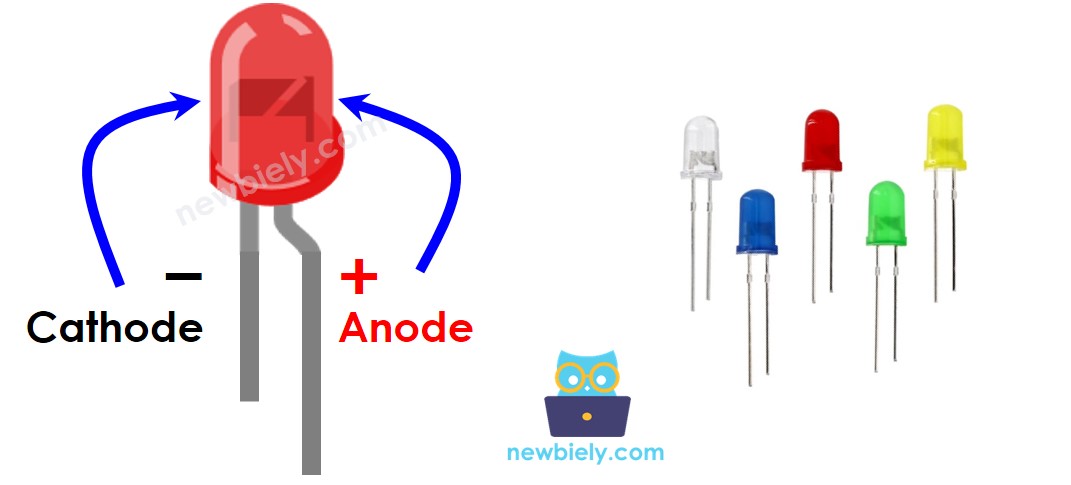
How It Works
The below table shows the LED state according to how the power connects to LED's pin
LED cathode(-) pin | LED anode(+) pin | Condition | LED state |
---|---|---|---|
GND | VCC | via a resistor | ON |
GND | PWM | via a resistor | ON, variable brightness |
GND | GND | any | OFF |
VCC | VCC | any | OFF |
VCC | GND | any | burned! cautious! |
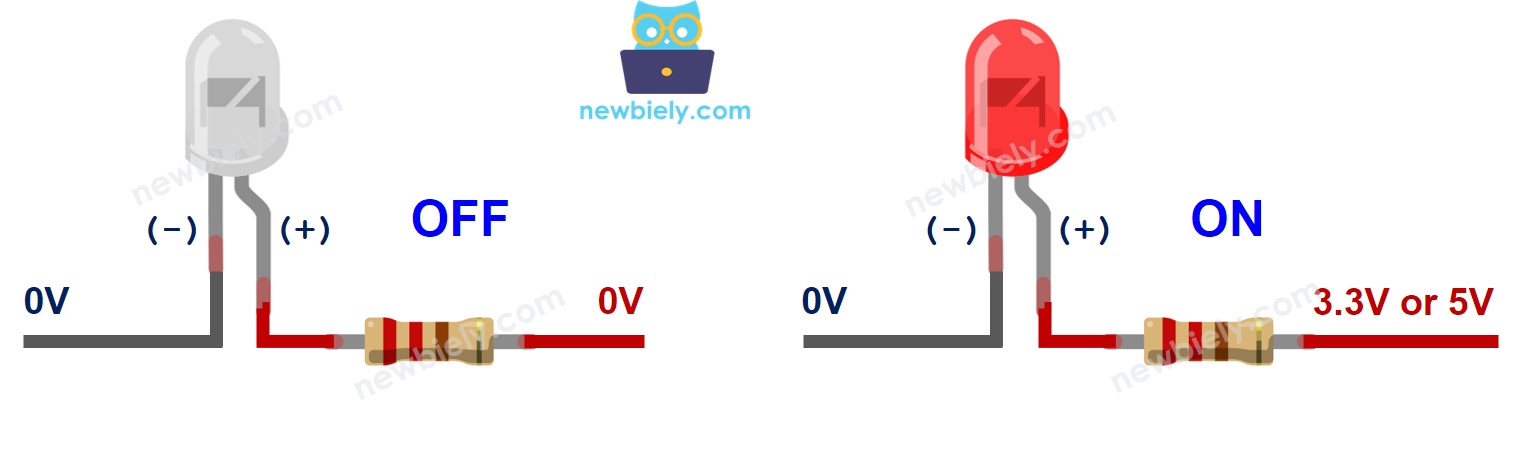
As shown on the above table, by generating a PWM signal to the anode (+) of an LED, the brightness of the LED varies in accordance with the PWM value. This has been explained in detail in Arduino Nano fade LED tutorial.
※ NOTE THAT:
- For most of LED, a resistor is required to protect LED from the current. There are two options to place the resistor: between the anode(+) and VCC, or between the cathode(-) and GND. The value of the resistor depends on the specification of the LED.
- Some kinds of LEDs have a built-in resistor. In this case, the resistor is not required.
Arduino Nano - LED
When an Arduino's pin is set up as a digital output, it can be programmed to have either a GND or VCC voltage. Connect the Arduino's pin to the anode(+) pin of the LED with a resistor. This will enable us to control the LED's state through programming.
Wiring Diagram
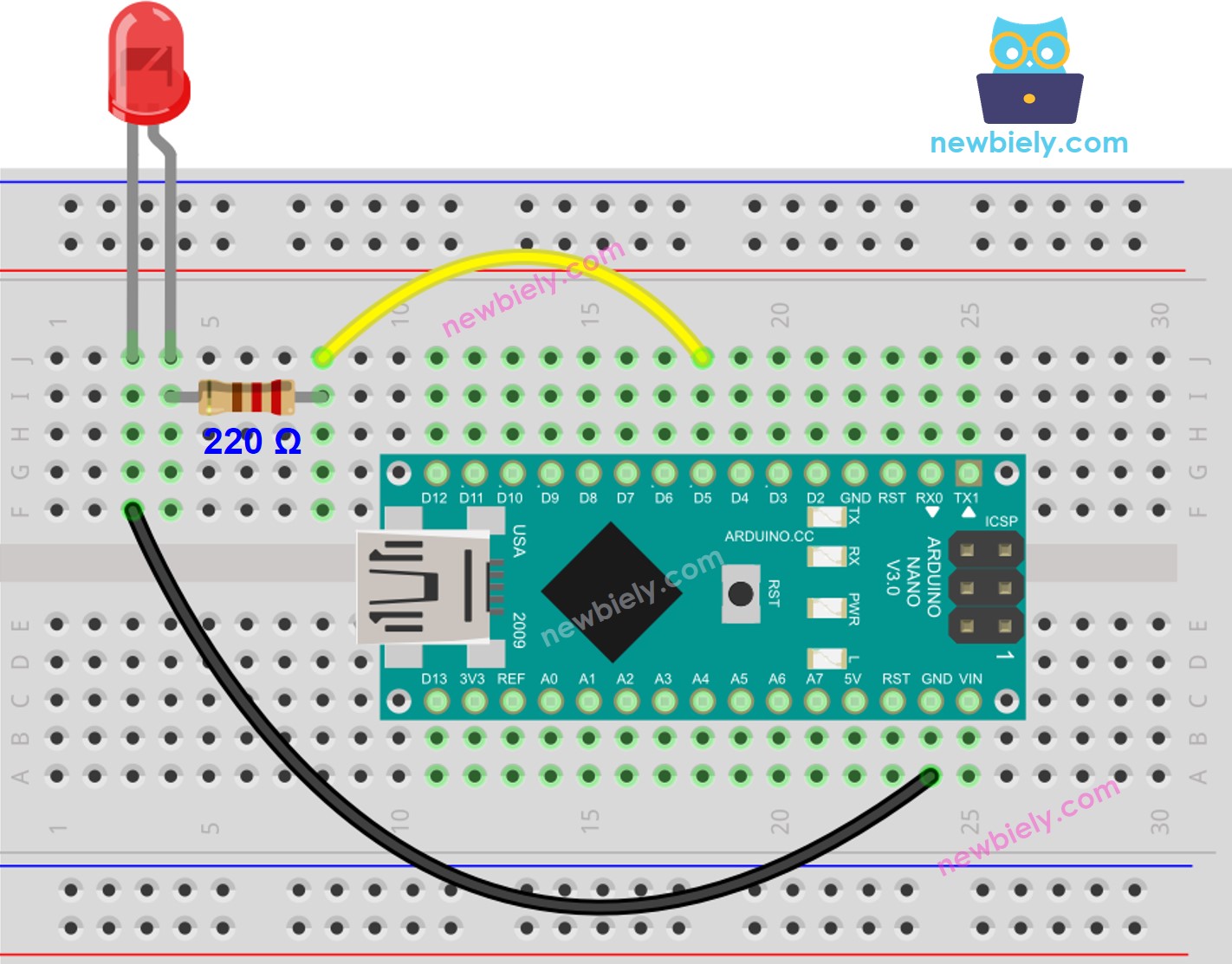
This image is created using Fritzing. Click to enlarge image
How To Program
- Set up an Arduino pin as a digital output using the pinMode() function. For example:
Arduino Nano Code for controlling the LED
The below is a complete code for Arduino Nano that controls the LED
Detailed Instructions
- Connect an Arduino Nano to a computer using a USB cable.
- Open the Arduino IDE, select the appropriate board and port.
- Copy the code above and open it with the Arduino IDE.
- Click the Upload button on the Arduino IDE to compile and upload the code to the Arduino Nano.
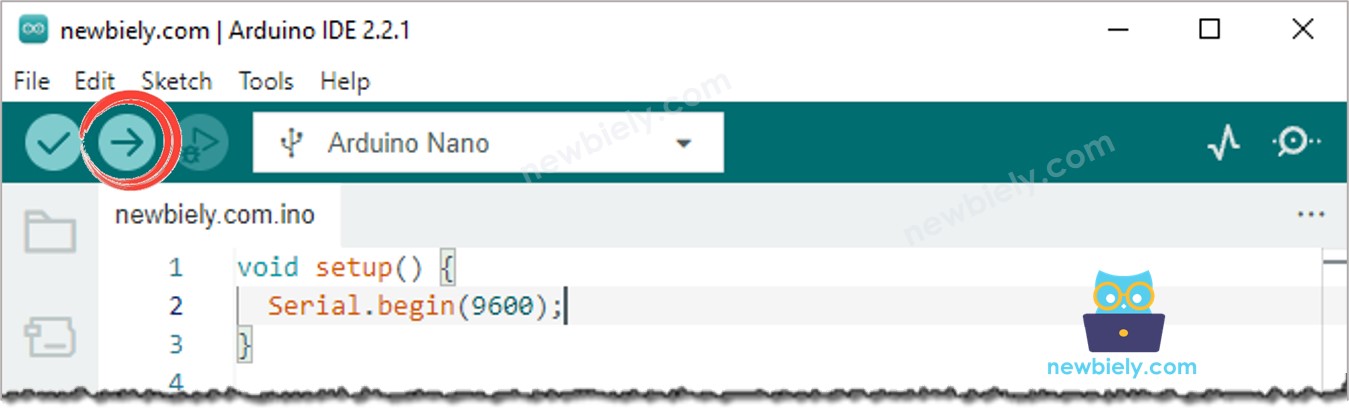
- Check out the outcome: The integrated LED will alternate between being ON and OFF at regular intervals of one second.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
※ NOTE THAT:
The code above makes use of delay(). This function prevents Arduino Nano from carrying out other operations while the delay is in effect. If your project necessitates completing certain tasks, it is best to avoid blocking Arduino Nano by utilizing the non-blocking method for Arduino.
Video Tutorial
Additional Knowledge
- Arduino Nano pins 0 through 13 and pins A0 through A5 can be utilized as an output pin to control an LED. Pin A6, A7 are used for the analog input only
- At one moment, a pin can only handle one job. If you have already used it for something else (e.g., digital input, analog input, PWM, UART...), you should NOT use it as a digital output to control an LED. For instance, if we use the Serial.println() function, we should NOT use pins 0 and 1 for any other purpose since they are used for Serial.
- This tutorial demonstrates how to use the output pin of an Arduino Nano to control an LED. We can use this code to switch ON/OFF any apparatus, including large machines.
- For devices/machines that require a high power supply (greater than 5v) and/or high-current consumption, it is necessary to use a relay between the output pin and the device/machine. Further information can be found in the Arduino Nano - Relay tutorial.