Arduino Nano - Keypad - Servo Motor
This tutorial instructs you how to use Arduino Nano and keypad to control servo motor. In detail:
- When an authorized password is entered on the keypad, Arduino Nano will rotate the servo motor to 90°.
- Subsequently, the Arduino Nano will rotate the servo motor back to 0°.
The Arduino Nano code can accepts multiple passwords.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of Keypad and Servo Motor
If you are not familiar with keypad and servo motor (including pinout, functioning and programming), the following tutorials can help you:
- Arduino Nano - Keypad tutorial
- Arduino Nano - Servo Motor tutorial
Wiring Diagram
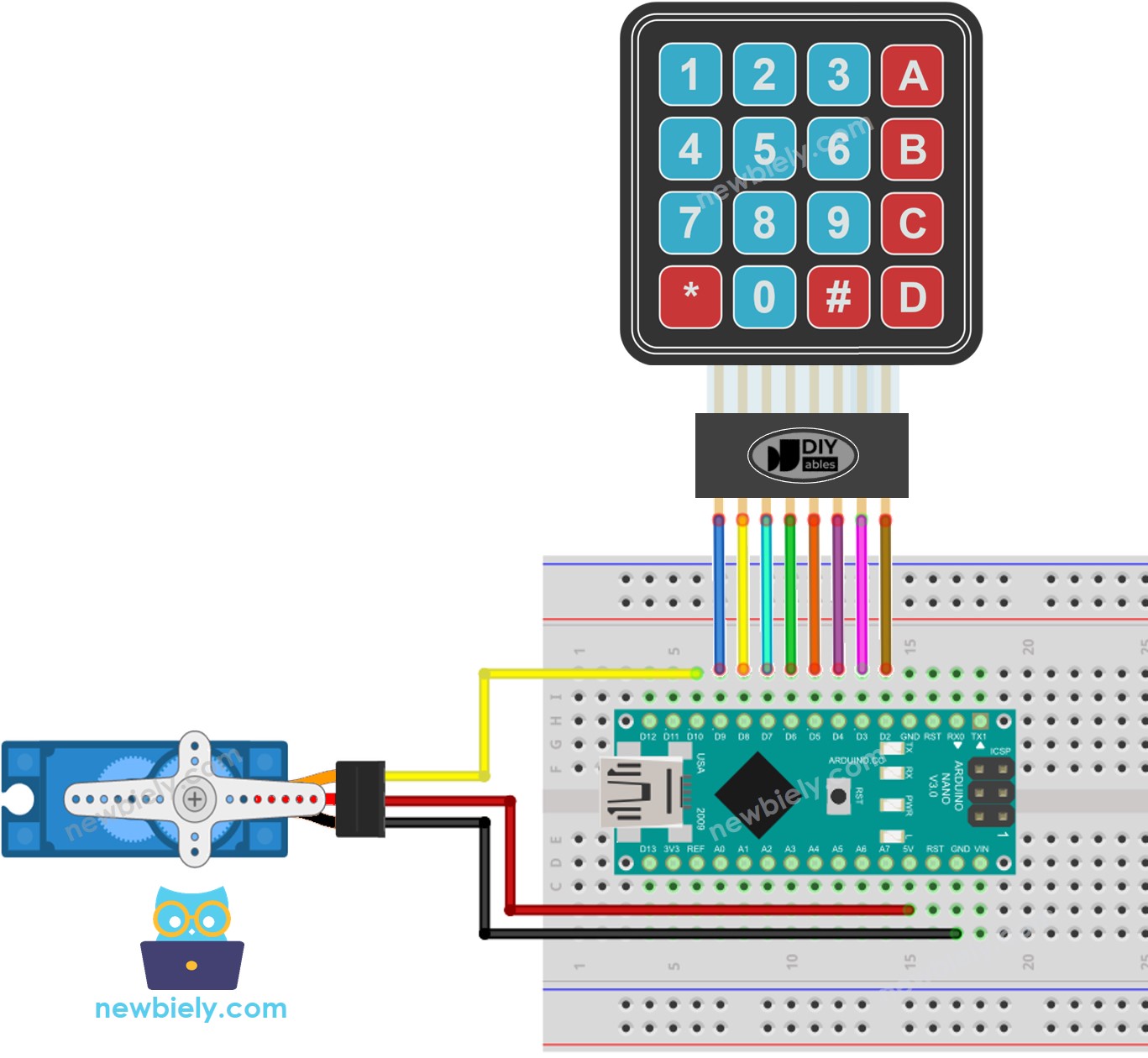
This image is created using Fritzing. Click to enlarge image
It should be noted that the wiring diagram shown above is only suitable for a servo motor with low torque. In case the motor vibrates instead of rotating, an external power source must be utilized to operate the servo motor. The following wiring diagram demonstrates how to connect the servo motor to an external power source.
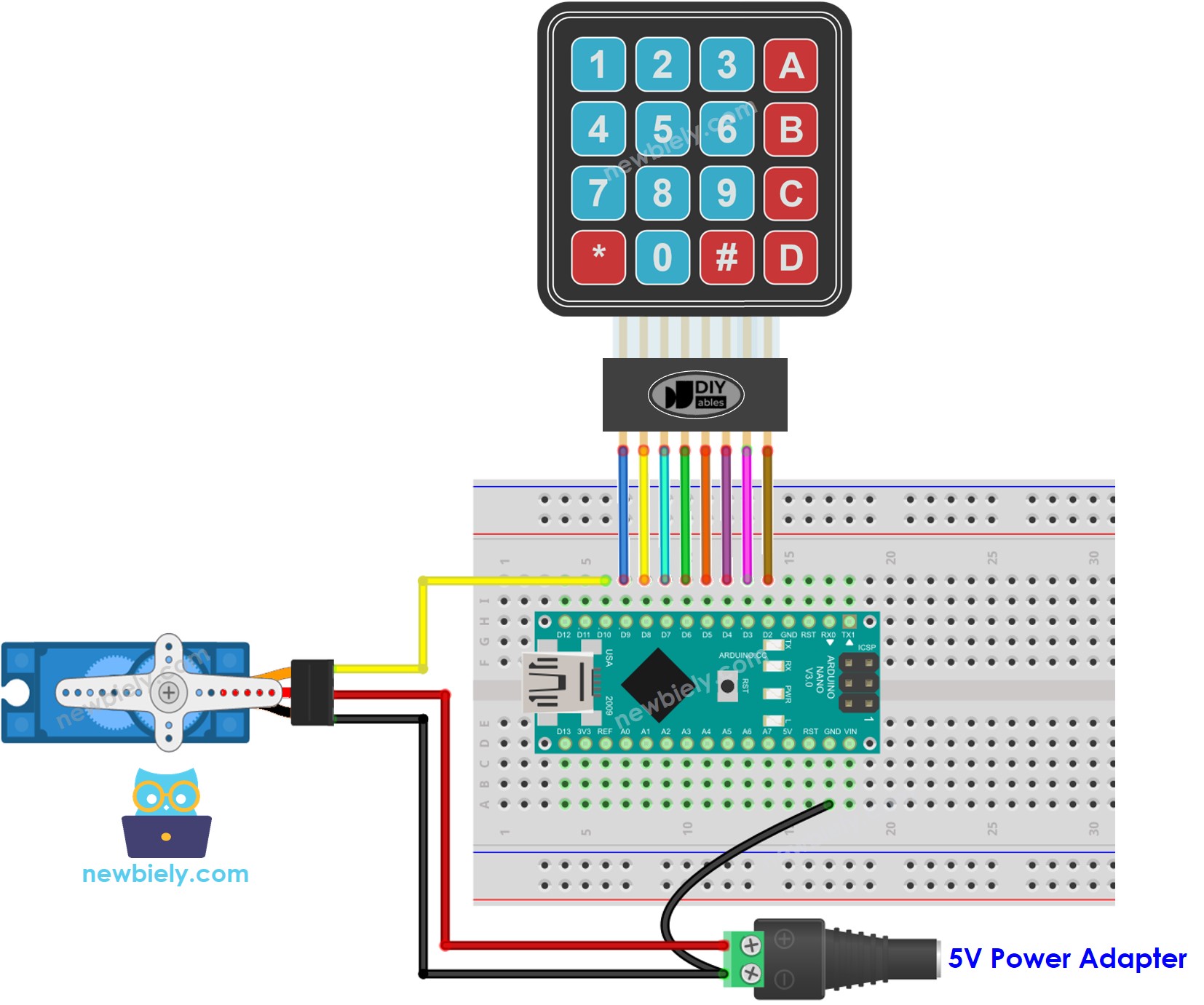
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Please do not forget to connect GND of the external power to GND of Arduino.
Arduino Nano Code - rotates Servo Motor if the password is correct
If the password is correct, the servo motor will be set to 90° for 5 seconds. After this period of time, it will be turned to 0°.
Detailed Instructions
- Connect a USB cable between the Arduino Nano and the PC.
- Open the Arduino IDE, select the correct board and port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “keypad” and locate the keypad library created by Mark Stanley and Alexander Brevig.
- Then, press the Install button to complete the installation of the keypad library.
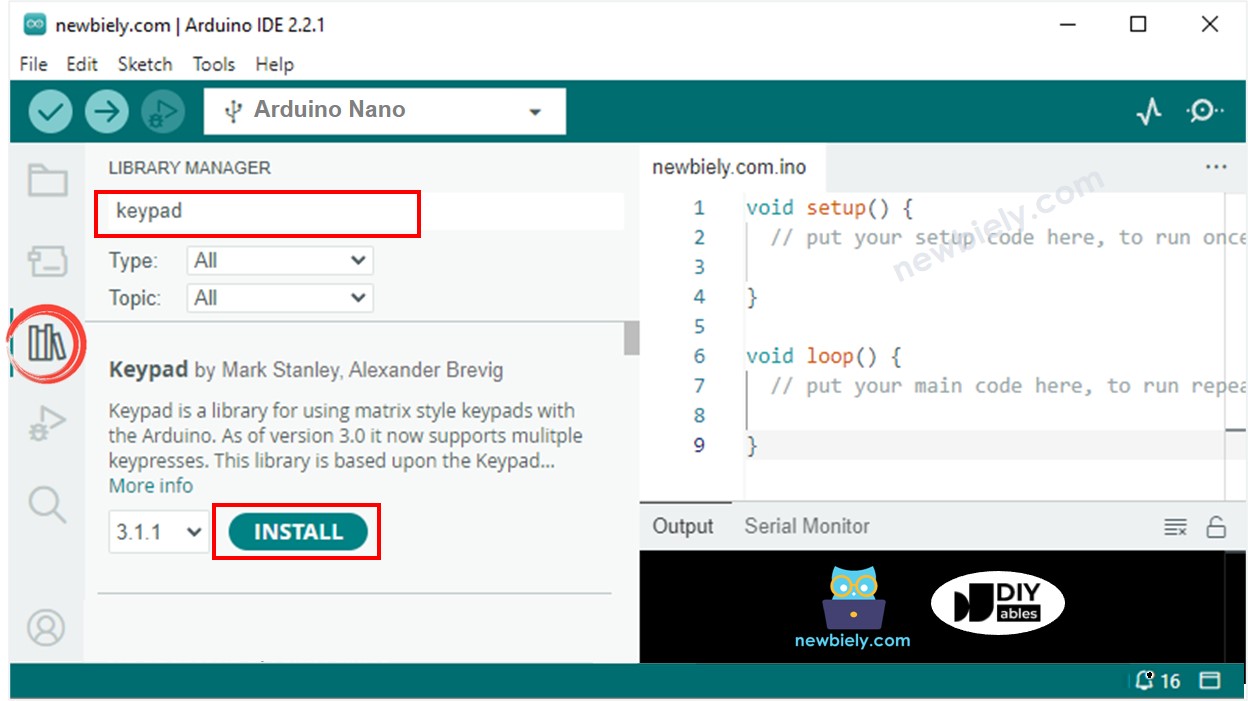
- Copy the code and open it with the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to the Arduino Nano.
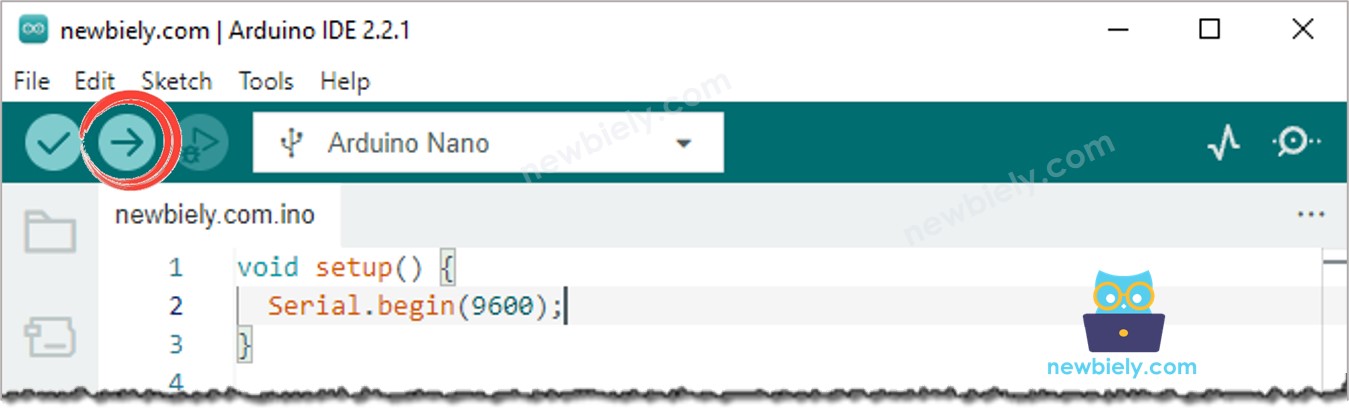
- Enter 12345# into the keypad, followed by 09876#.
- Check the Serial Monitor and observe the state of the servo motor.
Code Explanation
The valid passwords are pre-defined in the Arduino Nano code. A string, referred to as input_password, is used to store the password inputted by users. On the keypad, two keys (* and #) are used for special purposes: clearing the password and terminating the password. When a key is pressed:
- If the key is not one of the two special keys, it is added to the input_password.
- If the key is *, the input_password is cleared. This can be used to start or restart the password input.
- If the key is #:
- The Arduino Nano compares input_password with the pre-defined passwords. If it matches one of them, the servo motor is rotated to 90°.
- Regardless of whether the password is correct or not, the input_password is cleared for the next input.
- After a timeout, Arduino Nano rotates the servo motor to 0°.