Arduino Nano - Touch Sensor - Solenoid Lock
This tutorial instructs you how to use the Arduino Nano and touch sensor to control the solenoid lock.
Application 1 - The solenoid lock state is synchronized with the touch sensor state. In detail:
- Arduino Nano turns on the solenoid lock when the touch sensor is being touched.
- Arduino Nano turns off the solenoid lock when the touch sensor is NOT being touched.
Application 2 - The solenoid lock state is toggled each time the touch sensor is touched. More specifically:
- If Arduino Nano detects that the touch sensor has been touched (changing from a HIGH state to a LOW state), it will turn ON the solenoid lock if it's currently OFF, or turn OFF the solenoid lock if it's currently ON.
- Releasing the touch sensor does not affect to the solenoid lock state.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Solenoid Lock and Touch Sensor
If you are unfamiliar with solenoid lock and touch sensor (including pinout, operation, and programming), the following tutorials can help:
Wiring Diagram
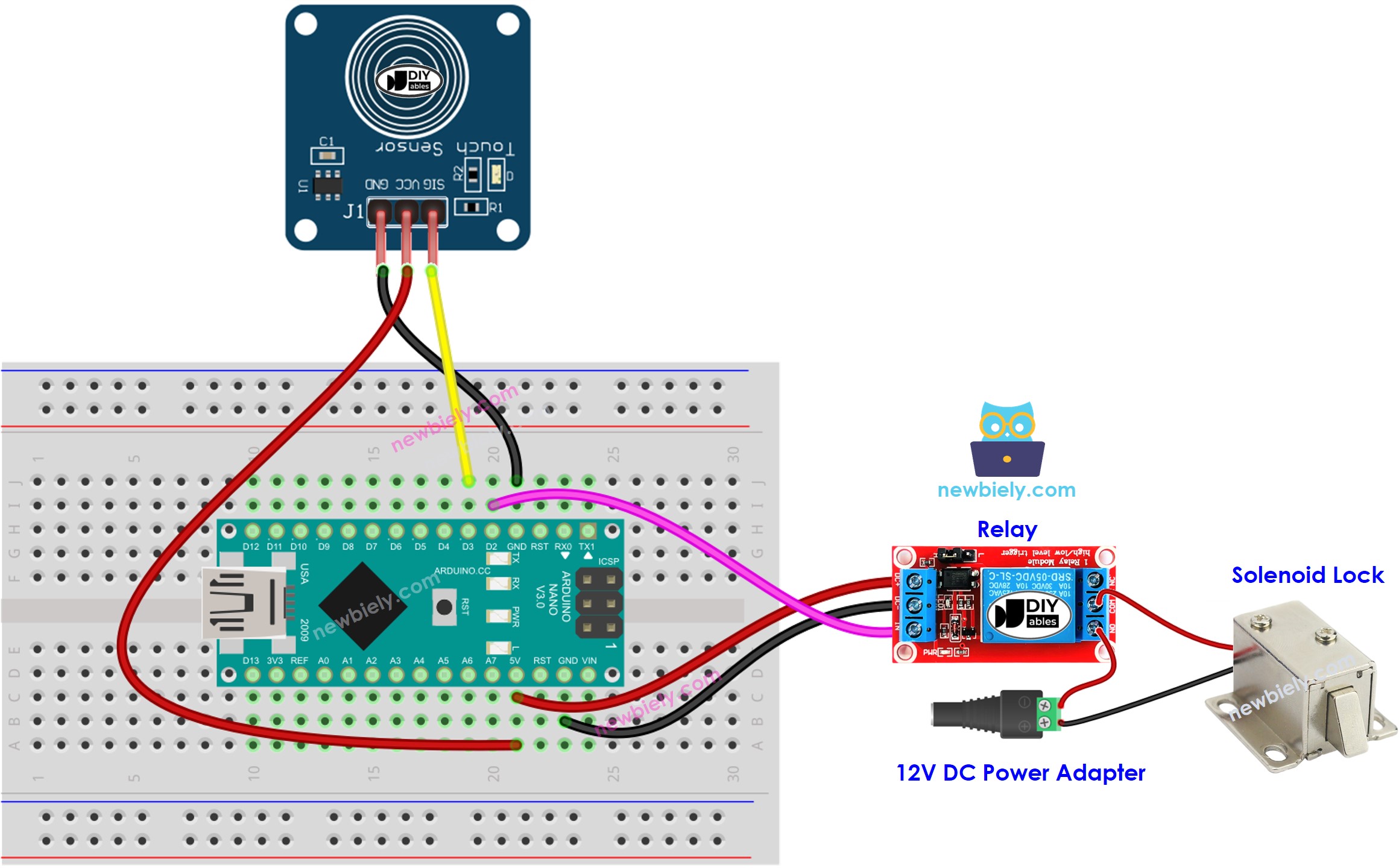
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Application 1 - The solenoid lock state is in sync with the touch sensor state
Arduino Nano Code
Detailed Instructions
- Connect an Arduino Nano to your computer with a USB cable.
- Launch the Arduino IDE, and select the correct board and port.
- Copy the code and open it in the Arduino IDE.
- Click the Upload button on the Arduino IDE to compile and upload the code to the Arduino Nano.
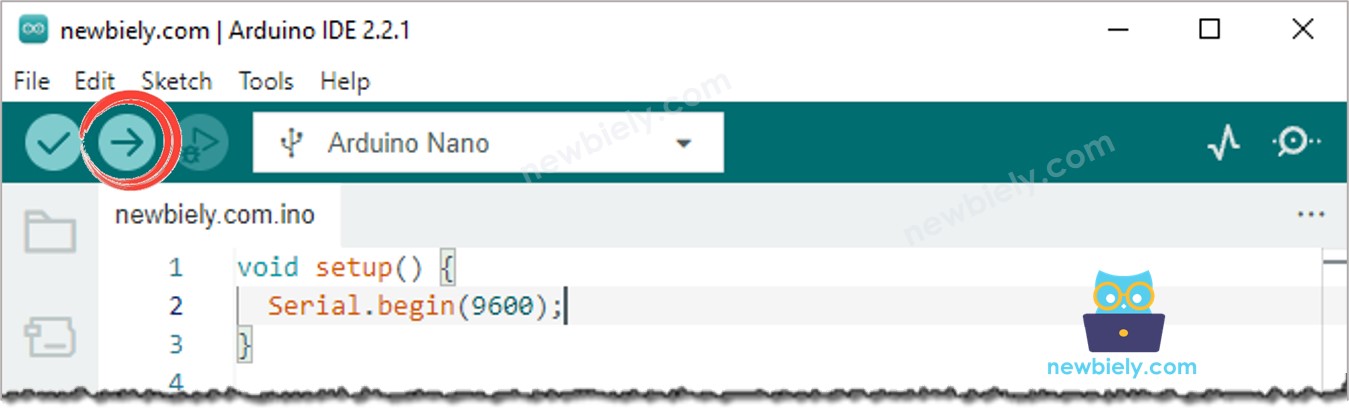
- Touch the touch sensor and hold it for a few seconds.
- Check out the change in the solenoid lock's condition.
You will see that the solenoid lock state is in sync with the touch sensor state.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
Application 2 - Touch Sensor toggles Solenoid Lock
Arduino Nano Code - Touch Sensor toggles Solenoid Lock
Code Explanation
You can locate the explanation in the comment lines of the Arduino Nano code above.
In the code, the expression solenoidLockState = !solenoidLockState is equivalent to the following code:
Detailed Instructions
- Copy the code and open it in the Arduino IDE.
- Upload the code to the Arduino Nano.
- Touch and release the touch sensor several times.
- Check out the change in the solenoid lock's state.
You'll notice that the solenoid lock will switch on or off one time whenever you touch the touch sensor.