Arduino Nano - Water Sensor
This tutorial instructs you how to use the water sensor with Arduino Nano. We will learn:
- How to connect the water sensor to Arduino Nano
- How to program Arduino Nano to read the state from the water sensor.
- How to calibrate the water sensor.
This can be applied to applications that detect water leakage, rainfall, tank overflow...
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Water Level Sensor
Water Level Sensor Pinout
The water level sensor has 3 pins:
- The S (Signal) pin is an analog output that will be connected to one of the analog inputs on your Arduino Nano.
- The + (VCC) pin supplies power for the sensor and it is suggested to use a voltage between 3.3V – 5V.
- The - (GND) pin is a ground connection.
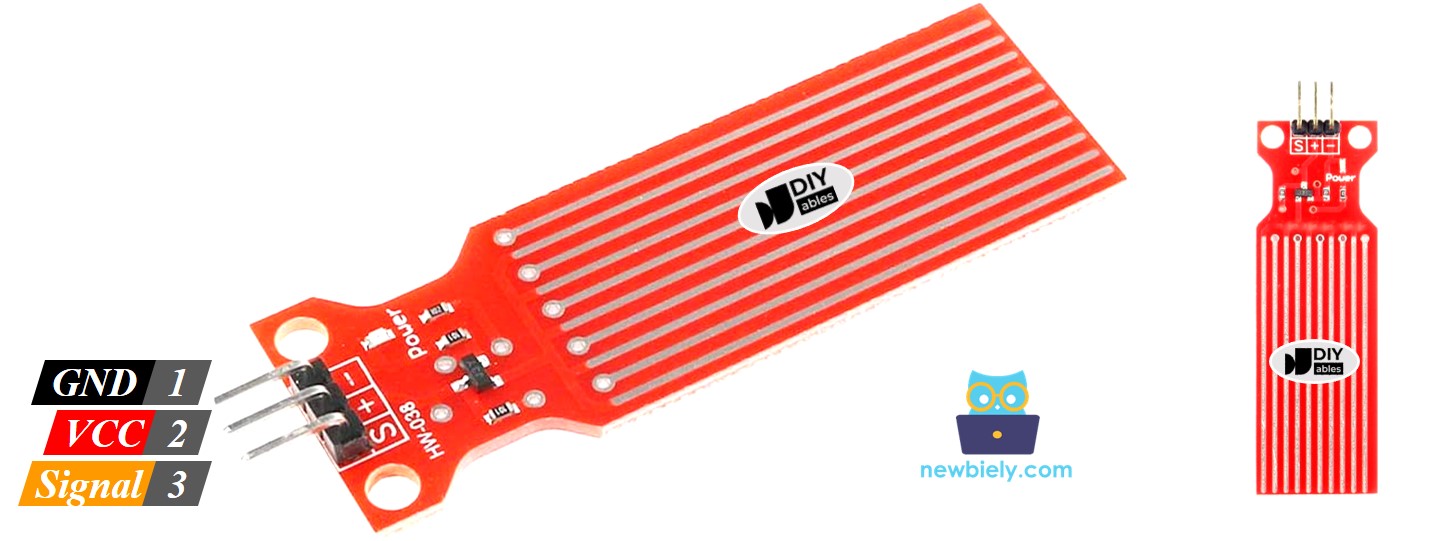
How Water Level Sensor Works
In short, The greater the amount of water the sensor is submerged in, the higher the voltage of the signal pin will be.
Let's take a closer look.
The sensor has a set of ten exposed copper tracks:
- Five of them are power tracks
- The other five are sense tracks.
The tracks are arranged in parallel with one sense track between every two power tracks. Unless they are bridged by water when submerged, the tracks are not connected.
The traces act as a variable resistor, similar to a potentiometer, whose resistance varies with the water level:
- The resistance is determined by the distance from the top of the sensor to the surface of the water.
- The resistance is inversely proportional to the amount of water present:
- When more water is present, the conductivity is improved and the resistance is lowered.
- When less water is present, the conductivity is reduced and the resistance is increased.
- The sensor produces an output voltage based on the resistance.
Determining the water level can be done by measuring the voltage.
Wiring Diagram
In theory, the VCC and GND pins of the sensor can be connected to the 5v and GND pins of the Arduino Nano in order to provide power to the sensor.
However, it is not advised to take this approach in practice. In a humid environment, if the sensor is supplied with power continuously, it will be electrochemically corroded faster, thus reducing its lifespan.
To avoid this, we suggest not to keep the sensor powered all the time, but only when reading its value. This can be done by connecting the sensor's VCC pin to a digital pin of an Arduino Nano, and setting the Arduino's pin to HIGH before reading and LOW afterwards.
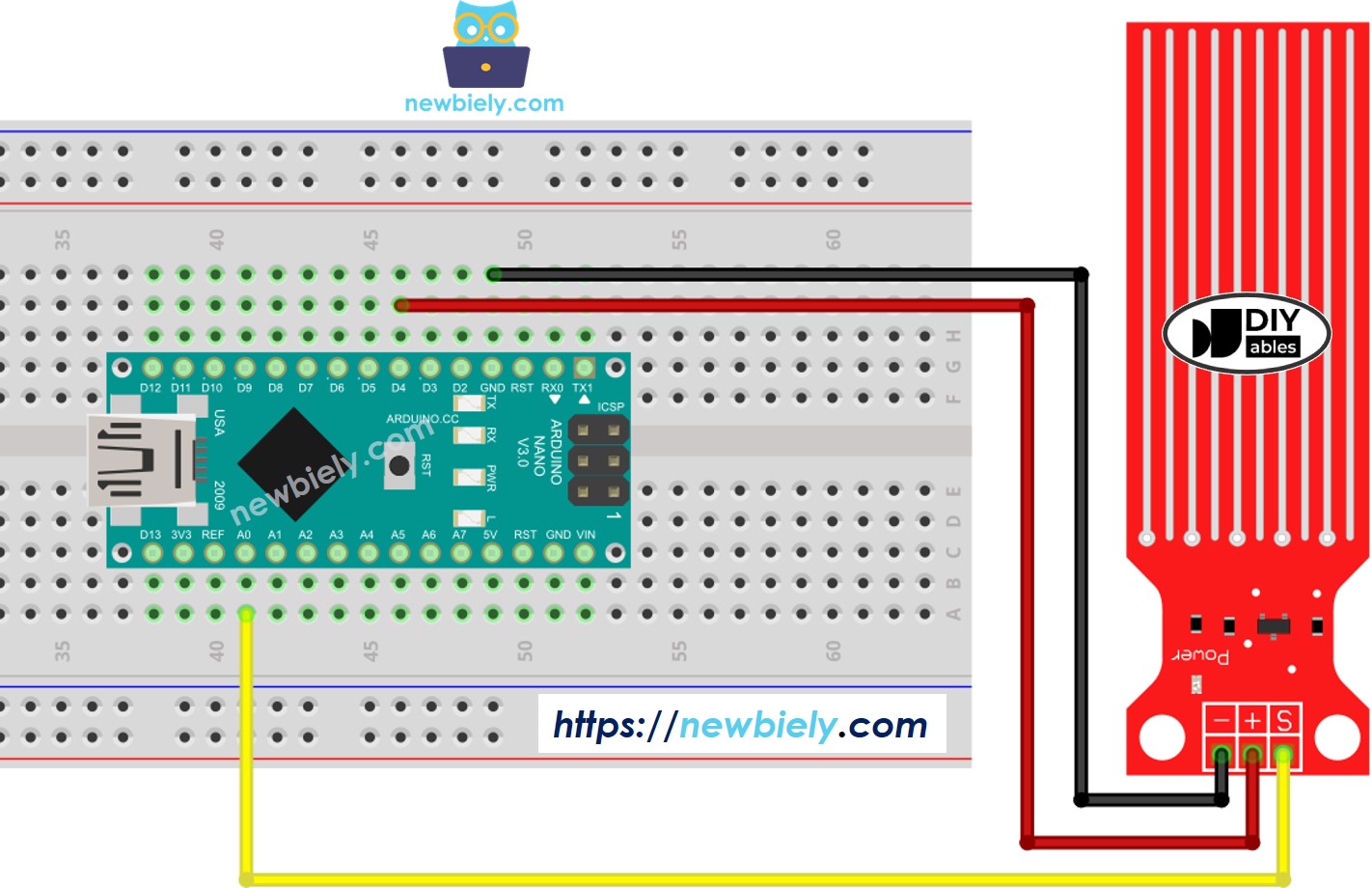
This image is created using Fritzing. Click to enlarge image
Arduino Nano Code - Reading Value from Water Sensor
Detailed Instructions
- Copy the code above and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to the Arduino Nano.
- Gently lower the sensor into a glass of water.
- Check the Serial Monitor to see the result; it should be 0 when the sensor is not touching anything.
※ NOTE THAT:
The sensor should not be completely immersed in water; only the exposed traces on the PCB should come into contact with it. Please take care when installing it.
How To Detect Water Leakage
To identify water leakage, rainfall, and tank overflow, we just have to compare the reading value with a threshold value that is determined in the calibration section of this tutorial.
Let's look at a particular instance. If water is detected, Arduino Nano will activate an LED.
Wiring Diagram
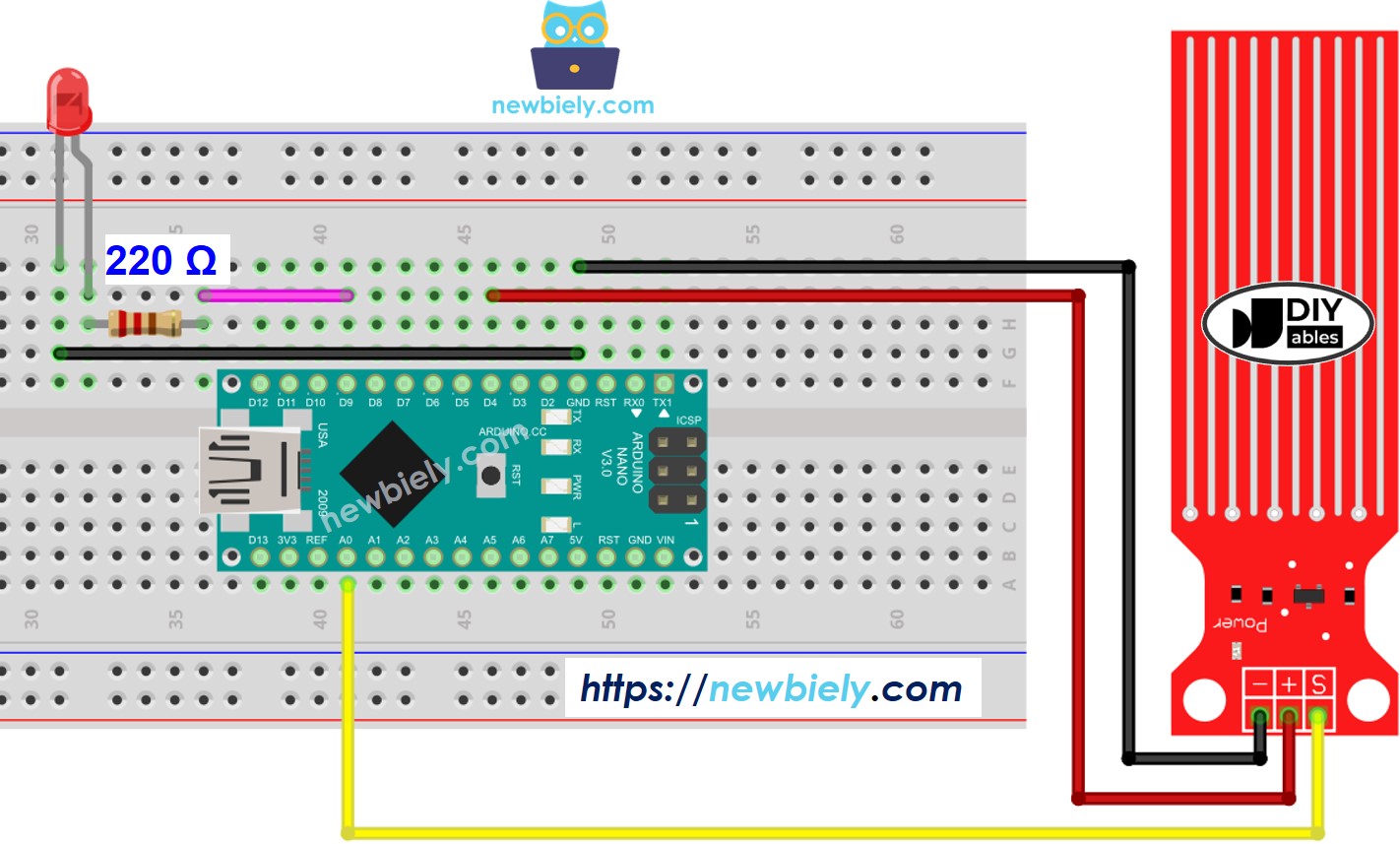
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Arduino Nano Code - Detecting Water Leakage
How To Measure The Water Level
If you wish to partition the maximum water height into various levels and measure the present level, you can utilize the technique in the code below. Note that the maximum water height is equivalent to the height of the sensor. The code below divides the maximum height into 4 levels.
※ NOTE THAT:
- SENSOR_MIN and SENSOR_MAX are determined through calibration.
- The mapping method mentioned is not precise, yet it is suitable for many applications.
- If you wish to make it more accurate, you can measure the threshold values for each level. Refer to the calibration section of the manual for further information.
Water Level Sensor Calibration
The output of the sensor is influenced by both the water level and the conductivity of the water. Pure water has no conductivity, whereas water with minerals and impurities is conductive. The higher the conductivity of the water, the more sensitive the sensor will be. Additionally, the output value is also affected by the voltage supplied to the VCC pin of the sensor.
To ensure accuracy when reading the water sensor, we suggest calibrating the sensor for the specific type of water to be monitored.
Prior to establishing the limit for activating a response, it is necessary to assess the genuine value obtained from the sensor by conducting an experiment.
Instructions for the test:
- Refer to the sketch provided above when reading sensor values.
- Place the sensor in the water at the desired threshold level.
- Record the value that the sensor displays in the Serial Monitor.
- Utilize this value as the threshold to activate an action.
It may require experimentation to complete this test. Be prepared to try different approaches. Be ready to experiment with various strategies. Have patience and don't give up. Have perseverance and don't surrender.
The test can be utilized to uncover:
- SENSOR_MIN value, when the sensor is not submerged in the liquid
- SENSOR_MAX value, when the sensor is totally submerged in the water
- A threshold value for recognizing water spillage
- The threshold values for each level of your degree scale.
Video Tutorial
Challenge Yourself
- When water leakage is detected:
- Send an email
- Send a SMS message
- Make a sound alarm