Arduino Nano - DS1307 RTC Module
This tutorial instructs you how to use Arduino Nano to read date and time from DS1307 RTC module. In detail, we will learn:
How to connect DS1307 Real-Time Clock module to Arduino Nano.
How to program Arduino Nano to read date and time (second, minute, hour, day of week, day off month, month, and year) from the DS1307 RTC module.
How to program Arduino Nano to create daily schedules using DS1307 RTC module.
How to program Arduino Nano to create weekly schedules using DS1307 RTC module.
How to program Arduino Nano to create schedules on a specific date using DS1307 RTC module.
Or you can buy the following sensor kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
Arduino Nano includes some time-related functions, such as millis() and micros(). However, these do not provide date and time information (seconds, minutes, hours, day, date, month, and year). To obtain this data, we must use a Real-Time Clock (RTC) module, such as DS3231 or DS1370. The DS3231 Module is more precise than the DS1370. See DS3231 vs DS1307 for more details.
The Real-Time Clock DS1307 Module has 12 pins, but only 4 of them are necessary for its normal use: SCL, SDA, VCC, and GND.
SCL pin: This is a serial clock pin for the I2C interface.
SDA pin: This is a serial data pin for the I2C interface.
VCC pin: This supplies power to the module and can range from 3.3V to 5.5V.
GND pin: This is the ground pin.
The DS1307 Module has a battery holder for a CR2032 battery.
If the battery is inserted, the time on the module will remain even when the main power is disconnected.
If the battery is not inserted, the time information will be lost if the main power is disconnected and it must be reset.
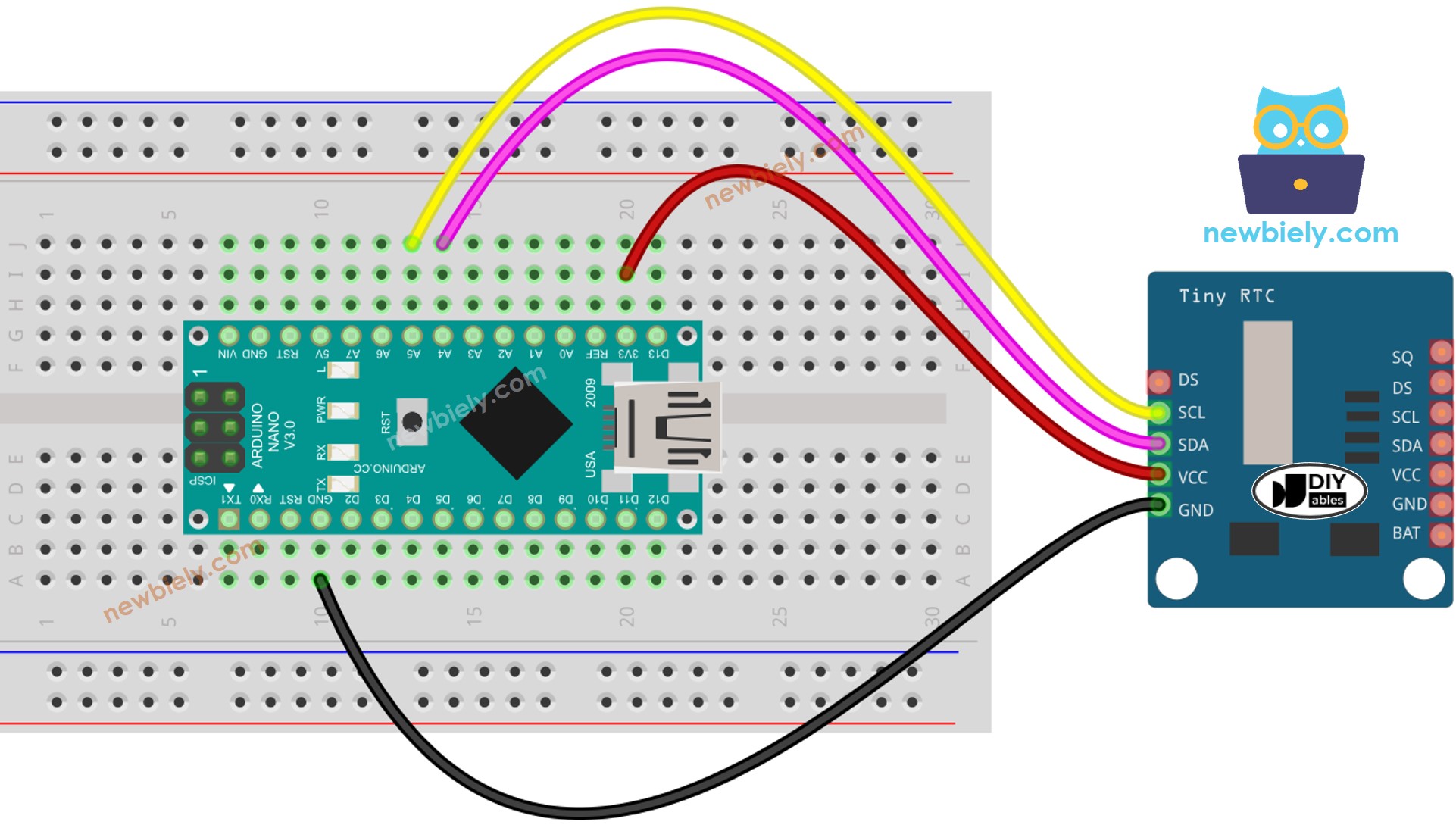
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
DS1307 RTC Module | Arduino Nano |
Vin | 3.3V |
GND | GND |
SDA | A4 |
SCL | A5 |
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
while (1);
}
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
DateTime now = rtc.now();
Serial.print("Date & Time: ");
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(" (");
Serial.print(now.dayOfTheWeek());
Serial.print(") ");
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.println(now.second(), DEC);
#include <RTClib.h>
RTC_DS1307 rtc;
char daysOfTheWeek[7][12] = {
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday"
};
void setup () {
Serial.begin(9600);
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
Serial.flush();
while (1);
}
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
}
void loop () {
DateTime now = rtc.now();
Serial.print("Date & Time: ");
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(" (");
Serial.print(daysOfTheWeek[now.dayOfTheWeek()]);
Serial.print(") ");
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.println(now.second(), DEC);
delay(1000);
}
Click to the Libraries icon on the left bar of the Arduino IDE.
Search for “RTClib” and locate the RTC library by Adafruit.
Press the Install button to install the RTC library.
Copy the code and open it with Arduino IDE.
Click the Upload button to send the code to Arduino Nano.
Open the Serial Monitor.
Check out the result on the Serial Monitor.
Date & Time: 2021/10/6 (Wednesday) 9:9:35
Date & Time: 2021/10/6 (Wednesday) 9:9:36
Date & Time: 2021/10/6 (Wednesday) 9:9:37
Date & Time: 2021/10/6 (Wednesday) 9:9:38
Date & Time: 2021/10/6 (Wednesday) 9:9:39
Date & Time: 2021/10/6 (Wednesday) 9:9:40
Date & Time: 2021/10/6 (Wednesday) 9:9:41
Date & Time: 2021/10/6 (Wednesday) 9:9:42
Date & Time: 2021/10/6 (Wednesday) 9:9:43
Date & Time: 2021/10/6 (Wednesday) 9:9:44
#include <RTClib.h>
uint8_t DAILY_EVENT_START_HH = 13;
uint8_t DAILY_EVENT_START_MM = 50;
uint8_t DAILY_EVENT_END_HH = 14;
uint8_t DAILY_EVENT_END_MM = 10;
RTC_DS1307 rtc;
char daysOfTheWeek[7][12] = {
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday"
};
void setup () {
Serial.begin(9600);
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
while (1);
}
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
}
void loop () {
DateTime now = rtc.now();
if (now.hour() >= DAILY_EVENT_START_HH &&
now.minute() >= DAILY_EVENT_START_MM &&
now.hour() < DAILY_EVENT_END_HH &&
now.minute() < DAILY_EVENT_END_MM) {
Serial.println("It is on scheduled time");
} else {
Serial.println("It is NOT on scheduled time");
}
printTime(now);
}
void printTime(DateTime time) {
Serial.print("TIME: ");
Serial.print(time.year(), DEC);
Serial.print('/');
Serial.print(time.month(), DEC);
Serial.print('/');
Serial.print(time.day(), DEC);
Serial.print(" (");
Serial.print(daysOfTheWeek[time.dayOfTheWeek()]);
Serial.print(") ");
Serial.print(time.hour(), DEC);
Serial.print(':');
Serial.print(time.minute(), DEC);
Serial.print(':');
Serial.println(time.second(), DEC);
}
#include <RTClib.h>
#define SUNDAY 0
#define MONDAY 1
#define TUESDAY 2
#define WEDNESDAY 3
#define THURSDAY 4
#define FRIDAY 5
#define SATURDAY 6
uint8_t WEEKLY_EVENT_DAY = MONDAY;
uint8_t WEEKLY_EVENT_START_HH = 13;
uint8_t WEEKLY_EVENT_START_MM = 50;
uint8_t WEEKLY_EVENT_END_HH = 14;
uint8_t WEEKLY_EVENT_END_MM = 10;
RTC_DS1307 rtc;
char daysOfTheWeek[7][12] = {
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday"
};
void setup () {
Serial.begin(9600);
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
while (1);
}
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
}
void loop () {
DateTime now = rtc.now();
if (now.dayOfTheWeek() == WEEKLY_EVENT_DAY &&
now.hour() >= WEEKLY_EVENT_START_HH &&
now.minute() >= WEEKLY_EVENT_START_MM &&
now.hour() < WEEKLY_EVENT_END_HH &&
now.minute() < WEEKLY_EVENT_END_MM) {
Serial.println("It is on scheduled time");
} else {
Serial.println("It is NOT on scheduled time");
}
printTime(now);
}
void printTime(DateTime time) {
Serial.print("TIME: ");
Serial.print(time.year(), DEC);
Serial.print('/');
Serial.print(time.month(), DEC);
Serial.print('/');
Serial.print(time.day(), DEC);
Serial.print(" (");
Serial.print(daysOfTheWeek[time.dayOfTheWeek()]);
Serial.print(") ");
Serial.print(time.hour(), DEC);
Serial.print(':');
Serial.print(time.minute(), DEC);
Serial.print(':');
Serial.println(time.second(), DEC);
}
#include <RTClib.h>
#define SUNDAY 0
#define MONDAY 1
#define TUESDAY 2
#define WEDNESDAY 3
#define THURSDAY 4
#define FRIDAY 5
#define SATURDAY 6
#define JANUARY 1
#define FEBRUARY 2
#define MARCH 3
#define APRIL 4
#define MAY 5
#define JUNE 6
#define JULY 7
#define AUGUST 8
#define SEPTEMBER 9
#define OCTOBER 10
#define NOVEMBER 11
#define DECEMBER 12
DateTime EVENT_START(2021, AUGUST, 15, 13, 50);
DateTime EVENT_END(2021, SEPTEMBER, 29, 14, 10);
RTC_DS1307 rtc;
char daysOfTheWeek[7][12] = {
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday"
};
void setup () {
Serial.begin(9600);
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
Serial.flush();
while (1);
}
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
}
void loop () {
DateTime now = rtc.now();
if (now.secondstime() >= EVENT_START.secondstime() &&
now.secondstime() < EVENT_END.secondstime()) {
Serial.println("It is on scheduled time");
} else {
Serial.println("It is NOT on scheduled time");
}
printTime(now);
}
void printTime(DateTime time) {
Serial.print("TIME: ");
Serial.print(time.year(), DEC);
Serial.print('/');
Serial.print(time.month(), DEC);
Serial.print('/');
Serial.print(time.day(), DEC);
Serial.print(" (");
Serial.print(daysOfTheWeek[time.dayOfTheWeek()]);
Serial.print(") ");
Serial.print(time.hour(), DEC);
Serial.print(':');
Serial.print(time.minute(), DEC);
Serial.print(':');
Serial.println(time.second(), DEC);
}