Arduino Nano - Ultrasonic Sensor
This tutorial instructs you how to use Arduino Nano and ultrasonic sensor to measure the distance to obstacles or objects. In detail, we will learn:
- How ultrasonic sensor works
- How to connect the ultrasonic sensor to Arduino Nano
- How to program Arduino Nano to measure distance using the ultrasonic sensor
- How to filter out noise from the distance measurements of the ultrasonic sensor in Arduino Nano code
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Ultrasonic Sensor
The HC-SR04 ultrasonic sensor is used to measure the distance to an object by using ultrasonic waves.
The Ultrasonic Sensor Pinout
The HC-SR04 ultrasonic sensor has four pins:
- VCC pin: must be connected to VCC (5V)
- GND pin: must be connected to GND (0V)
- TRIG pin: receives a control signal (pulse) from Arduino Nano
- ECHO pin: sends a signal (pulse) to Arduino Nano. Arduino Nano measures the length of the pulse to calculate the distance.
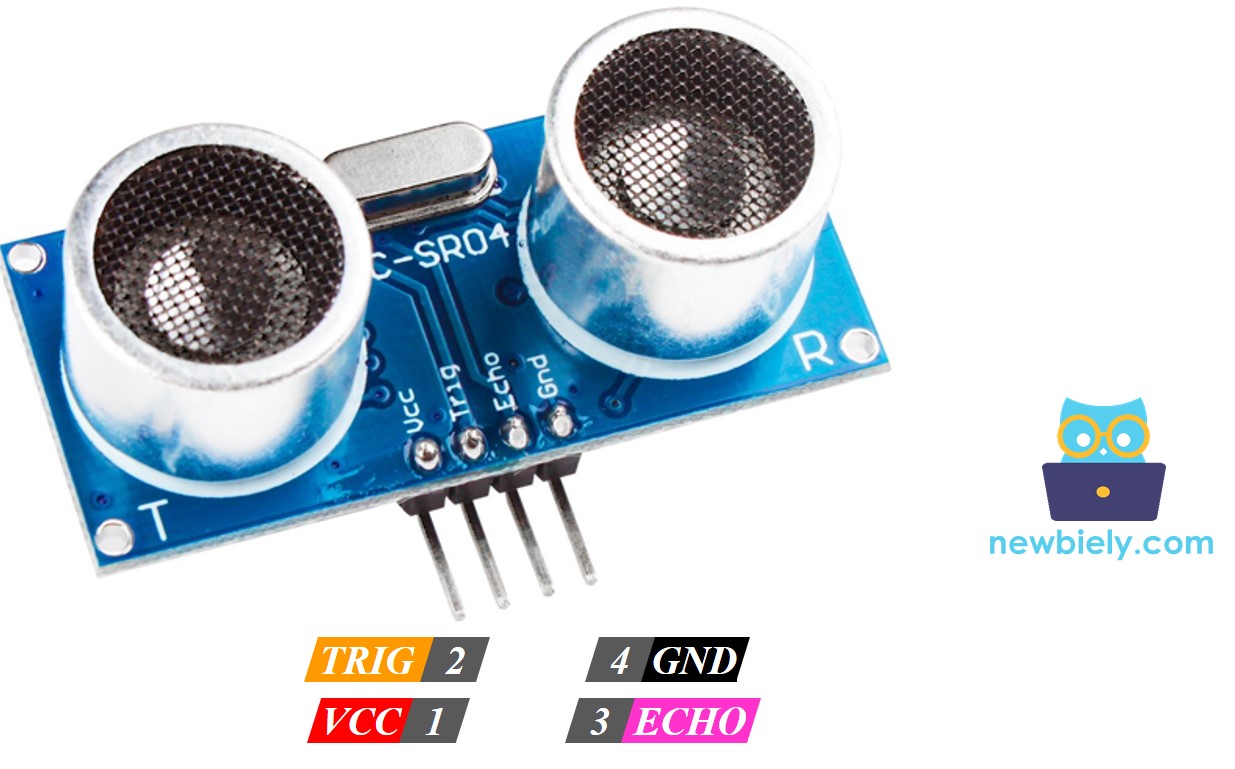
How It Works
- The Arduino Nano produces a 10-microsecond pulse on the TRIG pin, which causes the ultrasonic sensor to emit ultrasonic waves.
- When the waves hit an obstacle, they are reflected back.
- The ultrasonic sensor is able to detect the reflected wave and measure its travel time.
- The sensor then generates a pulse to the ECHO pin, with a duration equal to the travel time of the ultrasonic wave.
- The Arduino Nano measures the pulse duration in the ECHO pin and calculates the distance between the sensor and the obstacle.
How to Get Distance From Ultrasonic Sensor
To calculate the distance from the ultrasonic sensor, We just need to write Arduino code to do two steps (1 and 6 on How It Works):
- Arduino Nano generates a 10-microsecond pulse on the TRIG pin.
- Arduino Nano measures the pulse duration in the ECHO pin.
- Then, Arduino Nano uses the measured pulse duration to calculate the distance between the sensor and obstacle.
Distance Calculation
We have:
- The travel time of the ultrasonic wave (µs): travel_time = pulse_duration
- The speed of the ultrasonic wave: speed = SPEED_OF_SOUND = 340 m/s = 0.034 cm/µs
So:
- The travel distance of the ultrasonic wave (cm): travel_distance = speed × travel_time = 0.034 × pulse_duration
- The distance between sensor and obstacle (cm): distance = travel_distance / 2 = 0.034 × pulse_duration / 2 = 0.017 × pulse_duration
Arduino Nano - Ultrasonic Sensor
The Arduino Nano's pins can produce a 10-microsecond pulse and measure the pulse duration. This allows us to use two of the Arduino Nano's pins to determine the distance from the ultrasonic sensor to an object. We just need to:
- Connect one pin of Arduino Nano to the TRIG pin of ultrasonic sensor. This Arduino Nano pin is used to generate a 10µs pulse to the TRIG pin of the sensor
- Connect another pin of Arduino Nano to the ECHO pin of ultrasonic sensor. This Arduino Nano pin is used to measure the pulse from the sensor
Wiring Diagram
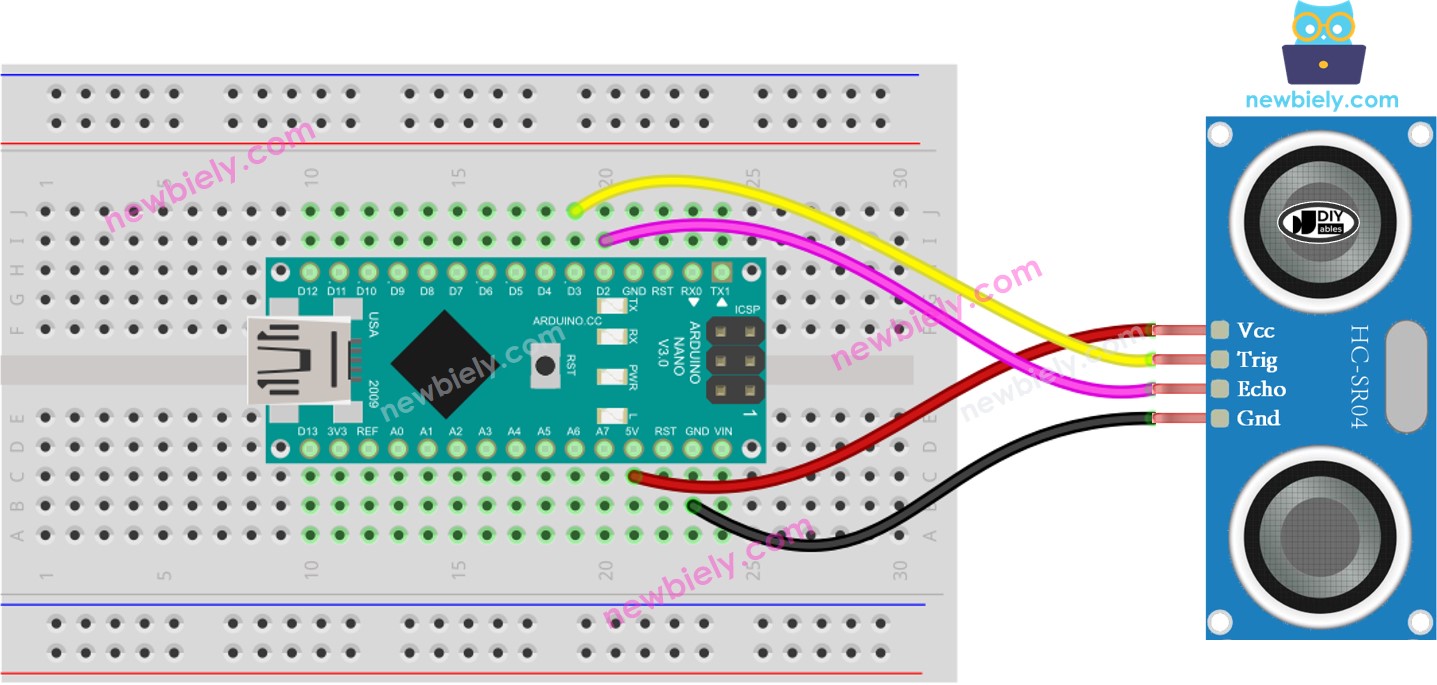
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
How To Program For Ultrasonic Sensor
- Generate a 10-microsecond pulse on Arduino's pin 3 by utilizing digitalWrite() and delayMicroseconds() functions. For instance:
- Measure the pulse duration (in microseconds) on Arduino's pin 2 by utilizing the pulseIn() function. For instance:
- Calculate the distance (cm):
Arduino Nano Code
Detailed Instructions
- Copy the code and open it with the Arduino IDE.
- Then, click the Upload button in the Arduino IDE to compile and upload the code to the Arduino Nano.
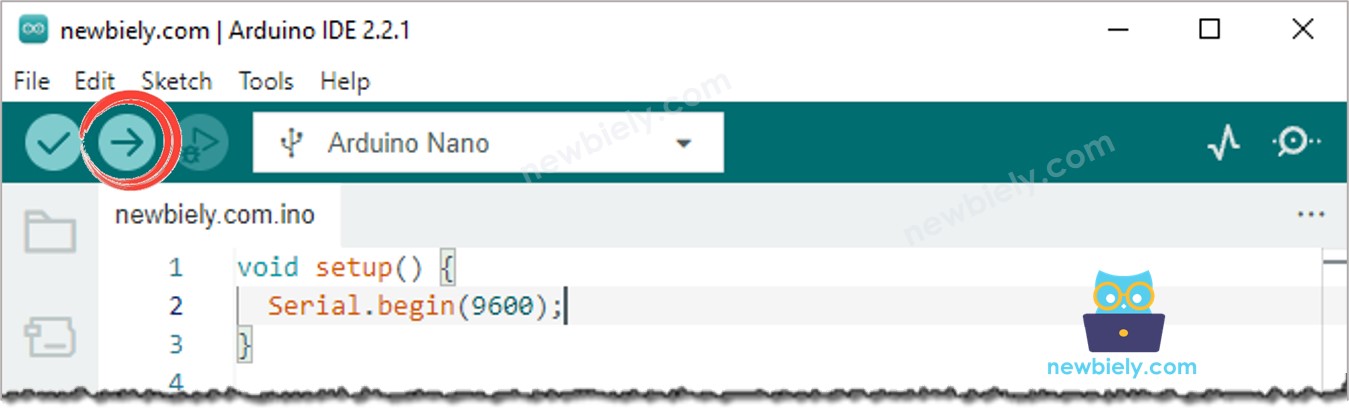
- Open the Serial Monitor
- Place your hand in front of the ultrasonic sensor
- Check out the distance from the sensor to your hand being displayed on the Serial Monitor
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
How to Filter Noise from Distance Measurements of Ultrasonic Sensor
The measurement result from an ultrasonic sensor may contain noise, which can lead to unwanted operations in some applications. To remove the noise, we can use the following algorithm:
- Take multiple measurements and store them in an array
- Sort the array in ascending order
- Filter out noise by:
- The smallest samples are considered as noise and should be ignored
- The biggest samples are considered as noise and should be ignored
- The average of the middle samples should be taken
- Ignore the five smallest samples.
- Ignore the five biggest samples.
- Get the average of the 10 middle samples from the fifth to the fourteenth.
The below example code takes 20 measurements:
Video Tutorial
Challenge Yourself
Employ an ultrasonic sensor for any of the following projects:
- Building a collision avoidance system for a remote-controlled car.
- Establishing the fullness of a dustbin.
- Tracking the level of a dustbin.
- Automatically opening and closing a dustbin. Hint: See Arduino Nano - Servo Motor.
Ultrasonic Sensor Applications
- Avoiding Collisions
- Detecting Fullness
- Measuring Level
- Detecting Proximity