Arduino Nano - Log Data with Timestamp to SD Card
This tutorial instructs you how to write log with timestamp to the Micro SD Card using Arduino Nano. Specifically, we will cover:
Arduino Nano - Logging data with timestamp to a single file on Micro SD Card
Arduino Nano - Logging data with timestamp to multiple files on Micro SD Card, one file per day
The time is obtained from a RTC module and stored in a Micro SD Card together with the data.
The information that is stored on the Micro SD Card can be any type of data. This could include:
In a nutshell, this tutorial takes the values from two analog pins as an illustration of data. It is straightforward to modify the code to fit any type of data.
Or you can buy the following sensor kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you. We appreciate your support.
If you are unfamiliar with Micro SD Card Module and RTC module, including their pinouts, how they work, and how to program them, the following tutorials can help:
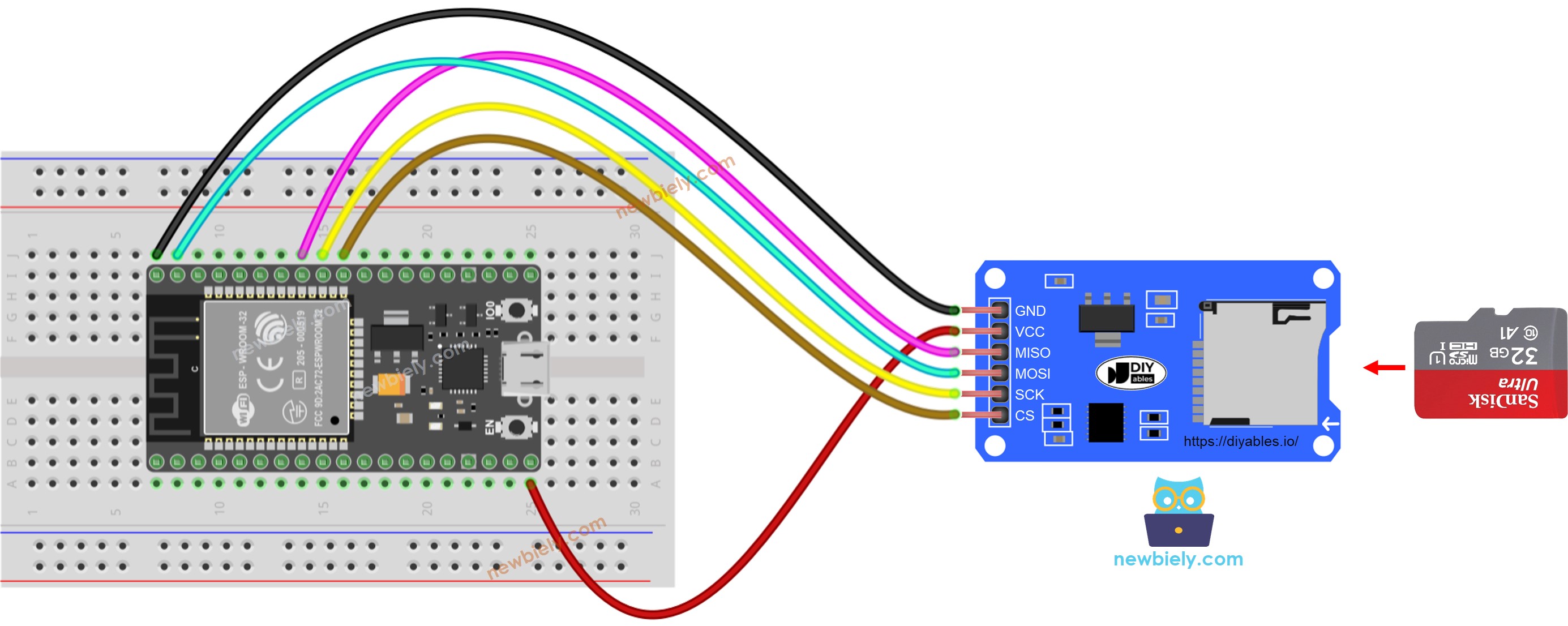
This image is created using Fritzing. Click to enlarge image
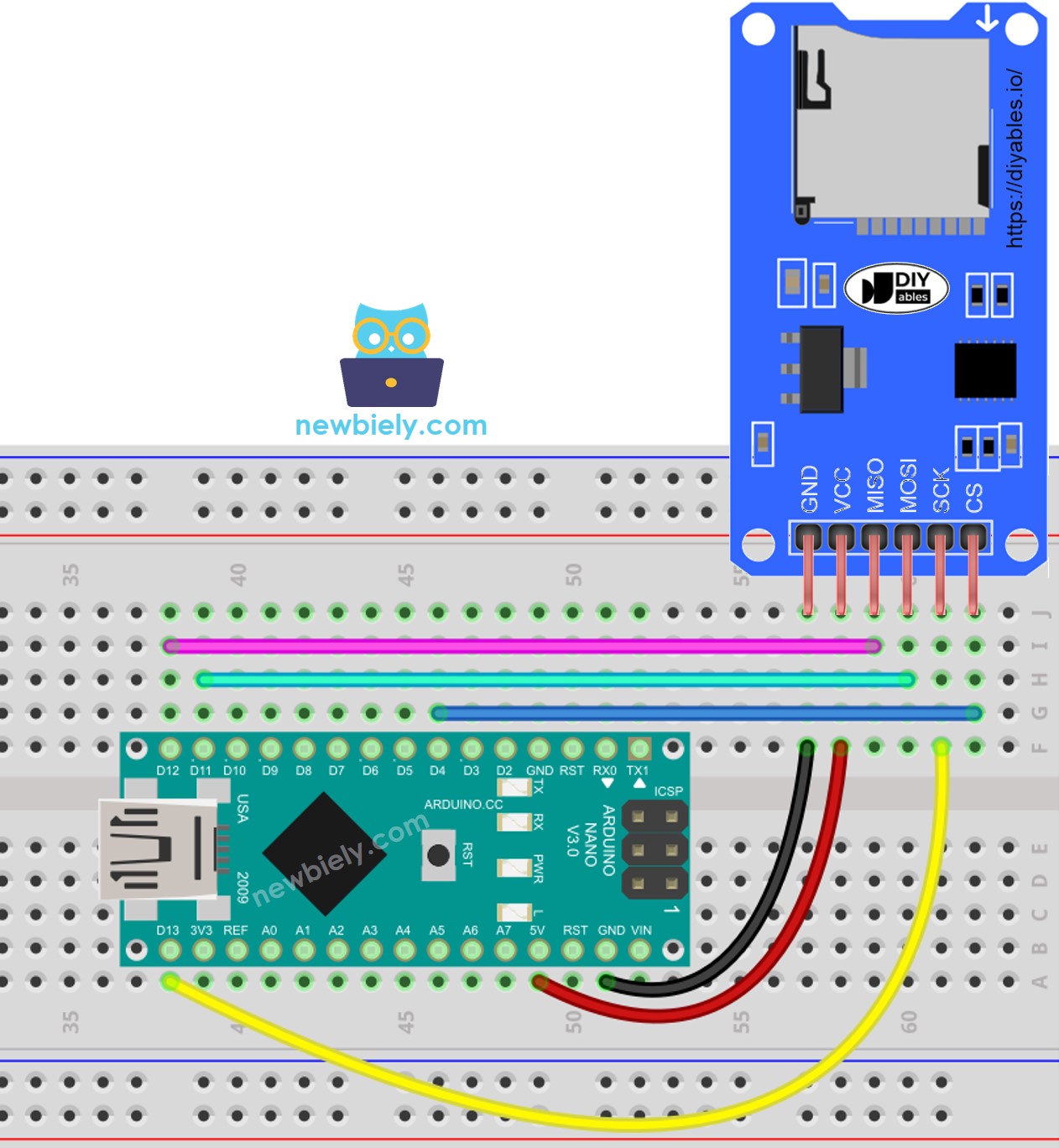
This image is created using Fritzing. Click to enlarge image
#include <SD.h>
#include <RTClib.h>
#define PIN_SPI_CS 4
#define FILE_NAME "log.txt"
RTC_DS3231 rtc;
File myFile;
void setup() {
Serial.begin(9600);
if (!rtc.begin()) {
Serial.println(F("Couldn't find RTC"));
while (1);
}
if (!SD.begin(PIN_SPI_CS)) {
Serial.println(F("SD CARD FAILED, OR NOT PRESENT!"));
while (1);
}
Serial.println(F("SD CARD INITIALIZED."));
Serial.println(F("--------------------"));
}
void loop() {
myFile = SD.open(FILE_NAME, FILE_WRITE);
if (myFile) {
Serial.println(F("Writing log to SD Card"));
DateTime now = rtc.now();
myFile.print(now.year(), DEC);
myFile.print('-');
myFile.print(now.month(), DEC);
myFile.print('-');
myFile.print(now.day(), DEC);
myFile.print(' ');
myFile.print(now.hour(), DEC);
myFile.print(':');
myFile.print(now.minute(), DEC);
myFile.print(':');
myFile.print(now.second(), DEC);
myFile.print(" ");
int analog_1 = analogRead(A0);
int analog_2 = analogRead(A1);
myFile.print("analog_1 = ");
myFile.print(analog_1);
myFile.print(", ");
myFile.print("analog_2 = ");
myFile.print(analog_2);
myFile.write("\n");
myFile.close();
} else {
Serial.print(F("SD Card: error on opening file "));
Serial.println(FILE_NAME);
}
delay(2000);
}
Ensure that the Micro SD Card is formatted as either FAT16 or FAT32 (you can find instructions on how to do this via a Google search).
Copy the code and open it in the Arduino IDE.
Click the Upload button in the Arduino IDE to send the code to the Arduino Nano.
Check the results in the Serial Monitor.
SD CARD INITIALIZED.
--------------------
Writing log to SD Card
Writing log to SD Card
Writing log to SD Card
Writing log to SD Card
Writing log to SD Card
Writing log to SD Card
Writing log to SD Card
Remove the Micro SD Card from the Micro SD Card module.
Insert the Micro SD Card into an USB SD Card reader.
Attach the USB SD Card reader to the PC.
Open the log.txt file on your computer; it will appear as follows.
If you do not possess an USB SD Card reader, you can examine the content of the log file by executing the Arduino Nano Code below.
#include <SD.h>
#define PIN_SPI_CS 4
#define FILE_NAME "log.txt"
File myFile;
void setup() {
Serial.begin(9600);
if (!SD.begin(PIN_SPI_CS)) {
Serial.println(F("SD CARD FAILED, OR NOT PRESENT!"));
while (1);
}
Serial.println(F("SD CARD INITIALIZED."));
myFile = SD.open(FILE_NAME, FILE_READ);
if (myFile) {
while (myFile.available()) {
char ch = myFile.read();
Serial.print(ch);
}
myFile.close();
} else {
Serial.print(F("SD Card: error on opening file "));
Serial.println(FILE_NAME);
}
}
void loop() {
}
Logging to a single file can lead to a large file size over time and make it hard to review. The code below will split the log into multiple files, with:
#include <SD.h>
#include <RTClib.h>
#define PIN_SPI_CS 4
RTC_DS3231 rtc;
File myFile;
char filename[] = "yyyymmdd.txt";
void setup() {
Serial.begin(9600);
if (!rtc.begin()) {
Serial.println(F("Couldn't find RTC"));
while (1);
}
if (!SD.begin(PIN_SPI_CS)) {
Serial.println(F("SD CARD FAILED, OR NOT PRESENT!"));
while (1);
}
Serial.println(F("SD CARD INITIALIZED."));
Serial.println(F("--------------------"));
}
void loop() {
DateTime now = rtc.now();
int year = now.year();
int month = now.month();
int day = now.day();
filename[0] = (year / 1000) + '0';
filename[1] = ((year % 1000) / 100) + '0';
filename[2] = ((year % 100) / 10) + '0';
filename[3] = (year % 10) + '0';
filename[4] = (month / 10) + '0';
filename[5] = (month % 10) + '0';
filename[6] = (day / 10) + '0';
filename[7] = (day % 10) + '0';
myFile = SD.open(filename, FILE_WRITE);
if (myFile) {
Serial.println(F("Writing log to SD Card"));
myFile.print(now.year(), DEC);
myFile.print('-');
myFile.print(now.month(), DEC);
myFile.print('-');
myFile.print(now.day(), DEC);
myFile.print(' ');
myFile.print(now.hour(), DEC);
myFile.print(':');
myFile.print(now.minute(), DEC);
myFile.print(':');
myFile.print(now.second(), DEC);
myFile.print(" ");
int analog_1 = analogRead(A0);
int analog_2 = analogRead(A1);
myFile.print("analog_1 = ");
myFile.print(analog_1);
myFile.print(", ");
myFile.print("analog_2 = ");
myFile.print(analog_2);
myFile.write("\n");
myFile.close();
} else {
Serial.print(F("SD Card: error on opening file "));
Serial.println(filename);
}
delay(2000);
}
Once you have completed a lengthy run, if you:
Remove the Micro SD Card from the Micro SD Card module
Place the Micro SD Card into an USB SD Card reader
Plug the USB SD Card reader into your PC
You will be able to view the files as follows: